02
MayAngular Roadmap to become an Angular Developer
Overview of Angular Developer Roadmap
The learning path for prospective Angular developers is described in this roadmap. It begins with web development fundamentals and moves to more advanced Angular topics that will strengthen your angular skills such as RxJS and state management. It also includes topics such as testing, deployment, and key soft skills.
Introduction to Angular
Angular is a development platform based on TypeScript. As a platform, Angular provides a component-based architecture for developing scalable online applications. A collection of well-integrated libraries that provide a wide range of capabilities such as routing, form management, client-server communication, and others.
Who is an Angular Developer?
An Angular developer is a software engineer who focuses on using the Angular framework to construct dynamic and interactive web apps. They are skilled at designing and implementing scalable front-end solutions using TypeScript, HTML, CSS, and Angular-specific tools, including data binding, routing, and component-based architecture.
Roles and Responsibilities of Angular Developer
- Create self-contained, reusable, & testable modules and components.
- Ensure a clear dependency chain in terms of both application logic and file dependencies.
- Ensure great performance on mobile and desktop.
- Writing non-blocking code and using advanced techniques like multi-threading where necessary.
- Collaboration with the backend developer in the process of establishing the RESTful API.
- Communicate with external web services.
- Profile optimization (memory and performance).
- Experience with NodeJS and ReactJS, as well as back-end technologies like Node.js and Express, is advantageous.
- Strong skills with HTML, CSS3, and JavaScript.
- Familiarity with the latest ECMAScript requirements is also a significant advantage.
Angular JS Developer Roadmap
1.) Fundamentals of Web Development
>HTML
- Learn HTML5 tags, including <header>, <nav>, <section>, <article>, <footer>, etc.
- Understand semantic markup to increase accessibility and SEO.
- Explore the HTML5 form components and attributes.
- Learn about HTML5 multimedia elements, such as <video> and <audio>.
>CSS
- Understand CSS3 selectors, properties, and values.
- Learn how to use media queries to create responsive designs.
- Explore the Flexbox layout for more flexible and efficient designs.
- Learn how to use CSS Grid to create sophisticated layout structures.
>JavaScript
- Understand ES6+ syntax, which includes let/const for variable declaration, arrow functions for concise syntax & template literals for string interpolation.
- Learn destructuring to easily extract values from objects and arrays.
- Study ES6 modules for modular code organization.
- Master asynchronous programming by working with promises and async/await.
- Get familiar with DOM manipulation techniques so you can interact with HTML components dynamically.
🚀 Download Our Comprehensive Angular RoadMap PDF |
2.) Angular Development Tools
1. Angular CLI
- Learn how to generate projects, create components, and serve apps.
- Learn how to create & deploy Angular applications using the Angular CLI.
- Discover advanced features like code scaffolding, testing utilities, & Angular schematics.
2. Code Editors
- Learn to use popular code editors such as Visual Studio Code and IntelliJ Idea.
- Learn about key features like code navigation, syntax highlighting, & code completion.
- Learn how to install and maintain Angular development extensions, which provide additional tools and capabilities to increase productivity.
- Explore editor setups and customization options to personalize the environment to your needs and workflow.
3. Package Managers
- Learn about NPM (Node Package Manager) and Yarn for managing project dependencies.
- Learn how to use package.json to set up a new project, install packages, and manage dependencies.
- Understand the distinctions between NPM & Yarn, including their commands and workflows.
- Discover best practices for package management, including versioning, dependency resolution, & package-lock.json/yarn.lock management.
Typescript
- Understand the fundamentals of TypeScript, a JavaScript superset that includes static typing and other capabilities.
- Learn how TypeScript improves JavaScript by adding features like type annotations, interfaces, and classes.
- Learn about TypeScript's numerous data types, which include primitive types such as number, text, and boolean.
- Understand sophisticated data structures like arrays, tuples, and objects.
- Learn how to declare variables in TypeScript with the let and const keywords.
- Understand the fundamentals of TypeScript function declaration, parameters, and return types.
- Learn about optional and default parameter values.
- Explore arrow functions.
- Learn how to overload functions in TypeScript to provide various function signatures for the same function name.
- Learn how to create TypeScript classes for object-oriented programming.
- Understand class elements like properties and methods, as well as access modifiers like public, private, and protected.
- Understand constructors, inheritance, and method overriding in TypeScript classes.
- Understand how inheritance works in TypeScript classes, where child classes can inherit properties and methods from their parents.
- Understand the extends keyword and how to override inherited members in child classes.
- Learn how to use TypeScript interfaces to describe the structure of things.
- Understand the concept of generics in TypeScript to create reusable and type-safe code.
- Learn how to define generic functions, classes, and interfaces with type parameters.
- Learn how to divide TypeScript code into modules for better code organization and reuse.
- Understand the export and import keywords when exporting and importing modules.
- Explore the many module formats supported by TypeScript, including CommonJS, AMD, and ES6 modules.
