11
JulSoftware Architect Design Patterns
Software Architect Design Patterns are simple and smart ways to solve common problems while building software. They help make the software easier to build, understand, and change. Let's understand this in a real-world scenario.
Have you ever seen a house being built or been part of building your own house? I think most of you have. Before creating a home or any structure, there should be a proper plan. Without a plan, the architect cannot correctly complete the construction. The same thing applies when you are building a software system.
In the Design Pattern tutorial, you will learn software architecture design patterns and more, which help you solve common coding problems and make your software easier to manage.
What is Software Architecture?
Software architecture is the structure of a software system, showing how its parts work together. It ensures the system is efficient, scalable, and easy to maintain.
Software architect design patterns are proven solutions used in software architecture to address common problems. By applying software architect design patterns, developers can create systems that are more reliable and easier to manage over time.
What are Design Patterns in Software Architecture?
Design patterns in software architecture are standard solutions to common problems in building software systems. They help you organize code in a way that makes it easier to understand, maintain, and scale, while also improving flexibility and reducing errors.
- Provide proven solutions to common software problems.
- Help structure code for easier maintenance and scalability.
- Improve communication between developers by using common patterns.
- Make it easier to add new features or change parts of the system without breaking it.
Why Are Design Patterns Important?
Design patterns are important because they help you build better software in less time and with fewer mistakes.
- They offer ready-made solutions to common design problems.
- Help you write cleaner, more organized, and reusable code.
- Make it easier to understand and maintain large codebases.
- Improve teamwork by giving everyone a shared way to solve problems.
- Save time by avoiding the need to "reinvent the wheel" for every project.
Software Architecture Design Patterns Fundamentals
The fundamentals of software architecture design patterns help you understand how to build strong, well-structured software systems. Learning software architect design patterns gives you the tools to solve common problems with smart and reusable solutions.
How do Design Patterns work?
To understand how software architecture design patterns actually work, let’s go through the process step by step:
1. Identify the Problem
First, look at your project and find a common issue you’re facing, like code that’s hard to reuse, too tightly connected, or difficult to scale. This is where software architect design patterns can help by giving you a clear path to solve it.
2. Choose the Right Design Pattern
Now that you know the problem, choose the software architect design pattern that fits best. Each pattern is made to solve a specific type of issue, for example, if you need to separate object creation from usage, the Factory Pattern might be a good choice.
3. Understand the Pattern Structure
Before using it, take time to learn how the chosen software architect design pattern works. Understand its parts, how the classes or components interact, and what role each part plays in solving the problem.
4. Apply the Pattern to Your Code
Use the pattern as a guide and apply it to your actual project. Follow the steps of the software architect design pattern to shape your code — this helps keep your code clean, reusable, and easier to manage.
5. Test and Refine
Finally, test your solution to see if everything works as expected. If needed, make small changes to improve performance or clarity while still keeping the benefits of the software architect design pattern you used.
Key Principles of Software Architecture Patterns
Key principles of software architecture patterns help you build software that works well and is easy to manage. These ideas are the reason why software architect design patterns are so helpful in solving real problems.
1. Keep things separate
Each part of your code should do one job. Software architect design patterns help you split things up so your code stays clean and simple.
2. Easy to grow and change
Your software should be ready for future updates. Software architect design patterns make it easier to add new features without breaking things.
3. Use it again
You don’t need to solve the same problem again and again. With software architect design patterns, you can reuse smart solutions in other parts of your project.
4. Easy to fix and update
When your code is well-organized, it’s easier to fix bugs or make changes. That’s why software architect design patterns help keep your project easy to maintain.
Popular Software Architecture Patterns in Real-World Applications
1. Layered (N-Tier) Architecture
One of the most common software architect design patterns is layered architecture, in which the system is divided into layers like UI, business logic, and data. This pattern helps organize code neatly, so each layer handles its own job. Many developers use this pattern in web and enterprise applications to keep things clean and manageable.
2. Event-Driven Architecture
In this software architecture design pattern, parts of the system talk to each other by sending and receiving events. It’s useful when actions happen at different times, like a user clicking a button or a message being received. Software architect design patterns like this make your system more flexible and easier to scale for real-time features.
3. Microservices Architecture
Microservices Architecture design pattern breaks an app into small, separate services that work independently. Each service does one job, which makes it easier to test, update, and manage. Software architect design patterns like microservices are great for big systems like Netflix or Amazon, where you need flexibility and speed.
4. Model-View-Controller (MVC) Architecture
Software architect design patterns like MVC split an app into three parts:Model (data),View (UI), andController (logic). This separation makes it easy to work on one part without breaking the others.MVCis a popular pattern used in many frameworks, such asDjango, Laravel, and Rails.
5. Client-Server Architecture
This software architect design pattern separates the system into two sides: the client (user interface) and the server (data and processing). It’s one of the oldest and most used software architect design patterns, seen in apps like email services or online games. This pattern helps in building reliable systems that work over a network.
Major Categories of Design Patterns in Software Architecture
Major categories of design patterns in software architecture group common solutions by their purpose. They help you choose the right software architect design patterns for your project easily.
Creational Patterns
Creational design patterns are software architect design patterns that focus on how objects are created in a flexible and reusable way. They help you manage object creation without tightly connecting the code to specific classes.
There are five main types of creational design patterns: Singleton, Factory Method, Abstract Factory, Builder, and Prototype.
