25
AprIdentifiers in C# - A Beginner's Guide ( With Examples )
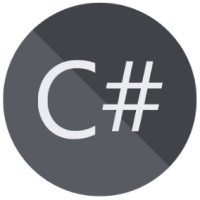
C# Programming For Beginners Free Course
Identifiers in C#: An Overview
Identifiers in C# serve as names for various program elements like variables, methods, and classes. Understanding their rules and conventions is crucial for writing clean and readable code. This article explores the significance of identifiers and their best practices in C# programming.
What is an Identifier in C#?
In C#, an identifier is a user-defined name used to represent variables, methods, classes, interfaces, or other program entities. Identifiers must start with a letter or underscore, followed by letters, digits, or underscores, and cannot be a reserved keyword. Identifiers are case-sensitive and enhance code readability.
Read More - C Sharp Interview Questions
Rules of Naming Identifiers in C#
- Start with a Letter or Underscore: Identifiers must begin with a letter (A-Z, a-z) or an underscore (_).
- Subsequent Characters: Following the initial character, identifiers can include letters, digits (0-9), and underscores (_).
- Case Sensitivity: C# is case-sensitive; uppercase and lowercase letters are considered distinct. For
example,
variableName
andVariableName
are different identifiers. - Keywords: Identifiers cannot be a reserved keyword; they have specific meanings in the C# language and cannot be used as identifiers.
- Length: There is no specific length limit for identifiers, but it's a good practice to keep them reasonably short and descriptive for readability.
- Unicode Characters: C# supports Unicode characters, allowing identifiers to include characters from various languages and symbols.
- Meaningful and Descriptive: Identifiers should be meaningful and descriptive, reflecting the purpose or content they represent, and enhancing code readability and maintainability.
- Camel Case: It's a common convention to use camel case for identifiers (e.g.,
myVariableName
) to improve readability. Classes often use Pascal case (e.g.,MyClassName
) for naming. - Avoid Special Characters: Special characters like @, $, and % are not allowed in identifiers.
- Consistency: Maintain a consistent naming convention throughout the codebase to enhance collaboration and understanding among developers.
Example
using System;
namespace IdentifierExample
{
class Program
{
static void Main(string[] args)
{
// Variable identifiers
int myVariable = 42;
string myString = "Hello, World!";
// Method identifiers
SayHello();
int sum = AddNumbers(5, 7);
// Class identifiers
MyClass myObject = new MyClass();
myObject.PrintMessage();
// Namespace identifier
System.Console.WriteLine("Using System namespace");
Console.WriteLine($"Variable identifier: {myVariable}");
Console.WriteLine($"String identifier: {myString}");
Console.WriteLine($"Method identifier: Sum = {sum}");
}
// Method identifiers
static void SayHello()
{
Console.WriteLine("Hello from SayHello() method!");
}
static int AddNumbers(int a, int b)
{
return a + b;
}
// Class identifier
class MyClass
{
public void PrintMessage()
{
Console.WriteLine("Printing a message from MyClass.");
}
}
}
}
Explanation
This C# program in the C# Compiler demonstrates the usage of different types of identifiers in C#. It includes variable identifiers (myVariable, myString), method identifiers (SayHello, AddNumbers), a class identifier (MyClass), and a namespace identifier (System). The program invokes methods, creates objects, and displays output to showcase these identifiers' usage.
Output
Hello from SayHello() method!
Printing a message from MyClass.
Using System namespace
Variable identifier: 42
String identifier: Hello, World!
Method identifier: Sum = 12
Conclusion
In conclusion, identifiers in C# are crucial elements used to name various program entities. They play a vital role in making code readable, maintainable, and self-explanatory. Choosing meaningful and consistent identifiers is a fundamental practice for writing clean and effective C# code.
Take our free csharp skill challenge to evaluate your skill
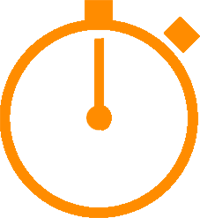
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.