18
JulLoop in C++: A Detailed Discussion with Examples
26 Jan 2025
Beginner
20.8K Views
13 min read
Loops in C ++: An Overview
Are you interested in programming but don't know where to start? Have you ever heard the term
loop
? Looping is one of the key concepts behind programming, and learning how to use loops
in C++ can open up a new world of code. Gain the foundational skills from this C++ tutorial and move to the advanced C++ Full Course Free that includes in-depth coverage of loops
and other essential programming concepts.What are loops in C++?
Loops
are a block of code that executes itself until the specified condition becomes false. In this section, we will look in detail at the types of loops
used in C++ programming.What is the need for Looping Statements in C++?
We can give some uses ofloops
in C++:Loops
allow the user to execute the same set of statements repeatedly without writing the same code multiple times.- It saves time and effort and increases the efficiency.
- It reduces the chance of getting errors during compilation.
Loop
makes the code readable and easier to understand, especially when dealing with complex logic or large data sets.- It promotes code reusability.
Loops
help traverse data structures like arrays or linked lists.
Read More - C++ Interview Questions Interview Questions for Freshers
Types of Loops in C++
There are three types of
loops
used in C++ programming:for
loop
while
loopdo while
loop
In this article, we will look at the C++ for
loop in detail. The other two loops will be covered subsequently after this.
1.) for loop in C++
Afor
loop is a control structure that enables a set of instructions to get executed for a specified number of iterations. It is an entry-controlled loop.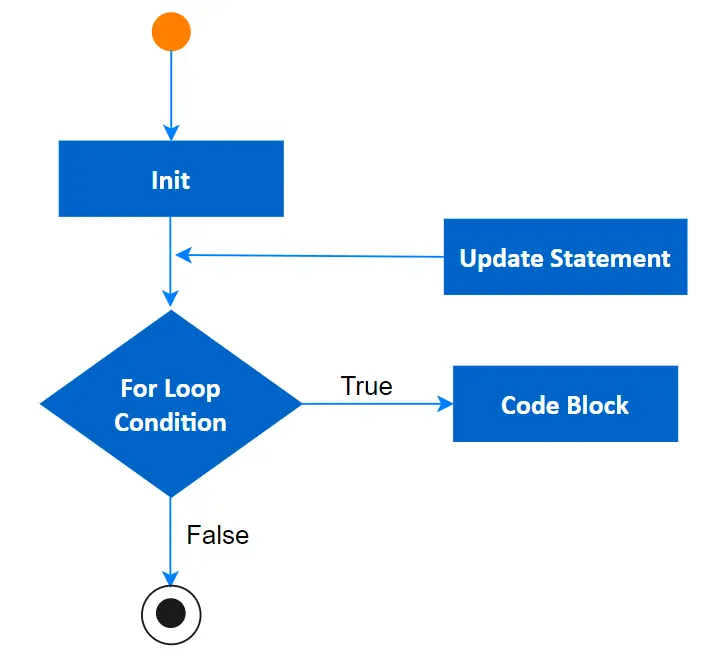
Syntax
for(initialization; testcondition; updateExpression)
{
//code to be executed
}
- Here, the
initialization
statement is executed first and only once. - The
testcondition
is checked, iffalse
the loop terminates - If the
testcondition
istrue
, the body of the loop executes - The
update expression
gets updated - Again the
testcondition
is evaluated - The process repeats until the
testcondition
becomesfalse
.
Example in C++ Compiler
// Program to print numbers from 1 to 10
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 10; i++) {
cout << i << endl;
}
return 0;
}
The above code prints the numbers from 1 to 10 using a
for
loop in C++.- We know it will take 10 iterations to print 10 numbers so, we have used the
for
loop. i
is initialized to 0.- The condition
i<10
will be checked. It is true, thereforei+1
i.e. 1 gets printed. - Then
i
increments to 1 again the condition,i<10
is evaluated. - The process will repeat until
i
become 10.
Output
1
2
3
4
5
6
7
8
9
10
2.) Nested for loop in C++
It is any type of loop inside a
for
loop.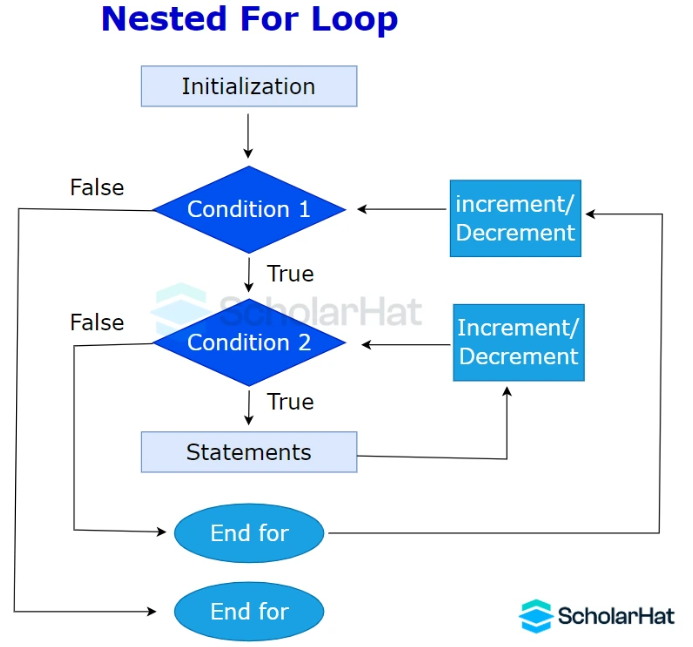
Example
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= i; j++) {
cout << j << " ";
}
cout << endl;
}
return 0;
}
- This C++ code generates a pattern of numbers using nested loops.
- The inner loop
j
iterates from 1 to the current value ofi
, printing numbers and spaces - The outer loop
i
iterates from 1 to 5, regulating the number of rows. - As a result, there is a pattern of numerals rising in each row, with a new line between each row.
Output
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
3.) Infinite for loop in C++
An infinitefor
loop gets created if the for
loop condition remains true
, or if the programmer forgets to apply the test condition/terminating statement
within the for
loop statement.Syntax
for(; ;)
{
// body of the for loop.
}
In the above syntax, there is no condition hence, this loop will execute infinite times.
Example
#include <iostream>
int main() {
for (;;) {
std::cout << "Hello World" << std::endl;
}
return 0;
}
In the above code, we have run the
for
loop infinite times, so "Hello World" will be displayed infinitely.Output
Hello World Hello World Hello World Hello World Hello World ...
4.) for-each loop/ range-based for loop
This is a new kind of loop introduced in theC++11
version. It is used exclusively to traverse through elements in an array
and vector
.Syntax
for (variableName : arrayName/vectorName) {
// code block to be executed
}
Here, for every value in the array
or vector
, the for
loop is executed and the value is assigned to the variable.
Example in C++ Editor
#include <iostream>
using namespace std;
int main() {
int num[7] = {10, 20, 30, 40, 50, 60, 70};
for (int i : num) {
cout << i << "\n";
}
return 0;
}
Output
10
20
30
40
50
60
70
Summary
In this tutorial, we looked at the
for
loop in C++ programming. We also saw infinite
and nested for
loop. Finally, we also discussed the new concept of for-each
loop. If you want to learn more about programming in C++, you can enrol in our C++ certification training or check out our website for tutorials on various topics.