1. Singleton Pattern
The Singleton pattern is one of the most used software architect design patterns that ensures only one object of a class is created. This is useful when you need a single shared resource, like a settings manager or a database connection. Using this software architect design pattern helps keep control and consistency across your application.
2. Factory Method Pattern
TheFactory Method design pattern allows a class to create objects without directly knowing which class it needs. The Factory Method pattern makes your code more flexible and easier to extend. It’s a useful software architecture design pattern when your app needs to decide what object to create at runtime.
3. Abstract Factory Pattern
The Abstract Factory pattern creates families of related objects without saying exactly which class will be used. It is a powerful software architect design pattern when your app needs to work with multiple themes, designs, or product sets. This software architect design pattern keeps the code clean and makes switching between object families easy.
4. Builder Pattern
The Builder pattern is a software architect design pattern used to construct complex objects step by step. It is helpful when an object has many parts or options, like a custom form or a complex report. This software architect design pattern gives more control over the creation process.
5. Prototype Pattern
The Prototype pattern is a software architect design pattern that creates a new object by copying an existing one. It is useful when you need to make many similar objects quickly without repeating the whole setup. This software architect design pattern saves time and improves performance.
Structural Design Patterns
Structural design patterns are software architect design patterns that help you organize classes and objects to form larger, flexible structures. These patterns focus on how parts of a system fit together while keeping the code easy to manage.
There are six common structural design patterns: Adapter, Bridge, Composite, Facade, Flyweight, and Proxy.
1. Adapter Pattern
The Adapter pattern is a software architect design pattern that lets two different interfaces work together. It acts like a translator between incompatible classes. This software architect design pattern is useful when you want to reuse existing code without changing it.
2. Bridge Pattern
The Bridge pattern is a software architect design pattern that separates an object’s structure from its implementation. It allows you to change or extend parts without affecting the whole system. This software architect design pattern is great for long-term flexibility.
3. Composite Pattern
The Composite pattern is a software architect design pattern used to treat a group of objects as a single object. It’s useful when you build tree-like structures, such as menus or file systems. This software architect design pattern makes it easy to work with both single items and groups in the same way.
4. Facade Pattern
The Facade pattern is a software architect design pattern that provides a simple interface to a complex system. It hides all the inner details and gives you an easy way to interact with the system. This software architect design pattern helps reduce confusion and makes code easier to use.
5. Flyweight Pattern
The Flyweight pattern is a software architect design pattern that saves memory by reusing objects that are similar. Instead of creating many copies, it shares common data. This software architect design pattern is helpful in apps with lots of repeating elements, like games or text editors.
6. Proxy Pattern
The Proxy pattern is a software architect design pattern that acts as a middleman between the client and the real object. It controls access, adds extra features, or delays the object creation. This software architect design pattern is often used for security, logging, or performance.
Behavioral Design Patterns
Behavioral design patterns are software architect design patterns that focus on how objects communicate and interact with each other. These patterns help manage relationships, responsibilities, and the flow of messages between parts of your system.
There are many behavioral design patterns, but here are a few popular ones:
1. Observer Pattern
The Observer pattern is a software architect design pattern where one object updates other objects when something changes. It’s useful for things like notifications, where many parts need to know when an event happens. This software architect design pattern keeps your system connected but not tightly tied together.
2. Strategy Pattern
The Strategy pattern lets you change the behavior of a class without changing its code. You choose a "strategy" or method at runtime. This software architect design pattern is great when you have different ways to perform an action.
3. Command Pattern
The Command pattern turns a request into a standalone object that can be stored, passed, or executed later. It’s a helpful software architect design pattern when you want to add undo/redo features or delay actions.
4. Chain of Responsibility Pattern
The Chain of Responsibility software design patternpasses a request along a chain of handlers until one can process it.It’s great for building flexible systems, like in logging or approval processes. This pattern makes your code easier to extend and manage.
5. Template Method Pattern
The Template Method pattern defines the basic steps of an algorithm, but lets subclasses fill in the details. It’s a good software architect design pattern when different parts of your app need similar processes with a few changes.
Benefits of using Design Patterns
Using software architect design patterns comes with many useful benefits that can make your development process smoother and smarter.
- Design patterns give you ready-made solutions, so you don’t have to start from scratch every time.
- When you use software architect design patterns, others (and future you!) can understand your code more easily.
- Design patterns help you organize your code better, making it clean and less confusing.
- You don’t have to figure out everything yourself; a software architect's design patterns guide you with proven ideas.
Want to become a better software developer? Join the Software Architecture and Design Certification Training! Learn how to plan and build software step by step in a simple and easy way. Great for students! |
Conclusion
In conclusion, Software architect design patterns are proven solutions that make your software easier to build, understand, and maintain. They help you solve common problems quickly and structure your code in a way that's flexible and scalable. By using these patterns, you can improve the quality of your software and save time in the long run.
If you are preparing for .NET Design Patterns interviews, this .NET Design Patterns Interview Questions and Answers Free Book can really help you. It has simple questions and answers that are easy to understand, download and read it for free today!
Test your skills with the following MCQs
Dear learners, please attempt the following multiple-choice questions (MCQs) on Software Architecture Design Patterns.
Q1: Which pattern separates object creation from its usage?
FAQs
Take our Designpatterns skill challenge to evaluate yourself!
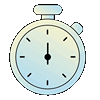
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.