14
JunTop 50 Mostly Asked C Interview Questions and Answers
C Interview Questions and Answers: An Overview
Are you preparing for a C interview? Feeling excited but quite nervous at the same time? fear not..! This comprehensive guide is here to guide you to demonstrate a solid grasp of C concepts, help you to grow your problem-solving skills, and also make you feel confident to ace your interview.
Why are C Interview Questions Important?
In the Programming world, C remains a foundational language for systems programming, embedded systems, and performance-critical applications. By understanding its complexities, you will conquer half of the programming languages that exist. Interviewers often use C-specific terms to analyze the understanding of your C knowledge such as
- Memory management: C gives you direct control over memory, which can be powerful and challenging. Be prepared to explain concepts like pointers, dynamic allocation, and common memory-related pitfalls.
- Problem-solving and algorithmic thinking: Interviewers want to see how you approach problems analytically, break them down into manageable steps, and write efficient C code to achieve the desired outcome.
- Best practices and coding style: Following good practices demonstrates your professionalism and attention to detail. Be familiar with common C conventions, coding guidelines, and readability tips.
C Interview Questions: A Roadmap to Success
Now that you have a solid understanding of why C interview questions and how to prepare for them, let's see some advanced terms you might encounter in an interview. This guide covers various categories:
- Basics and Syntax: Test your understanding of fundamental C concepts, data types, operators, and control flow.
- Memory Management: Master pointers, dynamic allocation, and memory-related pitfalls.
- Data Structures and Algorithms: Explore common C data structures (arrays, linked lists, stacks, queues, trees, etc.) and demonstrate your ability to implement algorithms using them.
- Advanced Concepts: Get comfortable with preprocessor directives, bitwise operations, unions, structures, and advanced function pointers.
- Problem Solving and Coding: Apply your knowledge to solve real-world programming challenges using efficient and well-structured C code.
Keep in mind for a good communicative interview Sometimes be in the interviewer's shoes too, Don't just answer, ask! Show your genuine interest by crafting insightful questions about the company, role, and team.
Explore the depth of C programming with our comprehensive guide to 50 C Interview Questions and Answers. Whether you're a beginner or an experienced developer, this resource provides valuable insights, helping you master essential concepts and excel in C-related interviews.
C Interview Questions and Answers for Freshers
1. Why is C called a mid-level programming language?
C possesses features of both lower-level and higher-level, or assembly-level, languages. C is hence frequently referred to be a middle-level language. One may write both a menu-driven consumer billing system and an operating system in C.
2. What are the features of the C language?
- Middle-level language: Provides low-level memory access and high-level abstractions, balancing efficiency and ease of use.
- Portability: Code written in C can run on different platforms with minimal modifications.
- Efficiency: Offers efficient memory management and execution speed.
- Roch Standard Library: Provides a comprehensive set of functions for various tasks.
- Procedural Language: Follows a structured, step-by-step approach to solving problems.
3. What is a token?
Tokens are the discrete components of a program. There are 6 tokens in C language, those are-
- Identifiers
- Keywords
- Constants
- Operators
- Special Characters
- Strings
4. What is the use of printf() and scanf() functions? Also, explain format specifiers?
Printf()- To print the output to the screen, use print().
Scanf()- To read formatted data from the keyboard, use scanf().
The following are a few datatype format specifiers that can be used for scanning and printing:
- %d- It's a format specifier for datatypes that prints and scans integer values.
- %s- This datatype format specifier is used to print and scan strings in the program.
- %c- It is a format specifier for a datatype that is used to display and scan character values.
- %f- The float value may be seen and scanned using the datatype format specifier %f.
5. What's the value of the expression 5["zyzuv"]?
The answer is "f". In C-style languages, the expression 5["zyzuv"] is equivalent to "zyzuv"[5], which accesses the 6th character of the string "zyzuv". Therefore, the value of the expression is "v".
