What is C?
"C" is a foundational programming language created by Dennis Ritchie in 1972. It is known for its efficiency, portability, and broad use in system programming, including developing operating systems like UNIX.
Why Learn C?
- It is quite popular all across the world.
- As C has a comparable syntax to Java, Python, C++, and C#, learning them is easy.
- C is much faster than languages like Java and Python.
- It can be used in a variety of technologies and applications due to its adaptability.
History of the C Programming
In 1972, Ritchie invented the C programming language at AT&T's Bell Laboratories in the United States.

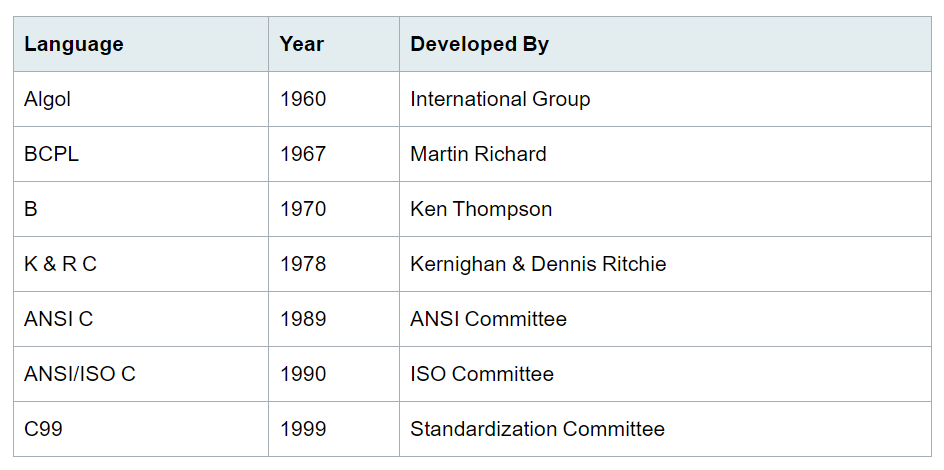
Features of C
- Simple and Efficient Syntax: C has a simple and efficient syntax, making code writing and comprehension easy.
- Portable: C programs are portable, meaning they can be used on a variety of platforms and devices without requiring major adjustments.
- Function-Rich Library: C provides a comprehensive library with built-in functions to improve development efficiency.
- Dynamic Memory Management: C supports dynamic memory allocation and deallocation during program execution, which improves resource utilization.
- Pointers: C allows for direct memory manipulation via pointers, resulting in efficient memory access and manipulation.
- Structured Language: C encourages code organization through functions and libraries, which promotes modularity and code reuse.
- Versatility in mid-level programming languages: C provides flexibility and control in program creation by acting as a high-level and low-level language.
- Quick compilation and execution: C programs are perfect for time-sensitive applications because they compile and execute quickly.
- Support for recursive function calls: C allows for recursive function calls, which makes it easier to solve problems elegantly and effectively.
- Extensible nature: C has an extensible nature, which allows it to smoothly absorb new features and functionalities, providing adaptation to changing programming requirements.
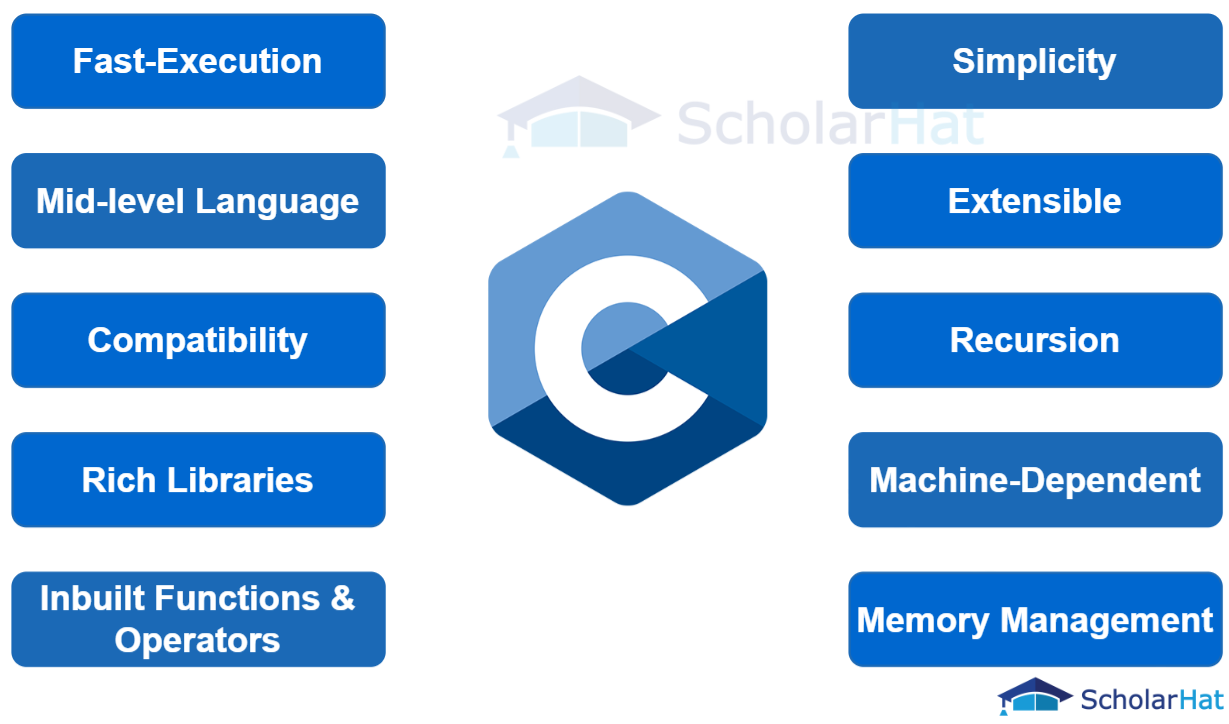
Uses of C Language
- Computational and graphical approaches.
- Applications with graphical user interfaces.
- Browsers for the Internet.
- Banking.
- Compilers.
- Distributed systems and cloud computing.
- Enterprise-wide software libraries.
System Requirements for Programming in C
To begin coding in C:
- Select a text editor (for example, Sublime Text or Notepad).
- Download a compiler such as Turbo C++.
- Alternatively, use an Integrated Development Environment (IDE), such as Eclipse or Visual Studio Code.
Applications of C
- Operating systems: C is commonly used to create operating systems like Unix, Linux, and Windows.
