14
JunTop 50 Mostly Asked C++ Interview Questions and Answers
Are you going for an interview on C++ in the upcoming days and want a quick recap that will help you in your interview preparation? So, you need to worry about that because we have created the Top 50 C++ Interview Questions and Answers series that will surely help you. Whether you're a beginner or an experienced developer, this resource equips you with in-depth knowledge to excel in C++ interviews with the help of these C++ language questions.
In the C++ tutorial, Master key concepts, coding challenges, and problem-solving techniques to boost your confidence and land your dream job. To make things easier for you,
C++ Interview Questions and Answers for Freshers
Let's explore the top most asked C++ interview Questions and Answers.
1. What are the Advantages of C++?
- Object-Oriented Programming: Supports classes and objects, enabling better organization of code and data encapsulation.
- Efficiency: Allows low-level memory manipulation, resulting in faster execution and efficient use of system resources.
- Inheritance and Polymorphism: Supports inheritance, allowing the creation of new classes based on existing ones, and polymorphism enables dynamic method binding.
- Standard Template Library (STL): Provides a collection of useful classes and functions, enhancing code reuse and productivity.
- Flexibility: Allows both high-level and low-level programming, catering to a wide range of applications.
2. What are the different Data Types present in C++?
There are 4 data types in C++:
- Primitive Datatype(basic datatype). Example- char, short, int, float, long, double, bool, etc.
- Derived datatype. Example- array, pointer, etc.
- Enumeration. Example- enum
- User-defined data types. Example- structure, class, etc.
3. Define ‘std'.
Standard is another name for "std," or it can be seen as a namespace. The compiler is instructed to add all objects under the std namespace and import them into the global namespace by using the command "using namespace std." Because of the global namespace instillation, we may use "cout" and "cin" instead of "std::_operator_."
4. What is the difference between C and C++?
Factor | C Language | C++ Language |
Paradigm | Procedural programming language focused on functions and structured programming. | The hybrid language supports both procedural and object-oriented programming paradigms. |
Syntax | Simpler syntax with a limited set of keywords. | Extended syntax with additional keywords for classes, objects, and other OOP features. |
Data Handling | Relies on structures and functions for data manipulation. | Supports classes and objects, allowing data encapsulation and abstraction. |
Function Overloading | Does not support function overloading. | Allows defining multiple functions with the same name but different parameters. |
Operator Overloading | Does not support operator overloading. | Permits defining custom behaviors for operators in user-defined classes. |
Standard Template Library (STL) | Lacks built-in support for containers, algorithms, and iterators. | Provides STL, a powerful library offering generic classes and functions for various data structures and algorithms. |
Exception Handling | Relies on error codes and conditional statements for error handling. | Supports try-catch blocks for robust exception handling. |
5. What are References in C++?
A variable becomes an alias of an existing variable when it is described as a reference. To put it simply, a referred variable is a named variable that is another variable that already exists, with the understanding that any changes made to the reference variable would also affect the previously existing variable. A reference variable has a '&' before it.
Syntax: int DNT = 10;
// reference variable
int& ref = DNT;
int DNT = 10;
// reference variable
int& ref = DNT;
6. What are Classes and Objects in C++?
Class:
- Blueprint: A class is a blueprint or a template for creating objects.
- Data and Functions:The encapsulation of data (attributes) and functions (methods) that operate on the data.
- Abstraction: Provides a way to represent real-world entities with properties, behaviors, and expressions.
Object:
- Instance: An object is an instance of a class representing a specific entity.
- Attributes: Objects store data defined in the class's attributes.
- Methods: Objects can invoke the methods defined in the class, performing operations specific to that object's type.
Example: If "Car" is a class, an object could be a specific car with its unique attributes and behaviors.
7. What do you mean by Call by Value and Call by Reference in C++?
Call by Value:
- Value Passing: When a function is called by value, the actual value of the variable is passed to the function.