6. What are basic data types supported in the C Programming Language?
In C programming, basic data types include:
- Primitive Data type- There are two other categories of primitive data types: integer and floating.
- User-Defined data types- The user defines these data types to improve program readability.
- Derived data types- Data types that come from pre-built or basic data types.
7. What is a built-in function in C?
The built-in C functions scanf(), printf(), strcpy, strlwr, strcmp, strlen, strcat, and many more are used often.
The term "built-function" refers to library functions that the system provides to developers to help them with specific frequently performed predefined tasks, hence simplifying their lives. For instance, printf() in C is used when you need to print your program's output to the terminal.
8. What do you mean by the scope of the variable?
In programming languages, scope refers to the block or region where a specified variable will live; if the variable extends outside of that block or region, it will be immediately destroyed. Every variable has a certain range of use. To put it simply, a variable's scope is determined by how long it remains in the program. Three different places can define the variable. They are as follows:
- Local Variables: Located within a certain block or function
- Global Variables: Among all the functions that the program does globally.
- Formal parameters:These are limited to in-function parameters.
9. What is a Preprocessor?
A software application known as a preprocessor is used to handle source files before submitting them to be built. The preprocessor allows for the inclusion of header files, macro expansions, conditional compilation, and line control.
10. What are preprocessor directives in C?
Preprocessor directives in C are built-in predefined functions or macros that are performed before program execution and serve as instructions to the compiler. The process of creating and running a C program involves several phases. Macros, File Inclusion, Conditional Compilation, and Other directives like #undef, #pragma, etc. are the main categories of Preprocessor Directives.
11. What is the use of static variables in C?
In the C programming language, data values are preserved across function calls even after they have exited their scope thanks to the usage of static variables. Static variables don't require initialization each time they are used in a program; their values are preserved inside their scope. Without initialization, the initial value of a static variable is set to 0.
// C program to print initial
// value of static variable
#include <stdio.h>
int main()
{
static int var;
int x;
printf("Initial value of static variable %d\n", var);
printf("Initial value of variable without static %d",
x);
return 0;
}
12. What is the difference between malloc() and calloc() in the C programming language?
The library methods malloc() and calloc() are used to allocate dynamic memory. The memory that is allocated from the heap section while the program is running is known as dynamic memory. The header file "stdlib.h" is what makes dynamic memory allocation in the C programming language possible.
Parameter | Malloc() | Calloc() |
Definition | It is a function that generates a single, fixed-size memory block. | It is a function that allows a single variable to be assigned to several memory blocks. |
Number of arguments | One argument is all that is needed. | There must be two arguments. |
Speed | Calloc() is slower than the malloc() procedure. | Compared to malloc(), calloc() is slower. |
Efficiency | It is quite efficient with time. | It is not very time-efficient. |
Usage | It serves as a memory allocation indicator. | It serves as a signal for contiguous memory allocation. |
13. What is Recursion in C?
Recursion in C is a programming technique where a function calls itself directly or indirectly to solve a problem. In recursive functions, the function breaks down the problem into smaller subproblems and calls itself with modified arguments until a base case is reached, stopping the recursive calls.
Syntax of recursive function in the C Compiler
void do_recursion()
{
... .. ...
do_recursion();
... .. ...]
}
int main()
{
... .. ...
do_recursion();
... .. ...
}
|
14. What is the difference between the Local and Global Variables in C?
Local Variables | Global Variables |
Declared within a function or a block. | Variables declared outside of a function or block. |
Local Variables store a garbage value by default | Global Variables store the default value of zero |
After a block or function, the local variables' life is terminated. | The global variable remains valid till the execution of the program. |
If the programmer does not specify a variable, it is kept inside the stack. | The compiler determines where the global variable is stored. |
Parameter passing is necessary for other functions to access the local variables. | Passing parameters is not necessary. During the program, they are visible to everyone worldwide. |
15. What is a Pointer in C?
A pointer in C is a variable that holds the memory address of another variable. It allows direct manipulation of memory locations, enabling dynamic memory allocation, efficient array handling, and indirect access to data. Pointers are a fundamental feature used for tasks like data structures and dynamic memory management.
