Single Inheritance in Java
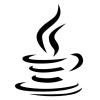
Java Programming For Beginners Free Course
What Is Inheritance in Java?
Inheritance is one of the powerful Object-Oriented Programming concepts in Java. In which a class can inherit attributes and behaviors from superclasses. This allows systematic designing and structuring of classes, enabling access to properties of different methods or classes. In this Java Tutorial, we are going to discuss specifically Single Inheritance in Java, which will include What is Single Inheritance in Java? and Why Use Single Inheritance? We will also explore Single inheritance in Java with example. So, Let's first discuss a little bit about "What is Single Inheritance ?".
What is Single Inheritance?
- Single inheritance allows a child class to inherit properties and behavior from a single-parent class.
- It enables code reusability as well as you can add new features to the existing code.
- This makes the code less repetitive.
- Single inheritance is more secure than multiple inheritance if it is approached in the right way.
- It also enables a child class to call the parent class implementation for a specific method if this method is overridden in the child class or the parent class constructor.
Syntax of Single Inheritance in Java
class Childclass-name extends Parentclass-name
{
//methods
}
Example of Single Inheritance in Java
class Student{
void Fee() {
System.out.println("Student Fee= 20000");
}
}
class Student_Name extends Student{
void Name() {
System.out.println("Student Name=Jayanti");
}
}
class College {
public static void main(String args[]) {
Student_Name p = new Student_Name();
p.Fee();
p.Name();
}
}
Output:
Student Fee= 20000
Student Name=Jayanti
In the above example, we have taken two classes- The Student class and the Student_Name class.
The Student_Name class extends the Student class, which is an example of a single inheritance. The relationship “is a” is established here, i.e., a Student_Name is a Student. In the main method, we create an object ‘p’ of the class Student_Name. p.Fee() calls the method of the Student class, which is inherited by the Student_Name class, and p.Name() calls the method of the Student_Name class.
Why Do We Need Java Inheritance?
- Encapsulation: In encapsulation when a code involves some common variables, The programmer encapsulates these in a parent class and simply provides particular attributes to child classes.
- Polymorphism: In this, The same class acts differently to ensure according to the form of the Child’s class.
- Code Reusability: Using Inheritance we can reuse the code whenever we want.
Types of inheritance in java
- Multiple inheritances
- Multi-level inheritance.
- Hierarchical Inheritance.
- Hybrid Inheritance.
1. Multiple Inheritance
- Java doesn’t support multiple inheritances in classes because it can create a diamond problem.
- Consider Article Multiple Inheritance in Java for a better understanding of the diamond problem
2. Multi-level inheritance.
- In Multi-Level Inheritance, a class extends to another class that is already extended from another class.
- For example, if A daughter extends the feature of the mother and the mother extends from the grandmother, then this scenario is known to follow Multi-level Inheritance.
- As the name shows, numerous base classes are involved in multi-level inheritance.
- The newly derived class from the parent class becomes the base class for another newly derived class.
- The inherited features in multilevel inheritance in Java likewise come from several base classes.
3. Hierarchical Inheritance.
- Hierarchical inheritance in Java is the sort of inheritance when many subclasses derive from a single class.
- In short, It is a mixture of various inheritance types called hierarchical inheritance.
- Hybrid Inheritance in Java is a combination of all inheritances.
- Single and hierarchical inheritances or multiple inheritance.
Benefits of Single Inheritance in Java
- In single inheritance, a code can be used again and again
- It enhances the properties of the class, which means that the property of the parent class will automatically be inherited by the child class
- Inheritance allows access to properties and methods of a Parent class by a Child class. The "extend" keyword is used to inherit all the properties of the Parent classes by Child classes. (Subclass is a class derived from another class)
Limitations of Single Inheritance in Java
- A Child class can only inherit from a single parent Class which results in less flexibility for class designs.
- It can limit the ability to reuse code as some code might not fit in a hierarchy caused by the single inheritance.
- It can cause ambiguity in code or throw errors, as some inherited methods can have the same names but have different operations.
Advantages and Disadvantages of Inheritance
Advantages | Disadvantages |
It allows for code reuse, saving you ample time and effort during software development. | It creates a rigid and complex design that is hard to adapt or change, especially when the program requirements evolve with time. |
It enables the creation of a class hierarchy, which is useful for modeling real-world objects and establishing their relationships. | It can be hard to understand, particularly when an inheritance hierarchy or multiple inheritances are used. |
It divides code into parent and child classes, which helps to write more organized and modular code. | It restricts code flexibility due to tight coupling. |
It enables the creation of abstract classes defining a common interface for a group of related classes, which makes it easier to maintain and extend code. | Decreased Code Performance due to sometimes of complexity. |
Conclusion
FAQs
Q1. What is the single inheritance in Java?
Q2. What are the 4 types of inheritance in Java?
- Single-level inheritance.
- Multi-level Inheritance.
- Hierarchical Inheritance.
- Multiple Inheritance.
- Hybrid Inheritance.