28
JunWhat is Package in Java - Types, Uses & Work
A package in Java is a namespace that organizes a set of related classes and interfaces. It helps avoid class name conflicts, makes code easier to maintain, and improves access control. Java packages can be built-in (like java.util, java.io) or user-defined. Itis a way to group related classes, interfaces, and sub-packages into a single organized unit.
The package in Java helps manage code better, prevents name conflicts, and makes large programs easier to maintain. Java includes many built-in packages like java.util, and you can also create your own custom packages. Using a package in Java improves code structure, reusability, and readability.
In this Java tutorial, we'll have a detailed discussion regarding all the aspects of packages in Java.
What is a Package in Java?
A package in Java is like a folder that groups related classes and interfaces to keep your code well-organized. It also helps prevent problems when two classes have the same name by separating them into different packages.
- Packages help you organize your code better, especially in large projects.
- They prevent name conflicts if two classes from different packages have the same name.
- Java provides built-in packages like java.util, java.io, and java.sql to make development easier.
- You can also create your own packages to group your custom classes based on functionality.
- Using packages makes your code cleaner, reusable, and easier to maintain.
Why is a Package Important in Java?
There are some common uses of packages in Java:
- Encapsulation: Packages hide implementation details and display only the necessary functionalities.
- Code Reusability: You can create reusable components and use them anywhere in the application by importing them.
- Modularity and Organization: Maintenance and search are easy as packages consist of all related classes and interfaces.
- Namespace Management: Packages create unique namespaces for different classes and interfaces, thus avoiding naming conflicts when classes with the same name appear in two or more packages. It helps reduce ambiguity in code.
- Access Control: Java provides different access levels with different access modifiers (private, protected, public, and package-private) to restrict access to classes and their members. Protected and default members have package-level access control.
How to Create a Package in Java
A package in Java is like a folder where you keep related classes together. It helps you keep your project neat and organized.
Step-by-Step Guide:
Step 1: Create a Class with Package Name
At the top of your Java file, write the package name using the package keyword.
// File: MyClass.java
package mypackage;
public class MyClass {
public void showMessage() {
System.out.println("Hello from MyClass!");
}
}
Save this file as MyClass.java.Keep it inside a folder named mypackage.
Step 2: Compile the File
Open your terminal (or command prompt) and run this command:
javac -d . MyClass.java
This creates the mypackage folder and puts the class file inside it.
Step 3: Create Another File to Use It
Now, make a new Java file outside the folder to use the class.
// File: TestPackage.java
import mypackage.MyClass;
public class TestPackage {
public static void main(String[] args) {
MyClass obj = new MyClass();
obj.showMessage();
}
}
Step 4: Compile and Run
- Compile:javac TestPackage.java
- Run:java TestPackage
- Output: Hello from MyClass!
How Do Packages in Java Work?
Packages in Java work by grouping related classes and interfaces into a single folder-like structure. When you create a class and place it in a package, Java uses the package name to locate and manage that class. You can import packages using the import keyword, which allows you to use the classes inside them.
- You define a package using the package keyword at the top of your Java file.
- Classes in the same package can easily use each other without importing.
- To use a class from another package, you import it using import packagename.ClassName.
- Java uses the folder structure of your project to match the package name.
- Packages make your code organized, reusable, and easier to navigate.