16. Define Loops in C language. What are the types of loops in C?
Loops in C are programming constructs that repeatedly execute a block of code as long as a specified condition is true. They enable efficient iteration through data, simplifying tasks like array manipulation or repetitive operations, ensuring code efficiency and maintainability. Common loop types in C include for, while, and do-while.
|
17. How can we create an Infinite loop in C?
Let's see how we create infinite loop in C
// C program for infinite loop
// using for, while, do-while
#include
// Driver code
int main()
{
for (;;) {
printf("Infinite-loop\n");
}
while (1) {
printf("Infinite-loop\n");
}
do {
printf("Infinite-loop\n");
} while (1);
return 0;
}
18. What is the Pointer to Pointer in C?
In C, a pointer to a pointer, also known as a double pointer, is a variable that holds the address of another pointer variable. It allows indirect access to a memory location, providing a way to modify pointers and dynamically allocate multi-dimensional arrays, enhancing flexibility in memory management and data structures.
int **p; // pointer to a pointer which is pointing to an integer
19. What is Typecasting in C?
Typecasting in C is the process of converting one data type into another. It allows a variable to be treated as a different type temporarily. For example, converting an integer to a float or a pointer from one data type to another is done using typecasting operators like (float) or (int*).
Syntax
(data_type)expression;
Example
int x;
for(x=97; x<=122; x++)
{
printf("%c", (char)x); /*Explicit casting from int to char*/
}
Explanation
The given C code in theC Online Compiler initializes an integer variable x with the value 97 and then uses a for loop to print the characters corresponding to ASCII values from 97 to 122. In the ASCII table, these values represent lowercase English letters from 'a' to 'z'.
Output
abcdefghijklmnopqrstuvwxyz
20. What is the Difference between Type Casting and Type Conversion?
Type Casting | Type Conversion |
With the use of a casting operator, a programmer transforms the data type into another data type. | A compiler transforms the data type into another data type. |
It may be used on incompatible as well as compatible forms of data. | Only suitable data types can be used with type conversion. |
A caste operator is required in type casting to cast the data type into another data type. | A casting operator is not required during type conversion. |
Type casting is more dependable and efficient. | Compared to type casting, type conversion is less dependable and efficient. |
Syntax: destination_data_type = (target_data_type) variable_to_be_converted; | Syntax: int a = 20; float b; b = a; // a = 20.0000 |
C Programming Interview Questions for Intermediates
21. What are header files in C and their uses?
In C programming, header files are files containing function declarations and macro definitions. They allow code reuse by providing function prototypes to other source files. Commonly used header files include <stdio.h> for input/output functions and <stdlib.h> for memory allocation functions.
Header files in C serve several purposes:
22. What is the difference between macro and functions?
A pre-processor command that designates a group of C statements is called a macro. The pre-processor directive defines a macro. Since macros are preprocessed, our program would not have been compiled until after all of the macros had been preprocessed. Functions are compiled rather than preprocessed, though.
Macro | Function |
Preprocessed macros are used. | The functions are compiled. |
Using a macro, the code length is enhanced. | Using a function does not affect code length. |
With a macro, execution speed is increased. | Using a function results in reduced execution speed. |
The macro name is replaced by the macro value before compilation. | Control is transferred during the function call. |
23. Specify different types of decision control statements in C
A program executes each sentence one at a time, starting at the top and working down. Depending on the circumstance, control statements are used to execute or move control from one area of the program to another. The different types of control statements are:
- Simple if statement
- if...else statement
- if else...if ladder
- nested if...else statement
- Switch statement.
24. How to convert a String to Numbers in C?
There are two primary techniques in C for converting strings to numbers: string streams and the stoi() and atoi() library functions.
sscanf()-
Instead of using normal input, it reads data from a string.atoi()-
This method returns an integer value when it receives a character array or a string literal as input.25. What are Reserved Keywords in C?
