What is Buffer?
A buffer is used as temporary storage for data transit between source and destination, handling small data chunks efficiently. Buffers are specifically created for Node.js and deal with binary data, providing speed and lightweight functionality similar to integer arrays but without resizing capability.
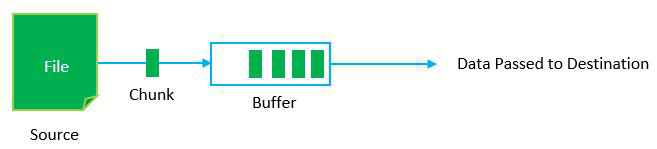
Creating buffers
Buffers can be generated using a variety of methods, including Buffer.from(), Buffer.alloc(), and directly allocating memory using Buffer.allocUnsafe().
Methods to Create Buffers
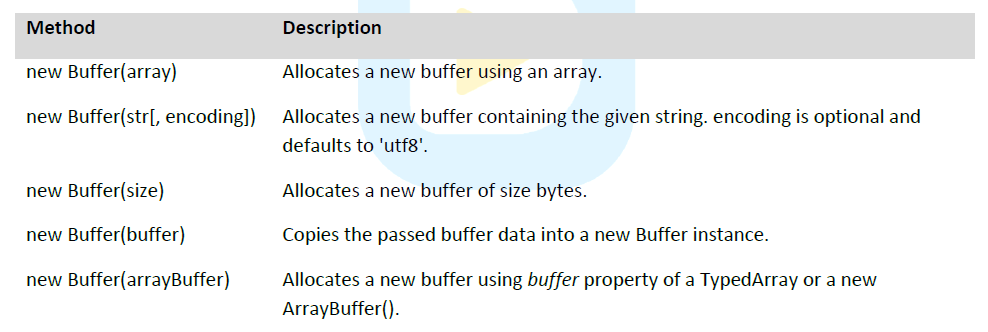
Working with Buffers
- Buffers can be manipulated with methods such as write(), read(), slice(), and copy().
- They are commonly used for binary data processing, such as reading from or writing to streams, file operations, network connections, etc.
Buffer Methods
- alloc: Creates a Buffer object with the provided length.
- allocUnsafe: Allocates a non-zero Buffer of the specified length.
- allocUnsafeSlow: Creates a non-zero, non-pooled Buffer of the supplied length.
- byteLength: Gets the number of bytes in a given object.
- compare: Compares two Buffers.
- concat: Combines an array of Buffer objects into a single Buffer object.
- copy: Copy a Buffer object's supplied number of bytes.
- entries: Returns an iterator of "index" and "byte" pairs from a Buffer object.
- equals: Compares two Buffer objects, returning true if they match and false otherwise.
- fill: Fills a Buffer object with the provided values.
- from: Generates a Buffer object from an object (string, array, buffer).
- includes: Determines whether the Buffer object has the provided value. Returns true if there is a match, and false otherwise.
- indexOf: Determines whether a Buffer object contains the provided value. Returns the first occurrence or -1.
- isBuffer: Determines if an object is a Buffer object.
- isEncoding: Determines whether the Buffer object supports the provided encoding.
- keys: An array of keys in a Buffer object.
- lastIndexOf: Determines whether the Buffer object has the provided value. Returns the first occurrence, starting from the end, or -1.
- length: Returns the length of a Buffer object in bytes.
- poolSize: Sets or returns the number of bytes allocated for pooling.
- readDoubleBE: Extracts a 64-bit double from a Buffer object and returns the result in big-endian.
- readDoubleLE: Retrieves a 64-bit double from a Buffer object and returns the result in little-endian.
- readFloatBE: Reads a 32-bit float from a Buffer and returns the result in big-endian.
- readFloatLE: Reads a 32-bit float from a Buffer object and returns the value in little-endian.
- readInt8: Reads an 8-bit integer from a Buffer object.
- readInt16BE: Extracts a 16-bit integer from a Buffer object and returns the result in big-endian.
- readInt16LE: Reads a 16-bit integer from a Buffer object and returns the value in little-endian.
- readInt32BE: Extracts a 32-bit integer from a Buffer object and returns the result in big-endian.
- readInt32LE: Reads a 32-bit integer from a Buffer object and returns the value in little-endian.
- readIntBE: Reads a specified number of bytes from the Buffer object and returns the result in big-endian.
- readIntLE: Reads the provided number of bytes from the Buffer object and returns the value in little-endian.