Accessing Classes inside a Package
// Importing the Random class from the java.util package
import java.util.Random;
// Importing all classes from the java.util package
import java.util.*;
// Importing all classes and interfaces from a specific package
import mypackage.*;
// Importing only a specific class from a package
import mypackage.MyClass;
// Using fully qualified names to avoid naming conflicts
import java.util.HashMap;
import mypackage.HashMap;
Example
import java.util.Stack;
public class StackExample {
public static void main(String[] args) {
Stack stack = new Stack<>();
// Push elements onto the stack
stack.push(100);
stack.push(200);
stack.push(300);
// Print the top element of the stack
System.out.println("Top element:"+stack.peek());
// Pop elements from the stack
int poppedElement=stack.pop();
System.out.println("Popped element:"+poppedElement);
// Check if the stack is empty
System.out.println("Is the stack empty? "+stack.isEmpty());
// Get the size of the stack
System.out.println("Stack size:"+stack.size());
System.out.println("Stack elements:");
for (Integer element:stack)
{
System.out.println(element);
}
}
}
Output
Top element:300
Popped element:300
Is the stack empty? false
Stack size:2
Stack elements:
100
200
Read More: Top 50 Java Full Stack Developer Interview Questions and Answers |
Types of Packages in Java
1. Built-in packages
The classes in these packages are part of the Java API. These packages get installed when you install Java on your computer. The JAVA API is the library of pre-defined classes available in the Java Development Environment. Following are some of the built-in packages in Java
- Java.lang: package of fundamental classes
- Java.io: package of input and output function classes
- Java.awt: package of abstract window toolkit classes
- Java.swing: package of windows application GUI toolkit classes
- Java.net: package of network infrastructure classes
- Java.util: package of collection framework classes
- Java.applet: package of creating applet classes
- Java.sql: package of related data processing classes
The built-in packages are further classified into extension packages. These extension packages start with javax. For example:
- Javax.swing
- Javax.servlet
- Javax.sql
The only default package without an explicit import statement is the java.lang package.
public class Main {
public static void main(String[] args) {
// Using String (java.lang.String)
String message = "Hello, World!";
System.out.println(message);
// Using Math (java.lang.Math)
int max = Math.max(10, 20);
System.out.println("Max: " + max);
// Using System (java.lang.System)
long currentTime = System.currentTimeMillis();
System.out.println("Current Time: " + currentTime);
}
}
Output
Hello, World!
Max: 20
Current Time: 1716034810123
In the above code, we implicitly import java.lang.* to have access to some of the fundamental classes provided by Java, such as Math, String, and System.
2. User-defined packages
As the name suggests, these packages are created by the developers to define the classes and interfaces as per the application requirements.
Syntax to create user-defined packages
package packageName;
The package keyword is used to define the package name. Declare the package before any import statements in the Java class. In your created package, ensure that all the classes should be public so that one can access them outside the package.
Read More: |
package package_first;
public class FirstClass {
public void printFunctionFirst()
{
System.out.println("Hello this is our first package");
}
}
package package_second;
public class SecondClass {
public void printFunctionSecond()
{
System.out.println("Hello this is our second package");
}
}
import package_first.FirstClass;
import package_second.SecondClass;
public class Testing {
public static void main(String[] args)
{
FirstClass a = new FirstClass();
SecondClass b = new SecondClass();
a.printFunctionFirst();
b.printFunctionSecond();
}
}
Output
Hello this is our first package
Hello this is our second package
Using Static Import
This feature is included in the Java Version 5 and above. Using static import, one can use the static fields and methods defined in a class as public static without specifying the class in which the field is defined.
import static java.lang.System.*;
class StaticImportExample {
public static void main(String args[])
{
// out without System prefix
out.println("Welcome to ScholarHat");
}
}
Output
Welcome to ScholarHat
Packages in Java: Handling Name Conflicts
Understanding the Issue
Consider two classes with the same name, but in different packages:
com.example.utils.Date
java.util.Date
If both classes are imported into the same Java file, the compiler cannot distinguish between them when the class name is used directly.
Strategies to Handle Name Conflicts
1. Use Fully Qualified Names
Instead of importing both classes, you can use the fully qualified name of the class when referring to it. This approach removes ambiguity.
// Using java.util.Date
java.util.Date utilDate = new java.util.Date();
// Using com.example.utils.Date
com.example.utils.Date customDate = new com.example.utils.Date();
2. Import One Class and Use Fully Qualified Name for the Other
You can import one class to use it directly and use the fully qualified name for the other class.
import java.util.Date;
public class Example {
public static void main(String[] args) {
Date utilDate = new Date(); // java.util.Date
com.example.utils.Date customDate = new com.example.utils.Date(); // Fully qualified name
}
}
3. Avoid Wildcard Imports
Using wildcard imports (e.g., import java.util.*;
) can lead to name conflicts as it imports all classes from the package. Instead, explicitly import only the required classes.
import java.util.Date;
import com.example.utils.Date; // Specific imports to avoid conflict
4. Refactor Class Names
If you control the code, consider renaming one of the conflicting classes or placing it in a more descriptive package to minimize the chance of conflicts.
