14
Junif else if statements in C Programming
if else...if Statement
if else...if statement is a part of a conditional statement in C. It is an extension of the if...else statement. If you want to check multiple conditions if the first “if” condition becomes false, use the if else...if ladder. If all the if conditions become false the else block gets executed.
In this C Tutorial, we will explore more about the if else...if statement in C Programming which will include the if else...if statement in C example, if else...if statement in C syntax, and if else...if statement in C flowchart. To deepen your understanding of such concepts, you can enroll in an online C programming course with a certificate free.
Syntax of if-else-if statement
if(test condition1){
//code to be executed if condition1 is true
}else if(test condition2){
//code to be executed if condition2 is true
}
else if(test condition3){
//code to be executed if condition3 is true
}
...
else{
//code to be executed if all the conditions are false
}
Flowchart:
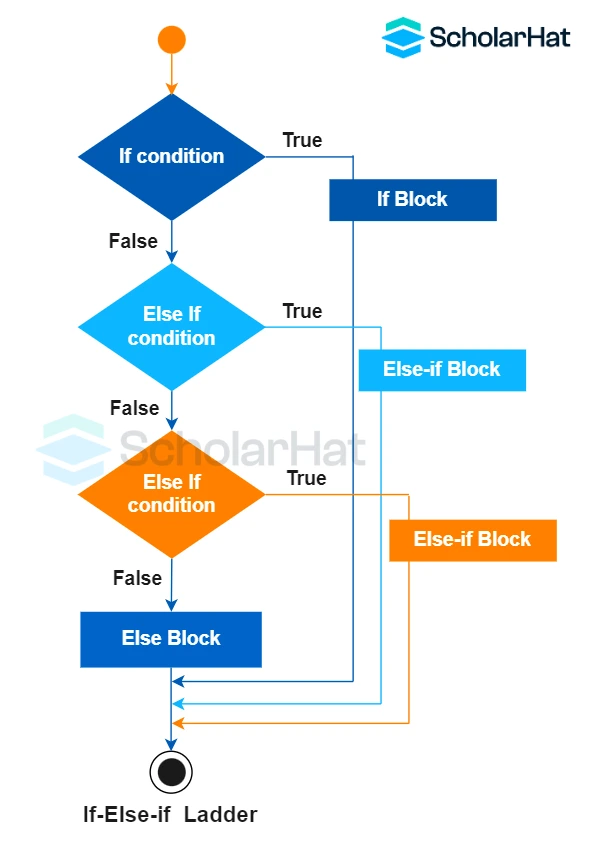
Example
#include <stdio.h>
int main(){
int number=5;
if(number==20){
printf("number is equals to 20");
}
else if(number==50){
printf("number is equal to 50");
}
else if(number==100){
printf("number is equal to 100");
}
else{
printf("number is not equal to 20, 50 or 100");
}
return 0;
}
Output
number is not equal to 20, 50 or 100
Explanation
- In the given code, the program evaluates multiple conditions.
- If the first if condition becomes false, the control goes to the else-if statements.
- If all the conditions fail, the statement inside the else block gets printed.
How to use If-Else If Statements in C?
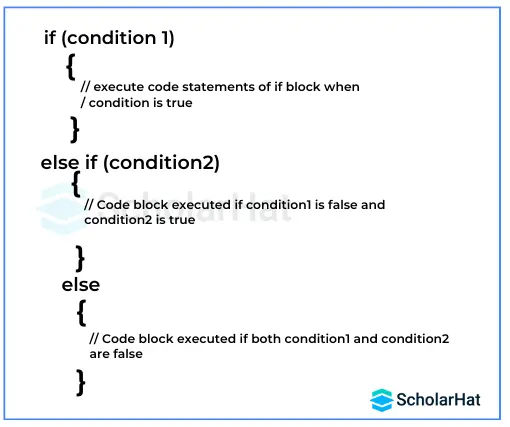
Handling Multiple Conditions
In the following example, we will see how to handle multiple conditions in an if-else-if statement.
Example:
#include
int main() {
int No= 10;
if (No> 0 && No% 2 == 0) {
printf("Number is positive and even\n");
}
else if (No> 0 && No% 2 != 0) {
printf("Number is positive and odd\n");
}
else if (No< 0) {
printf("Number is negative\n");
}
else {
printf("Number is zero\n");
}
return 0;
}
Explanation:
- So in this example supposeIf No is greater than 0 and divisible by 2 with no remainder (No% 2 == 0), it prints "Number is positive and even".
- In the second condition, If No is greater than 0 and not divisible by 2 (No % 2 != 0), it prints "Number is positive and odd".
- In the third condition, If the No is less than 0, it prints "Number is negative".
- In the last condition, If No is 0, it prints "Number is zero".
- For this kind of program, You can use the && operator as the logical AND operator, Because it checks if both conditions on its left and right sides are true.
- For that, You can use multiple conditions within the same if or else if statement.
Advantages
- Multiple Decision-Making: It allows us to manage multiple conditions in a structured way.
- Efficient: Using if else if allows the program to skip the operation of particular conditions once a true condition is found, Because of this it improves the efficiency of the code, especially if there are various conditions.
- Readable: if-else if statements make the code more simple and easy to read.
Disadvantages
- Complex in nature: As the multiple conditions increase, the if-else-if ladder can become complex and difficult to understand.
- High Maintenance: Adding or modifying multiple conditions requires careful consideration to ensure that the logic remains correct and all possible scenarios are accounted for which can increase its maintenance.
- More Execution: Each condition in an if-else if must be executed sequentially until a true condition is found. In short more conditions mean more execution.
Conclusion
So we have explored the if-else-if statement in C. I hope you enjoyed learning these concepts while programming with C. Feel free to ask any questions from your side. Your valuable feedback or comments about this article are always welcome. Level up your career in basics in C with our C Programming Course.