Do-While Loop in Java
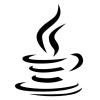
Java Programming For Beginners Free Course
do...while Loop in Java: An Overview
do...while loop in Java is the third type of looping statement in Java. In the Previous Java Tutorial, you have already learnt about the other two loops in Java, that are, for loop in Java and while loop in Java. In this tutorial, you will learn about What is do while loop in Java?, do while loop in Java syntax, do while loop in Java example and much more. To explore more about different concepts of Java Programming, enroll in our Java Certification Training right now!!
Read More: Top 50 Java Interview Questions and Answers
What is do...while Loop in Java?
The do...while loop in Java is also known as Exit Control Loop. It is used by Java Developers when they want that a block of code is executed at least once before checking the condition. After the execution, the specified condition is evaluated to decide whether the loop will be repeated or not.
Flowchart of do...while loop in Java
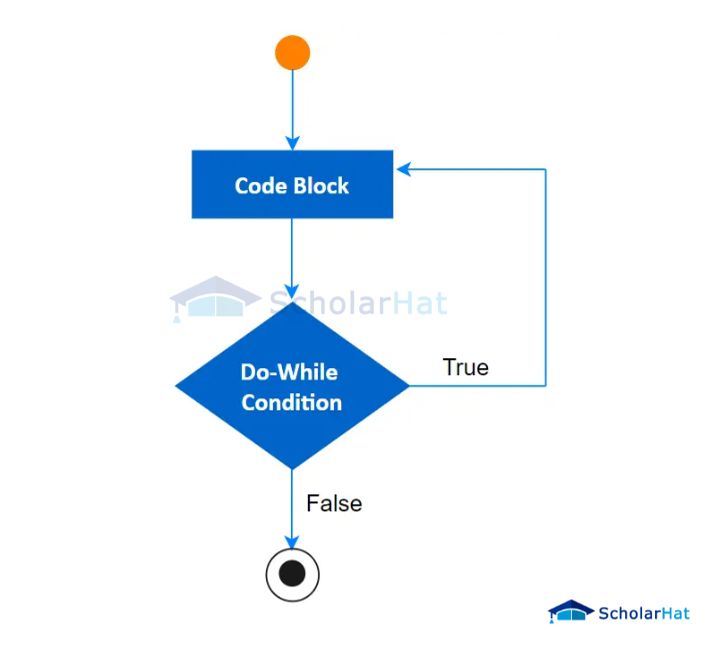
Syntax of do...while loop in Java
do {
// code to be executed
} while (condition);
Explanation:
- The 'do' block has a block of code that is executed first.
- Then, the loop checks the condition that is specified inside the 'while' statement.
- If that condition evaluates to be 'True', the loop body will execute again. It repeats execution until the condition is true.
- Once the condition becomes 'False', the loop will terminate and the program will carry on to the next statement that is just after the do...while loop.
How do...while loop works?
- Initialization The loop starts and the code that is in the 'do' block is executed first.
- Execution The program executes all every statement that is present in the 'do' block in sequence.
- Condition Check When the execution of the loop body is done once, the condition specified in the 'while' statement is checked.
- Conditional Execution If the condition evaluates to be 'True', the execution of the loop body is repeated. The process repeats from step 2. But if the condition evaluates to be 'False', the loop will immediately terminate and the program will carry on with the next statement just after the do...while loop.
- Loop Termination In this way, the loop will continue repeating execution of the loop body until the condition is true. Once it becomes false, the loop will terminate.
Read More: Java Developer Salary Guide in India – For Freshers & Experienced
do...while loop in java with example
1. Sum of Numbers
public class DoWhileExample1 {
public static void main(String[] args) {
int i = 1;
int sum = 0;
do {
sum += i;
i++;
} while (i <= 5);
System.out.println("Sum of numbers from 1 to 5 is: " + sum);
}
}
Output
Sum of numbers from 1 to 5 is: 15
2. Guessing Game
import java.util.Scanner;
public class DoWhileExample2 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int secretNumber = 3;
int guess;
do {
System.out.print("Guess the number between 1 and 5: ");
guess = scanner.nextInt();
if (guess != secretNumber) {
System.out.println("Wrong guess. Try again!");
}
} while (guess != secretNumber);
System.out.println("Congratulations! You guessed the correct number.");
scanner.close();
}
}
Output
Guess the number between 1 and 5: 2
Wrong guess. Try again!
Guess the number between 1 and 5: 4
Wrong guess. Try again!
Guess the number between 1 and 5: 3
Congratulations! You guessed the correct number.
Components of do...while Loop
1. Test Expression
2. Update Expression
Example:
int i = 1;
do {
System.out.println(i);
i++;
} while (i <= 5);
- 'i <= 5' is the Test Expression
- 'i++' is the Update Expression
Use Cases of do...while Loop
1. Menu-Driven Programs
2. Input Validation
3. Game Loops
4. Sequential Processing
5. Timed Loops
Advantages of do...while loop in Java
- a do...while loop makes sure that the loop body is executed at least once before checking the condition.
- Any necessary initialization can be done easily as it the loop condition is checked after executing it once.
- The code is more readable and intuitive.
- It is very useful in cases where input validation is required.
- Repetitive codes can be encapsulated inside the loop body when using a do...while loop making the code reusable and reducing redundancy.
Disadvantages of do...while loop in Java
- Using do...while loops can be quite complex sometimes especially when they are used with multiple conditions or nested loops.
- There are chances of do...while loops resulting in to infinite loops where the loop execution continues indefinitely. This can happen if the loop condition is not properly defined or if it is not updated within the loop body.
- There is less control over the starting of the loop as the loop body is executed once for sure before checking the loop condition.
- It has limited use cases as compared to the other two loops in Java, that are, for loop and while loop.
Summary
FAQs
Q1. What is do-while loop in Java?
Q2. What is the difference between a while and a do-while loop?
Q3. What is called do-while loop?
Q4. What is do-while loop syntax?
do { // Code block to execute } while (condition);
Q5. What are the two types of while loop?
- while loop
- do-while loop