In a program, each keyword is designed to carry out a certain function. They cannot be used for reasons other than those for which they were designed since their meaning has already been established. The programming language C has 32 keywords supported. Reserved keywords include things like auto, else, if, long, int, switch, typedef, and so on.
26. What is a Structure in C?
In C, a structure is a user-defined data type that allows you to group variables of different data types under a single name. It is used to represent a record containing different fields, enabling you to store and manipulate related data more efficiently. Example// C Program to show the
// use of structure
#include
#include
// Structure student declared
struct student {
char name[20];
int roll_no;
char address[50];
char branch[50];
};
// Driver code
int main()
{
struct student obj;
strcpy(obj.name, "Urmi_Bose");
obj.roll_no = 19;
strcpy(obj.address, "Kolkata");
strcpy(obj.branch, "Computer Science And Engineering");
// Accessing members of student obj
printf("Name: %s\n", obj.name);
printf("Roll_No: %d \n", obj.roll_no);
printf("Address: %s\n", obj.address);
printf("Branch: %s", obj.branch);
return 0;
}
Explanation
The given C program in C Editor defines a structure student with member name, roll_no, address, and branch. It then creates an object of this structure, initializes its members, and prints the values of these membersOutput
Name: Urmi_Bose
Roll_No: 19
Address: Kolkata
Branch: Computer Science And Engineering
27. What is Union in C?
In C, a union is a user-defined data type that allows different data types to be stored in the same memory location. Unlike structures, unions use the same memory space for all members, allowing only one member to hold a value at any given time. Unions are often used to save memory when different data types need to share the same memory location.
Syntax
union name_of_union
{
data_type name;
data_type name;
};
28. What is an r-value and l-value?
When an object has an address or a unique position in memory, it is referred to as an "l-value." Either the left or right side of the assignment operator(=) will display a "l-value." A data value kept in memory at a specific location is called a "r-value." An object without a known location in memory is referred to as an "r-value" (i.e. without an address). An expression that cannot have a value assigned to it is known as a "r-value," and it can only appear on the right side of an assignment operator (=).Example
int val = 20;Here,
val is the ‘l-value’, and 20 is the ‘r-value’.
29. What is the Call by value in C?
In C, call by value is a method of passing arguments to functions. When a function is called with parameters, the values of the parameters are copied into the function's parameters. Any modifications made to the parameters inside the function do not affect the original values outside the function.30. What is the Call by reference in C?
Call by reference in C involves passing the memory address of a variable to a function, allowing the function to modify the actual value at that address. Changes made to the parameter inside the function are reflected outside the function, as the function operates on the original data in memory.
|
31. What is the difference between Call by Value and Call by Reference in C?
Certainly, here are the differences between call by value and call by reference in C:
Call by Value | Call by Reference |
Parameters are passed by value, meaning the actual values are passed to the function. | Parameters are passed by reference, meaning the memory address (reference) of the actual variable is passed. |
The function receives a copy of the argument's value, and any modifications made inside the function do not affect the original variable. | The function receives the memory address of the argument, allowing modifications inside the function to directly affect the original variable. |
Suitable for basic data types like int, float, and char, where changes made inside the function don't reflect outside the function scope. | Suitable for complex data structures like arrays and structs, where changes made inside the function reflect outside the function scope. |
Efficient in terms of memory, as only values are passed. | More memory-intensive, as it involves passing memory addresses. |
32. What are the Enumerations in C?
In C, enumerations (enums) are user-defined data types that consist of a set of named integer constants. Enums provide a way to create symbolic names (enumerators) for integral values, making code more readable and understandable. Enums are often used to define sets of related integer values with meaningful names.
