11
JulTwo dimensional Array In Data Structure
Two-dimensional Array: An Overview
In the Data Structures and Algorithms (DSA), two-dimensional arrays play a crucial role in representing and manipulating data efficiently. This DSA online Tutorial delves into the fundamentals of two-dimensional arrays, their representation, and their significance in various algorithms and applications. A two-dimensional array provides a structured way to organize and store data.For a more detailed theoretical and practical understanding, consider our DSA Certification course.
What is a two-dimensional array?
A two-dimensional array can be defined as a collection of elements arranged in rows and columns. It can be visualized as a table with rows and columns intersecting to form cells. Each cell contains a specific value, and the array can hold elements of the same or different data types. In programming languages like C, C++, Java, and Python, two-dimensional arrays are typically represented as an array of arrays.
Syntax
data_type array_name[rows][columns];
Example
int twodimen[4][3];
Here, 4 is the number of rows, and 3 is the number of columns.
Declaration and Initialization
If the definition and initialization of the 1D array are done simultaneously, we don't need to mention the array size. This, however, will not work with 2D arrays. We must define at least the array's second dimension. The two-dimensional array can be declared and defined as shown below.
int matrix[3][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
Read More - Data Structure Interview Questions for Freshers
Common Operations on Two-dimensional Arrays
1. Accessing Elements
2. Insertion and Deletion
3. Updating Elements
4. Traversing
5. Row and Column Operations:
6. Transpose:
7. Matrix Multiplication:
8. Rotation:
Example of Two-dimensional Array
# Function to print a 2D array
def print_array(array):
for row in array:
for element in row:
print(element, end="\t")
print()
# Define the dimensions of the matrix
rows = 3
columns = 4
# Declare and initialize a 2D array
matrix = [
[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]
]
# Print the original matrix
print("Original Matrix:")
print_array(matrix)
# Accessing and modifying elements
print("\nElement at matrix[1][2]:", matrix[1][2])
matrix[1][2] = 20
print("Modified Matrix:")
print_array(matrix)
# Sum of elements in each row
for i in range(rows):
row_sum = sum(matrix[i])
print(f"\nSum of elements in Row {i + 1}: {row_sum}")
# Transpose the matrix
print("\nTransposed Matrix:")
for i in range(columns):
for j in range(rows):
print(matrix[j][i], end="\t")
print()
public class TwoDArrayExample {
// Function to print a 2D array
static void printArray(int[][] array) {
for (int i = 0; i < array.length; i++) {
for (int j = 0; j < array[i].length; j++) {
System.out.print(array[i][j] + "\t");
}
System.out.println();
}
}
public static void main(String[] args) {
// Define the dimensions of the matrix
int rows = 3;
int columns = 4;
// Declare and initialize a 2D array
int[][] matrix = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
// Print the original matrix
System.out.println("Original Matrix:");
printArray(matrix);
// Accessing and modifying elements
System.out.println("\nElement at matrix[1][2]: " + matrix[1][2]);
matrix[1][2] = 20;
System.out.println("Modified Matrix:");
printArray(matrix);
// Sum of elements in each row
for (int i = 0; i < rows; i++) {
int rowSum = 0;
for (int j = 0; j < columns; j++) {
rowSum += matrix[i][j];
}
System.out.println("\nSum of elements in Row " + (i + 1) + ": " + rowSum);
}
// Transpose the matrix
System.out.println("\nTransposed Matrix:");
for (int i = 0; i < columns; i++) {
for (int j = 0; j < rows; j++) {
System.out.print(matrix[j][i] + "\t");
}
System.out.println();
}
}
}
#include
// Function to print a 2D array
void printArray(int rows, int columns, int array[rows][columns]) {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
std::cout << array[i][j] << "\t";
}
std::cout << std::endl;
}
}
int main() {
// Define the dimensions of the matrix
int rows = 3;
int columns = 4;
// Declare and initialize a 2D array
int matrix[3][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
// Print the original matrix
std::cout << "Original Matrix:" << std::endl;
printArray(rows, columns, matrix);
// Accessing and modifying elements
std::cout << "\nElement at matrix[1][2]: " << matrix[1][2] << std::endl;
matrix[1][2] = 20;
std::cout << "Modified Matrix:" << std::endl;
printArray(rows, columns, matrix);
// Sum of elements in each row
for (int i = 0; i < rows; i++) {
int rowSum = 0;
for (int j = 0; j < columns; j++) {
rowSum += matrix[i][j];
}
std::cout << "\nSum of elements in Row " << i + 1 << ": " << rowSum << std::endl;
}
// Transpose the matrix
std::cout << "\nTransposed Matrix:" << std::endl;
for (int i = 0; i < columns; i++) {
for (int j = 0; j < rows; j++) {
std::cout << matrix[j][i] << "\t";
}
std::cout << std::endl;
}
return 0;
}
This example demonstrates the creation and manipulation of a 3x4 matrix. It prints the original matrix, modifies an element, calculates the sum of elements in each row, and transposes the matrix.
Output
Original Matrix:
1 2 3 4
5 6 7 8
9 10 11 12
Element at matrix[1][2]: 7
Modified Matrix:
1 2 3 4
5 6 20 8
9 10 11 12
Sum of elements in Row 1: 10
Sum of elements in Row 2: 39
Sum of elements in Row 3: 42
Transposed Matrix:
1 5 9
2 6 10
3 20 11
4 8 12
Applications in Algorithms
1. Matrices and Graphs:
- Two-dimensional arrays are commonly used to represent matrices in mathematical operations, such as matrix multiplication.
- Adjacency matrices in graph theory use two-dimensional arrays to represent connections between vertices in a graph.
2. Dynamic Programming:
- Dynamic programming algorithms often utilize two-dimensional arrays to store intermediate results efficiently, reducing redundant computations.
3. Image Processing:
- In image processing, two-dimensional arrays are employed to represent pixels in an image, facilitating various manipulations and transformations.
4. Board Games:
- Games like chess or tic-tac-toe can be modeled using two-dimensional arrays to represent the game board.
Advantages and Challenges
Advantages
- Efficient organization and representation of structured data.
- Simplifies access and manipulation of grid-based information.
- Facilitates implementation of various algorithms in a more intuitive manner.
Challenges
- Fixed Size: Two-dimensional arrays have a fixed size, which can be limiting when dealing with dynamic data.
- Contiguous Memory: Allocating large two-dimensional arrays may require contiguous memory, which can be challenging in memory-constrained environments.
>>> Read More:- Differences Between Array and Linked List |
Summary
FAQs
Take our Datastructures skill challenge to evaluate yourself!
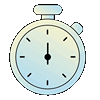
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.