For Loop in Java: Its Types and Examples
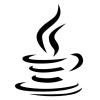
Java Programming For Beginners Free Course
for Loop in Java: An Overview
for loop in Java is one of the basic concepts of Java that you should definitely know. In the last Java Tutorial, you got a glimpse of Loops in Java and its different types, where you got a short overview of Java for loops. In this tutorial, you will get to learn about What is for loop in Java?, Different Types of for loops in Java with examples and much more in detail. To explore more about different concepts of Java, consider enrolling in our Java Certification Training right now!
Read More: Top 50 Java Interview Questions and Answers
What is for loop in Java?
Java for loop is a looping statement in Java. It allows the Java developers to repeat execution of a block of code for a specified number of times.
Flowchart of for loop in Java:
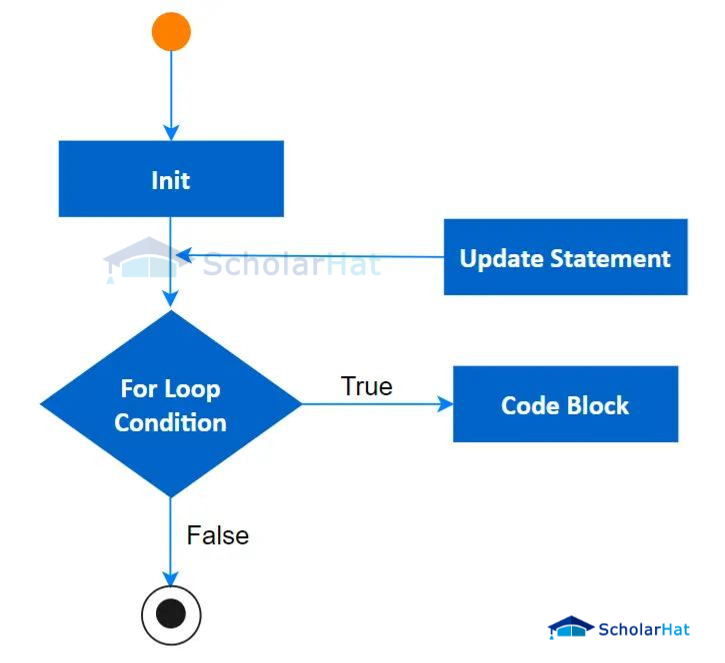
Syntax of for loop in Java
for (initialization; condition; update) {
// code to be executed
}
Explanation:
- Initialization is used for initializing the variable of the loop.
- Condition is used for the evaluation of the variable to check if it is 'True' and continues further. If it is evaluated to be 'False', the loop terminates.
- Update is used for modifying the variable after each iteration is completed.
Example of for loop in Java in Java Compiler:
for (int i = 1; i <= 5; i++) {
System.out.println(i);
}
Explanation:
- We have initialized the loop variable 'i' to'1'.
- Then, we check if i is less than or equal to 5. If the condition is 'True', the loop will continue. If it turns out to be 'False', the loop will terminate.
- With 'i++', there will be an increment of 1 in 'i' after each iteration.
Output
1
2
3
4
5
Read More: Java Developer Salary Guide in India
Types of for Loops in Java
1. Simple for Loop in Java
Example of simple for loop:
for (int i = 1; i <= 5; i++) {
System.out.println("Number: " + i);
}
Output
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
2. for-each Loop in Java
Example of for-each loop:
int[] numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
System.out.println("Number: " + num);
}
Output
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
3. Labeled for Loop in Java
Example of labeled for loop:
outerLoop:
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
if (i == 2 && j == 2) {
break outerLoop;
}
System.out.println("i: " + i + ", j: " + j);
}
}
Output
i: 1, j: 1
i: 1, j: 2
4. Nested for Loop in Java
Example of nested for loop:
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 2; j++) {
System.out.println("i: " + i + ", j: " + j);
}
}
Output
i: 1, j: 1
i: 1, j: 2
i: 2, j: 1
i: 2, j: 2
i: 3, j: 1
i: 3, j: 2
5. Infinite for Loop in Java
Example of infinite for loop:
for (;;) {
System.out.println("This is an infinite loop");
}
Output
This is an infinite loop
This is an infinite loop
This is an infinite loop
This is an infinite loop...
Difference Between for, while Loop and do...while Loop in Java
Aspect | for Loop | while Loop | do...while Loop |
Initialization | Initialization is done inside the loop. | Initialization is done before entering the loop. | Initialization is done before entering the loop. |
Condition Check | Condition checking occurs at the beginning of each iteration. | Condition is checked at the beginning of each iteration. | It checks the condition at the end of each iteration. |
Execution | It will execute the code block only if condition is 'True'. | It will execute the code block only if condition is 'True'. | It will execute the code block at least and then, check the condition. |
Updation | The loop variable is updated after each iteration. | The loop variable is updated within or at the end of the loop. | The loop variable is updated after each iteration. |
Use Case | It is suitable when the number of iteration is known in advance. | It is suitable when the number of iterations is not predetermined. | It is suitable when you want that the code block of the loop is executed at least once. |
Summary
FAQs
Q1. What is for loop syntax in Java?
for (initialization; condition; update) { // code to be executed }
Q2. What is the syntax of a loop?
for (initialization; condition; update) { // code to be executed }
Q3. What is the syntax of while loop in Java?
while (condition) { // code to be executed }
Q4. What are the 3 types of loops in Java?
- for loop
- while loop
- do-while loop