Syntax
enum enumeration_name{constant1, constant2, ... };
Example
include
// Enum declaration
enum Days {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY
};
int main() {
// Enum variable declaration
enum Days today;
// Assigning a value to the enum variable
today = WEDNESDAY;
// Printing the value of the enum variable
printf("Today is: ");
// Switch case to determine the day
switch (today) {
case SUNDAY:
printf("Sunday");
break;
case MONDAY:
printf("Monday");
break;
case TUESDAY:
printf("Tuesday");
break;
case WEDNESDAY:
printf("Wednesday");
break;
case THURSDAY:
printf("Thursday");
break;
case FRIDAY:
printf("Friday");
break;
case SATURDAY:
printf("Saturday");
break;
default:
printf("Invalid day");
break;
}
return 0;
}
Explanation
In this code in the C Online Editor, an enumeration Days is defined with the days of the week. The program sets the today variable to WEDNESDAY and prints the corresponding day using a switch statement.
Output
Today is: Wednesday
33. Write a C program to print the Fibonacci series using recursion and without using recursion.
// C program to print Fibonacci Series
// with recursion and without recursion
#include
// Recursive Function to print
// Fibonacci Series
void Fibonacci(int num, int first, int second, int third)
{
if (num > 0) {
third = first + second;
first = second;
second = third;
printf("%d ", third);
// Recursive call for it's
// n-1 value
Fibonacci(num - 1, first, second, third)
}
}
// Driver code
int main()
{
int num;
printf("Please Enter number of Elements: ");
scanf("%d", &num);
printf(
"Fibonacci Series with the help of Recursion:\n");
printf("%d %d ", 0, 1);
// we are passing n-2 because we have
// already printed 2 numbers i.e, 0 and 1
Fibonacci(num - 2, 0, 1, 0);
printf("\nFibonacci Series without Using Recursion:\n");
int first = 0, second = 1, third = 0;
printf("%d %d ", 0, 1);
// Loop will start from 2 because we have
// already printed 0 and 1
for (int i = 2; i < num; i++) {
third = first + second;
printf("%d ", third);
first = second;
second = third;
}
return 0;
}
Output
Please Enter number of Elements: 5
Fibonacci Series with the help of Recursion:
0 1 1 2 3
Fibonacci Series without Using Recursion:
0 1 1 2 3
34. What is the difference between Struct and Union in C?
Struct | Union | |
Memory Allocation: | Each member in a struct has its own memory location, and the total memory occupied by a struct is the sum of the sizes of its members. | All members share the same memory location. The size of a union is determined by the largest member within it. |
Usage: | Useful when different types of data need to be stored simultaneously, allowing access to each member independently. | Ideal when only one type of data needs to be stored at a time, conserving memory by sharing the same memory location for different members. |
Memory Efficiency: | Allocates memory for each member, which can lead to higher memory usage when storing multiple types of data. | Saves memory by sharing memory space; however, accessing the correct member is crucial to avoid data misinterpretation. |
Accessing Members: | Members are accessed independently using the dot (.) operator. | Only one member can be accessed at a time, using the dot (.) operator like structs. |
Example (Struct):
struct Point {
int x;
int y;
};
struct Point p1;
p1.x = 1;
p1.y = 2;
Example (Union):
union Data {
int i;
float f;
char c;
};
union Data d;
d.i = 10;
printf("%d", d.i); // Output: 10
d.f = 3.14;
printf("%f", d.f); // Output: 3.140000
35. What are Static Memory Allocation and Dynamic Memory Allocation in C?
Static memory allocation- Static memory allocation is the type of memory allocation that occurs during compilation. Allocating static memory reduces operating time. Because memory is allocated from the stack, it is quicker than dynamic memory allocation. Compared to dynamic memory allocation, this form of memory allocation is less effective. Within the array, it is mostly favored.
Dynamic memory allocation- Dynamic memory allocation is the process of allocating memory during runtime or execution. Because dynamic memory allocation uses the heap for memory allocation, it is slower than static memory allocation. When compared to static memory allocation, this form of memory allocation is more efficient. Most people in the linked list favor it.
