25
AprUnderstanding Boxing and Unboxing in C#
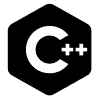
C++ Programming For Beginners
Boxing and Unboxing: An Overview
Boxing and unboxing are the most important concepts you always get asked in your interviews. Actually, it's really easy to understand and simply refers to the allocation of a value type (e.g. int, char, etc.) on the heap rather than the stack.
While working with data types such as strings, objects, and arrays, we often need to convert the value types to the reference types or vice-versa. Because both have different characteristics and .NET stores them differently in the memory location, This must be required to do some work or define some settings that work internally to convert them from one data type to another. These conversion processes are generally called as a terms boxing and unboxing. In this C# Tutorial, We will explore about boxing and unboxing which includes, What is boxing in C#, What is unboxing in C#, Difference between boxing and unboxing in C#.
What is Boxing?
- Implicit conversion of a value type (int, char, etc.) to a reference type (object), is known as Boxing.
- In the boxing process, a value type is allocated on the heap rather than the stack.
- A boxing conversion is intended for creating a copy of the value being boxed. This type of conversion is completely different from a conversion of a reference type to a type object, in which the value that is being converted continues to reference the same instance and simply is regarded as the less derived type object.
Syntax
Declare one variable with its value
int z = 222;
boxing operation
object x= z;
Here Boxing copies the value of z into object x. Let's elaborate on this in C# Compiler
Example of Boxing
class TestBoxing
{
static void Main()
{
int z = 222;
object x = z;
z = 444;
System.Console.WriteLine("The value-type value = {0}", z);
System.Console.WriteLine("The object-type value = {0}", x);
}
}
Output
The value-type value = 444.The object-type value=222
Explanation
At first, we initialized one variable"z" along with its value. Then we did a boxing operation and assigned that variable to one object. After that, we gave another value to "z".But because of boxing, the change in "z" doesn't affect the value stored in x.What is Unboxing?
- Explicit conversion of the same reference type (which is being created by the boxing process)
- back to a value type is known as unboxing.
- In the unboxing process, the boxed value type is unboxed from the heap and assigned to a value type that is being allocated on the stack.
- In other words, The unboxing conversion is a completely reversed process of boxing that we have seen already.
- Unboxing is the process of converting a reference data type to a value type such as a string or integer. The unboxing conversion extracts the value from the reference type and assigns it to a value type.
Syntax
// int (value type) is created on the Stack
int stackVar = 12;
// Boxing = int is created on the Heap (reference type)
object boxedVar = stackVar;
// Unboxing = boxed int is unboxed from the heap and assigned to an int stack variable
int unBoxed = (int)boxedVar;
Example of Unboxing
int i = 10;
ArrayList arrlst = new ArrayList();
//ArrayList contains object type value
//So, int i is being created on heap
arrlst.Add(i); // Boxing occurs automatically
int j = (int)arrlst[0]; // Unboxing occurs
Note
Sometimes boxing is necessary, but you should avoid it if possible since it will slow down the performance and increase memory requirements.
For example, when a value type is boxed, a new reference type is created and the value is copied from the value type to the newly created reference type. This process takes time and requires extra memory (around twice the memory of the original value type).
Attempting to unbox a null causes a NullReferenceException.
int? stackVar = null; // Boxing= Integer is created on the Heap object boxedVar = stackVar; // NullReferenceException int unBoxed = (int)boxedVar; //Object reference not set to an instance of an object.
Attempting to unbox a reference to an incompatible value type causes an InvalidCastException.
int stackVar = 12; // Boxing= Integer is created on the Heap object boxedVar = stackVar; // InvalidCastException float unBoxed = (float)boxedVar; //Specified cast is not valid.
Difference Between Boxing and Unboxing in C#
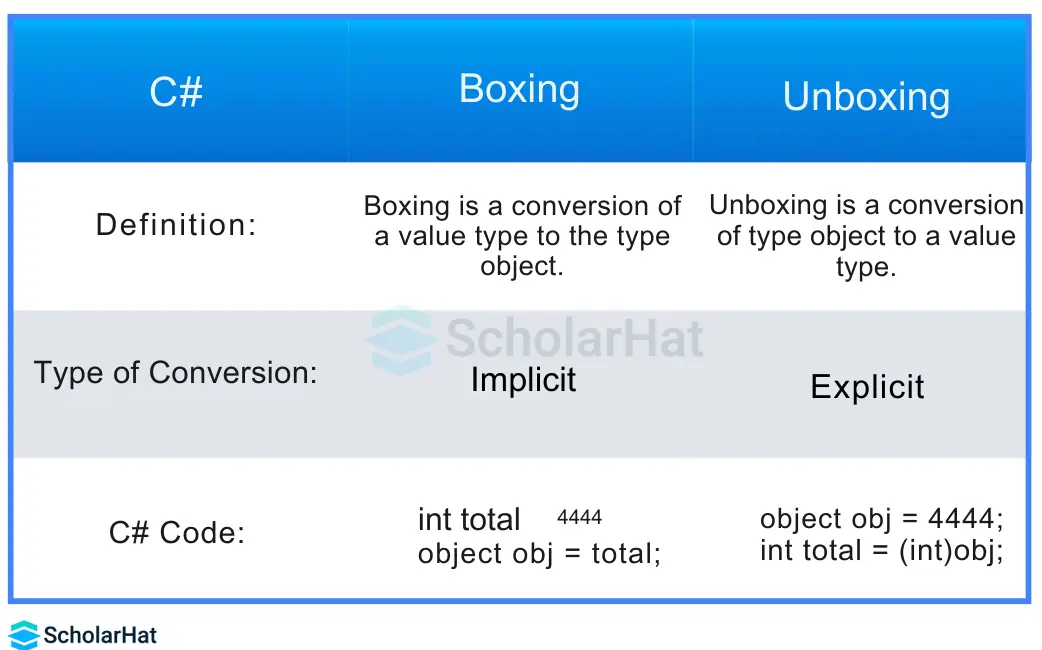
Conclusion:
The boxing and unboxing type system unification provides the value types with the benefits of object characteristics without introducing the unnecessary overhead of the type conversions. I hope you will enjoy the tips while programming with C#. I would like to have feedback from my blog readers. Your valuable feedback, questions, or comments about this article are always welcome. Also, consider our C# Programming Course for a better understanding of C# concepts.