14
JunWhat's new in ASP.NET Core 8 - Features, Optimizations, and Benefits
What's new in ASP.NET Core 8? An Overview
We know that recently, the successor of .NET 7, .NET 8 has been released. It offers enhanced performance, improved diagnostic and observability, expanded cross-platform support, advanced tooling and integration, long-term support (LTS), and much more. ASP.NET Core is the open-source version of ASP.NET. In this article, we will see some of the significant updates and optimizations done in ASP.NET Core in .NET 8.
If you’re looking to build your skills in this ecosystem, you can explore dot net training online free with certification to get started without any cost.
Blazor
1. Full-stack web UI
Blazor is a full-stack web UI framework for developing apps that render content at either the component or page level with:
- Static Server rendering (also called static server-side rendering, static SSR) to generate static HTML on the server.
- Interactive Server rendering (also called interactive server-side rendering, interactive SSR) generates interactive components with prerendering on the server.
2. New article on class libraries with static server-side rendering (static SSR)
Microsoft has added a new article that discusses component library authorship in Razor class libraries (RCLs) with static server-side rendering (static SSR).
3. New Article on HTTP Caching Issues
A new article discussing HTTP caching issues that can occur when upgrading Blazor apps across major versions and how to address HTTP caching issues has been added in this update.
4. New Blazor Web App template
The new template provides a single starting point for using Blazor components to build any style of web UI. The template combines the strengths of the existing Blazor Server and Blazor WebAssembly hosting models.
5. New JS initializers for Blazor Web Apps
For Blazor Web Apps, a new set of JS initializers are used: beforeWebStart, afterWebStarted, beforeServerStart, afterServerStarted, beforeWebAssemblyStart, and afterWebAssemblyStarted.
6. Split of prerendering and integration guidance
This time above subjects have been separated into the following new articles, which have been updated for .NET 8:
- Prerender ASP.NET Core Razor components
- Integrate ASP.NET Core Razor components into ASP.NET Core apps
7. Persist component state in a Blazor Web App
You can persist and read component state in a Blazor Web App using the existing PersistentComponentState service. This is useful for persisting component state during prerendering.
8. Render Razor components outside of ASP.NET Core
One can now render Razor components outside the context of an HTTP request. You can render Razor components as HTML directly to a string or stream independently of the ASP.NET Core hosting environment.
9. Root-level cascading values
Root-level cascading values can be registered for the entire component hierarchy. Named cascading values and subscriptions for update notifications are supported.
10. Content Security Policy (CSP) compatibility
Blazor WebAssembly no longer requires enabling the unsafe-eval script source when specifying a Content Security Policy (CSP).
SignalR
1. New approach to set the server timeout and Keep-Alive interval
- Prior approach for JavaScript clients
The following example shows the assignment of values that are double the default values in ASP.NET Core 7.0 or earlier:
var connection = new signalR.HubConnectionBuilder() .withUrl("/chatHub") .build(); connection.serverTimeoutInMilliseconds = 60000; connection.keepAliveIntervalInMilliseconds = 30000;
- New approach for JavaScript clients
The following example shows the new approach for assigning values the default values in ASP.NET Core 8.0 or later:
var connection = new signalR.HubConnectionBuilder() .withUrl("/chatHub") .withServerTimeoutInMilliseconds(60000) .withKeepAliveIntervalInMilliseconds(30000) .build();
- New approach for JavaScript clients
- Prior approach for the JavaScript client of a Blazor Server app
The following example shows the assignment of values that are double the default values in ASP.NET Core 7.0 or earlier:
Blazor.start({ configureSignalR: function (builder) { let c = builder.build(); c.serverTimeoutInMilliseconds = 60000; c.keepAliveIntervalInMilliseconds = 30000; builder.build = () => { return c; }; } });
- New approach for the JavaScript client of server-side Blazor app
The following example shows the new approach for assigning values that are double the default values in ASP.NET Core 8.0 or later for Blazor Web Apps and Blazor Server.
- Blazor Web App:
Blazor.start({ circuit: { configureSignalR: function (builder) { builder.withServerTimeout(60000).withKeepAliveInterval(30000); } } });
- Blazor Server:
Blazor.start({ configureSignalR: function (builder) { builder.withServerTimeout(60000).withKeepAliveInterval(30000); } });
- Blazor Web App:
- New approach for the JavaScript client of server-side Blazor app
- Prior approach for .NET clients
The following example shows the assignment of values that are double the default values in ASP.NET Core 7.0 or earlier:
var builder = new HubConnectionBuilder() .WithUrl(Navigation.ToAbsoluteUri("/chathub")) .Build(); builder.ServerTimeout = TimeSpan.FromSeconds(60); builder.KeepAliveInterval = TimeSpan.FromSeconds(30); builder.On("ReceiveMessage", (user, message) => ... await builder.StartAsync();
- New approach for .NET clients
The following example shows the new approach for the assignment of values that are double the default values in ASP.NET Core 8.0 or later:
var builder = new HubConnectionBuilder() .WithUrl(Navigation.ToAbsoluteUri("/chathub")) .WithServerTimeout(TimeSpan.FromSeconds(60)) .WithKeepAliveInterval(TimeSpan.FromSeconds(30)) .Build(); builder.On("ReceiveMessage", (user, message) => ... await builder.StartAsync();
- New approach for .NET clients
2. SignalR stateful reconnect
SignalR stateful reconnect reduces the perceived downtime of clients that have a temporary disconnect in their network connection, such as when switching network connections or a short temporary loss in access.
