Arithmetic Operators in C Programming
Arithmetic Operators in C
Arithmetic Operators are used in various expressions to perform functions such, as addition, subtraction, multiplication, division, and calculating the remainder. These Operators are essential, for managing data and performing calculations in C programs.
In this C Tutorial, we will see in-depth information about arithmetic operators including arithmetic operators in c programming with examples
What are C Arithmetic Operators?
- In C programming Arithmetic Operators play a role, in carrying out tasks within a program.
- These operators help define expressions and mathematical calculations using symbols to perform operations on operands.
- C offers a total of 9 operators enabling users to execute arithmetic functions, like addition subtraction, multiplication, and more.
Syntax
In C, arithmetic operators have a simple syntax. To compute a result, they operate in between operands, which are variables or constants. The basic syntax for employing arithmetic operators is as follows:
result = operand1 operator operand2;
Example
int result;
result = 5 + 3; // Using the addition operator
Types of Arithmetic Operators in C
- Binary operators.
- Unary operators.
These operators perform basic mathematical operations like addition, subtraction, multiplication, and division. We will categorize the arithmetic operators into two categories based on the number of operands and then look at their functionality.
Type | Operator | Name of Operator | Functionality |
Unary | ++ | Increment | Increases the value by 1 |
- - | Decrement | Decreases the value by 1 | |
+ | Unary plus | No change in the operand value | |
- | Unary minus | changes the negative number to the positive and vice-versa | |
Binary | + | Addition | Add two values |
- | Subtraction | Subtracts one value from the other | |
* | Multiplication | Multiplies two values | |
/ | Division | Divides one by the other value | |
% | Modulus | Finds the remainder after division |
Flowchart of Arithmetic Operators in C
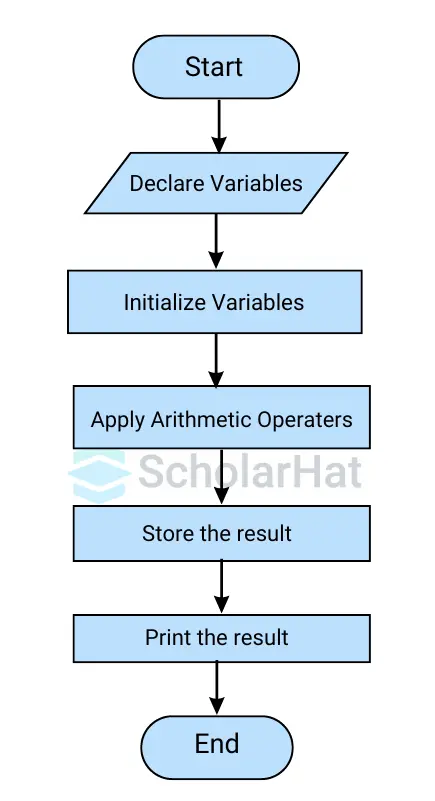
1. Binary Arithmetic Operators
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
- Modulus (%)
Example
#include <stdio.h>
int main()
{
int x = 25, y = 5, operation;
printf("if x is %d and y is %d\n", x, y);
operation = x + y; // addition Operation
printf("Addition of x + y is %d\n", operation);
operation = x - y; // subtraction
printf("Substraction of x - y is %d\n", operation);
operation = x * y; // multiplication
printf("Multiplication of x * y is %d\n", operation);
operation = x / y; // division
printf("Division of x / y is %d\n", operation);
operation = x % y; // modulus
printf("Modulas of x %% y is %d\n", operation);
return 0;
}
Output
if x is 25 and y is 5
Addition of x + y is 30
Substraction of x - y is 20
Multiplication of x * y is 125
Division of x / y is 5
Modulas of x % y is 0
2. Unary Arithmetic Operators
- + (Unary plus)
- - (Unary minus)
- ++ (Increment)
- -- (Decrement)
Example
#include <stdio.h>
int main()
{
int x = 91, y = 99, Operation;
printf("Post Increment and Decrement\n");
Operation = x++;
printf("x is %d and result is %d\n", x,
Operation); // x becomes 91 now
// post-decrement example:
// Operation is assigned 91 only, x is not updated yet
Operation = x--;
printf("x is %d and result is %d\n", x,
Operation);
printf("\nPre Increment and Decrement\n");
Operation = ++x;
printf("x is %d and result is %d\n", x, Operation);
Operation = --x;
// a and Operation have same values = 10
printf("x is %d and result is %d\n", x, Operation);
return 0;
}
Output
Post Increment and Decrement
x is 92 and result is 91
x is 91 and result is 92
Pre Increment and Decrement
x is 92 and result is 92
x is 91 and result is 91
Multiple Operators in a Single Expression
int result = a + b * c - d / e;
Examples of C Arithmetic Operators
#include <stdio.h>
int main()
{
//unary operators
int x = 5, y=6, z=7, w=8;
printf("%d\n", ++x); //increments the value of x
printf("%d\n", --y); //decrements the value of x
printf("%d\n", +z); // unary +
printf("%d\n", -w); // unary - on a positive number
printf("%d\n",-(-w)); // unary - on a negative number
// binary operators
int a = 10, b = 3;
int sum = a + b;
int difference = a - b;
int product = a * b;
int quotient = a / b;
int remainder = a % b;
printf("Sum: %d\n", sum);
printf("Difference: %d\n", difference);
printf("Product: %d\n", product);
printf("Quotient: %d\n", quotient);
printf("Remainder: %d\n", remainder);
return 0;
}
In the above code in the C Compiler, we have performed all the arithmetic operations on unary as well as binary operands.
Output
6
5
7
-8
8
Sum: 13
Difference: 7
Product: 30
Quotient: 3
Remainder: 1
Arithmetic Operations with Char Data Type In C
#include <stdio.h>
int main() {
char a = 'A';
char b = 2;
char result = a + b;
printf("Result: %c\n", result); // Outputs 'C'
return 0;
}
Advantages of Arithmetic Operators
- Ease; They offer a method to carry out math computations.
- Speed; Math symbols are finely tuned for quick execution.
- Versatility; They work well with data formats such, as numbers, decimal numbers, and letters.
Conclusion:
Understanding the syntax, types, and examples of arithmetic operators, in C is crucial, for performing mathematical operations and manipulating data. This knowledge enables you to create optimized C programs. Also, Master your coding base in C programming to the next level by pursuing our C Programming Course.
Similar Articles of C |
C Programming Assignment Operators |
Operators in C: Types of Operators |
Bitwise Operators in C: AND, OR, XOR, Shift & Complement |
Interview Article of C |
Top 50 Most Asked C Interview Questions and Answers |
FAQs
Q1. What are operators in C?
Q2. What are the 7 operator in C?
- Arithmetic Operators.
- Relational Operators.
- Logical Operators.
- Bitwise Operators.
- Assignment Operators.
- Conditional Operator.