Variables in Java: Local, Instance and Static Variables
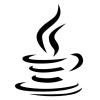
Java Programming For Beginners Free Course
Variables in Java: An Overview
Read More - Top 50 Java Interview Questions For Freshers
What is a Variable in Java?
Variable Declaration
Various types of declaration happen by using Java variables such as
- Strings- which stores text, Such as "Hello world".
- Int- it stores any integer, specifically any whole number without decimal.
- Float- stores "floating point" numbers that include decimal values.
- Char- it stores characters such as 'x', and 'y'. It is denoted by single quotes.
- Boolean- store values if true or false state.
- datatype: It denotes the types of Data that are going to be stored.
- data_name: A particular name of that variable
Rules for Declaring Variables in Java
- You can begin the variable's name with an alphabet, a dollar or underscore symbol, or a currency symbol, but not any other special symbol.
- A variable's name cannot contain more than 64 characters.
- You cannot use blank spaces while declaring a variable.
- Java-reserved keywords cannot be used as variable names.
- The variable name must appear to the left of the assignment operators.
Let's look at the variable declaration in our Java Playground.
Example
class Example
{
public static void main ( String[] args )
{
long payAmount = 184; //the declaration of the variable
System.out.println("The variable contains: " + payAmount );
}
}
Output
Long payAmount = 184;
Variable Initialization
- datatype: it defines what type of data is going to be stored in a variable
- variable_name: Particular name of the variable
- value: initially stored value in the variable
Read More - Java Web Developer Salary
Types of Variables in Java
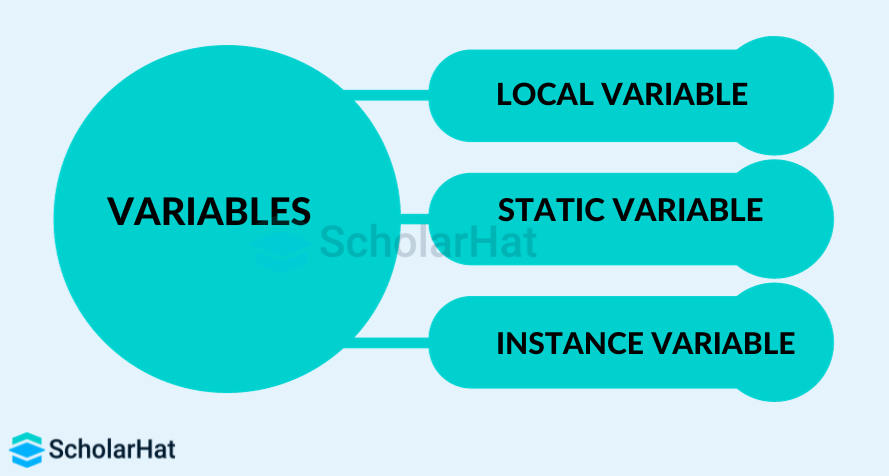
1. Local Variables in Java
- It is created when a block is entered in the storage and then it calls and destroys the block just after exiting from the function.
- It is important to initialize a local variable before using it.
Example
import java.io.*;
class DNT
{
public static void main(String[] args)
{
int var = 89; // Declared a Local Variable
// This variable is local to this main method only
System.out.println("Local Variable: " + var);
}
}
This demonstration in our Java Compiler illustrates the concept of local variables with a scope that is only the main method by declaring and initializing a local variable "var" with the value 89.
Output
Local Variable: 89
2. Instance Variables in Java
- An instance variable is created when an object class is generated and it gets destroyed when the same object class is destroyed
- Programmers can use access specifiers for instance variables
- It is not mandatory to initialize instance variables
Example
import java.io.*;
class DNT
{
public String student; // Declared Instance Variable
public DNT()
{ // Default Constructor
this.student= "Urmi Bose"; // initializing Instance Variable
}
//Main Method
public static void main(String[] args)
{
// Object Creation
DNT name = new DNT();
System.out.println("Student name is: " + name.student);
}
}
In this Java example, the class "DNT" contains a declaration for an instance variable named "student" that is initialized in the default constructor to demonstrate the use of instance variables. An object of the class is then constructed to allow access to and printing of the student's name.
Output
Student name is : Urmi Bose
3. Static Variables in Java
- Static variables are declared as instance variables.
- It is created to start the execution of the program and then destroy it automatically after the execution ends.
- It uses static keywords for the declaration of the static variable within a class
Example
import java.io.*;
class DNT
{
public static String student= "Urmi Bose"; //Declared static variable
public static void main (String[] args) {
//student variable can be accessed without object creation
System.out.println("Student Name is : "+DNT.student);
}
}
This Java example prints the value "Urmi Bose" from a static variable named "student" that is declared inside the class "DNT," enabling access by class name simply without creating an object.
Output
Student Name is: Urmi Bose
Difference between Instance and Static Variable in Java
- In Java, instance variables are stored in separate copies within each object, but static variables are shared across all objects within a class to facilitate memory management.
- While changes to static variables impact all objects since the class shares them, changes to instance variables are limited to the object to which they belong.
- Static variables can only be accessed directly with the class name; instance variables can only be accessed through object references.
- Static variables are produced at the program start and destroyed at the program conclusion, whereas instance variables are created and destroyed along with objects.