- Embedded systems: C is a popular language for creating embedded systems including microcontrollers, microprocessors, and other electronics.
- System software: C is used to create system software such as device drivers, compilers, and assemblers.
- Networking: C is commonly used to create networking programs such as web servers, network protocols, and drivers.
- Database systems: C is used to create database systems like Oracle, MySQL, and PostgreSQL.
- Gaming: C's ability to handle low-level hardware interactions makes it a popular choice for computer game development.
- Artificial Intelligence: C is used to create artificial intelligence and machine learning applications like neural networks and deep learning algorithms.
- Scientific applications: C is used to create scientific applications like simulation software and numerical analysis tools.
- Financial applications: C is used to create financial apps such as stock market analysis and trading platforms.
Structure of a C Program
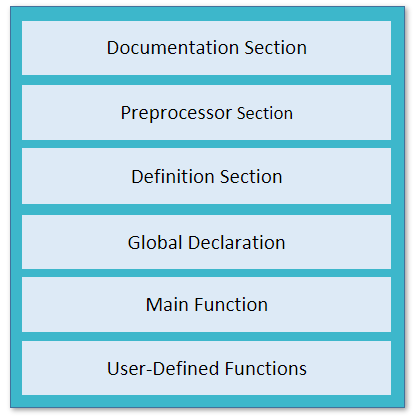
C Programme Components
Header Files Addition
In C programming, header files with the .h extension contain function definitions and macros that can be shared between source files. A preprocessor processes lines that begin with #, copying code from the header file into the program. Here are some of the C header files:
- stddef.h: Defines various important types and macros.
- stdint.h: This file defines the exact width integer types.
- stdio.h: Defines the main input and output functions.
- stdlib.h: Defines numeric conversion routines, a pseudo-random number generator, and memory allocation.
- string.h: Defines string handling functions.
- math.h: Defines common mathematical functions.
Declaring the Main Method
In C, the main() function is the program's entry point, and execution normally begins with the first line. The empty brackets indicate that there are no arguments, and the int preceding main indicates the return type, which indicates that the program has terminated.
Body of Main Method
In the C programming language, the body of a function refers to the statements that make it. It could be anything from manipulations to searching, sorting, and printing. A pair of curly brackets denotes the body of a function. Every function must begin and end with curly brackets.
Statement
Statements are the instructions sent to the compiler. In C, a statement is always finished with a semicolon.
Return Statement
In C, the return statement denotes the function's return value, which matches its return type. In main(), the return statement returns a value indicating the program's termination status. Usually, 0 indicates a successful result.