25
AprBrute Force Algorithm in Data Structures: Types, Advantages, Disadvantages
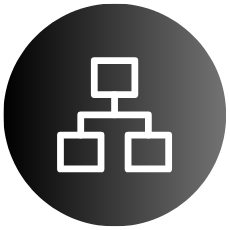
Data Structures & Algorithms Free Course
Brute-force Algorithm in Data Structures: An Overview
While learning the Types of Algorithms in the Data Structures and Algorithms tutorial, we saw that the Brute Force Algorithm is the first and the simplest approach to solving a problem. This DSA tutorial will look at the Brute Force Algorithm- its characteristics, needs, types, etc. For a more detailed theoretical and practical understanding, consider our Best Data Structures And Algorithms Course.
What is the Brute-force Algorithm in Data Structures?
The Brute force Algorithm is the first approach that comes to our mind upon seeing the problem. It's a method of solving a problem by iterating through all possible solutions, which can be an incredibly time-consuming process. However, the Brute force Algorithm is also highly effective in finding solutions that other algorithms might miss.
In essence, the Brute force Algorithm is all about trial and error, letting a computer work systematically through a range of possibilities until it arrives at the correct solution. Although this process may take longer than other algorithms, it can be very rewarding once you see the results.
Here's a general outline of the brute force algorithm:
- Define the problem: Clearly understand the problem you are trying to solve and the constraints involved.
- Enumerate all possible solutions: Generate or iterate through all possible candidates for the solution. The number of candidates typically depends on the size of the problem and the constraints involved.
- Test each candidate: For each candidate solution, apply the problem constraints and evaluate whether it satisfies the required conditions.
- Select the correct solution: If a candidate solution satisfies all the required conditions, it is considered the correct solution. If multiple solutions are possible, you can choose the best one based on specific criteria.
- Analyze the time complexity: Brute force algorithms often have a high time complexity, especially when dealing with large problem spaces. It is important to analyze the efficiency of the algorithm and consider optimization techniques if necessary.
Visual Illustration of Brute Force Algorithm in Data Structures
Let's consider a simple example of finding the maximum value in an array of numbers.
- Start with an array of numbers:
[3, 7, 2, 9, 5]
- Initialize a variable, let's call it max_value, to store the maximum value. Set max_value to the first element of the array:
max_value = 3
- Iterate through the remaining elements of the array and compare each element with the current max_value. If a greater value is found, update max_value to the new maximum:
- Compare 7 with 3: Since 7 is greater, update max_value to 7.
- Compare 2 with 7: 7 is still greater, so no update is made.
- Compare 9 with 7: Since 9 is greater, update max_value to 9.
- Compare 5 with 9: 9 is still greater, so no update is made.
- After iterating through all elements, max_value will contain the maximum value in the array:
max_value = 9
Here's a visualization of the steps described above:
- Initial array: [3, 7, 2, 9, 5]
3 7 2 9 5 \ | | | / \ | | | / \ | | | / \| | | / v v v +---+ | | | | | 3 | | | | | +---+ v | | | | 7 | ---> | | +---+ | v | | | 2 | | ---> | +---+ v | v | | 9 | ---> | | +---+ v | | 5 | ----> | +---+
- Final maximum value: 9
This visualization demonstrates the brute force approach of comparing each element with the current maximum and updating it if a greater value is found. However, keep in mind that brute force algorithms are not always the most efficient solution for complex problems, as they can be computationally expensive.
Read More - Best Data Structure Interview Questions and Answers
Types of Brute Force Algorithm in Data Structures
- Optimizing: In this case, the best solution is found. To find the best solution, it may either find all the possible solutions to find the best solution or if the value of the best solution is known, it stops finding when the best solution is found. For example: Finding the best path for the traveling salesman problem. Here the best path means that traveling all the cities and the cost of travelling should be minimal.
- Satisficing: It stops finding the solution as soon as the satisfactory solution is found. For example, finding the traveling salesman path within 10% of optimal.
Advantages of Brute Force Algorithm
- Simplicity: Brute force algorithms are usually easy to understand and implement. They involve basic looping and iteration constructs, making them accessible even to novice programmers.
- Correctness: By systematically considering all possible solutions, a brute force algorithm guarantees to find the optimal solution, if one exists. It exhaustively checks all possibilities, leaving no room for overlooking potential solutions.
- Reliability: Brute force algorithms are highly reliable due to their exhaustive nature. They are not influenced by specific data distributions or assumptions, as they evaluate every possible solution.
- Versatility: Brute force algorithms can be applied to various problem domains. They do not require any specific problem-specific knowledge or heuristics, making them applicable in various contexts. Additionally, they can be used as a benchmark to evaluate the effectiveness of more sophisticated algorithms, providing a baseline for comparison
Disadvantages of Brute Force Algorithm
- Time Complexity: Brute-force algorithms typically have a high time complexity, especially for large problem sizes. They explore all possible solutions exhaustively, which can result in an exponential number of iterations. As a result, the execution time can quickly become unmanageable or even infeasible for complex problems.
- Inefficiency: Brute-force algorithms often evaluate many irrelevant or redundant solutions before finding the correct one. This inefficiency can be especially pronounced when the search space is large or when there are many constraints involved. Consequently, brute-force algorithms may waste computational resources on unnecessary calculations.
- Memory Usage: Some brute-force algorithms require storing a large amount of data in memory, which can be problematic for memory-constrained systems or when dealing with massive data sets. As the problem size increases, the memory requirements of brute-force algorithms can become prohibitive.
- Lack of Scalability: Brute-force algorithms are generally not scalable, meaning that they struggle to handle larger problem instances. As the input size grows, the exponential growth in computation time makes brute-force approaches impractical or impossible to apply.
- Suboptimal Solutions: Brute-force algorithms explore all possible solutions, including those that are suboptimal. This exhaustive search approach may not be necessary in many cases, as there may be more efficient algorithms or techniques available to solve the problem. Consequently, brute-force algorithms may not provide the most optimal solution within a reasonable timeframe.
- Dependency on Problem Structure: The effectiveness of brute-force algorithms heavily relies on the structure of the problem. If the problem has inherent patterns or symmetries that can be exploited, more efficient algorithms specifically designed for that problem may exist. Brute-force approaches often overlook these structural properties, leading to unnecessarily long computation times.
- Lack of Adaptability: Brute-force algorithms are typically rigid and inflexible. They follow a fixed pattern of exploring all possible solutions without adapting to the characteristics of the problem or leveraging any problem-specific knowledge.
Summary
We thus covered the simplest algorithm with all its aspects. The brute force algorithm is a technique that guarantees solutions for problems of any domain but takes a lot of run time and is inefficient. However, it is effective in finding short-term solutions to difficult problems. So if you're looking for an efficient way to approach impenetrable computing problems, then why not to join our Dsa Training.