- readUInt8: Reads an unsigned 8-bit integer from the Buffer object.
- readUInt16BE: Extracts an unsigned 16-bit integer from a Buffer object and returns the result in big-endian.
- readUInt16LE: Reads an unsigned 16-bit integer from a Buffer object and returns the value in little-endian.
- readUInt32BE: Extracts an unsigned 32-bit integer from a Buffer object and returns the result in big-endian.
- readUInt32LE: Reads an unsigned 32-bit integer from a Buffer object and returns the value in little-endian.
- readUintBE: Reads the given number of bytes from a Buffer object and returns an unsigned integer.
- readUIntLE: Reads the given number of bytes from a Buffer object and returns an unsigned integer.
- slice: Splits a Buffer object into new Buffer objects that begin and stop at the specified places.
- swap16: Changes the byte order of a 16-bit Buffer object.
- swap32: Changes the byte order of a 32-bit Buffer object.
- swap64: Changes the byte order of a 64-bit Buffer object.
- toString: Returns the string representation of a Buffer object.
- toJSON: Returns the JSON representation of a Buffer object.
- values: Returns an array of Buffer values.
- write: Sends a specified string to a Buffer object.
- writeDoubleBE: Writes the provided bytes to a Buffer object in big-endian format. The bytes should be 64-bit doubles.
- writeDoubleLE: Writes the provided bytes to a Buffer object in little-endian format. The bytes should be 64-bit doubles.
- writeFloatBE: Writes the provided bytes to a Buffer object in big-endian format. The bytes should be 32-bit floats.
- writeFloatLE: Writes the provided bytes to a Buffer object in little-endian format. The bytes should be 32-bit floats.
- writeInt8: Transfers the supplied bytes to a Buffer. The bytes should be 8-bit integers.
- writeInt16BE: Writes the provided bytes to a Buffer object in big-endian format. The bytes should be 16-bit integers.
- writeInt16LE: Writes the provided bytes to a Buffer object in little-endian format. The bytes should be 16-bit integers.
- writeInt32BE: Writes the provided bytes to a Buffer object in big-endian format. The bytes should be 32-bit integers.
- writeInt32LE: Writes the provided bytes to a Buffer object in little-endian format. The bytes should be 32-bit integers.
- writeIntBE: Writes the supplied bytes to a Buffer object in big-endian format.
- writeIntLE: Writes the provided bytes to a Buffer object in little-endian format.
- writeUInt8: Writes the provided bytes to a Buffer object. The bytes should be 8-bit unsigned integers.
- writeUInt16BE: Writes the provided bytes to a Buffer object using big-endian. The bytes should be 16-bit unsigned integers.
- writeUInt16LE: Writes the provided bytes to a Buffer object with little endian. The bytes should be 16-bit unsigned integers.
- writeUInt32BE: Writes the provided bytes to a Buffer object using big-endian. The bytes should be 32-bit unsigned integers.
- writeUInt32LE: Writes the supplied bytes to a Buffer object in little-endian format. The bytes should be 32-bit unsigned integers.
- writeUIntBE: Writes the provided bytes to a Buffer object in big-endian format.
- writeUIntLE: Writes the provided bytes to a Buffer object with little endian.
What is Buffer encoding?
Buffers can be encoded and decoded using several character encodings, including UTF-8, ASCII, and Base64. When constructing a buffer from a string, you can specify the encoding with the Buffer.from(string, encoding) function.
Buffer and Streams
Node.js streams use buffers to read and write data chunks. They effectively process enormous amounts of data because they reduce memory allocation and copying.
Buffer Conversion
Buffer conversion in Node.js is the process of converting data between Buffer objects and other data types like strings or arrays.
Buffer Conversion to JavaScript Strings Using Encoding Methods
Buffers can be turned into JavaScript string objects using the following encoding method:
- buf.toString("utf8") - by the default encoding format
- buf.toString("ascii")
- buf.toString("base64")
- buf.toString("binary")
- buf.toString("hex")
Buffer performance considerations
Buffers allocate raw memory outside of the V8 heap, which allows them to handle binary data quickly and efficiently. However, improper utilization of buffers might result in memory leaks or performance concerns, particularly when dealing with huge amounts of data.
Considerations for Buffer Security
Buffers have the potential to disclose sensitive data if not handled securely, particularly when working with user input or other data sources. Avoid exposing buffer data directly to untrusted sources, and utilize proper validation and sanitization methods.