- Copy of Data: A copy of the actual parameter's value is created in the function's parameter variable.
- Changes are Local: Changes made to the parameter inside the function are local to the function and do not affect the original variable outside the function.
- Efficiency: Slower for large data structures as it involves copying the entire data.
- Syntax: void function(int x) { ... }
Call by Reference:
- Reference Passing: When a function is called by reference, the memory address of the actual variable is passed to the function.
- Direct Access: The function directly operates on the original data, not on a copy.
- Changes are Global: Modifications inside the function affect the original variable outside the function.
- Efficiency: Faster and memory-efficient as it avoids creating a copy of the data.
- Syntax: void function(int &x) { ... }
|
8. Define token in C++
In C++, a token is a fundamental unit of source code, representing individual elements recognized by the compiler during lexical analysis:
- Identifiers: Names for variables, functions, classes, etc.
- Keywords: Reserved words with specific meanings, e.g., if, else, int.
- Literals: Constants like numbers (42, 3.14) or strings ("hello").
- Operators: Symbols for mathematical (+, -) or logical (&&, ||) operations.
- Comments: Single-line (//) or multi-line (/* */) comments ignored by the compiler.
- Whitespace: Spaces, tabs, and newlines separating tokens but generally not stored for further processing.
9. What is the difference between struct and class in C++?
Factors | Struct | Class |
Access Control | Members are public by default. | Members are private by default. |
Inheritance | Does not support member access control keywords like private and protected. | Supports member access control, enabling private, protected, and public inheritance. |
Usage | Primarily used for grouping data members and creating simple data structures with minimal methods. | Used for creating complex data structures with methods, encapsulation, and data hiding. |
Default Member Access | Members are public unless explicitly specified otherwise | Members are private unless explicitly specified otherwise. |
Methods | Cannot have member functions until C++11 standard. | It can have member functions for encapsulating behavior and data manipulation. |
10. What is the difference between Reference and Pointer in C++?
The difference between Reference and Pointer in C++
Pointers in C++ | References in C++ |
Pointers store the memory address of a variable. | References are aliases for existing variables. |
Defined using * symbol, e.g., int *ptr. | Defined using & symbol during declaration, e.g., int &ref = var. |
This can be NULL or reassigned to point to different addresses. | It cannot be NULL; it must be initialized to a variable. |
Pointers can be reassigned to point to different variables or addresses. | This cannot be reassigned to refer to a different variable after initialization. |
Requires * operator to access the value stored at the address. | No need for an operator; it is accessed like a regular variable. |
|
11. What is Operator Overloading in C++?
Operator overloading in C++ allows defining custom behaviors for operators when used with user-defined classes or data types. It enables objects of a class to behave like built-in types, allowing programmers to provide their implementation of operators such as +, -, *, etc., tailored to specific class instances.
Example in C++ Compiler
#include <iostream>
using namespace std;
class Complex {
private:
float real;
float imag;
public:
Complex() : real(0), imag(0) {}
Complex operator + (const Complex& obj) {
Complex temp;
temp.real = real + obj.real;
temp.imag = imag + obj.imag;
return temp;
}
void input() {
cout << "Enter real part: ";
cin >> real;
cout << "Enter imaginary part: ";
cin >> imag;
}
void display() {
if (imag < 0) {
cout << "Sum = " << real << " - " << -imag << "i";
} else {
cout << "Sum = " << real << " + " << imag << "i";
}
}
};
int main() {
Complex num1, num2, result;
cout << "Enter the first complex number:\n";
num1.input();
cout << "Enter the second complex number:\n";
num2.input();
result = num1 + num2;
result.display();
return 0;
}
Explanation
In this code, the Complex class represents a complex number with real and imaginary parts. The + operator is overloaded inside the class to add two complex numbers. The input() function is used to input complex numbers, and the display() function is used to display the result. When you run the program, it will prompt you to enter the real and imaginary parts of two complex numbers and then display the sum.
