11
JulKeywords in C#: Types of Keywords with Examples
Keywords in C#: An Overview
In this comprehensive guide, we will explore the power of keywords in C#, one of the most popular programming languages used today. To enhance your knowledge and apply these best practices effectively, you can enroll in a C Sharp Online Course Free. This course will provide you with a deeper understanding of C# programming, including the proper use of identifiers and other essential concepts.
What are Keywords in C#?
Keywords are predefined sets of reserved words that have special meaning in a program. The meaning of keywords can not be changed, nor can they be directly used as identifiers in a program.
Keywords serve as building blocks for constructing statements, defining types, controlling program flow, and implementing various programming constructs.
Example
char studentName;
Here, “studentName
” is a variable (identifier) and long is a keyword. In C#, the word "char
" has a special meaning; it is used to declare variables of the type character and has a fixed function.
char, int, long, and other keywords cannot be used as identifiers. We, therefore, cannot have the following:
char char;
Read More - C# Interview Questions For Freshers
Reserved Keywords in C#
If the question is how many reserved keywords are in c# then the answer is, that there are a total of 77 reserved keywords. These keywords are predefined and have a specific meaning in the language.
abstract | base | as | bool | break | catch | case |
byte | char | checked | class | const | continue | decimal |
private | protected | public | return | readonly | ref | sbyte |
explicit | extern | false | finally | fixed | float | for |
foreach | goto | if | implicit | in | in (generic modifier) | int |
ulong | ushort | unchecked | using | unsafe | virtual | void |
null | object | operator | out | out (generic modifier) | override | params |
default | delegate | do | double | else | enum | event |
sealed | short | sizeof | stackalloc | static | string | struct |
switch | this | throw | true | try | typeof | uint |
abstract | base | as | bool | break | catch | case |
volatile | while |
Example
using System;
class Scholarhat
{
static public void Main ()
{
int a = 10;
Console.WriteLine("The value of a is: {0}",a);
// this is not a valid identifier
// removing comment will give compile time error
// double int = 10;
}
}
Explanation
This C# code in the C# Compiler defines a class named "Scholarhat" with a static "Main" method. It declares an integer variable "a" with a value of 10 and then prints its value. There's a commented-out line attempting to declare a variable named "int," which is not a valid identifier, so it's commented out to avoid a compile-time error.
Output
The value of a is: 10
Types of different keywords
Keywords are divided into 10 types, those are:
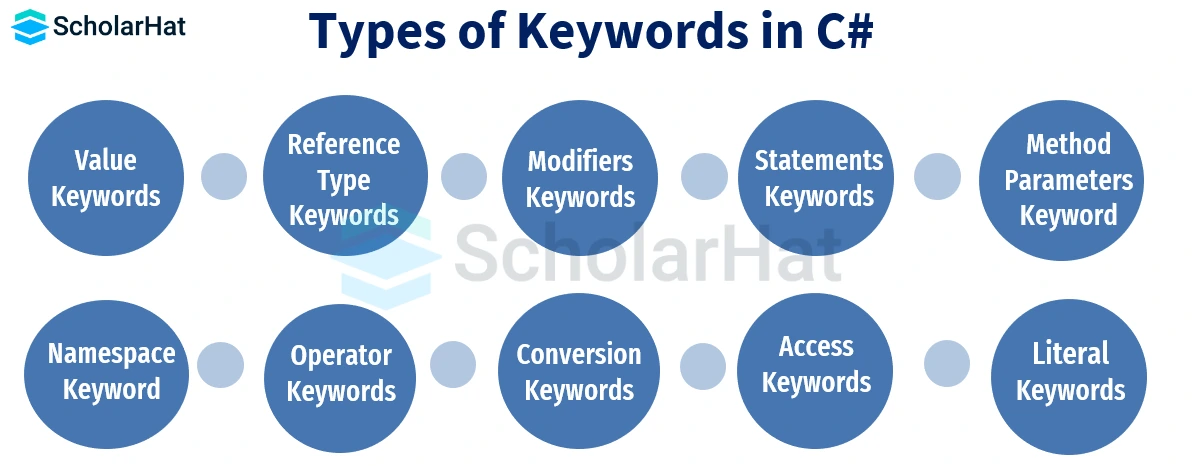
Value keywords
In C#, keywords are reserved words that have special meanings and are used to define the syntax and structure of the language. The following are the value type keywords in C#:
bool | byte | char | decimal |
double | enum | float | int |
long | sbyte | short | struct |
unit | ulong | ushort |
Example
using System;
class DNT
{
static public void Main ()
{
byte a = 47;
Console.WriteLine("The value of a is: {0}",a);
bool b = true;
Console.WriteLine("The value of b is: {0}",b);
}
}
Explanation
This C# code defines a class named "DNT" with a static "Main" method. It declares a byte variable "a" with a value of 47 and a boolean variable "b" with a value of true. It then prints the values of these variables to the console.