Opt-in to stateful reconnect at both the server hub endpoint and the client:
- Update the server hub endpoint configuration to enable the AllowStatefulReconnects option:
app.MapHub("/hubName", options => { options.AllowStatefulReconnects = true; });
Optionally, the maximum buffer size in bytes allowed by the server can be set globally or for a specific hub with the StatefulReconnectBufferSize option:
The StatefulReconnectBufferSize option set globally:
builder.AddSignalR(o => o.StatefulReconnectBufferSize = 1000);
The StatefulReconnectBufferSize option set for a specific hub:
builder.AddSignalR().AddHubOptions(o => o.StatefulReconnectBufferSize = 1000);
The StatefulReconnectBufferSize option is optional with a default of 100,000 bytes.
- Update JavaScript or TypeScript client code to enable the withStatefulReconnect option:
const builder = new signalR.HubConnectionBuilder() .withUrl("/hubname") .withStatefulReconnect({ bufferSize: 1000 }); // Optional, defaults to 100,000 const connection = builder.build();
The bufferSize option is optional with a default of 100,000 bytes.
- Update .NET client code to enable the WithStatefulReconnect option:
var builder = new HubConnectionBuilder() .WithUrl("") .WithStatefulReconnect(); builder.Services.Configure(o => o.StatefulReconnectBufferSize = 1000); var hubConnection = builder.Build();
The StatefulReconnectBufferSize option is optional with a default of 100,000 bytes.
Minimal APIs
This section describes new features for minimal APIs.
User override culture
Starting in ASP.NET Core 8.0, the RequestLocalizationOptions.CultureInfoUseUserOverride property allows the application to decide whether or not to use non-default Windows settings for the CultureInfo DateTimeFormat and NumberFormat properties. This has no impact on Linux. This directly corresponds to UseUserOverride.
app.UseRequestLocalization(options =>
{
options.CultureInfoUseUserOverride = false;
});
1. Antiforgery with Minimal APIs
This release adds middleware for validating antiforgery tokens, which mitigate cross-site request forgery attacks. Call AddAntiforgery to register antiforgery services in DI. WebApplicationBuilder automatically adds the middleware when the antiforgery services have been registered in the DI container.
var builder = WebApplication.CreateBuilder();
builder.Services.AddAntiforgery();
var app = builder.Build();
app.UseAntiforgery();
app.MapGet("/", () => "Hello World!");
app.Run();
2. New IResettable interface in ObjectPool
Microsoft.Extensions.ObjectPool provides support for pooling object instances in memory. Apps can use an object pool if the values are expensive to allocate or initialize.
In this release, the object pool has become easier to use by adding the IResettable interface. Reusable types often need to be reset back to a default state between uses. IResettable types are automatically reset when returned to an object pool.
Native AOT
Apps that are published using AOT can have substantially better performance: smaller app size, less memory usage, and faster startup time.
1. New project template
The native AOT project template (short name webapiaot) creates a project with AOT publish enabled. For more information, see The Web API (native AOT) template.
2. New CreateEmptyBuilder method
There as come a new WebApplicationBuilder factory method for building small apps that only contain necessary features: WebApplication.CreateEmptyBuilder(WebApplicationOptions options). This WebApplicationBuilder is created with no built-in behavior. The app it builds contains only the services and middleware that are explicitly configured.
Here’s an example of using this API to create a small web application:
var builder = WebApplication.CreateEmptyBuilder(new WebApplicationOptions());
builder.WebHost.UseKestrelCore();
var app = builder.Build();
app.Use(async (context, next) =>
{
await context.Response.WriteAsync("Hello, World!");
await next(context);
});
Console.WriteLine("Running...");
app.Run();
Summary
Here, in the above blog post, we have provided you the information regarding some of the important updates done by Microsoft in the ASP.NET Core in its 8th version. If you want to get into more details, see this documentation: ASP.NET Core 8.0 Release Notes. For beginners interested in learning more, there are several net free course with certificate options available to kick-start your journey in modern web development.
FAQs
Take our Aspnet skill challenge to evaluate yourself!
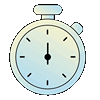
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.