12. What is Polymorphism in C++?
Polymorphism in C++ allows objects of different classes to be treated as objects of a common superclass. It enables functions to operate on objects of multiple derived classes through pointers or references to the base class, simplifying code and enhancing flexibility in object-oriented programming.
The two types of polymorphism in C++ are:
- Compile Time Polymorphism
- Runtime Polymorphism
13. Explain the Constructor in C++
In C++, a constructor is a special member function with the same name as the class. It is automatically invoked when an object of the class is created. Constructors initialize the object's data members and ensure the object is in a valid state upon creation.
Example
#include <iostream>
using namespace std;
class MyClass {
private:
int num;
public:
// Default constructor
MyClass() {
num = 0;
cout << "Default Constructor Called! Num is set to 0." << endl;
}
// Parameterized constructor
MyClass(int value) {
num = value;
cout << "Parameterized Constructor Called! Num is set to " << num << "." << endl;
}
// Member function to display the value
void display() {
cout << "Value of num: " << num << endl;
}
};
int main() {
// Creating objects of MyClass using constructors
MyClass obj1; // Calls default constructor
MyClass obj2(42); // Calls parameterized constructor
// Calling member function to display the values
cout << "Object 1: ";
obj1.display(); // Displays num = 0
cout << "Object 2: ";
obj2.display(); // Displays num = 42
return 0;
}
Explanation
In this example, the MyClass class has a default constructor and a parameterized constructor. When objects obj1 and obj2 are created, the constructors are automatically called. The default constructor initializes num to 0, and the parameterized constructor initializes num with the provided value (42 in this case). The display() member function is used to display the values of num for the created objects.
Output
Default Constructor Called! Num is set to 0.
Parameterized Constructor Called! Num is set to 42.
Object 1: Value of num: 0
Object 2: Value of num: 42
|
14. Compare Compile-Time Polymorphism and Runtime Polymorphism in C++.
Let's Understand the Compile-Time Polymorphism and Runtime Polymorphism in C++.
Compile-time Polymorphism | Runtime Polymorphism |
Occurs during compilation. | Occurs during program execution. |
Method binding is done at compile-time | Method binding is done at runtime using virtual functions and inheritance. |
Achieved through function overloading and operator overloading. | Achieved through inheritance and virtual functions. |
Resolves calls at compile-time, resulting in faster execution. | Resolves call at runtime, allowing flexibility but incurring slight performance overhead. |
Examples include function overloading and templates. | Example: Function overriding using virtual functions in base and derived classes. |
15. What are the C++ Access Specifiers?
Access specifiers in C++ control the visibility and accessibility of class members. There are three types:
- Public: Members are accessible from outside the class.
- Private: Members are only accessible within the class.
- Protected: Members are accessible within the class and its subclasses (derived classes).
16. What is a Reference in C++?
In C++, a reference is an alias for a variable. It allows you to use an existing variable through a different name. References are often used as function parameters to modify variables outside the function or to avoid unnecessary copying of large data structures, enhancing performance and readability.
Example
int x=10;
int &ref=x; //reference variable
Any changes we make to the value of ref will show up in x. A reference variable cannot refer to another variable once it has been initialized. An array of references cannot be declared, although an array of pointers may.
17. What do you mean by Abstraction in C++?
In C++, Abstraction in C++refers to the concept of hiding complex implementation details and showing only the necessary features of an object. It allows programmers to create user-defined data types and focus on what an object does rather than how it achieves its functionality, enhancing code simplicity and reusability.
18. Is Deconstructor Overloading Possible? If Yes, then Explain, and if no, then why?
Overloading a destructor is not feasible. There is just one method to delete an object since destroyers don't accept parameters. Destructor overloading is, therefore, not feasible.
