25
AprC# Developer Roadmap
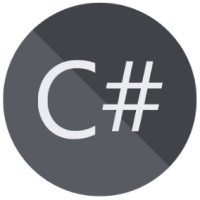
C# Programming For Beginners Free Course
C# Developer Roadmap: An Overview
C# is a modern, object-oriented programming language developed by Microsoft. It was first introduced in 2002 as part of Microsoft’s .NET framework. It is a simple, powerful, and type-safe programming language used to build desktop, web, game, and mobile applications. It supports both static and dynamic typing.
In this C# tutorial, we will look at the C# roadmap for aspiring .NET/C# developers.
Characteristics and Advantages of C#
- Fast: C# is a fast programming language that executes quickly.
- Simple: C# doesn’t require you to include header files — it has a structured approach that allows you to break the problem into parts and has a rich set of libraries and data types.
- Object-Oriented: C# supports OOPS concepts like inheritance, polymorphism, abstraction, encapsulation, interfaces, etc. that make maintenance and development easier.
- Type-Safe: It allows you to write and develop type-safe code, improving security. You will not be able to perform unsafe casts, such as converting a double data type to a boolean. It automatically initializes data types and objects as well as classes to zero or null values.
- Interoperability: It can interact well with other programming languages. It increases the reusability of the code and creates an efficient program. It can use COM objects irrespective of the language that’s used to write them.
- Structured: C# divides a large program into modules, procedures, or functions to solve specific problems.
- Component-Oriented: C# is a component-oriented programming language, meaning it uses events, properties, attributes, methods, etc., to allow and create self-contained and described components called functionalities.
- Platform-Independent: Any platform that has .NET installed on it can run C#.
- Automatic Garbage Collection: The system does not get messed up or hung during execution because C# has an automatic garbage collection that automatically removes dangling items during runtime.
- No Memory Leak: It has a strong and high memory backup that helps avoid memory leakage, unlike C++.
Why one must Learn C# in 2024?
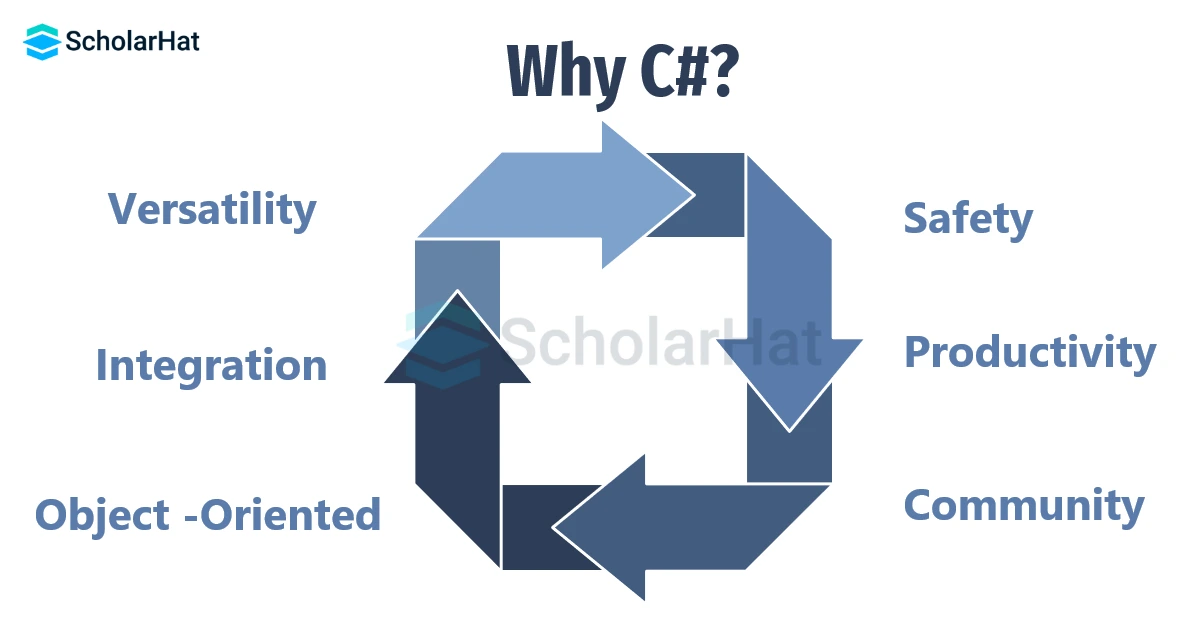
Following are some of the reasons to learn C# in 2024
- It’s simple, easy to use, intuitive, and readable.
