16
MayStorage Classes in C++: Types of Storage Classes with Examples
Storage Classes in C++: An Overview
Have you ever wondered how storage classes
in C++ work together? In this blog post of C++ tutorial, we'll explore the ins and outs of this concept so that you can learn more about the powerful programming language. You'll come away with an understanding of how code structures work, what storage class
is all about, and a look into each of these storage classes along with their characteristics. Whether you're a beginner or an advanced user, by reading through this post, your understanding of C++ will be greatly increased!
To dive even deeper, you can enroll in a Free C++ Course. This course provides structured lessons and practical examples to master the intricacies of C++ programming, including storage classes.
What is Storage Class in C++ language?
Storage classes are keywords
used to define the scope (visibility) and lifetime (duration)of variables e.g. auto
, register
, and static
. They determine how memory is allocated and deallocated for variables in a C++ program.
Types of Storage Classes in C++ Programming
There are 6 types of storage classes in C++ programming:
1. Automatic Storage Class in C++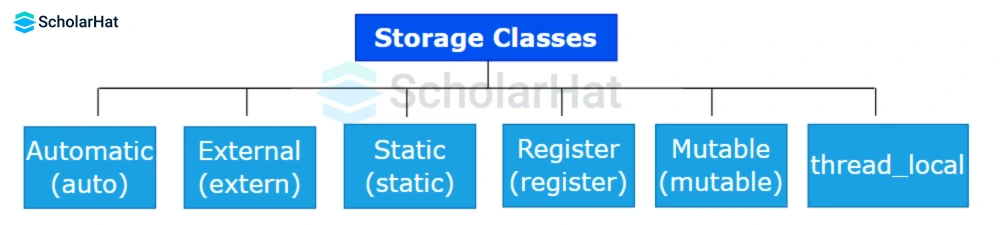
1. Automatic Storage Class in C++
- All the
local
variables areautomatic
variables by default. - This class enables the user to declare variables without specifying their type at run time.
- It also provides a way for C++ programmers to declare variables of a specific type, such as
int
,double
, andchar
. - Automatic Storage Class is so named because it allows the compiler to automatically assign space from the storage pool.
- It facilitates quicker memory access & logical data organization and boosts program clarity and effectiveness.
Example in C++ Compiler
#include <iostream>
#include <string> // Include the header for std::string
using namespace std;
void autoStorageClass()
{
cout << "Demonstrating auto class\n";
// Declaring an auto variable
int A = 45;
float B = 4.5;
const char* C = "Scholarhat";
char D = 'S';
// printing the auto variables
cout << A << " \n";
cout << B << " \n";
cout << C << " \n";
cout << D << " \n";
}
int main()
{
// To demonstrate auto Storage Class
autoStorageClass();
return 0;
}
The auto
storage class for variables is used in this C++ code. After the coming up of C++11
, auto
keyword's meaning has changed. Now the auto
keyword cannot be used to declare the auto
variables explicitly.
Output
Demonstrating auto class
45
4.5
Scholarhat
S
Read More - Advanced C++ Interview Interview Questions and Answers
2. Static Storage Class in C++
- A static variable is defined using the
static
keyword. - You can declare a
static
variable any number of times but, the value can be assigned only once. - The default value of this variable is
0
ornull
- The lifetime of a
static
variable initiates when the function is called and ends only when the program ends i.e. the value of astatic
variable persists till the end of the program. - The visibility of
static local variables
is limited to the function in which they are defined whereas forglobal
it is limited to the program in which they are declared.
Example
#include <iostream>
using namespace std;
// Function containing static variables
// memory is retained during execution
int staticFun()
{
cout << "For static variables: ";
static int count = 0;
count++;
return count;
}
// Function containing non-static variables
// memory is destroyed
int nonStaticFun()
{
cout << "For Non-Static variables: ";
int count = 0;
count++;
return count;
}
int main()
{
// Calling the static parts
cout << staticFun() << "\n";
cout << staticFun() << "\n";
// Calling the non-static parts
cout << nonStaticFun() << "\n";
cout << nonStaticFun() << "\n";
return 0;
}
The behavior of static
& non-static
variables within functions is demonstrated by the code. While non-static variables are reset in the nonStaticFun()
, static
variables maintain their values between calls. The program calls both functions to show the differences.
Output
For static variables: 1
For static variables: 2
For Non-Static variables: 1
For Non-Static variables: 1
3. Register Storage Class in C++
- The
register
keyword used with a variable instructs the compiler to store that variable within the microprocessor's high-speed registers instead of standard memory. - You can access a
register
variable faster than alocal
orautomatic
variable though both have the same functionality. - The default value is
0
. - No referencing which means
&
operator cannot be used with aregister
variable. - Modern compilers don’t require
register
variables for code optimization. - However, this keyword has been removed in
C++11
and should not be used.
Example
#include <iostream>
using namespace std;
void registerStorageClass()
{
cout << "Demonstrating register class\n";
// declaring a register variable
register char a = 'S';
// printing the register variable 'a'
cout << "Value of the variable 'a'"
<< " declared as register: " << a;
}
int main()
{
// To demonstrate register Storage Class
registerStorageClass();
return 0;
}
The register
storage class is used in this piece of C++ code for a variable a
. However, keep in mind that because current compilers are capable of automatically optimizing variable storage and access, they frequently ignore the register
keyword.