19. What is the Difference between Function Overloading and Operator Overloading?
Function overloading and operator overloading are both techniques in C++ that provide multiple definitions for functions or operators, but they differ in their application:Function Overloading | Operator Overloading |
It involves defining multiple functions with the same name but different parameters. | Involves defining custom behaviors for C++ operators like +, -, *, etc. |
Enables a function to perform different tasks based on the input arguments. | Allows objects of user-defined classes to work with operators, extending their functionality. |
It helps improve code readability and flexibility. | Provides a natural syntax for user-defined types, enhancing code expressiveness and clarity |
20. What are Destructors in C++?
Destructors in C++in C++ are special member functions of a class that are used to clean up resources allocated by objects. They have the same name as the class prefixed with a tilde (~) and are automatically invoked when an object goes out of scope or is explicitly deleted, ensuring proper resource deallocation.
Syntax
class X {
public:
// Constructor for class X
X();
// Destructor for class X ~X();};
C++ Programming Interview Questions for Intermediates
1. What are the Static Members and Static Member Functions in C++?
When a class variable is marked static, memory is set aside for it for the duration of the program. There is only one copy of the static member, regardless of how many objects of that class have been produced. This means that all of the objects in that class can access the same static member.
Even in the absence of any class objects, a static member function can still be invoked since it can be reached with just the class name and the scope resolution operator::
2. Explain Inheritance in C++
Inheritance in C++is a fundamental object-oriented programming concept that allows a class (derived or child class) to inherit properties and behaviors from another class (base or parent class). Derived classes can access public and protected members of the base class, promoting code reusability and establishing a relationship between classes. This mechanism enables the creation of a hierarchy of classes where child classes inherit attributes and methods from their parent, facilitating efficient and organized code development.
|
3. Difference between equal to (==) and assignment operator(=)?
- The equal to operator == determines if two values are equal. It returns false; otherwise, if they are equal, then it is true.
- The assignment operator = gives the left operand the value of the right-side expression.
4. What is the difference between an Array and a List in C++?
the difference between an Array and a List in C++?
Factor | Array | List |
Memory Allocation | Contiguous memory allocation for elements, allowing direct access via indexing. | Elements are stored in nodes scattered across memory, accessed through pointers, offering dynamic size and flexibility. |
Size | Fixed-size: must specify the size during declaration. | Dynamic size: can grow or shrink during runtime. |
Insertion and Deletion | Array Insertion and deletion can be inefficient due to shifting elements. | Efficient insertion and deletion as elements can be easily added or removed without moving other nodes. |
Access Time | Constant time access for elements using indices. | Linear time access, iterating through nodes for specific elements. |
Functionality | Limited functionality; doesn't provide built-in methods for operations like insertion or deletion. | Provides built-in methods for various operations, making it versatile for dynamic data structures. |
5. What is Loops in C++? Explain different types of loops in C++
Loops in C++ are control structures that allow the execution of a block of code repeatedly as long as a specified condition is true. There are three types of loops in C++:
- For Loop: Executes a block of code a specified number of times, with an initialization, condition check, and increment/decrement statement.
- While Loop: Executes a block of code as long as a specified condition is true.
- Do-While Loop: Similar to the while loop, but ensures the code block is executed at least once before checking the condition.
6. What is the difference between a for loop and a while loop in C++?
The difference between a for loop and a while loop in C++
Factors | For Loop | While Loop |
Initialization | For loop initializes a counter variable within the loop header. | While loop initializes the counter variable before the loop. |
Structure | For loop has a built-in structure for initialization, condition, and increment. | While loop relies on manual control of these aspects within the loop. |
Use Cases | For loops are suitable for iterating a specific number of times. | While loops are more flexible for looping until a condition is met or for indefinite loops. |
Code Readability | For loops can be more concise and easier to read for certain repetitive tasks. | While loops offer greater control but may require more code for simple iterations |
