Switch Statement in C#: Difference between if-else and Switch
Switch Statement in C#: An Overview
The C# switch statement is a fundamental construct in the world of C# programming, offering developers a powerful tool for efficient decision-making within their code. In this article, we will delve into the intricacies of the C# switch statement, exploring its syntax, capabilities, and best practices, empowering you to make informed decisions in your code and write more concise and readable programs.
The Switch Statement in C#
A switch statement in C# is a control flow statement that allows you to select one of several code blocks to execute based on the value of an expression. In C#, Switch statement is a multiway branch statement. It provides an efficient way to transfer the execution to different parts of a code based on the value of the expression. The switch expression is of integer type such as int, char, byte, or short, or of an enumeration type, or of string type. The expression is checked for different cases and the one match is executed.
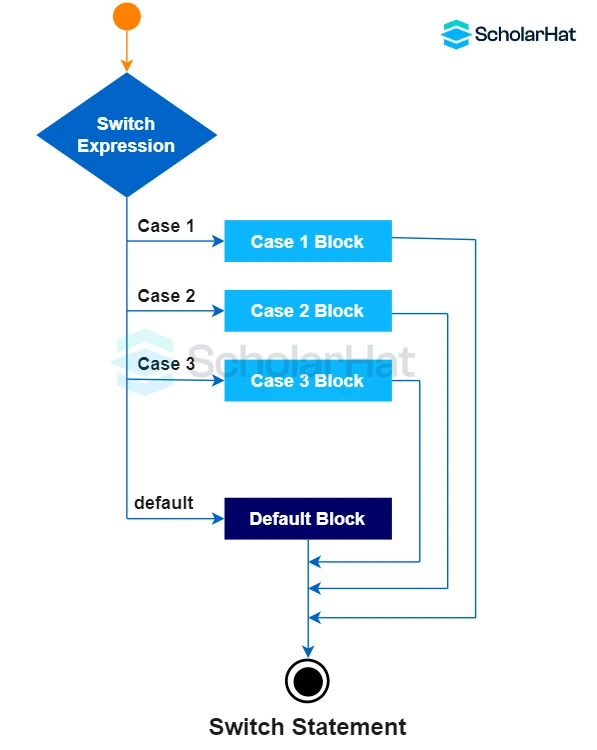
Syntax
switch (expression) {
case value1: // statement sequence
break;
case value2: // statement sequence
break;
.
.
.
case valueN: // statement sequence
break;
default: // default statement sequence
}
Example
using System;
int dayOfWeek = 3;
switch (dayOfWeek)
{
case 1:
Console.WriteLine("Today is Monday.");
break;
case 2:
Console.WriteLine("Today is Tuesday.");
break;
case 3:
Console.WriteLine("Today is Wednesday.");
break;
case 4:
Console.WriteLine("Today is Thursday.");
break;
case 5:
Console.WriteLine("Today is Friday.");
break;
default:
Console.WriteLine("It's the weekend!");
break;
}
Explanation
This C# in the C# Compiler code uses a switch statement to evaluate the value of the dayOfWeek variable and then executes the corresponding case block. It prints the day of the week message based on the value of dayOfWeek. If none of the cases match, it executes the default block and prints "It's the weekend!" In this case, it prints "Today is Wednesday" because dayOfWeek is set to 3.
Output
Today is Wednesday.
Read More - C# Interview Questions For Freshers
Important points to remember about using switch statements in C#
- Switch statements in C# are used for efficient branching in code.
- They evaluate an expression and compare it to case labels.
- Each case label represents a specific value or range of values.
- The default case is executed if no matches are found.
- Cases can fall through without a break statement if desired.
- Switches can handle different data types, including enums and strings.
- Avoid nesting switch statements excessively for readability.
- C# 8.0 introduced pattern matching in switch expressions for more powerful handling.
- Use switch for multiple branching options and if-else for complex conditions.
- Consider using switch expressions when returning values instead of statements for concise code.
Why do we use Switch Statements instead of if-else statements?
- Readability: Switch statements can make code more readable when you have a series of conditions to check against a single value or expression. Each case represents a distinct branch of logic, improving code organization and comprehension.
- Efficiency: Switch statements are often more efficient than long chains of if-else statements because they use a jump table or similar mechanisms, resulting in faster execution for large numbers of cases.
- Intent: Using a switch statement conveys the intention that you are comparing a single value against multiple possible values, making your code more self-explanatory.
- Maintenance: Switch statements are easier to maintain when you need to add or remove cases because you only need to modify the switch block, whereas if-else chains require changes throughout the code.
- Enumerations: Switch statements work well with enumerated types, making code cleaner and less error-prone when dealing with predefined sets of values.
- Code Quality: Code analyzers and compilers can better optimize switch statements, leading to potentially more efficient and error-free code.
However, if-else statements are still valuable for handling more complex conditions or when you need to evaluate expressions or conditions that cannot be easily represented by simple value comparisons. The choice between switch and if-else depends on the specific requirements of your code and readability considerations.
