Storage Classes in C: Auto, Extern, Static, Register
Storage Classes in C: An Overview
Suppose you're eager to gain a better understanding of computer programming and love the challenge of learning new skills and building complex programs. In that case, an excellent starting point for you is to explore the fundamentals of Storage Class in C through C online training.
This article covers the storage classes in C, how they affect memory usage and variable declaration, and how they affect aspiring programmers' productivity and dependability. Dive into this journey with us as we explore what lies beneath the surface of storage classes in C for beginners.
What is the Storage Class in C?
Storage classes in C play a pivotal role in determining the scope, visibility, and lifetime of variables and C language functions within the program. Expert C programmers use the storage classes auto, register, static, & external to improve project optimization and problem solutions.
- In C, storage classes are keywords used to define the scope (visibility) and lifetime (duration) of variables.
- These storage classes determine how memory is allocated and deallocated for variables in a C program.
So, embark on the coding journey and unleash the full potential of C programming by understanding and making use of storage classes in C.
Use of Storage Class in C
Storage class & type are two of the attributes that a variable in a C program will have. The storage class, which controls the variable's lifetime, visibility, and scope, and type, which refers to the data type of any particular variable, are both used in this context.
Read More - Top 50 Mostly Asked C Interview Questions
Types of Storage Class in C
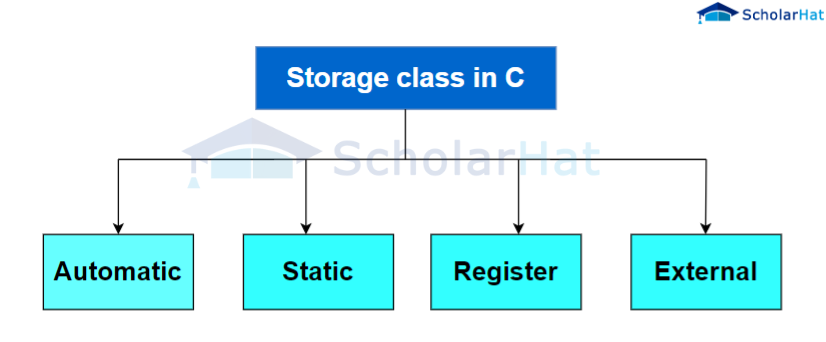
- Automatic storage class in C
- Static storage class in C
- Register storage class in C
- External storage class in C
Storage Classes | Names of class | Storage Place | Default Value | Scope | Lifetime |
auto | Automatic | RAM | Garbage Value | Local | Within function |
extern | External | RAM | Zero | Global | Till the end of the main program Maybe declared anywhere in the program |
static | Static | RAM | Zero | Local | Till the end of the main program, Retains value between multiple functions call |
register | Register | Register | Garbage Value | Local | Within function |
Automatic Storage Class in C
- Automatic storage class in C is one of the most basic and important aspects of the C programming language.
- This class enables the user to declare variables without having to specify their type at run time.
- It also provides a way for c-programmers to declare variables of a specific type, such as int, double, and char.
- Automatic Storage Class in C is so named because it allows the computer's compiler to automatically assign storage space from a given pool that is available.
- By facilitating quicker memory access & logical data organization and boosting program clarity and effectiveness,
- Automatic Storage Class increases code efficiency.
Example
#include <stdio.h>
int main()
{
int a; //auto
char b;
float c;
printf("%d %c %f",a,b,c); // printing initial default value of automatic variables a, b, and c.
return 0;
}
The automatic variables 'a' (int), 'b' (char), & 'c' (float) are declared in this piece of C code in the C Compiler without being initialized. Then, because they are uninitialized, it prints their initial default values, which may result in behavior that is not defined.
Output
garbage garbage garbage
Static Storage Class in C
- The static storage class in C is a powerful tool for programmers, allowing them to promote optimal memory management and facilitate efficient program execution.
- By removing the requirement for constant re-initialization, the "static" keyword in programming enables variables to keep their values across calls to a single function, increasing code reliability and efficiency.
- By limiting variables to a local scope and minimizing potential conflicts, also improves code clarity.
- Furthermore, by employing the static storage class in C, programmers can vastly increase the performance of their applications and achieve more sophisticated solutions to complex problems.
Example
#include<stdio.h>
static char c;
static int i;
static float f;
static char s[100];
void main ()
{
printf("%d %d %f %s",c,i,f); // the initial default value of c, i, and f will be printed.
}
The static variables 'c' (char), 'i' (int), 'f' (float), and 's' (char array) are declared with global scope in this C code. Then it publishes the initial default values for the letters "c," "i," "f," and "s," which are, respectively, 0, 0, 0.0, and an empty string. For standard compliance, the "main" function should return an "int" rather than a "void."
Output
0 0 0.000000 (null)
Register Storage Class in C
- The register storage class in C provides an exciting opportunity for programmers to optimize the execution time of their code.
- By utilizing the register keyword while declaring a variable using C programming language, programmers instruct the compiler to store that variable within the microprocessor's high-speed registers instead of standard memory.
- The ' register' storage class streamlines crucial code portions by allowing quicker access and variable manipulation.
- It works best with small, often-used variables but is constrained by the registers that the microprocessor has available.
- Applications are modified for quick-paced computing environments by "Register," which improves code performance.
Example
#include <stdio.h>
int main()
{
register int a; // variable a is allocated memory in the CPU register. The initial default value of a is 0.
printf("%d",a);
}
This C code declares a variable named "a" with the storage class "register," allocating memory in the CPU register for quicker access. The default value of 'a' is then printed, which is 0.
Output
0
External Storage Class in C
- The external storage class in C is an intriguing feature that allows extending a program's functionality by sharing variables across multiple source files.
- By allowing variables initialized in one file to be utilized in another, the 'extern' keyword in C encourages modularity and improves the organization and maintenance of the code.
- It makes it simpler to collaborate and construct ambitious, complex projects since it promotes communication across code segments.
Example
#include <stdio.h>
int a;
int main()
{
extern int a; // variable a is defined globally, the memory will not be allocated to a
printf("%d",a);
}
A global declaration of the integer variable 'a' appears in this section of C code in the C Editor. The globally defined variable "a" is referred to inside the "main" function using the "extern" keyword without needing to allocate new memory. It outputs the value 'a''s default beginning value, which is 0.
Output
0
FAQs
1. What is a storage class in C?
A storage class specifier in the C programming language controls how variables are allocated, accessed, and stored in memory. It establishes a variable's range, lifetime, and visibility within a program.
2. What are the types of storage classes in C?
Storage classes in C often fall into one of four categories: auto, register, static, or extern. Each type controls the quantity, range, and linking of variables, giving programmers the ability to define how they should behave.
3. What is the purpose of the storage classes in C with examples?
The scope, lifetime, and visibility of variables are specified by storage classes in C. While the"auto" storage class is used for local variables with automatic storage duration, the"static" storage class, for example, allows a variable to keep its value across function calls.
4. What does a storage class do?
In C, a storage class describes the properties of variables, such as where they are kept in memory, how long they last, and how easily they may be accessed. Programmers can modify variable behavior to meet the needs of their programs by designating a storage class, which enhances program structure and resource efficiency.