7. What is the difference between a while loop and a do-while loop in C++?
While both while and do-while loops in C++ are used for repetitive execution, they differ in their loop control flow:
While Loop | Do-While Loop |
Condition is evaluated before entering the loop; the loop body may never execute if the condition is initially false. | The loop body is executed at least once before checking the condition; it always runs at least once. |
It's a pre-test loop, as it checks the condition before executing the loop's body. | It's a post-test loop, as it ensures the loop body is executed before condition checking. |
Suitable for scenarios where the loop may not need to run at all, based on the condition. | Useful when you want to guarantee that the loop body runs at least once, regardless of the initial condition, such as when taking user input validation. |
8. Discuss the difference between prefix and postfix in C++?
In C++, prefix and postfix are used to increment or decrement variables. The key differences between them are:
Prefix (++i, --i) | Postfix (i++, i--) |
Increments or decrements the variable's value before its current value is used in the expression. | Uses the current value of the variable in the expression before incrementing or decrementing it. |
Changes the original variable and then evaluates the expression. | Evaluate the expression and then change the variable. |
Offers slightly better performance as it avoids creating a temporary variable. | Involves the creation of a temporary variable to store the original value, which may have a minor impact on performance. |
9. What is the difference between new and malloc() in C++?
The difference between new and malloc() in C++are:
Factor | new | malloc() |
Syntax | new is an operator in C++ that automatically calculates the size of the object being allocated and returns a pointer to the appropriate type. The syntax is: new Type; or new Type[size]; | malloc() is a function in C that allocates a specific number of bytes of memory and returns a pointer to the first byte. The syntax is: malloc(size); |
Type Safety | new is type-safe. It allocates memory for the specified data type and returns a pointer of that type. For example, if you allocate memory for an integer using new, the pointer returned will be of type int*. | malloc() does not provide type information. It always returns a pointer to void (void*). You need to cast the pointer to the appropriate type before using it. |
Initialization | When you use new, the allocated memory is initialized by calling the constructor of the object (if available). For primitive types like int, the memory is initialized to zero. | Memory allocated by malloc() is not initialized. You need to explicitly set the initial values for the allocated memory. |
Return Value | new throws a std::bad_alloc exception if it fails to allocate memory. | malloc() returns a null pointer (nullptr in C++) if it fails to allocate memory. |
Deallocation | Memory allocated with new should be deallocated using delete for single objects or delete[] for arrays to avoid memory leaks. | Memory allocated with malloc() should be deallocated using free(). |
10. What is the difference between virtual functions and pure virtual functions in C++?
Virtual functions and pure virtual functions are concepts in C++ related to polymorphism and inheritance:
Virtual Functions:
- Declared in the base class with the virtual keyword.
- It can be overridden in derived classes.
- Provides a default implementation in the base class.
- Objects of the derived class can be used through pointers or references of the base class type.
Pure Virtual Functions:
- Declared in the base class with the virtual keyword and set to 0.
- Has no implementation in the base class.
- Forces derived classes to provide an implementation.
- Classes containing pure virtual functions are abstract and cannot be instantiated.
11. What is Function Overriding in C++?
Function overriding in C++ occurs when a derived class provides a specific implementation for a function that is already defined in its base class. To achieve this, the function in the derived class must have the same name, return type, and parameters as the one in the base class. When an object of the derived class calls the overridden function, the derived class's implementation is executed instead of the base class's version, allowing for customization and polymorphic behavior in object-oriented programming.
12. What are the various OOP concepts in C++?
In C++, Object-Oriented Programming (OOP) concepts include:
- Classes & Objects: Blueprint for creating objects.
- Encapsulation: Binding data and methods that operate on the data, restricting direct access.
- Inheritance: Creating new classes from existing ones, inheriting properties and behaviors.
- Polymorphism: Objects of different classes can be treated as objects of a common base class.
- Abstraction: Hiding complex implementation details and showing only essential features.
- Constructor & Destructor: Special member functions for object initialization and cleanup, respectively.