- It’s a strongly typed language that enhances developer productivity and makes common tasks easy to do.
- It’s a multi-paradigm, object-oriented programming language that supports programming styles such as generic, imperative, functional, and declarative.
- You can create multiple applications such as console applications, desktop applications, and applications for native mobile, AI, IoT, and cloud.
- C# runs on a well-engineered, robust, solid .NET framework.
- It’s platform-independent, modern, mature, and very actively developed and maintained.
- It’s open-source, well-documented, and has an active, vibrant community.
- It can be used for software development since it has built-in design practices and patterns.
- It is very fast to execute and has a rich set of libraries.
Read More: Introduction to C#: A Beginner's Guide
Prerequisites for Learning C#
To learn any new language, the programming fundamentals must be clear. If you are good in at least a single language, your basics of programming are sufficient to learn C#.
- Basic computer knowledge such as working with command-line, file systems, and installing applications such as compilers, interpreters, etc.
- Knowledge of object-oriented programming because C# is an object-oriented programming language. You must have a basic understanding of concepts like encapsulation, polymorphism, abstraction, inheritance, interfaces, etc.
- Basic knowledge of languages like C, C++, or Java so that you can write the C# syntax correctly.
Integrated Development Environments (IDE) for C#
An IDE or Integrated Development Environment provides us with important tools like an editor, debugger, auto-complete tools, automation facilities, extensions, plugins, etc., that help you create a perfect environment for development.
Let us now discuss some of the top IDEs that you can use to debug and execute C# programs.
1. Visual Studio Code
VS Code is one of the most popular IDE for C#. C# and Visual Studio are both Microsoft products and are perfectly aligned to work with each other. It has built-in support for .Net and C# and has tons of plugins to make development easier. It has both a free and paid version, but it’s best to start with the free community edition for individual purposes.
Features of Visual Studio Code
- Automatic code completion with IntelliSense.
- Built-in Git commands.
- Tons of extensions to customize the environment.
- Free and easy to install and use.
2. Project Rider
Project Rider is a cross-platform IDE that supports .NET, .NET Core, and .NET Framework. It supports languages such as C#, HTML, XAML, JS, and more. It is based on IntelliJ and Resharper and comes with navigation, unit testing, debugging, Nuget clients, database integration, etc.
Features of Project Rider
- It has a Java-based platform for C# and supports the latest versions of C#.
- It has Unity integration.
- It performs debugging in a docker container and has a spell checker integrated.
How to become a C# Developer - The Complete Roadmap
Now let us look in detail at the learning path for understanding the ever-evolving and versatile C# language
1. Syntaxes, Variables, and Data Types
You might know that learning the syntax is the basic step to getting started with any programming language. Therefore you must start with the syntax of C# at first. You must get a grip on learning how to declare variables, the different data types, comments, input-output, type-conversion, etc.
