Logical operators in Java
Logical Operators in Java: An Overview
We already discussed the Types of Operators in the previous article. In this Java tutorial, we'll explore the syntax, types, and examples of logical operators in Java. Because Java is a flexible and widely used programming language, it has a powerful set of logical operators for manipulating Boolean values.
To further enhance your understanding and application of logical operator's concepts, consider enrolling in the best Java Certification Course, to gain knowledge about effective utilization of logical operators for improved problem-solving and time management.
What are the Logical Operators in Java?
Logical operators in Java are special symbols or keywords that perform logical operations on Boolean values. These operators allow developers to combine or manipulate Boolean expressions, resulting in a single Boolean value. The three main logical operators in Java are:
- The Logical AND operator produces true if both conditions under evaluation are true; otherwise, it returns false.
- The Logical OR operator produces true if either of the supplied conditions is true. The OR operator returns false if and only if both conditions under evaluation are false.
- The Logical NOT operator receives a single value as input and returns its inverse. In contrast to the Logical AND and, Logical OR operators, this is a unary operator.
Syntax:
result = operand1 logical_operator operand2;
Here, operand1 and operand2 are numeric values or variables, and logical_operator is the logical operator that defines the operation to be performed.
Read More - Java 50 Interview Questions
Read More - Advanced Java Multithreading Interview Questions
Types of Logical Operators
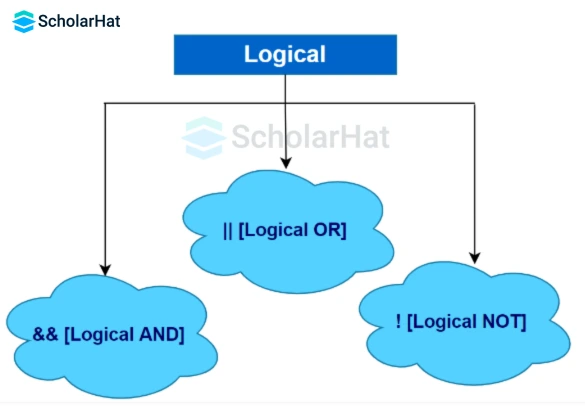
1. && (Logical AND)
Syntax
result = operand1 && operand2;
Example
import java.util.Scanner;
class LogicalOperator {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter value of x: ");
int x = sc.nextInt();
System.out.print("Enter value of y: ");
int y = sc.nextInt();
System.out.print("Enter value of z: ");
int z = sc.nextInt();
if ((x < y) && (x < z)) {
System.out.println("Minimum: " + x);
}
else if ((y < x) && (y < z)) {
System.out.println("Minimum: " + y);
}
else {
System.out.println("Minimum: " + z);
}
sc.close();
}
}
Explanation
Output
Enter value of x: 10
Enter value of y: 50
Enter value of z: 40
Minimum: 10
2. || (Logical OR)
Syntax
boolean result = operand1 || operand2;
Example
import java.util.Scanner;
class LogicalOperator {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter value of x: ");
int x = sc.nextInt();
System.out.print("Enter value of y: ");
int y = sc.nextInt();
System.out.print("Enter value of z: ");
int z = sc.nextInt();
// || operator
System.out.println((x < y) || (z > x));
System.out.println((x > y) || (z < x));
System.out.println((x < y) || (z < x));
sc.close();
}
}
Explanation
Output
Enter value of x: 10
Enter value of y: 20
Enter value of z: 30
true
false
true
3. ! (Logical NOT)
Syntax
boolean result = !operand;
Example
import java.util.Scanner;
class LogicalOperator {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter value of x: ");
int x = sc.nextInt();
System.out.print("Enter value of y: ");
int y = sc.nextInt();
System.out.println(!(x == y));
System.out.println(!(x > y));
sc.close();
}
}
Explanation
Output
Enter value of x: 26
Enter value of y: 12
true
false
Read More - Java Certification Salary
Examples of Logical Operators in Java
class LogicalOperator {
public static void main(String[] args) {
// && operator
System.out.println((4 > 3) && (7 > 6)); // true
System.out.println((4 > 3) && (7 < 6)); // false
// || operator
System.out.println((4 < 3) || (7 > 6)); // true
System.out.println((4 > 3) || (7 < 6)); // true
System.out.println((4 < 3) || (7 < 6)); // false
// ! operator
System.out.println(!(4 == 3)); // true
System.out.println(!(4 > 3)); // false
}
}
Explanation
The above code in the Java Playground illustrates how logical operators combine and manipulate Boolean values to produce different results based on the specified conditions. It is a practical example that showcases the behavior of logical operators in Java.
Output
true
false
true
true
false
true
false
Logical Operators Table
Operand 1 | Operand 2 | && (Logical AND) | || (Logical OR) | ! (Logical NOT) |
true | true | true | true | false |
true | false | false | true | false |
false | true | false | true | false |
false | false | false | false | true |
Advantages of Logical Operators in Java
- Logical operators increase code readability by simplifying difficult situations, resulting in greater comprehension among developers.
- They increase flexibility by mixing operators in different ways, allowing for dynamic responses to program changes.
- They promote code reuse, reducing redundancy and streamlining the development process.
- Furthermore, logical operators make debugging easier, aiding in the detection of unusual behavior and speeding up issue resolution.
Disadvantages of Logical Operators in Java
- Logical operators increase code readability by simplifying difficult situations, resulting in greater comprehension among developers.
- They increase flexibility by mixing operators in different ways, allowing for dynamic responses to program changes.
- They promote code reuse, reducing redundancy and streamlining the development process.
- Furthermore, logical operators make debugging easier, aiding in the detection of unusual behavior and speeding up issue resolution.
Explore More Operators in Java
- Relational operators in Java
- Arithmetic operators in Java
- Assignment operator in Java
- Unary operator in Java
- Bitwise operator in Java
- Ternary operator in Java
Summary
This was all about the types of logical operators in Java. Logical operators in Java are essential tools for constructing complex conditions and decision-making structures in programs. Understanding the syntax and behavior of logical operators is crucial for writing efficient and error-free code. If you're interested in more tips and guidance, you may also consider our Java Online Course, which can validate your skills and enhance your credibility in the field.