02
MayRelational Operators in Java - Types of Relational Operators
Relational Operators in Java: An Overview
Are you eager to dive into the world of Java programming and master the art of using relational operators? Previously, in our Java Certification Course, we learned about different types of operators briefly. In this Java tutorial, we'll take you through a comprehensive journey covering various types of Relational operators in Java. Get ready for hands-on examples. Relational operators in Java play a crucial role in comparing values and making decisions based on these comparisons.
Ready to become a full stack developer? Enroll in our Java Full Stack Developer Course Training now!
What are the Relational Operators in Java?
We frequently need to compare the relationship between two operands in Java programming, such as equality, inequality, and so on. In Java, relational operators are used to check the relationships between two operands. These operators allow developers to create logical conditions that form the backbone of control flow in Java programs.
The relational operators return a Boolean value after comparison. Relational operators are mostly used for conditional checks such as if, else, for, while, and so on. Java includes relational operators such as greater than (>), less than (), greater than or equal to (>=), less than or equal to (=), equal to (==), and not equal to (!=).
Syntax
Here is the Java syntax for relational operators:
operand1 relational_operator operand2
Read More - Advanced Java Interview Questions
Read More - Java Multithreading Interview Questions
Types of Relational Operators in Java
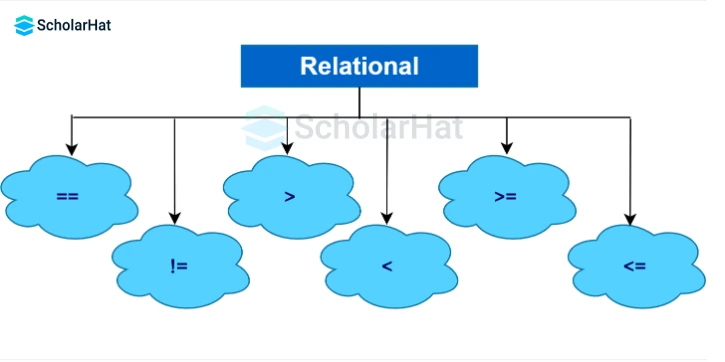
The commonly used relational operators in Java are:
- Equal to (==): Checks if two values are equal.
- Not equal to (!=): Verifies if two values are not equal.
- Greater than (>): Tests if the left operand is greater than the right operand.
- Less than (<): Determines if the left operand is less than the right operand.
- Greater than or equal to (>=): Check if the left operand is greater than or equal to the right operand.
- Less than or equal to (<=): Verifies if the left operand is less than or equal to the right operand.
1. Equal to (==)
The Equal To (==) operator determines whether the two operands are equal. If the operand on the left is equal to the operand on the right, the operator returns true; otherwise, it returns false.
operand1 == operand2
Example
import java.io.*;
public class RelationalOperatorExample {
public static void main(String[] args) {
int a = 5;
int b = 7;
boolean result = (a == b);
System.out.println("Is a equal to b? " + result);
}
}
Output
Is a equal to b? false
2. Not equal to (!=)
The Not Equal To (!=) operator determines whether the two operands are not equal. It has the opposite effect of the equal-to-operator. It returns true if the operand on the left is not equal to the operand on the right, it is false.
operand1 != operand2
Example
import java.io.*;
public class RelationalOperatorExample {
public static void main(String[] args) {
int x = 10;
int y = 10;
boolean result = (x != y);
System.out.println("Is x equal to y? " + result);
}
}
In this case, the Java Compiler will print false since x and y are equal.
Output
Is x equal to y? false
3. Greater than (>)
This determines whether or not the first operand is greater than the second operand. When the operand on the left-hand side is greater than the operand on the right-hand side, the operator returns true.
operand1 >= operand2
Example
import java.io.*;
public class RelationalOperatorExample {
public static void main(String[] args) {
double num1 = 8.5;
double num2 = 6.3;
boolean result = (num1 > num2);
System.out.println("Is num1 is grater than num2 " + result);
}
}
The result will be true because num1 is greater than num2.