Best Practices
- Organize Packages Logically: Use descriptive package names that reflect the purpose or organization, such as
com.company.project.module
. - Explicit Imports: Avoid wildcard imports to make dependencies clear and prevent accidental name clashes.
- Consistent Naming Conventions: Follow naming conventions for packages and classes to reduce potential overlaps.
Subpackage in Java
A subpackage is a package inside another package. It improves the package structure. You can use it to create related classes, group them, and make them a part of the bigger package. Let's take an example and understand.
Suppose there's a package called Vehicles. Now you want to create some classes related to a specific type of vehicle, let's say bikes. In this case, you can create a subpackage named Bikes inside the major package, Vehicles. This organizes your package structure like files in a directory.
In the above image, one can look at how the package structure is organized. The more the complexity of the application grows, the more organized the package structure.
Example of Subpackage
- Create a utility class in the com.example.util subpackage.
package com.example.util; public class Utility { public static void printMessage(String message) { System.out.println(message); } }
- Create the main class in the com.example.main package and use the Utility class from the com.example.util subpackage.
package com.example.main; import com.example.util.Utility; public class Main { public static void main(String[] args) { String message = "Hello from subpackage example!"; Utility.printMessage(message); } }
To compile and run this code,
- Navigate to the src directory
cd src
- Compile the Java files
javac com/example/util/Utility.java com/example/main/Main.java
- Run the Main class
java com.example.main.Main
What are User-Defined Packages in Java?
User-defined packages are the packages that you, as a programmer, create to group your own classes and interfaces. They help you organize your code, avoid naming conflicts, and make your program modular and easier to manage.
How to Create a User-Defined Package in Java
Let's see step by step how to create a User-Defined Package in Java:
Step 1: Declare a Package at the Top of the File
Use the package keyword followed by the package name.
package mypackage;
public class MyClass {
public void showMessage() {
System.out.println("Hello from MyClass!");
}
}
Step 2: Compile the File with -d Option
javac -d . MyClass.java
This creates a folder mypackage and places the compiled .class file inside it.
Step 3: Use the Package in Another Program
import mypackage.MyClass;
public class Test {
public static void main(String[] args) {
MyClass obj = new MyClass();
obj.showMessage();
}
}
Compile and run:
- javac Test.java
- java Test
Benefits of User-Defined Packages
- Keeps your code organized and modular
- Makes it easier to reuse your classes
- Helps avoid naming conflicts
- Enables access control using modifiers like public, private, etc.
Advantages of Packages in Java
1. Better Code Organization
Packages help group related classes and interfaces together, just like putting similar files into folders. This makes your project clean and easy to manage.
2. Code Reusability
You can create classes once in a package and reuse them anywhere in your program by importing them. This saves time and avoids writing the same code again.
- import mypackage.MyClass;
3. Avoid Naming Conflicts
Packages help you avoid confusion when two classes have the same name. Java can tell them apart by using the package name.
Example:
- java.util.Date
- java.sql.Date
4. Access Control
Java packages support access control. You can decide which classes or methods are visible to other parts of your program using public, private, or protected.
5. Encapsulation
Packages help hide internal code and show only the necessary parts. This protects your code and keeps it simple for others to use.
6. Supports Modularity
Packages promote modular programming by dividing the program into smaller, independent parts. This makes it easy to test, update, or fix issues.
Conclusion
In conclusion, the package in Java is a simple but powerful feature that helps organize code in a clean and manageable way. It groups related classes together, avoids name conflicts, and improves reusability. Whether you use Java’s built-in packages or create your own, using packages makes your code more structured, readable, and easier to maintain in larger projects. If you're a beginner to Java programming, consider enrolling in our Java Full Stack Developer Certification Course to get a comprehensive, step-by-step guide to mastering Java from basics to advanced concepts.
FAQs
- Built-in packages
- User-defined packages
- Use lowercase letters only.
- Separate words with periods (.).
- Traditionally, they follow a reverse domain name structure (e.g., com.example.mypackage).
Take our Java skill challenge to evaluate yourself!
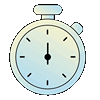
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.