Hierarchical Inheritance in Java
Inheritance in Java: An Overview
What is Hierarchical Inheritance in Java?
- Hierarchical inheritance is one of the crucial types of inheritance in Object-Oriented Programming.
- Where more than one class is derived from the parent class.
- There is a single base class and multiple derived classes.
- It allows users to code reusability and promotes modularity.
- It enables the customization of classes.
- However, changes to the base class can impact all derived classes and make the system less flexible.
Now Let's see Hierarchical Inheritance with an example in Java compiler.
Read More
Example of Hierarchical Inheritance in Java
Step 1: Create the Superclass Called ParentClass
class ParentClass
{
int PAge = 10;
}
Step 2: Create Subclasses called ChildClass1, ChildClass2, ChildClass3.
class ChildClass1 extends ParentClass
{
int CAge1 = 1;
}
class ChildClass2 extends ParentClass
{
int CAge2 = 2;
}
class ChildClass3 extends ParentClass
{
int CAge3 = 3;
}
Step 3: Utilize the Subclasses using objects.
classMainpublicstaticvoidmain(String args[])ChildClass1C1=newChildClass1ChildClass2C2=newChildClass2ChildClass3C3=newChildClass3"PAge * CAge1 = ""PAge * CAge2 = ""PAge * CAge3 = "
class ParentClass
{
int PAge = 10;
}
class ChildClass1 extends ParentClass
{
int CAge1 = 1;
}
class ChildClass2 extends ParentClass
{
int CAge2 = 2;
}
class ChildClass3 extends ParentClass
{
int CAge3 = 3;
}
public class Main
{
public static void main(String args[])
{
ChildClass1 C1 = new ChildClass1();
ChildClass2 C2 = new ChildClass2();
ChildClass3 C3 = new ChildClass3();
System.out.println("PAge * CAge1 = " + C1.PAge * C1.CAge1);
System.out.println("PAge * CAge2 = " + C2.PAge * C2.CAge2);
System.out.println("PAge * CAge3 = " + C3.PAge * C3.CAge3);
}
}
102030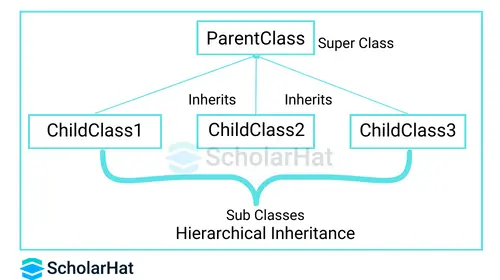
Real-World Example of Hierarchical Inheritance in Java
// Superclass
class Company {
void CompanyName() {
System.out.println("Company name is Dotnettricks.");
}
}
// Subclass 1
class Employee1 extends Company {
void EmployeeName1() {
System.out.println("EmployeeName1 is Sakshi.");
}
}
// Subclass 2
class Employee2 extends Company {
void EmployeeName2() {
System.out.println("EmployeeName2 is Sourav.");
}
}
// Main class
public class Main {
public static void main(String[] args) {
Employee1 E1 = new Employee1();
E1.CompanyName();
E1.EmployeeName1();
Employee2 E2 = new Employee2();
E2.CompanyName();
E2.EmployeeName2();
}
}
Output:
Company name is Dotnettricks.
EmployeeName1 is Sakshi.
Company name is Dotnettricks.
EmployeeName2 is Sourav.
Program Explanation:
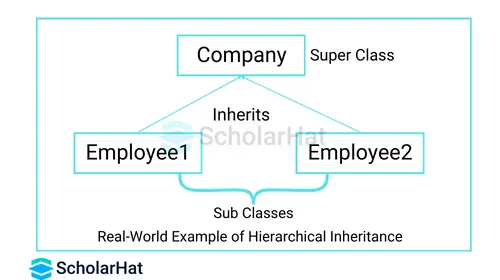
First We created One superclass called Company And Then we Created more two classes called Employee1, and Employee2 which inherit the Parent Class. Employee1 and Employee2 are derived classes. As shown in the program We created objects E1, and E2 of Derived classes and fetched properties of Parent Class "Company" through it As shown in Output.
Use of Hierarchical Inheritance in Java
- The use of Hierarchical Inheritance in Java plays an important role. Method Overriding is one of Use of it.
- Method Overriding occurs when the child class has the same method as declared in the parent class.
- This is mainly used for runtime polymorphism, also known as Dynamic binding.
- It is implemented by virtual functions and pointers.
- Hierarchical Inheritance is also used to Increase code reusability.
Which Inheritance Is Not Supported in Java?
- The Multiple inheritances using classes in Java are not supported.
- Java only allows for single inheritance, where a class can inherit from only one superclass to avoid the complexities that arise from multiple inheritances.
- There are very few situations where multiple inheritances are truly necessary.
- So it is recommended to avoid keeping the codebase simple and manageable.
- One of the challenges with multiple inheritances is the Diamond problem.
Advantages & Disadvantages of Hierarchical Inheritance in Java
Advantages | Disadvantages |
It allows the developer to reuse existing code in many situations. A class can be created once and it can be reused again and again to create many child classes. | In Inheritance base class and child classes are tightly coupled. Hence If you change the code of the parent class, it will affect to all the child classes. |
It saves a lot of time and effort to write the same classes again. | In a class hierarchy, many data members remain unused and the memory allocated to them is not utilized. |
A base class is already compiled and tested properly. This class can be used in a new application without compiling it again. The use of existing classes increases program reliability. | It affects the performance of your program if you have not implemented inheritance correctly. |
Conclusion