25
AprIdentifiers in C++: Differences between keywords and identifiers
18 Apr 2024
Beginner
4.6K Views
4 min read
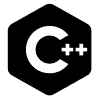
Learn via Video Course & by Doing Hands-on Labs
C++ Programming For Beginners
Identifiers in C++: Overview
Understanding how to useidentifiers
in C++ can be a daunting task for any beginner. If you're a beginner in programming struggling to understand identifiers
in C++, enroll in our C++ beginner course and get the guidance you need. Identifiers are essential concepts in C++. In this C++ tutorial, we'll look at understanding the common identifiers used in the C++ language, including their characteristics, examples, as well as important conventions.
What are Identifiers in C++?
Identifiers are names used to identify memory locations, functions in C++, and variables in C++. These names serve as labels to identify and access these elements within your C++ program.- It’s a collection of alphanumeric characters that begins with an alphabetical character or an underscore.
- As they must be unique, they are case-sensitive.
- There is a total of 63 alphanumerical characters that represent the identifiers.
Identifier Naming Rules in C++
- It can only consist of letters (both uppercase and lowercase), digits, and underscores.
- It must begin with a letter or an underscore. It cannot be a digit.
- They are case-sensitive, which means uppercase and lowercase letters are considered different characters. E.g., "myVar" and "myvar" are two different identifiers.
- They cannot be the same as any reserved words in the C++ language. Examples of reserved words in C++ include "if", "while", and "for".
- Identifiers cannot contain any spaces or special characters such as @, #, $, %, ^, &, *, etc.
Examples of Valid Identifiers
total, sum, average, _m _, sum_1
Example of Invalid Identifiers
2sum (starts with a numerical digit)
int (reserved word)
char (reserved word)
m+n (special character, i.e., '+')
Read More - Advanced C++ Interview Interview Questions and Answers
Types of Identifiers in C++
- Internal Identifiers
- They aren’t used in external linkage. Ex. local variables
- They are often used to assign significant meaning to portions of code that are shared between different functions in C++ language, classes, etc.
- They make it easier to maintain, extend, and debug code in C++.
- They discourage repetitive copy and paste of code between various program parts.
- Internal identifiers for variable & function names improve the efficiency and structure of C++ development, resulting in clear, succinct, and error-free code.
- External Identifiers
- Variables, constants, and functions that can be accessed outside of their scope in C++ are given distinctive names known as external identifiers.
- These identifiers are essential for larger programs with several files and libraries since they ensure organization and proper reference of items outside of their original scope.
- They are often written in lowercase and start with an underscore (_).
Differences between keywords and identifiers in C++
Identifiers | Keywords |
Identifiers are mainly a particular kind of name that identifies elements of that program by the programmers. | Keywords are mainly a particular type of word whose meaning is stored by compilers. |
It contains digits, letters, and underscore. | It contains letters only. |
The name of the variable can be identified by identifiers | The types of entities in the program are specified by Keywords |
The initial letter of the identifiers can be uppercase, lowercase, or underscore | The initial letter of the keyword is started in lowercase |
The only special character is used in identifiers are underscore | In keywords, there are no special characters |
There are two types of identifiers, those are internal and external identifiers | There are no classifications in keywords |
Examples of identifiers are, results, sum, test, power, and many more | Examples of keywords are, if, for, break, else, and many more |