3.) CSS Frameworks
- Understand CSS frameworks' function in frontend development for Angular applications.
- Learn how to integrate Bootstrap with Angular using ng-bootstrap or manual integration.
- Understand Bootstrap's grid system, typography, and components for responsive layouts.
- Learn how to use Angular Material and implement Material Design principles.
- Understand its various components, such as buttons, cards, menus, and dialogues.
- Discover Tailwind CSS, a utility-first CSS framework for Angular.
- Be familiar with alternatives such as Foundation, Bulma, and Semantic UI.
4.) Version Control System
Git
- Learn about Git and its use in Angular development collaboration.
- Learn the Git commands for repository management.
- Study techniques like feature branching for collaboration.
Github
- Learn how to use GitHub to host Angular repositories.
- Understand GitHub's collaborative features.
- Check out GitHub's best practices for managing Angular projects.
5.) Angular Core Concepts
> Components and Modules
- Learn how to structure Angular apps with components, modules, and templates.
- Learn about component inheritance to reuse functionality across multiple components.
- Study nested components for developing sophisticated UI architectures.
- Explore content projection for dynamic component composition.
- Learn ViewChild to access child components and elements.
- Understand the lifecycle hooks that manage component initialization, rendering, and destruction.
>Data Binding
- Learn property binding to dynamically update HTML components.
- Understand how to use event binding to handle user interactions.
- Study two-way binding for synchronizing data between components and views
>Directives
- Use built-in directives such as ngIf, ngFor, and ngSwitch for dynamic rendering.
- Learn how to create custom directives that enhance HTML capabilities.
> Services and Dependency Injection:
- Learn about services and dependency injection in Angular, which enable components to share data and functionality.
- Learn about dependency injection and how to provide instances of services to components.
> Change Detection:
- Learn about Angular's change detection method for updating the UI.
- Understand ways to improve change detection performance.
6.) Routing in Angular
- Understand how the Angular Router manages navigation in Angular apps.
- Learn how to configure routes and their associated components.
- Explore route guards for controlling access to routes based on specific conditions.
- Learn how to use lazy loading to load modules and components asynchronously, which improves performance.
7.) Forms
Forms Control & States
- Learn about Forms Control and States in Angular.
- Learn about the immaculate, dirty, touched, and undisturbed states.
- Explore form validation and error-handling techniques.
Template-Driven Forms
- Learn the fundamentals of template-driven forms to manage form data.
- Learn how to use the ngModel directive for basic form handling and validation.
- Discover template-driven form capabilities such as ngForm, ngModelGroup, and ngModelOptions.
Model-Driven Forms
- Learn about Model-Driven Forms (Reactive Forms), including reactive form controls, groups, and arrays to handle complicated forms effectively.
- Understand the FormBuilder service, which allows you to create form controls programmatically.
- Explore reactive form features like FormControl, FormGroup, and FormArray.
8.) HTTP Client and RxJS
Angular HTTP Client
- Learn how to make HTTP requests in Angular using the built-in HttpClient module.
- Learn how to handle responses and errors using HttpClient.
- Study features such as interceptors for global request/response management and error detection.
RxJS Fundamentals
- Learn the fundamentals of RxJS to manage asynchronous data streams in Angular apps.
- Learn about observables and observers, which are the foundation of RxJS.
- Explore operators such as map, filter, and mergeMap for transforming and merging observables.
- Understand the subjects for multicasting and exchanging data streams with multiple subscribers.
9.) Advanced Angular
Angular’s Change Detection Mechanism
- Learn about Angular's built-in change detection method to update the user interface.
- Learn about Zones and how Angular uses them for change detection.
- Explore ways for improving change detection performance, including the OnPush change detection strategy.
State Management Principles and Libraries
- Discover the concepts of state management in Angular applications.
- Learn about the significance of managing application state in complicated systems.
- Discover libraries like NgRx and Akita for Angular state management paradigms such as Redux.
- Understand the fundamental ideas of NgRx and Akita, such as actions, reducers, selectors, and effects.
10.) API & Security
API Integration
- Understand the principles of using APIs in Angular applications.
- Learn about RESTful services and how to use them with HttpClient.
- Learn about HTTP methods (GET, POST, PUT, DELETE) and URL parameters.
- Discover how to handle errors, authenticate, and authorize while working with APIs.
Security
- Understand the importance of security in Angular applications.
- Learn prevalent security threats and vulnerabilities in web apps.
- Explore the best practices for protecting Angular applications, such as input validation, authentication, and authorization.
- Study Angular-specific security features and libraries for building safe authentication and authorization systems.
11.) Testing
Testing Fundamentals
- Acknowledge the necessity of testing in Angular development.
- Learn about the many types of testing, including unit tests, integration tests, and end-to-end tests.
- Understand testing frameworks and technologies typically used in Angular applications, such as Jasmine and Karma.
Unit Testing
- Learn to create unit tests for Angular components, services, and pipes.
