25
AprMethod in C#: Learn How to Use Methods in C#
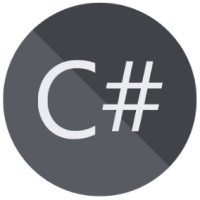
C# Programming For Beginners Free Course
C# Method: An Overview
C# methods are the building blocks of functionality in programming. They encapsulate code, enabling efficient and organized execution of tasks. This article explores the fundamental concepts of C# methods, illustrating how they enhance code modularity, reusability, and readability, essential for mastering software development in the C# language.
Methods in C#
A Method in C# is a block of code within a class or a struct that performs a specific task or operation. Methods are defined with a method signature, which includes the method name, return type, parameters, and access modifiers. They encapsulate logic and can be called to execute their functionality. Methods enhance code modularity, reusability, and readability in C# programs.
Declaring a Method in C#
- Access Modifier: Defines the visibility (public, private, etc.) of the method.
- Return Type: Specifies the data type the method returns or 'void' for no return.
- Method Name: Unique identifier for the method.
- Parameters: Input values the method accepts (optional).
- Body: Contains the method's code logic enclosed in curly braces {}.
Syntax
<Access_Modifier> <return_type> <method_name>([<param_list>])
Example in the C# Compiler
using System;
public class Calculator
{
public int CalculateSum(int a, int b)
{
int sum = a + b;
return sum;
}
public static void Main()
{
Calculator calculator = new Calculator();
int result = calculator.CalculateSum(5, 3);
Console.WriteLine("Sum is: " + result);
}
}
Explanation
- public is the access modifier, indicating the method is accessible from outside the class.
- int is the return type, specifying the method will return an integer.
- CalculateSum is the method name.
- (int a, int b) defines parameters a and b of type integer.
- The method body calculates the sum of a and b and returns the result using the return keyword.
Output
Sum is: 8
Read More - C# Interview Questions And Answers
Calling a Method in C#
Calling a method in C# involves using the dot notation. First, create an instance of the class containing the method. Then, use the instance variable followed by a dot and the method name with parentheses and any required parameters.
Example
// C# program to illustrate// method calling
using System;
namespace ConsoleApplication1 {
class scholar {
// Here Sum() method asks for two parameters from the user and calculates the sum of these and finally returns the result.
static int Sum(int x, int y)
{
// there are two local variables 'a' and 'b' where 'a' is assigned the value of parameter 'x' and
// 'b' is assigned the value of parameter 'y'
int a = x;
int b = y;
// The local variable calculates the sum of 'a' and 'b'
// and returns the result which is of 'int' type.
int result = a + b;
return result;
}
// Main Method
static void Main(string[] args)
{
int a = 12;
int b = 23;
// Method Sum() is invoked and the returned value is stored in the local variable say 'c'
int c = Sum(a, b);
// Display Result
Console.WriteLine("The Value of the sum is " + c);
}
}
}
Explanation
This C# program in the C# Editor defines a class Scholar with a static method Sum, which calculates the sum of two integers. The Main method initializes two variables, calls the Sum method with these variables, stores the result in a variable c, and then prints the result.
Output
The Value of the sum is 35
C# Method Return Type
In C#, the method return type defines the value a method can return after its execution. It specifies the data type of the value that the method produces. Return types can be any valid C# data type, including primitive types (int, float, bool, etc.), custom objects, or even void (indicating the method does not return any value). The return type is declared before the method name in the method signature.
Example
// C# program to illustrate Method With Parameters and With Return Value Type
using System;
namespace ConsoleApplication4 {
class Scholar {
// This method asks a number from the user and using that it calculates the factorial of it and returns the result
static int factorial(int n)
{
int f = 1;
// Method to calculate the factorial of a number
for (int i = 1; i<= n; i++)
{
f = f * i;
}
return f;
}
// Main Method
static void Main(string[] args)
{
int p = 4;
// displaying result by calling the function
Console.WriteLine("Factorial is : " + factorial(p));
}
}
}
Explanation
This C# program calculates the factorial of a number using a method with parameters and a return value. The factorial method takes an integer n as input and calculates its factorial. In the Main method, it calls factorial with the value 4 and prints the result, demonstrating method usage with parameters and return values.