- Operator Overloading: Redefining operators for user-defined types.
13. When should we use multiple inheritance in C++?
Multiple inheritance in C++ should be used cautiously and in specific scenarios where it simplifies the design without introducing complexity. It is appropriate when a class needs to inherit properties and behaviors from more than one unrelated class. For example, in GUI frameworks, a class may inherit from both a graphical component class and an event handling class. However, it requires careful planning to avoid ambiguity issues, and alternative design patterns like composition or interfaces should be considered to enhance code readability and maintainability.14. What is virtual inheritance in C++?
Virtual inheritance in C++ is a mechanism that prevents multiple instances of a base class in a class hierarchy. It ensures that only one instance of the base class exists when multiple derived classes inherit from it. This prevents issues like the "diamond problem," where ambiguity arises due to multiple inheritance paths.
15. What is a virtual destructor in C++?
A virtual destructor in C++ is a destructor declared in the base class with the virtual keyword. When a derived class object is deleted through a pointer to the base class, a virtual destructor ensures that the derived class's destructor is called, preventing memory leaks and ensuring proper cleanup of resources in polymorphic hierarchies.
C++ Programming Interview Questions for Experienced
1. Which operations are permitted on pointers?
The variables used to hold the address position of another variable are called pointers. The following operations on a pointer are allowed:
- Increment/Decrement of a Pointer
- Addition and Subtraction of integer to a pointer
- Comparison of pointers of the same type
2. What is the purpose of the “delete” operator in C++?
In C++, the delete operator is used to deallocate memory that was previously allocated using the new operator. It helps free up memory occupied by dynamically allocated objects, preventing memory leaks, and managing resources efficiently during runtime.
Example
int DNT = new int[100];
// uses DNT for deletion
delete [] DNT;
3. How delete [] is different from delete in C++?
In C++, delete and delete[] are used to deallocate memory previously allocated with new and new[] operators, respectively. The key differences are:
Memory Allocation Type:
- delete is used for single objects allocated with new.
- delete[] is used for arrays of objects allocated with new[].
Deallocation Process:
- delete calls the destructor of the single object before deallocating memory.
- delete[] calls the destructors of all objects in the array before deallocating the memory block.
4. What do you know about friend class and friend function in C++?
Friend class- In C++, a friend class is a class that is granted access to the private and protected members of another class. It is declared using the friend keyword in the class declaration. Friend classes can access private and protected members of the class they are friends with, providing controlled access for specific classes.
Example
class Class_1st {
// ClassB is a friend class of ClassA
friend class Class_2nd;
statements;
}
class Class_2nd {
statements;
}
Friend function- In C++,a friend function is a function that is not a member of a class but has access to its private and protected members. It is declared inside the class and can access the class's private and protected data, providing a way to allow external functions special privileges with a specific class.
Example
class DNT {
statements;
friend dataype function_Name(arguments);
statements;
}
OR
class DNT{
statements'
friend int divide(10,5);
statements;
}
5. What is an Overflow Error?
- Overflow occurs when the value is more than what the data type can handle, an error is produced. Said another way, it's an error type that falls inside the prescribed range but is valid outside of it.
- A variable of size 2,247,483,648 will result in an overflow error, for instance, because the range of the int data type is –2,147,483,648 to 2,147,483,647.
6. What does the Scope Resolution operator do in C++?
In C++, the Scope Resolution operator (::) is used to specify the class or namespace to which a particular identifier (such as a variable or function) belongs. It allows access to class members when there is a naming conflict between local and class-level variables or functions.
The Scope Resolution Operator (::) in C++ is used:
- To define member functions outside the class.
- To access static members of a class.
- To differentiate between class member functions/variables and global functions/variables with the same name.
- To access nested classes or namespaces.
- In the context of the C++ Standard Template Library (STL) for nested classes or functions.
7. What are the C++ access modifiers?
In C++, access modifiers control the visibility and accessibility of class members. There are three access modifiers:
1. Public
- Members declared as public are accessible from any part of the program.
