25
AprFirst C++ Program and Its Syntax
31 Mar 2024
Beginner
1.95K Views
9 min read
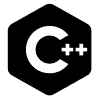
Learn via Video Course & by Doing Hands-on Labs
C++ Programming For Beginners
First C++ Program and its Syntax: An Overview
Are you excited to begin coding in C++? But, don't know where and how to start? Don't worry. All your questions will be answered in this article today. You will understand how to write a C++ program in a step-by-step manner. If you want to deepen your understanding of C++ programming skills, consider enrolling in our C++ Certification. In this C++ Tutorial, we'll learn the syntax and the meaning of each word or symbol in the C++ program.Begin your 1st C++ Program
- Open any text editor or IDE and create a new file with any name with a .cpp extension. e.g. helloworld.cpp
- Open the file and enter the below code in the C++ Compiler:
#include <iostream> using namespace std; int main() { cout << "Hello, World!" << endl; return 0; }
- Compile and run the code
Output
Hello, World!
Read More - C++ Interview Questions for Experienced
Syntax of C++
In the above section, you tried the very basic program of “Hello World” in C++. Did you understand the terms like iostream, cout written in the code? Don’t worry let us understand it one by one:#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
- In the very first line,
#include
signifies the header file. This header file is a library of C++ that helps the programmer with standard input-output objects. - In the next line, there is
using namespace std;
statement. It denotes that we can use names for objects and variables from the standard library. Thecout
object is defined inside the std namespace. - Then comes the
main()
function. It is the function due to which the program executes. Any C++ program without amain()
function cannot run. Only the code written within{}
executes during run time. cout
is an object that prints the string inside quotation marks" "
. It is followed by the insertion operator,<<
.- The
using namespace std;
line can be omitted and replaced with thestd
keyword, followed by the: :
operator for some objects as shown below in C++ Editor#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }
This is more preferred than using the statement,
using namespace std;
because it can create potential problems. - With the help of
cout
, our program printed the sentence “hello world” on the screen. There can be as manycout
statements as many you want in your program.#include <iostream> using namespace std; int main() { cout << "Hello, World!" << endl; cout << "This is my first program" << endl; return 0; }
Output
Hello, World! This is my first program
- The
endl
manipulator is used to insert a new line. That's why each output is displayed in a new line. - The
<<
operator can be used more than once if we want to print different variables, strings, and so on in a single statement. return 0
indicates the end of themain()
function.- The closing curly bracket
}
is used to end themain()
function. - Have you noticed
;
after each line of code in themain()
function? It is necessary to put it after each line of code. In C++ every line ends with a;
. If you forget to put a;
, it will show you a compile-time error. - C++ ignores white spaces but we use it to make the code more readable.
User Input in C++
In C++,cin
takes formatted input from standard input devices such as the keyboard. The cin
object is used along with the extraction operator, >>
for taking input.
Example of User Input in C++
#include <iostream>
using namespace std;
int main() {
int a;
cout << "Enter a number: ";
cin >> a; // Taking input
cout << "The number is: " << a;
return 0;
}
The above C++ code uses cin
to take an integer input from the user. The input is stored in the variable a
.
You will see variables and data types in the upcoming tutorials, Variables in C++ Programming and Data Types in C++
Output
Enter a number: 85
The number is: 85
- You can even use
std::cin
instead of cin if you don't want to use the statement,using namespace std;
.#include <iostream> int main() { int a; std::cout << "Enter a number: "; std::cin >> a; // Taking input std::cout << "The number is: " << a; return 0; }
- You can even take multiple inputs using a single
cin
statement#include <iostream> int main() { int a, b; std::cout << "Enter two numbers: "; std::cin >> a >> b; // Taking inputs std::cout << "The numbers are: " << a << "," << b; return 0; }
Output
Enter two numbers: 10 20
The numbers are: 10, 20