25
AprVariables in C#: Types of Variables with Examples
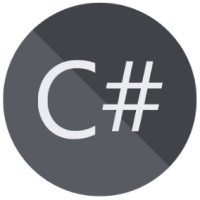
C# Programming For Beginners Free Course
Variables in C#: An Overview
In this tutorial, we will dive deep into what variables are and understand their basic types and operations available in C#. By understanding these concepts from a deeper level, you will have an improved approach while coding with C#. So let's get started!
What are the Variables in C#?
C# variables are used to store and manipulate data within a program. They are named memory locations that hold a specific type of data, such as numbers, characters, or objects. Variables allow you to store values and perform operations on them, making your program dynamic and responsive.
Syntax
<type> <variableName>;
Different Types of Variables in C#
Variable Type | Example |
Decimal types | decimal |
Boolean types | True or false value, as assigned |
Integral types | int, char, byte, short, long |
Floating point types | float and double |
Nullable types | Nullable data types |
Read More - C# Interview Questions And Answers
Rules of Defining Variables in C#
- Variable names must be unique.
- Variable names can contain letters, digits, and the underscore _ only.
- Variable names must start with a letter.
- Variable names are case-sensitive, num and Num are considered different names.
- Variable names cannot contain reserved keywords. Must prefix @ before keyword if want reserve keywords as identifiers.
Examples:
Valid Variables Names
int age;
float _dntemployee;
Invalid Variables Names
int if; // "if" is a keyword
float 42employeename; // Cannot start with digit
Declaring and Initializing Variables in C#
In C#, declaring and initializing variables can be done in several steps. Here's a general outline of the process:
- Declare the variable: Start by specifying the data type of the variable, followed by the variable name. For example:
int age;
- Initialize the variable: Assign a value to the variable using the assignment operator (=). This step is optional, and if you don't initialize the variable, it will be assigned a default value based on its data type. For example:
int age = 25;
- Declare and initialize multiple variables: You can declare and initialize multiple variables of the same data type in a single statement. Separate each variable name with a comma. For example:
int age = 25, height = 180, weight = 75;
- Declare and initialize variables using var: In C#, you can use the var keyword to declare a variable without explicitly specifying its data type. The compiler will infer the type based on the assigned value. For example:
var name = "John";
- Declare and initialize constants: If you want to declare a variable that cannot be changed after initialization, you can use the const keyword. Constants must be assigned a value at the time of declaration and cannot be modified later. For example:
const double PI = 3.14159;
Example
using System;
class Program
{
static void Main()
{
int age = 25;
double height = 1.75;
string name = "John Doe";
bool isStudent = true;
Console.WriteLine("Name: " + name);
Console.WriteLine("Age: " + age);
Console.WriteLine("Height: " + height);
Console.WriteLine("Is Student: " + isStudent);
}
}
Output
Name: John Doe
Age: 25
Height: 1.75
Is Student: True
Explanation
This C# code in the C# Compiler defines a program that declares and initializes variables for a person's name, age, height, and student status. It then uses Console.WriteLine to print these values to the console. The output displays the person's name, age, height, and whether they are a student.
Various Types of Variables in C#
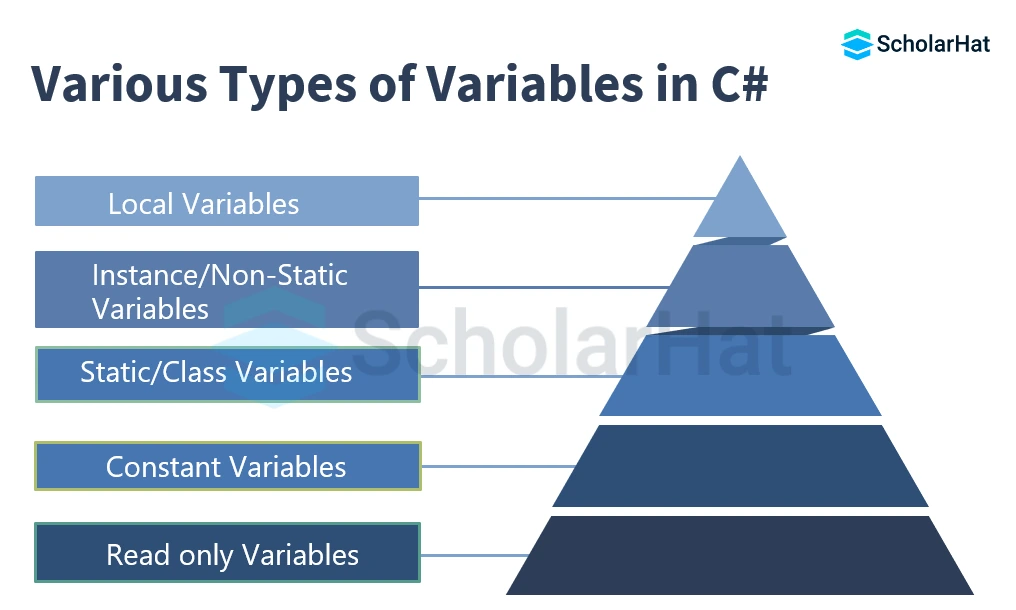
Local Variables in C#
Local variables in C# are variables declared within a method, constructor, or block of code. They have limited scope and are accessible only within the block where they are declared. Local variables must be initialized before use and are often used for temporary storage of data in a program.