- Public members can be accessed through class objects and pointers.
2. Private
- Members declared as private are only accessible within the class they are declared in.
- Private members cannot be accessed directly from outside the class.
3. Protected
- Protected members are accessible within the class and its subclasses (derived classes).
- They are not accessible from outside the class hierarchy.
8. Can you compile a program without the main function?
Indeed, a program may be compiled without a main() call. For instance, Use Macros that define the main
Example
// C++ program to demonstrate the
// a program without main()
#include <iostream>
#define fun main
int fun(void)
{
printf("ScholarHat");
return 0;
}
9. What is STL?
STL, or Standard Template Library, is a powerful set of C++ template classes and functions that provides general-purpose data structures and algorithms. It simplifies complex tasks, promotes code reuse, and enhances efficiency. STL includes containers, algorithms, iterators, and function objects, making C++ programming more efficient and convenient.
10. Define inline function. Can we have a recursive inline function in C++?
In C++, an inline function is a function that is expanded in place where it is called instead of being executed through a regular function call mechanism. It is declared with the inline keyword and helps reduce the function call overhead by inserting the function's code directly at the call site.
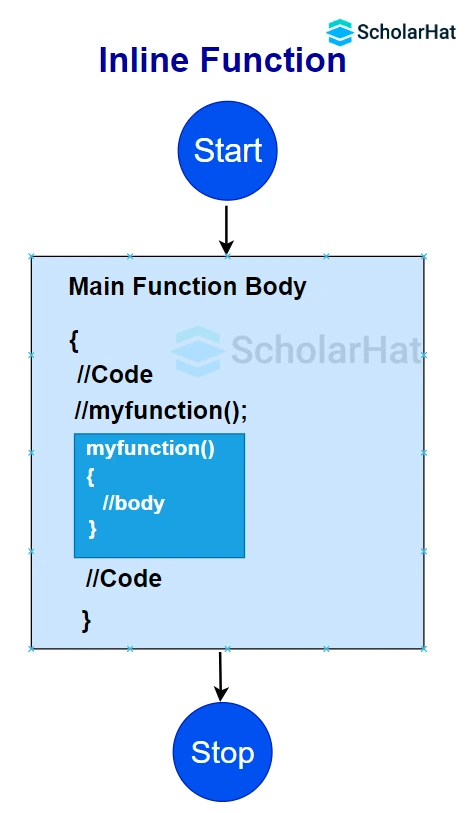
Syntax
inline data_type function_name()
{
Body;
}
The answer is No; It cannot be recursive.
- An inline function cannot be recursive because it simply loads the code into the location from where it is called without keeping track of any information on the stack that would be required for recursion.
- Additionally, the compiler will automatically disregard an inline keyword placed in front of a recursive function as it only interprets inlines as suggestions.
11. What is an abstract class, and when do you use it?
In C++, an abstract class is a class that cannot be instantiated and is meant to be subclassed by other classes. It serves as a blueprint for derived classes, defining abstract (pure virtual) methods without providing their implementations. Here's when and why you use abstract classes:
- Incomplete Implementation: Abstract classes contain one or more pure virtual functions without implementation details.
- Derived Class Requirement: Abstract classes are designed to be subclassed. Derived classes must provide concrete implementations for all pure virtual functions.
- Interface Definition: Abstract classes define interfaces, ensuring consistent method signatures across derived classes.
- Polymorphism: Abstract classes enable polymorphism, allowing objects of derived classes to be treated as objects of the abstract class type.
- Forcing Implementation: Abstract classes enforce derived classes to provide specific functionality, ensuring proper class hierarchy design.
Use abstract classes when you want to create a common interface for a group of related classes, ensuring that derived classes provide specific implementations for essential methods while allowing for polymorphic behavior.