Output
The value of a is: 47
The value of b is: True
Read More - C# Developer Roadmap
Reference Type Keywords
Reference types have six keywords that are used to store references to data or objects. The terms “class, delegate, interface, object, string, and void” fall under this category.
Example in C# Online Compiler
using System;
class Program
{
static void Main()
{
// Example 1: Classes (Reference Type)
Person person1 = new Person("John", 25);
Person person2 = person1; // Reference to the same object
person1.Name = "James"; // Modifying object through one reference
Console.WriteLine("Example 1:");
Console.WriteLine($"Person 1: Name - {person1.Name}, Age - {person1.Age}");
Console.WriteLine($"Person 2: Name - {person2.Name}, Age - {person2.Age}");
// Example 2: Arrays (Reference Type)
int[] arr1 = { 1, 2, 3 };
int[] arr2 = arr1; // Reference to the same array
arr1[0] = 10; // Modifying array through one reference
Console.WriteLine("\nExample 2:");
Console.WriteLine($"Array 1: [{string.Join(", ", arr1)}]");
Console.WriteLine($"Array 2: [{string.Join(", ", arr2)}]");
}
}
class Person
{
public string Name { get; set; }
public int Age { get; set; }
public Person(string name, int age)
{
Name = name;
Age = age;
}
}
Explanation
This C# code demonstrates reference types. In Example 1, two Person objects share the same reference, so modifying one affects the other. In Example 2, two arrays share the same reference, so changes to one array are reflected in the other. Reference types store references to data in memory, allowing multiple variables to refer to the same data.
Output
Example 1:
Person 1: Name - James, Age - 25
Person 2: Name - James, Age - 25
Example 2:
Array 1: [10, 2, 3]
Array 2: [10, 2, 3]
Modifiers Keywords
17 keywords in modifiers can be used to change member-type declarations.
public | private | internal | protected | abstract |
const | event | extern | new | override |
partial | readonly | sealed | static | unsafe |
virtual | volatile |
Example
using System;
class Scholar
{
class Mod
{
public int n1;
}
static void Main(string[] args)
{
Mod obj1 = new Mod();
obj1.n1 = 77;
Console.WriteLine("Value of n1: {0}", obj1.n1);
}
}
Explanation
This C# code defines a class "Scholar" containing a nested class "Mod" with a public integer field "n1." In the Main method, it creates an instance of "Mod" called "obj1" and assigns a value of 77 to its "n1" field. Then, it prints the value of "n1" to the console.
Output
Value of n1: 77
Statements Keywords
In all, 18 different keywords are employed in program instructions.
if | else | switch | do | for |
foreach | in | while | break | continue |
goto | return | throw | try | catch |
finally | checked | unchecked |
Example
using System;
class demoContinue
{
public static void Main()
{
for(int i = 1; i < 3; i++)
{
if(i == 2)
continue;
Console.WriteLine("Scholar Hat");
}
}
}
Explanation
This C# code in the C# Editor defines a class "demoContinue" with a Main method. It uses a for loop to iterate from 1 to 2. Inside the loop, when the value of "i" is 2, it encounters a "continue" statement, which skips the rest of the loop body for that iteration. As a result, "Scholar Hat" is printed only once.
Output
Scholar Hat
Method Parameters Keywords
There are a total of 4 keywords that can be used to alter how a method's parameters behave. The words "params," "in," "ref," and "out" are included in this category.