C Interview Questions and Answers for Experienced
36. What is a Memory Leak? How to avoid it?
Depending on the size of the data type, assigning a variable requires space in either our heap or RAM. Nevertheless, if a programmer uses heap memory and forgets to delta it, eventually all of the RAM's memory will be used up and there won't be any more memory, which could result in a memory leak.
int main()
{
char * ptr = malloc(sizeof(int));
/* Do some work */
/*Not freeing the allocated memory*/
return 0;
}
You can track every memory allocation you make, plan to the point where you wish to destroy (in a good sense) that memory, and then remove it there to prevent memory leaks. Using the C++ smart pointer in C to connect it to GNU compilers is an additional method.
37. What is typedef?
typedef in C is used to create custom data type aliases. It allows programmers to define names for existing data types, making the code more readable and adaptable. Custom types created with typedef can simplify complex declarations and enhance code clarity.
typedef <existing-type> <new-type-identifiers>;
An alias name for the current complex type definition is provided by typedef. You may easily make an alias for any type with typedef. Typedef will let you write shorter code, whether you're declaring a sophisticated function pointer, structure, or simple integer.
38. Write a C program to check whether a number is prime or not.
// C program to check if a
// number is prime
#include
#include
// Driver code
int main()
{
int num;
int check = 1;
printf("Enter a number: \n");
scanf("%d", &num);
// Iterating from 2 to sqrt(num)
for (int i = 2; i <= sqrt(num); i++) {
// If the given number is divisible by
// any number between 2 and n/2 then
// the given number is not a prime number
if (num % i == 0) {
check = 0;
break;
}
}
if (num <= 1) {
check = 0;
}
if (check == 1) {
printf("%d is a prime number", num);
}
else {
printf("%d is not a prime number", num);
}
return 0;
}
Explanation
This C program checks whether a given number is prime or not. It takes an input number, iterates from 2 to the square root of the input, and checks for divisibility. If the number is divisible by any value in this range, it's not prime. If it's less than or equal to 1, it's not prime either. The result is displayed accordingly.
39. How is Source Code different from Object Code?
Source Code | Object Code |
The programmer creates the source code. | A translator such as a compiler can produce object code. |
High-level, easily understood by humans code. | Low-level programming is incomprehensible to humans. |
Compared to object code, source code is easier to modify and has fewer statements. | Compared to source code, object code has more statements and cannot be changed. |
Source code is not system-specific and is subject to change over time. | Object code is system-specific and modifiable. |
40. What is Pass-by-Reference in functions?
A function that uses pass-by-reference can change a variable without copying it. Since the provided parameter and variable share the same memory address, any modifications made to the argument will also affect the variables.
Example
// C program to change a variable
// using pass by reference
#include
// * used to dereference the variable
void change(int* num)
{
// value of num changed to 34
*num = 34;
}
// Driver code
int main()
{
int num = 12;
printf("Value of num before passing is: %d\n", num);
// Calling change function by passing address
change(&num);
printf("Value of num after changing with the help of "
"function is: %d",
num);
return 0;
}
Explanation
In this code, the change function takes a pointer to an integer as a parameter and modifies the value stored at that memory location. The address of the num variable is passed to the change function using the & operator. As a result, the value of num inside the main function is changed to 34.
Output
Value of num before passing is: 12
Value of num after changing with the help of function is: 34
41. What is an auto keyword?
In the C programming language, each local variable in a function is referred to as an automatic variable. For every variable declared inside a function or block, the default storage class is Auto. Access to auto variables is restricted to the block or function in which they have been defined. With the aid of pointers, we may use them outside of their intended usage. Auto keywords by default have a garbage value.
42. Write a program to print “Hello-World” without using a semicolon.
// C program to print hello-world
// without using semicolon
#include
// Driver code
int main()
{
// This will print the hello-world
// on the screen without giving any error
if (printf(“Hello - World”)) {
}
return 0;
}
Explanation
This code uses the printf function inside the if statement condition. The printf function returns the number of characters printed. Since the string "Hello - World" has a non-zero length, the condition evaluates to true and the empty block { } under if is executed, leading to the printing of "Hello - World".