Output
Is num1 is grater than num2 true
4. Less than (<)
This determines whether or not the first operand is less than the second operand. When the operand on the left-hand side is less than the operand on the right-hand side, the operator returns true. It has the opposite effect of the greater-than operator.
operand1 < operand2
Example
import java.io.*;
public class RelationalOperatorExample {
public static void main(String[] args) {
int m = 15;
int n = 20;
boolean result = (m < n);
System.out.println("Is m is less than n " + result);
}
}
Here, the result will be true as m is less than n.
Output
Is m is less than n true
5. Greater than or equal to (>=)
This determines whether or not the first operand is bigger than or equal to the second operand. When the operand on the left-hand side is larger than or equal to the operand on the right-hand side, the operator returns true.
operand1 >= operand2
Example
import java.io.*;
public class RelationalOperatorExample {
public static void main(String[] args) {
int p = 30;
int q = 30;
boolean result = (p >= q);
System.out.println("Is p greater than or equal to q? " + result);
}
}
Since p is equal to q, the result will be true.
Output
Is p greater than or equal to q? true
6. Less than or equal to (<=)
This determines whether the first operand is less than or equal to the second operand. When the operand on the left-hand side is less than or equal to the operand on the right-hand side, the operator returns true.
operand1 >= operand2
Example
import java.io.*;
public class RelationalOperatorExample {
public static void main(String[] args) {
int value1 = 25;
int value2 = 18;
boolean result = (value1 <= value2);
System.out.println("Is value1 less than or equal to value2? " + result);
}
}
The result on exeution on Java Playground will be false because the value1 is not less than or equal to the value2.
Output
Is value1 less than or equal to value2? false
Read More - Java Developer Salary
Example of Relational Operators in Java
import java.io.*;
import java.util.Scanner;
public class RelationalOperators {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
int num1 =10;
int num2 = 20;
System.out.println("num1 > num2 is " + (num1 > num2));
System.out.println("num1 < num2 is " + (num1 < num2));
System.out.println("num1 >= num2 is " + (num1 >= num2));
System.out.println("num1 <= num2 is " + (num1 <= num2));
System.out.println("num1 == num2 is " + (num1 == num2));
System.out.println("num1 != num2 is " + (num1 != num2));
}
}
Output
num1 > num2 is false
num1 < num2 is true
num1 >= num2 is false
num1 <= num2 is true
num1 == num2 is false
num1 != num2 is true
Data Types Supported by Relational Operators
- Both objects and any primitive data type can be used with the == and != operators.
- Primitive data types that can be expressed as numerical values can be used with the <, >, <=, and >= operators.
- It won't work with a boolean, but it will with char, byte, short, int, etc. For objects, these operators are not available.
Advantages of Relational Operators in Java
- Relational operators are a convenient way to compare values in code, such as text or numbers.
- They handle various forms of data and are essential to program decision-making.
- Programs operate more smoothly thanks to relational operators' speed and accuracy.
- When you use them, your code becomes readable, reusable, and easier to update and maintain.
- By using variable comparisons to identify issues in the code, they can help with debugging.
Importance in Decision-Making
Relational operators are often used in decision-making structures, such as if statements and loops, to control the flow of a program based on certain conditions. For example,
import java.io.*;
import java.util.Scanner;
public class EligibilityChecker {
public static void main(String[] args) {
int age = 20;
if (age >= 18) {
System.out.println("You are eligible to vote!");
} else {
System.out.println("Sorry, you are not eligible to vote.");
}
}
}
Output
You are eligible to vote!
Explore More Operators in Java
- Arithmetic operators in Java
- Logical operators in Java
- Assignment operator in Java
- Unary operator in Java
- Bitwise operator in Java
- Ternary operator in Java
Summary
In this Java tutorial, relational operators are essential tools for comparing values and making decisions in your programs. Understanding how to successfully employ these operators allows you to write logical and efficient code. Mastering relational operators is essential for any Java developer, whether they are working on basic conditional statements or complicated algorithms. If you want to learn further about Operators in Java, then take the Java Full Stack Developer Course Online. This course covered all the concepts related to Java. After this course, you will be a master of Java programming.
FAQs
- = is the assignment operator used to assign a value to a variable.
- == is the equality operator used to compare two values for equality.
- == (Equal to)
- != (Not equal to)
- > (Greater than)
- < (Less than)
- >= (Greater than or equal to)
- <= (Less than or equal to)