Example
using System;
class Program
{
static void ModifyValue(ref int x)
{
x = x * 2;
}
static void Main()
{
int number = 5;
Console.WriteLine("Before calling ModifyValue: " + number);
ModifyValue(ref number);
Console.WriteLine("After calling ModifyValue: " + number);
}
}
Explanation
This C# code defines a program that demonstrates the use of the ref keyword for passing a variable by reference. It declares a method ModifyValue that doubles the value of an integer passed to it. In the Main method, a variable number is modified by ModifyValue, resulting in the output of the original and updated values.
Output
Before calling ModifyValue: 5
After calling ModifyValue: 10
Namespace Keywords
There are 3 keywords in total in this category that are namespace-related. The keywords include extern, namespace, and using.
Example
using System;
namespace MyNamespace
{
class Program
{
static void Main()
{
Console.WriteLine("Hello from MyNamespace!");
}
}
}
namespace AnotherNamespace
{
class Program
{
static void Main()
{
Console.WriteLine("Hello from AnotherNamespace!");
}
}
}
class Program
{
static void Main()
{
Console.WriteLine("Hello from the global namespace!");
}
}
Explanation
This C# code demonstrates the use of namespaces. It defines three namespaces: MyNamespace, AnotherNamespace, and the global namespace. Each namespace contains a Program class with its own Main method that prints a unique message. When executed, you can choose which Main method to call, resulting in different output messages.
Output
Hello from MyNamespace!
Hello from AnotherNamespace!
Hello from the global namespace!
Operator Keywords
There are a total of 8 keywords that can be used for a variety of tasks, including constructing objects and determining an object's size. Key words include "as is," "new," "sizeof," "typeof," "true," and "stackalloc."
Example
using System;
class Complex
{
public double Real { get; set; }
public double Imaginary { get; set; }
public Complex(double real, double imaginary)
{
Real = real;
Imaginary = imaginary;
}
// Overload the + operator
public static Complex operator +(Complex c1, Complex c2)
{
return new Complex(c1.Real + c2.Real, c1.Imaginary + c2.Imaginary);
}
public override string ToString()
{
return $"{Real} + {Imaginary}i";
}
}
class Program
{
static void Main()
{
Complex num1 = new Complex(1, 2);
Complex num2 = new Complex(3, 4);
Complex sum = num1 + num2;
Console.WriteLine("Sum: " + sum);
}
}
Explanation
In this example, we define a Complex class representing complex numbers. We overload the + operator using the operator keyword to enable the addition of two Complex objects. The Main method creates two complex numbers, adds them using the overloaded + operator, and prints the result. The code demonstrates how to use the operator keyword to define custom behaviors for operators like + for user-defined types.
Output
Sum: 4 + 6i
using System;
class Temperature
{
private double Celsius;
public Temperature(double celsius)
{
Celsius = celsius;
}
// Implicit conversion from Celsius to Fahrenheit
public static implicit operator Fahrenheit(Temperature temperature)
{
double fahrenheit = (temperature.Celsius * 9 / 5) + 32;
return new Fahrenheit(fahrenheit);
}
// Explicit conversion from Celsius to Kelvin
public static explicit operator Kelvin(Temperature temperature)
{
double kelvin = temperature.Celsius + 273.15;
return new Kelvin(kelvin);
}
public override string ToString()
{
return $"{Celsius}°C";
}
}
class Fahrenheit
{
public double Value { get; private set; }
public Fahrenheit(double value)
{
Value = value;
}
public override string ToString()
{
return $"{Value}°F";
}
}
class Kelvin
{
public double Value { get; private set; }
public Kelvin(double value)
{
Value = value;
}
public override string ToString()
{
return $"{Value}K";
}
}
class Program
{
static void Main()
{
Temperature celsiusTemp = new Temperature(25);
// Implicit conversion from Celsius to Fahrenheit
Fahrenheit fahrenheitTemp = celsiusTemp;
// Explicit conversion from Celsius to Kelvin
Kelvin kelvinTemp = (Kelvin)celsiusTemp;
Console.WriteLine($"Celsius: {celsiusTemp}");
Console.WriteLine($"Fahrenheit: {fahrenheitTemp}");
Console.WriteLine($"Kelvin: {kelvinTemp}");
}
}
Celsius: 25°C
Fahrenheit: 77°F
Kelvin: 298.15K
Access Keywords
The class or instance of the class can be accessed and referenced using 2 keywords. “Base” and “This” are the keywords.