Example
using System;
class StudentDetails {
public void StudentAge()
{
int age = 0;
age = age + 10;
Console.WriteLine("Student age is : " + age);
}
public static void Main(String[] args)
{
StudentDetails obj = new StudentDetails();
obj.StudentAge();
}
}
Explanation
This C# code defines a class named "StudentDetails" with a method "StudentAge" that increments a local variable "age" by 10 and prints the result. The Main method creates an instance of the class and calls the "StudentAge" method, displaying the student's age as 10
Output
Student age is: 10
Instance Variables or Non-Static Variables
In C#, instance variables are data members declared within a class but outside of any method. They represent the attributes or properties of objects created from the class. Each object instance has its own set of instance variables, which define the object's state and behaviour.
Example
using System;
class Marks
{
int engMarks;
int mathsMarks;
int phyMarks;
public static void Main(String[] args)
{
Marks obj1 = new Marks();
obj1.engMarks = 89;
obj1.mathsMarks = 76;
obj1.phyMarks = 65;
Marks obj2 = new Marks();
obj2.engMarks = 85;
obj2.mathsMarks = 98;
obj2.phyMarks = 91;
Console.WriteLine("Marks for first object:");
Console.WriteLine(obj1.engMarks);
Console.WriteLine(obj1.mathsMarks);
Console.WriteLine(obj1.phyMarks);
Console.WriteLine("Marks for second object:");
Console.WriteLine(obj2.engMarks);
Console.WriteLine(obj2.mathsMarks);
Console.WriteLine(obj2.phyMarks);
}
}
Explanation
This C# program defines a class "Marks" with three instance variables (engMarks, mathsMarks, and phyMarks) to store subject marks. It creates two objects (obj1 and obj2), assigns different marks to each object, and displays the marks for both objects using Console.WriteLine, demonstrates the use of instance variables to store and access data for different instances of the class.
Output
Marks for first object:
89
76
65
Marks for second object:
85
98
91
Static Variables or Class Variables
In C#, static variables are class-level variables that belong to the type itself rather than to specific instances of the class. They are shared among all instances of the class and retain their values throughout the program's execution, providing a common storage location for all objects of that class.
Example
using System;
class Emp {
static double salary;
static String name = "Rohan";
public static void Main(String[] args)
{
Emp.salary = 125000;
Console.WriteLine(Emp.name + "'s average salary:" + Emp.salary);
}
}
Explanation
This C# program defines a class "Emp" with static variables "salary" and "name." It sets the static salary to 125000 and the name to "Rohan." In the Main method, it prints the name and average salary using the static variables. Static variables are shared among all instances of the class and can be accessed using the class name.
Output
Rohan's average salary:125000
Constant Variables
In C#, a constant variable is a value that cannot be changed once it's defined. It's declared using the 'const' keyword and must be initialized at the time of declaration. Constants are typically used for values that should remain fixed throughout the program, such as mathematical constants or configuration settings, providing improved code readability and preventing accidental modification.
Example
using System;
class Program
{
const float max;
public static void Main()
{
Program obj = new Program();
Console.WriteLine("The value of b is = " + Program.b);
}
}
Explanation
This C# program in the C# Editor attempts to declare a constant variable "max" of type float without providing an initial value. However, this will result in a compilation error because constant variables must have a defined value at the time of declaration. Additionally, it attempts to access an undefined constant "b," which will also result in an error.
Output
The provided C# code has a compilation error because you cannot declare a constant variable without initializing it with a value.
Read-only Variables
In C#, read-only variables are declared using the "readonly" keyword. They can only be assigned a value at the time of declaration or within a constructor. Once assigned, their value cannot be changed throughout the program's execution, ensuring data integrity and immutability. This is useful for constants and values that should not be modified after initialization.
Example
using System;
class Program
{
int a = 80;
static int b = 40;
const float max = 50;
readonly int k;
public static void Main()
{
Program obj = new Program();
Console.WriteLine("The value of a is = " + obj.a);
Console.WriteLine("The value of b is = " + Program.b);
Console.WriteLine("The value of max is = " + Program.max);
Console.WriteLine("The value of k is = " + obj.k);
}
}
Explanation
This C# program demonstrates the use of instance variables (a), static variables (b), constant variables (max), and readonly variables (k). It initializes a, b, and max but doesn't initialize k, which is allowed for readonly variables. The program then prints the values of these variables.
Output
The value of a is = 80
The value of b is = 40
The value of max is = 50
The value of k is = 0
Conclusion
To summarize, variables in c# are essential for any developer looking to create complex projects. The different types of variable declarations allow developers to apply data in ways their projects need. Knowing the purpose and use of each will help improve project design and reduce development efforts. What is most important is that the developer understands the structure and significance of variables in c# when programming a new application or game. With these points in mind, you should be able to apply variables in c# as needed confidently.
Take our free csharp skill challenge to evaluate your skill
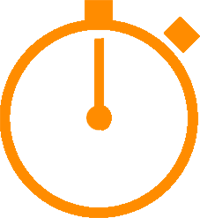
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.