Output
Hello - World
43. Explain Modifiers in C
In the C language, modifiers are keywords that are used to alter the meaning of fundamental data types. They outline how much memory should be set aside for the variable. There are five types of modifiers, which are
- long
- short
- signed
- unsinged
- long long
44. Write a program to print the factorial of a given number with the help of recursion.
// C program to find factorial
// of a given number
#include <stdio.h>
// Function to find factorial of
// a given number
unsigned int factorial(unsigned int n)
{
if (n == 0)
return 1;
return n * factorial(n - 1);
} // Driver code
int main()
{
int num = 5;
printf("Factorial of %d is %d", num, factorial(num));
return 0;
}
Explanation
This C program calculates the factorial of a given number using recursion. The factorial function recursively calculates the factorial: n! = n * (n-1)!. In the main function, the program calculates the factorial of 5. The program computes 5! Which is equal to 5 * 4 * 3 * 2 * 1 = 120, and prints the result.
Output
Factorial of 5 is 120
45. What is the difference between #include "..." and #include <...>?
In C programming, both #include "..." and #include <...> are used to include header files, but they differ in their search paths and usage:
#include "..." (Double Quotes): The C preprocessor searches the predefined list of system directories for the filename before checking user-specified directories (directories can be added to the predefined list by using the -I option).
#include <...> (Angle Brackets): The preprocessor follows the search path given for the #include <filename> form after first searching in the same directory as the file containing the directive. Include programmer-defined header files using this way.
Using double quotes ("...") is generally preferred for including project-specific headers, while angle brackets (<...>) are used for standard library or system headers to distinguish between user-defined and system-provided header files.
46. Write a program to check an Armstrong number.
// C program to determine whether
// the given number is Armstrong
// or not
#include
// Driver code
int main()
{
int n;
printf("Enter Number \n");
scanf("%d", &n);
int var = n;
int sum = 0;
// Loop to calculate the order of
// the given number
while (n > 0) {
int rem = n % 10;
sum = (sum) + (rem * rem * rem);
n = n / 10;
}
// If the order of the number will be
// equal to the number then it is
// Armstrong number.
if (var == sum) {
printf("%d is an Armstrong number \n", var);
}
else {
printf("%d is not an Armstrong number", var);
}
return 0;
}
Explanation
This C program checks if a given number is an Armstrong number or not. It calculates the sum of cubes of its digits and compares it with the original number. If they are equal, it's an Armstrong number. 1^3 + 5^3 + 3^3 = 153, which matches the original number.
Output
153 is an Armstrong number
47. Merge two sorted Linked Lists in C
include <stdio.h>
#include <stdlib.h>
// Node structure representing a node in the linked list
struct Node {
int data;
struct Node* next;
};
// Function to merge two sorted linked lists
struct Node* mergeSortedLists(struct Node* list1, struct Node* list2) {
if (list1 == NULL) return list2;
if (list2 == NULL) return list1;
if (list1->data < list2->data) {
list1->next = mergeSortedLists(list1->next, list2);
return list1;
} else {
list2->next = mergeSortedLists(list1, list2->next);
return list2;
}
}
// Function to create a new node with given data
struct Node* createNode(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// Function to print the merged linked list
void printList(struct Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
// Driver code
int main() {
struct Node* list1 = createNode(1);
list1->next = createNode(3);
list1->next->next = createNode(5);
struct Node* list2 = createNode(2);
list2->next = createNode(4);
list2->next->next = createNode(6);
printf("First sorted linked list: ");
printList(list1);
printf("Second sorted linked list: ");
printList(list2);
struct Node* mergedList = mergeSortedLists(list1, list2);
printf("Merged sorted linked list: ");
printList(mergedList);
return 0;
}
Explanation
In this program, two sorted linked lists (list1 and list2) are merged into a new sorted linked list (mergedList). The merged list is then printed.