Example
using System;
// Public access modifier (accessible from any code)
public class PublicClass
{
public void PublicMethod()
{
Console.WriteLine("This is a public method.");
}
}
// Internal access modifier (accessible within the same assembly)
internal class InternalClass
{
internal void InternalMethod()
{
Console.WriteLine("This is an internal method.");
}
}
// Private access modifier (accessible only within the same class)
public class AccessExample
{
private int privateField = 42;
private void PrivateMethod()
{
Console.WriteLine("This is a private method.");
}
public void AccessPrivate()
{
Console.WriteLine($"Accessing private field: {privateField}");
PrivateMethod();
}
}
class Program
{
static void Main()
{
PublicClass publicObj = new PublicClass();
publicObj.PublicMethod();
InternalClass internalObj = new InternalClass();
internalObj.InternalMethod();
AccessExample accessExample = new AccessExample();
accessExample.AccessPrivate();
}
}
Explanation
This C# code in the C# Playground demonstrates access modifiers. It defines three classes: PublicClass with public access, InternalClass with internal access, and AccessExample with private members. The Main method showcases access levels by creating instances and invoking methods, showing how public, internal, and private access works.
Output
This is a public method.
This is an internal method.
Accessing private field: 42
This is a private method.
Literal Keywords
Two keywords are used as literal or constant expressions. The keywords are default and null.
Example
using System;
class Program
{
static void Main()
{
bool isRaining = true;
bool isSunny = false;
Console.WriteLine("Is it raining? " + isRaining);
Console.WriteLine("Is it sunny? " + isSunny);
int apples = 5;
int oranges = 3;
Console.WriteLine("I have " + apples + " apples.");
Console.WriteLine("I have " + oranges + " oranges.");
}
}
Explanation
This C# code demonstrates the use of literal keywords. It initializes Boolean variables isRaining and isSunny, and integer variables apples and oranges. It then prints messages indicating weather conditions and fruit quantities using the literal keywords true, false, and numeric literals.
Output
Is it raining? True
Is it sunny? False
I have 5 apples.
I have 3 oranges.
Contextual keywords in C#
These are employed in the program to provide a particular meaning. In C#, new keywords are added to the contextual keywords list rather than the keyword category. This reduces the likelihood that programs created in earlier versions may crash.
Important Information:
These words are not restricted.
Contextual keywords were chosen because they can also be used as identifiers outside of the context.
In two or more situations, they can mean distinct things.
In C#, there are a total of 30 contextual keywords.
Example
using System;
public class Student
{
private string name = "DotNet Tricks";
public string Name
{
get
{
return name;
}
set
{
name = value;
}
}
}
class TestStudent
{
public static void Main(string[] args)
{
Student s = new Student();
s.Name = "DNT";
Console.WriteLine("Name: " + s.Name);
int get = 50;
int set = 70;
Console.WriteLine("Value of get is: {0}",get);
Console.WriteLine("Value of set is: {0}",set);
}
}
Explanation
This C# code defines a "Student" class with a private field "name" and a property "Name" to access it. In the "TestStudent" class, it creates a "Student" object, sets its "Name" property to "DNT," and prints the result. It also declares and prints the values of "get" and "set," which are unrelated to the "Student" class.
Output
Name: DNT
Value of get is: 50
Value of set is: 70
Conclusion
Keywords are an integral part of any programming language, including C#. They provide structure and meaning to your code, enabling the compiler to interpret your instructions accurately. Understanding the importance of keywords and following best practices in their usage is crucial for writing clean, efficient, and maintainable code.
Resources for further learning and practice
Online tutorials and courses: Explore Additional Learning and Practice Resources with us at https://www.scholarhat.com/training/csharp-certification-training
There are numerous online tutorials and courses available that specifically focus on C# language.
Take our Csharp skill challenge to evaluate yourself!
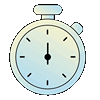
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.