Using goto in the Switch Statement
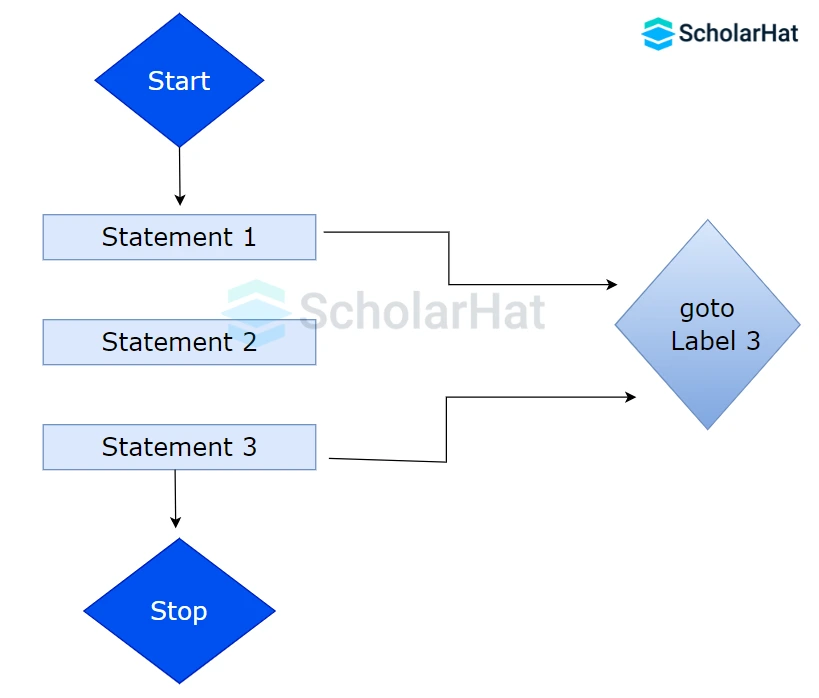
Using goto within a switch statement is possible in C#, but it should be used with caution. The goto statement can make code less readable and harder to maintain, so it's generally discouraged. However, in some rare situations, it might be a valid approach to control the flow of your program.
Example
using System;
class Program
{
static void Main()
{
int option = 2;
string result = "Undefined";
switch (option)
{
case 1:
result = "Option 1 is selected.";
break;
case 2:
goto case 1; // Jump to case 1
case 3:
result = "Option 3 is selected.";
break;
}
Console.WriteLine(result);
}
}
Explanation
In this code, the option is set to 2, and in the switch statement, when case 2 is encountered, there's a goto case 1; statement, which causes the execution to jump to case 1. As a result, the code under case 1 is executed, setting the result to "Option 1 is selected," and that value is printed to the console.
Output
Option 1 is selected.
Differences Between If-Else and Switch Statements
Parameters | If-else statement | Switch statement |
Expression Comparison | The if-else statement allows you to evaluate any Boolean expression as a condition. You can use relational operators, logical operators, and any valid expression to determine the flow of execution. | The switch statement, on the other hand, evaluates a single expression and compares it against various cases. It only supports equality comparisons (==) and cannot handle more complex conditions like ranges or logical operators. |
Multiple Conditions | With if-else, you can have multiple conditions and use logical operators (e.g., &&, ||) to combine them. | In switch, you can only compare a single expression against different cases. Each case represents a specific value and the control flow transfers to the matching case. It doesn't support combining multiple conditions directly. |
Execution Flow | In the if-else statement, the conditions are evaluated one by one from top to bottom until a true condition is found. Once a condition is true, the corresponding block of code executes, and the rest of the conditions are skipped. | In the switch statement, the expression is evaluated once, and the control jumps directly to the matching case (or the default case if no matches are found). After executing the code block for the matching case, the switch statement is exited, unless you explicitly use break statements to control the flow. |
Flexibility | The if-else statement provides more flexibility in terms of the conditions and actions you can perform. You can have nested if-else statements and handle more complex scenarios. It is suitable for conditions that involve ranges, multiple variables, or custom logic. | The switch statement is more concise and readable when you have a single expression to compare against different cases. It's commonly used for scenarios where you have a fixed set of possible values or enumerations. |
Conclusion
In conclusion, the C# switch statement is a versatile and powerful control structure that offers a cleaner and more efficient alternative to nested if-else statements in many scenarios. Its ability to evaluate expressions and handle multiple cases with ease makes it a valuable tool for developers seeking to enhance the readability and maintainability of their code. By understanding the nuances of the switch statement and its various use cases, developers can leverage this feature to write more concise and expressive code, ultimately contributing to better software design and development practices.
Take our Csharp skill challenge to evaluate yourself!
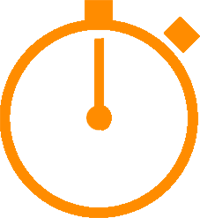
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.