Output
Factorial is : 24
C# Method Parameters
In C#, method parameters are variables that are used to pass values into a method. They are specified in the method's signature and act as placeholders for the values that the method expects to receive when it is called. Parameters allow methods to accept input, making them versatile and capable of performing different operations based on different data.
Example 1: C# Methods with Parameters
using System;
class Calculator
{
// Method to add two numbers passed as parameters
public int Add(int num1, int num2)
{
return num1 + num2;
}
}
class Program
{
static void Main(string[] args)
{
Calculator calculator = new Calculator();
// Calling the Add method with arguments 5 and 10
int sum = calculator.Add(5, 10);
// Output: Sum of 5 and 10 is 15
Console.WriteLine("Sum of 5 and 10 is " + sum);
}
}
Explanation
In this example, the Add method in the Calculator class takes two integers (num1 and num2) as parameters. When the method is called with arguments 5 and 10, it returns the sum (15), which is then displayed as output.
Output
Sum of 5 and 10 is 15
Example 2: C# Methods with Single Parameter
using System;
class Greet
{
// Method to greet a person based on the provided name
public void GreetPerson(string name)
{
Console.WriteLine($"Hello, {name}! Welcome to the C# world.");
}
}
class Program
{
static void Main(string[] args)
{
Greet greeter = new Greet();
// Calling the method with a single argument
greeter.GreetPerson("John");
// Calling the method with a different argument
greeter.GreetPerson("Alice");
}
}
Explanation
This C# code in the C# Online Editor defines a class Greet with a method GreetPerson that takes a string parameter. In the Main method, an instance of Greet is created, and the GreetPerson method is called twice with different names. The method greets each person by name, producing personalized output.
Output
Hello, John! Welcome to the C# world.
Hello, Alice! Welcome to the C# world.
Built-In Methods in C#
Built-in methods in C# are pre-defined functions provided by the .NET Framework that perform common tasks. These methods are part of various classes in the .NET library and cover functionalities like string manipulation, mathematical operations, file handling, and more. Developers can directly use these methods without defining their logic, enhancing productivity and reducing code complexity.
Example 1: Math.Sqrt() Method in C#
using System;
class Program
{
static void Main()
{
double number = 16;
// Calculating the square root of the number using Math.Sqrt() method
double squareRoot = Math.Sqrt(number);
// Output: Square root of 16 is 4
Console.WriteLine($"Square root of {number} is {squareRoot}");
}
}
Explanation
This C# code calculates the square root of the number 16 using the Math.Sqrt() method. The result is stored in the squareRoot variable and then printed to the console.
Output
Square root of 16 is 4
Example 2: ToUpper() Method in C#
using System;
class Program
{
static void Main()
{
string input = "hello, world!";
string upperCaseString = input.ToUpper();
Console.WriteLine("Original String: " + input);
Console.WriteLine("Uppercase String: " + upperCaseString);
}
}
Explanation
This C# code takes a lowercase string "hello, world!" and converts it to uppercase using the ToUpper() method. The original and uppercase strings are then displayed in the console. ToUpper() transforms the characters to uppercase, resulting in "HELLO, WORLD!" being printed as the output.
Output
Original String: hello, world!
Uppercase String: HELLO, WORLD!
Conclusion
In conclusion, methods in C# are fundamental to structuring code, enhancing modularity, and promoting reusability. They encapsulate logic, enabling efficient execution of tasks. By accepting parameters and returning values, methods empower developers to create versatile and dynamic applications, fostering clean, readable, and maintainable code in the C# programming language.
Take our free csharp skill challenge to evaluate your skill
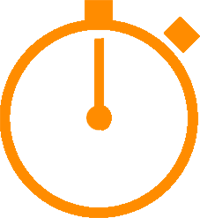
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.