Output
First sorted linked list: 1 3 5
Second sorted linked list: 2 4 6
Merged sorted linked list: 1 2 3 4 5 6
48. What is the use of an extern storage specifier?
In the C language, the extern keyword is used to increase the visibility of C variables and functions. External can be shortened to extern. When a specific file wants to access a variable from any other file, it is used. Variables containing the extern keyword are simply declared, not defined, which increases duplication. There is no need to declare or create extern functions because they are visible by default throughout the program.
49. What is the difference between getc(), getchar(), getch() and getche() in C?
- getc():
- Reads a character from the specified file stream (e.g., stdin, file).
- Requires stdio.h header.
- Offers flexibility for reading from different input sources.
- getchar():
- Reads a character from the standard input stream (stdin).
- Requires stdio.h header.
- Commonly used for simple character input from the keyboard.
- getch():
- Reads a character from the keyboard without echoing it to the screen.
- Typically used in older MS-DOS and Windows environments.
- Requires conio.h header (non-standard and not portable).
- getche():
- Reads a character from the keyboard and echoes it to the screen.
- Similar to getch(), but it displays the input character.
- Requires conio.h header (non-standard and not portable).
Note: getch() and getche() are not standard C functions and are not recommended for portable and modern C programming. Prefer using getc() or getchar() for standard and portable input operations.
50. How is import in Java different from #include in C?
Import is a keyword, but #include is a statement processed by pre-processor software. #include increases in the size of the code.
Related Interview Articles:
- C++ interview questions
- C# interview questions
- Java interview questions
- Javascript Interview Questions
- JQuery Interview Questions
Conclusion
In conclusion, mastering these top 50 C language interview questions is pivotal for aspiring programmers. These insights into fundamental concepts, data structures, and algorithms equip candidates with the knowledge needed to succeed in technical interviews. Practice, understanding, and confidence in these areas will undoubtedly pave the way to a successful C programming career. You can also consider doing our C programming tutorial from ScholarHat to upskill your career.
Download this PDF Now - C Interview Questions and Answers PDF By ScholarHat |
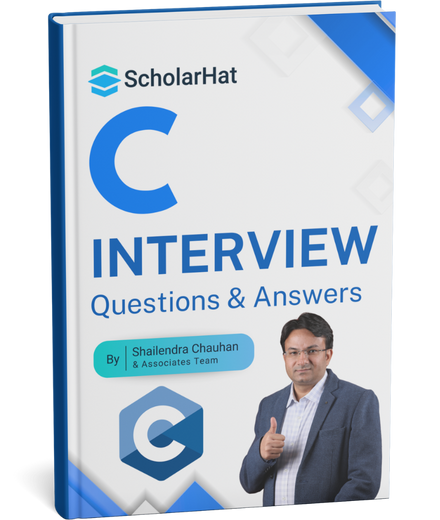
C Programming Interview Questions and Answers Bookers Book Unlock expert-level Docker interview preparation with our exclusive eBook! Get instant access to a curated collection of real-world interview questions, detailed Answers Bookers, and professional insights — all designed to help you succeed.
No downloads needed — just quick, free access to the ultimate guide for Docker interviews. Start your preparation today and move one step closer to your dream job!.
FAQs
- Beginner: Understanding core concepts like variables, operators, control flow, and functions.
- Intermediate: Pointers, arrays, structures, memory allocation, and recursion.
- Advanced: Algorithms, data structures (linked lists, trees), bit manipulation, and operating system concepts.
- Not understanding the question correctly: Listen carefully and rephrase if needed.
- Being unfamiliar with basic C concepts: Brush up on the fundamentals.
- Not explaining their thought process: Talk through your approach even if stuck.
- Poor code quality: Write clean, readable, and well-commented code.
- Lack of confidence: Practice and showcase your enthusiasm for C programming.