12. What are the static data members and static member functions in C++?
A class's static data member is a regular data member that is preceded by the term static. When a program runs, it runs before main() and is initialized to 0 upon the creation of the class's first object. Its scope is lifetime, but it is only accessible to a particular class.
Syntax
static Data_Type Data_Member;
The member function that is used to access other static members of the data or other static member functions is known as the static member function. A static keyword is also used to specify it. Either the class name or class objects can be used to access the static member method.
Syntax
classname::function name(parameter)
13. Define namespace in C++.
In C++, a namespace is a declarative region that provides a scope for identifiers to avoid naming conflicts. Here's a concise explanation within 80 words, using points:
- Scope Isolation: Namespaces prevent naming collisions by encapsulating identifiers within a distinct scope.
- Syntax: Declared using the namespace keyword followed by the namespace name and a code block {}.
- Usage: Members like variables, functions, and classes can be declared inside namespaces.
- Access Control: Namespaced elements are accessed using the scope resolution operator ::
- Example: namespace MyNamespace { int x; void func(); }
- Avoiding Ambiguity: Helps organize code, making it easier to read, maintain, and avoid conflicts in larger projects.
14. Define storage class in C++ and name some of them
The properties (lifetime and visibility) of a variable or function are defined by the storage class. These properties often aid in tracking a variable's existence while a program runs.
Syntax
storage_class var_data_type var_name;
In C++, there are four primary storage classes, each serving different purposes in controlling the scope, lifetime, and visibility of variables and functions:
1. Auto
- Local variables declared within a block are of automatic storage class by default.
- They are created when the block is entered and destroyed when the block is exited.
2. Static
- Variables declared with the static keyword have a lifetime throughout the program.
- Static local variables retain their values between function calls.
- Static global variables are accessible only within the file where they are declared.
3. Extern
- Variables declared with the extern keyword are defined elsewhere in the program.
- They have global scope and can be accessed across multiple files.
4. Register
- Variables declared with the register keyword suggest the compiler store them in a register for faster access.
Note: The register keyword is deprecated in modern C++ and has limited usage.
15. What is a mutable storage class specifier? How can they be used?
As implied by its name, the mutable storage class specifier modifies a class data member only when it is applied to an object that is defined as const, even if the member is a part of the object. Reference, static, or const members can't employ the mutable specifier. This pointer provided to the function becomes const when the function is declared as const.
Conclusion
In conclusion, mastering these top 50 C++ language interview questions is pivotal for aspiring programmers. These insights into fundamental concepts, data structures, and algorithms equip candidates with the knowledge needed to succeed in technical interviews.
If you are preparing for theC++ Interview Questions and Answers Book, this C++ Interview Questions and Answers Book can really help you. It has simple questions and answers that are easy to understand. Download and read it for free today!
You can also consider doing our C++ tutorial from ScholarHat to upskill your career. Practice, understanding, and confidence in these areas will undoubtedly pave the way to a successful C++ programming career.
Download this PDF Now - C++ Interview Questions and Answers PDF By ScholarHat |
Test Your Skills with the Following MCQs
Dear learners, test your skills in the following MCQs, and I hope this will surely help you to increase your knowledge.
Q 1: What is the main function in a C++ program?
FAQs
- Beginner: Understanding core concepts like variables, operators, control flow, functions, and basic pointers.
- Intermediate: Arrays, structures, unions, dynamic memory allocation (new/delete), references, inheritance, and polymorphism.
- Advanced: Templates, STL (Standard Template Library), multithreading, smart pointers, exception handling, and design patterns.
- Confusing pointers and references: Grasping the subtle differences is crucial.
- Struggling with memory management: Understanding memory leaks and proper deallocation is key.
- Overlooking the importance of clean code: Write readable, well-commented, and efficient code.
- Neglecting object-oriented concepts: OOP principles are fundamental in modern C++.
- Lacking familiarity with the STL: Utilize the power of ready-made data structures and algorithms.