Output
Demonstrating register class
Value of the variable 'a' declared as register: S
4. External Storage Class in C++
- External or global variables are declared using the ‘
extern
’ keyword. - You can declare a
external
variable any number of times but their value can be assigned only once. - Their default value is
0
ornull
. - External variables specify an external linkage and so such variables are not allocated any memory.
- The initialization must be done globally and not within any function or block.
- If an external variable is not initialized anywhere in the program, the compiler will show an error.
Example
#include <iostream>
using namespace std;
// declaring the variable which is to
// be made extern an initial value can
// also be initialized to y
int y;
void externStorageClass()
{
cout << "Demonstrating extern class\n";
// telling the compiler that the variable
// y is an extern variable and has been
// defined elsewhere (above the main
// function)
extern int y;
// printing the extern variables 'y'
cout << "Value of the variable 'y'"
<< "declared, as extern: " << y << "\n";
// value of extern variable y modified
y = 3;
// printing the modified values of
// extern variables 'y'
cout
<< "Modified value of the variable 'y'"
<< " declared as extern: \n"
<< y;
}
int main()
{
// To demonstrate extern Storage Class
externStorageClass();
return 0;
}
This C++ code in C++ Editor explains how to define and access a variable y
using the extern
storage class. The variable 'y
' is declared as an extern, indicating that it can be used and updated inside the current scope even though it is defined elsewhere in the program (usually outside the main function).
Output
Demonstrating extern class
Value of the variable 'y' declared, as extern: 0
Modified value of the variable 'y' declared as extern:
3
5. Mutable Storage Class in C++
- A
mutable
storage Class in C++ programming is used for modifying one or more data members of astructure
/class
through aconstant
function. - This task can easily be promoted by mutable keywords which are particularly used to allow a particular data member of an object to be a Modifier.
Example
#include <iostream>
using std::cout;
class Test
{
public:
int a;
// defining mutable variable b
// now this can be modified
mutable int b;
Test()
{
a = 4;
b = 10;
}
};
int main()
{
// t1 is set to constant
const Test t1;
// trying to change the value
t1.b = 20;
cout << t1.b;
// Uncommenting below lines
// will throw error
// t1.a = 8;
// cout << t1.a;
return 0;
}
The C++ mutable
keyword is used in this code to show how it works. A member variable b
of the class may be changed even within a const
object, but efforts to change a non-mutable member (a) within a const object would cause a compilation error.
Read more: You will learn about classes and objects in the section, Object Oriented Programming (OOPs) Concepts in C++.
Output
20
6. thread_local Storage Class in C++
- The new storage class introduced in
C++11
is calledthread_local Storage Class
. - To define the object as
thread-local
, we can use thethread_local storage class
specifier. - The attributes of the
thread_local object
alter under the combination of thethread_local variable
with other storage specifiers, such asstatic
orextern
.
Example
#include <iostream>
#include <thread>
// Define a thread-local variable
thread_local int threadLocalValue = 0;
// Function that uses the thread-local variable
void ThreadFunction(int threadId) {
// Modify the thread-local variable
threadLocalValue += threadId;
// Print the thread-local variable
std::cout << "Thread " << threadId << ": threadLocalValue = " << threadLocalValue << std::endl;
}
int main() {
// Create two threads
std::thread t1(ThreadFunction, 1);
std::thread t2(ThreadFunction, 2);
// Wait for both threads to finish
t1.join();
t2.join();
// Since threadLocalValue is thread-local, each thread has its own copy
// The main thread's threadLocalValue is still 0
std::cout << "Main Thread: threadLocalValue = " << threadLocalValue << std::endl;
return 0;
}
The thread_local storage class is used in this example of C++ code to make a unique copy of the variable threadLocalValue
for each thread. The main thread keeps a separate copy of threadLocalValue
while threads t1
and t2
edit their own copies. This demonstrates how thread-local variables
preserve thread separation.
Output
Thread 1: threadLocalValue = 1
Thread 2: threadLocalValue = 2
Main Thread: threadLocalValue = 0
FAQs
1. What are storage classes with an example of Storage class in C++?
The memory allocation, scope, & lifetime of variables are described by storage classes in C and C++. Auto, static, extern, register, and mutable are a few examples.
2. What is a Storage class in C++?
A storage class in C++ establishes the scope, linkage, and duration of a variable's storage. It manages the placement and organization of variables in memory.
3. Why storage classes are used?
To manage memory effectively and specify variable behavior, storage classes are used to regulate the lifetime & visibility of variables in a program.
4. What are the 3 general classes of storage?
Automatic storage, such as local variables, static storage, such as global variables, and dynamic storage, such as heap-allocated variables, are the three general forms of storage.
5. How many storage classes are there in C++ and C?
There are five storage classes in C++ and C: auto, static, extern, register, & mutable.
Summary
Storage class defines the scope and lifetime of variables and/or functions within a C++ training program. In this article, we looked at how storage classes help define variables' scope and lifetime. We also saw how automatic, register, static, mutable, and extern storage classes work with examples. They allow you to control the visibility and lifetime of variables, which can be very useful in larger programs. Thanks for reading! I hope this article helped understand these two concepts.