Learn more: Keywords in C#, Identifiers in C#, Variables in C#, Data Types in C#
2. Conditionals and Loops
The next step towards learning C# is to learn branching and looping statements. Loops help you create a program that can automate repetitive tasks instead of running the same code manually multiple times. Conditionals help you to give a flow to the program. The following are included in this topic:
- If/Else conditions in C#
- Switch statements
- Comparison operators in C#
- Logical operators in C#
- For loops
- For-each loops
3. Functions/Methods
Functions, methods, or procedures make us perform a specific function by writing a piece of code. To perform a specific operation multiple times, the same code could be used multiple times in different program sections. The following are the topics you need to learn in this topic:
- Defining and calling methods
- Parameters and arguments in methods
- Method overloading in C#
4. Classes and Objects
These are a part of OOPS concepts. Classes encapsulate related data together, and objects provide instances to access data stored inside classes. This topic includes the following:
- Classes
- Objects
- Access modifiers
- Fields
- Properties
- Constructors
- “this” keyword
- Constructor overloading
- Object dot notation
Read More - C# Interview Questions And Answers
5. Interfaces and Inheritance
Inheritance allows you to inherit functions, and variables of one or more classes, to another. Interfaces allow you to create classes like structures where you don’t need to define the methods, just declare them. At a later stage, when you require it, you can define these methods in their subclasses. This topic includes the following:
- Building interfaces
- Parent/child classes
- Accessing inherited components
- Method overriding in C#
6. Partial Class, Static Class, and Sealed Class
Partial Class
- Understand the role of partial classes in splitting a class definition across multiple files.
- Learn how these help in managing large codebases.
- Understand scenarios where partial methods are useful.
Static Class
- Grasp the concept of static classes and their role in C#. Learn to organize code without creating instances.
- Understand how to declare and use static fields within a static class.
Sealed Class
- Learn sealed classes to prevent further inheritance. Understand the use of ‘sealed’ to prevent method overriding.
7. C# Properties and Indexer
Properties
- Understand the role of properties in providing access to object states.
- Learn how properties are an alternative to public fields. Understand the syntax for declaring properties in C#. Explore the use of get and set accessors.
- Creating Read-Only and Write-Only Properties.
Indexers
- Understand the role of indexers in providing array-like access to objects.
- Learn how indexers are defined using this keyword. Explore the syntax for declaring indexers in C#.
8. C# Exception Handling and Attributes
Exception Handling
- Learn try-catch blocks for handling exceptions. Learn the applications of the finally block.
- Learn how to throw exceptions using the throw keyword. Explore when to create custom exception classes and how to use them.
Attributes
- Learn how attributes enhance the behavior of code elements like classes, methods, properties, etc.
- Explore common built-in attributes like Serializable, Obsolete, and DllImport, etc.
- Learn to create custom attributes and use them.
9. Build C# Projects
To practice the learned concepts, try to make projects like:
- Console Calculator: A basic calculator application to perform simple arithmetic operations like addition, subtraction, multiplication, and division.
- To-Do List App: A simple application to manage a to-do list. Users can add tasks, view the list of tasks, mark tasks as completed, and delete tasks.
- Weather App: A console application to fetch and display weather data from a weather API in a text-based presentation.
- Contact Book: A console application for managing a personal contact book. Users can add, view, edit, and delete contact information.
10. Staying Up-to-Date with C# and the Developer Community
It's very important to stay up-to-date with the latest trends, best practices, and technologies in the C# ecosystem as we know that this technology is constantly evolving. For that:
- Follow the official C# Blog
- Attending conferences, meetups, and webinars
- Participating in online forums and communities (Stack Overflow, Reddit, GitHub)
- Following industry leaders and influencers on social media
- Regularly reading books, articles, and tutorials on C# topics
Summary
This was all about the detailed procedure towards starting your journey in the direction of becoming a C# Developer in 2024. For practicing the learned concepts, consider our C# Certification Training.
FAQs
Q1. List out some features of C#.
- Fast
- Simple
- Object-Oriented
- Type-Safe
- Interoperability
Q2. What are the IDEs for executing C# programs.
Q3. What are some of the prerequisites for learning C#?
Q4. What is meant by partial class in C#?
Q5. What are interfaces in C#?
Take our free csharp skill challenge to evaluate your skill
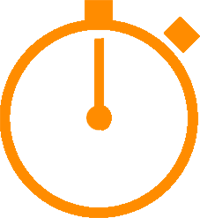
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.