- Understand the testing features provided by Angular's TestBed and ComponentFixture.
- Explore methods for mimicking dependencies and isolating parts of code for testing.
Integration Testing
- Learn how to create integration tests that test interactions across several components.
- Discover methods for testing Angular directives, templates, and forms.
- Learn the best approaches for organizing and structuring integration tests in Angular applications.
End-to-end Testing
- Learn how to create end-to-end tests for Angular applications with tools such as Protractor.
- Understand how Selenium WebDriver and Jasmine fit into end-to-end testing scenarios.
- Study strategies for creating and conducting end-to-end tests that imitate user interactions and scenarios.
12.) Build & Deployment
Building Angular Application
- Understand how to build Angular applications for production.
- Learn about the Angular CLI commands for creating applications with various setups.
- Consider options for optimizing builds, such as Ahead-of-Time (AOT) compilation and tree shaking.
Environment Configurations
- Learn how to handle environment-specific configurations in Angular applications.
- Understand the environment files generated by the Angular CLI and how to utilize them.
- Study strategies for managing environment variables and secrets in Angular applications.
Deployment Strategies
- Understand the various deployment techniques for Angular applications, such as regular server hosting and serverless deployment.
- Discover hosting platforms such as Firebase, Netlify, and AWS S3 for delivering Angular applications.
- Consider CI/CD (Continuous Integration/Continuous Deployment) pipelines to automate the deployment process.
13.) Performance Optimization in Angular
Understanding Performance Optimization
- Understand the significance of performance optimization in Angular applications.
- Discover what aspects influence performance, including rendering, network queries, and JavaScript execution.
- Learn how to measure and analyze performance with tools such as Chrome DevTools and Lighthouse.
Lazy Loading
- Learn about lazy loading modules and components in Angular to reduce initial page load time.
- Understand how lazy loading minimizes the size of initial bundles by loading modules as needed.
- Explore methods for implementing lazy loading in Angular applications via loadChildren in route settings.
Change Detection Strategies
- Learn about Angular change detection mechanisms and how they impact performance.
- Understand the default change detection strategy's limits in large applications.
- Study approaches for improving change detection performance, such as using the OnPush change detection strategy and immutable data structures.
Optimizing Network Request
- Understand how network requests affect application performance.
- Discover ways for optimizing network requests, such as HTTP caching and prefetching.
- Consider ways to reduce the number and size of network requests, such as code separation and bundle optimization.
Soft Skills
Problem-solving
- Break down complex activities into smaller, more manageable portions.
- Use debugging tools efficiently to detect and address problems.
- Practice coding problems and debugging activities regularly.
Communication
- Participate in Angular-focused online communities, forums, and social media groups.
- Contribute to open-source projects or work with colleagues on initiatives.
- Practice expressing complex concepts in simple ways to non-technical audiences.
Learning Mindset
- Accept the learning process and be willing to make mistakes.
- Seek input from peers, mentors, or online groups to continually improve.
- Keep up with the newest Angular releases, best practices, and industry trends.
Time Management
- Set precise objectives and timeframes for your learning journey.
- Prioritize jobs efficiently to maximize your learning time.
- Take breaks and keep a healthy work-life balance to avoid burnout.
Adaptability
- Be open to learning about new web development technologies and processes.
- Adapt to developments in the Angular environment and develop your skills accordingly.
- Learn from mistakes and be open to changing your strategy as needed.
Teamwork
- Collaborate with people on projects, whether through pair programming or by contributing to open-source.
- To strengthen teamwork, practice providing and receiving positive comments.
- When working with others, respect different points of view and strive for consensus.
Real-world Projects
- Job Portal: Create a job portal application where users can search and apply for job postings, while companies can post job vacancies, manage applications, and connect with candidates.
- Online Learning Platform: Create an online learning platform like Udemy or Coursera where users may enroll in courses, watch video lectures, take quizzes, and monitor their progress.
- Expense Tracker: Create an expense-tracking app to assist consumers manage their budgets. Include functionality such as expense recording, transaction categorization, budget management, and report generation.
- Weather Forecast App: Create a weather forecast application that shows current weather conditions, hourly forecasts, and extended forecasts for various areas around the world. Integrate a weather API to get real-time data.
- Travel Planner: Create a trip planner app that allows users to browse for destinations, plan itineraries, book flights, and hotels, and share their travel experiences with others.
Summary
In this complete Angular developer roadmap, students begin with fundamental web programming abilities, then graduate to Angular's core principles, advanced subjects such as state management and testing, and finally deployment methodologies. Along with technical proficiency, emphasis is placed on soft skills such as problem-solving, communication, and adaptation, resulting in well-rounded developers capable of succeeding in real-world projects.
Download this PDF Now - Angular Roadmap PDF By Scholarhat |
Take our Angular skill challenge to evaluate yourself!
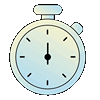
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.