Scope of Variables in C# : Class Level, Method Level and Block Level Scope
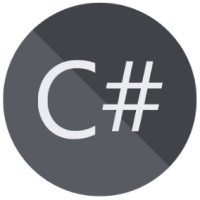
C# Programming For Beginners Free Course
Scope of Variables in C#: An Overview
Understanding the Scope of Variables in C# is essential for mastering the language. This article, delves into the intricacies of variable scope, exploring method, block, and class-level variables. Gain insights into their lifetimes and accessibility, equipping yourself with the knowledge to write efficient and error-free C# code.
What is the Scope of Variables in C#?
The scope of a variable refers to the part of the program where that variable is accessible. You can define a variable in a class, method, loop, etc. The scope of a variable can be determined at compile time and independently of the function call stack in C/C++ since all identifiers are lexically (or statically) scoped. However, the C# programs are organized in the form of classes.
Read More - C# Interview Questions For Freshers
Types of Variable Scope in C#
C# scope rules of variables can be divided into three categories as follows:
- Class Level Scope
- Method Level Scope
- Block Level Scope
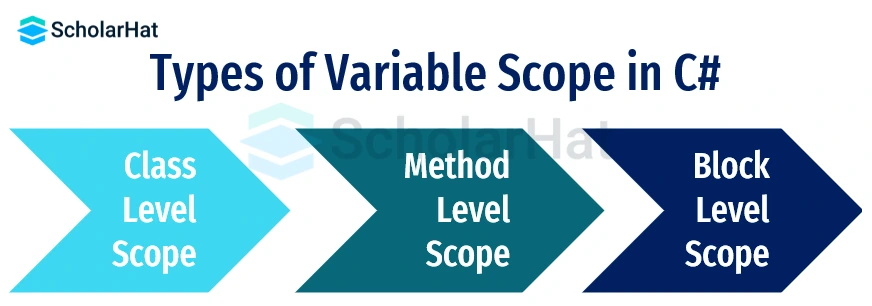
- Class Level Scope in C #
- Definition: Class level scope refers to the visibility and accessibility of variables and methods within a class.
- Accessibility: Variables and methods declared at the class level are accessible to all methods within the class.
- Encapsulation: Class level scope encapsulates data and behavior, ensuring they are limited to the class, promoting data security.
- Inheritance: Class-level members can be inherited by derived classes, providing a foundation for object-oriented programming.
- Modularity: Class-level scope facilitates modular design, allowing related functionality to be encapsulated within a class, enhancing code organization and reusability.
Example
using System;
// Declaring a Class
class DNT
{
// Class level variable with class level scope
int a = 10;
// Declaring a method
public void Display()
{
// Accessing class level variable
Console.WriteLine(a);
}
}
// Main class
class Program
{
static void Main()
{
// Creating an instance of the DNT class
DNT myObject = new DNT();
// Calling the Display method to access the class level variable
myObject.Display();
}
}
Explanation
This C# program in the C# Compiler demonstrates the concept of class-level scope in variables. It defines a class DNT with a variable a having class-level scope (accessible within the class). The display method prints the value of a. Class-level scope means the variable is accessible throughout the class but not outside it.
Output
10
- Method Level Scope in C#
- Method Scope: Variables declared within a method are accessible only within that method.
- Limited Access: Variables have limited visibility and cannot be accessed from outside the method.
- Local Variables: Method-level scope applies to local variables declared inside the method block.
- Encapsulation: Encourages encapsulation by restricting variable access to specific methods.
- Data Protection: Enhances data protection and prevents unintended variable modifications.
- Memory Management: Method-level scope aids efficient memory management by limiting variable lifetime.
- Reuse and Modularity: Promotes code reusability and modularity by encapsulating functionality within methods.
Example
// C# program to illustrate the Method Level Scope of variables
using System;
// declaring a Class
class DNT { // from here class level scope starts
// declaring a method
public void display()
{ // from here method level scope starts
// this variable has method level scope
int m = 47;
// accessing method level variable
Console.WriteLine(m);
} // here method level scope ends
// declaring a method
public void display1()
{ // from here method level scope starts
// it will give compile time error as
// you are trying to access the local variable of method display()
Console.WriteLine(m);
} // here method level scope ends
} // here class level scope ends
Explanation
This C# code demonstrates method-level variable scope. The display method defines a local variable m, which can only be accessed within that method. Attempting to access m in the display1 method results in a compile-time error because m is out of scope, showcasing the concept of variable visibility and scope limitations.
- Block Level Scope in C#
- Definition: Block-level scope in C# refers to the visibility and accessibility of variables, objects, or methods within a specific block of code.
- Limited Scope: Variables declared within a block, such as within loops, if statements, or methods, are accessible only within that block.
- Encapsulation: Helps in encapsulating variables, preventing unintended access and modification from outside the block.
- Local Variables: Variables declared within a method or a block have block-level scope, ensuring they can’t be accessed outside.
- Memory Management: Variables with block-level scope are automatically deallocated from memory once the block execution completes.
- Nested Blocks: Nested blocks create hierarchical scope, allowing inner blocks to access outer block variables, but not vice versa.
- Code Modularity: Encourages modular coding practices by limiting variable visibility to specific sections of code, enhancing code organization.
Example
// C# code to illustrate the Block Level scope of variables
using System;
// declaring a Class
class DNT
{ // from here class level scope starts
// declaring a method
public void display()
{ // from here method level scope starts
// this variable has method level scope
int i = 0;
for (i = 0; i < 4; i++) {
// accessing method level variable
Console.WriteLine(i);
}
// here j is block level variable
// it is only accessible inside this for loop
for (int j = 0; j < 5; j++) {
// accessing block level variable
Console.WriteLine(j);
}
// this will give error as block level variable can't be accessed outside the block
Console.WriteLine(j);
} // here method level scope ends
} // here class level scope ends
Explanation
This C# code demonstrates variable scopes in different levels. It defines a class "DNT" with a method "display". The method contains a method-level variable "i" accessible within the method, and a block-level variable "j" accessible only within its loop. Attempting to access "j" outside its block results in an error due to its limited scope.
Conclusion
In conclusion, understanding variable scope in C# is crucial for writing efficient and error-free code. Variables can have class-level, method-level, or block-level scope, each with its own accessibility rules. Properly managing variable scope ensures code clarity and prevents unintended errors.
Take our free csharp skill challenge to evaluate your skill
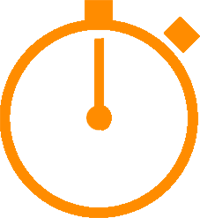
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.