Unary operator in Java
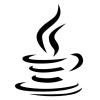
Java Programming For Beginners Free Course
Unary Operators in Java: An Overview
We already discussed the Types of Operators in the previous tutorial. In this Java unary operator article, we'll explore the syntax, and types, providing a comprehensive overview with example of unary operator in Java. In Java, unary operators are essential components of the programming language that perform operations on a single operand.
To further enhance your understanding and application of unary operator's concepts, consider enrolling in the best Java Certification Course, or Java Tutorial to gain knowledge about the effective utilization of unary operators for improved problem-solving and time management.
What are the Unary Operators in Java?
Unary operators are essential in Java programming because they allow developers to perform operations on a single operand with ease and efficiency. Unary operators are operators that perform operations on a single operand. In Java, there are several unary operators, each serving a distinct purpose. These operators can be applied to various data types, including numeric types, Boolean, and objects.
These operators are versatile and can be applied to various data types, including numeric types, Boolean, and objects.
Syntax of Unary Operator in Java:
The basic syntax for unary operators in Java is as follows:
result = unary_operator operand;
Here, the "unary_operator" is the unary operator being applied, and the "operand" is the value on which the operation is performed.
Read More - Top 50 Java Interview Questions For Freshers
Types of Unary Operators
Java supports several unary operators, each serving a specific purpose. The primary unary operators are:
- Unary Plus Operator
- Unary Minus Operator
- Increment Operator
- Decrement Operator
- Logical Complement Operator
1. Unary Plus (+)
The unary plus operator is used to indicate a positive value. It does not change the sign of the operand.
Syntax
+operand;
Example
import java.io.*;
class UnaryOperator{
public static void main(String[] args)
{
int num = 10;
System.out.println("Before operation = " + num);
num = +num;
System.out.println("After operation = " + num);
}
}
Explanation
Output
Before operation = 10
After operation = 10
2. Unary Minus (-)
The unary minus operator negates a numeric value, changing its sign.
Syntax
-operand;
Example
import java.io.*;
class UnaryOperator{
public static void main(String[] args)
{
int num = 10;
System.out.println("Before operation = " + num);
num = -num;
System.out.println("After operation = " + num);
}
}
Explanation
Output
Before operation = 10
After operation = -10
3. Increment Operator (++)
The increment operator is used to increase the value of a variable by 1. There are two forms of the increment operator:
- Pre-increment Operator:
When the Pre-increment Operator is used before the variable name, the operand's value is quickly incremented.
Syntax
++variable;
Example
import java.io.*;
class UnaryOperator{
public static void main(String[] args)
{
int num1 = 3;
int num2 = ++num1;
System.out.println("After Pre-Increment = " + num2);
}
}
Explanation
Output
After Pre-Increment = 4
- Post-increment Operator:
When the Post-increment Operator is placed after the variable name, the operand's value is increased, but the previous value is maintained temporarily until the end of this statement and updated before the end of the next statement.
Syntax
variable++;
Example
import java.io.*;
class UnaryOperator{
public static void main(String[] args)
{
int num1 = 3;
int num2 = num1++;
System.out.println("After Post-Increment = " + num2);
}
}
Explanation
Output
After Post-Increment = 3
4. Decrement Operator (--)
Similar to the increment operator, the decrement operator decreases the value of a variable by 1. It has two forms:
- Pre-decrement Operator:
Syntax
--variable;
Example
import java.io.*;
class UnaryOperator{
public static void main(String[] args)
{
int num1 = 3;
int num2 = --num1;
System.out.println("After Pre-Decrement = " + num2);
}
}
Explanation
Output
After Pre-Decrement = 2
- Post-decrement Operator:
Syntax
variable--;
Example
import java.io.*;
class UnaryOperator{
public static void main(String[] args)
{
int num1 = 3;
int num2 = num1--;
System.out.println("After Post-Decrement = " + num2);
}
}
Explanation
Output
After Post-Decrement = 3
5. Logical Complement Operator (!)
The logical complement operator is used to invert the value of a Boolean expression. If the operand is true, the result is false, and vice versa.
Syntax
!(variable);
Example
import java.io.*;
class UnaryOperator{
public static void main(String[] args)
{
boolean num1 = true;
boolean num2 = !num1;
System.out.println("After logical operator = " + num2);
}
}
Explanation
Output
After logical operator = false
Read More - Java Web Developer Salary
Example of Unary Operators in Java
public class UnaryOperatorsExample {
public static void main(String[] args) {
// Unary Plus and Minus
int originalNumber = 8;
int positiveValue = +originalNumber;
int negativeValue = -originalNumber;
System.out.println("Original Number: " + originalNumber);
System.out.println("Unary Plus: " + positiveValue);
System.out.println("Unary Minus: " + negativeValue);
// Increment and Decrement
int counter = 5;
counter++; // Increment by 1
System.out.println("After Increment: " + counter);
counter--; // Decrement by 1
System.out.println("After Decrement: " + counter);
// Logical Complement
boolean isJavaFun = true;
boolean isJavaNotFun = !isJavaFun;
System.out.println("Is Java fun? " + isJavaFun);
System.out.println("Is Java not fun? " + isJavaNotFun);
}
}
Explanation
Output
Original Number: 8
Unary Plus: 8
Unary Minus: -8
After Increment: 6
After Decrement: 5
Is Java fun? true
Is Java not fun? false
Advantages of Unary Operator in Java
- Concise and Simple to Use: Unary operators just require one operand to function. They are simple to understand, making code more readable and concise.
- Quicker than Other Operators: Compared to other operators, ternary operators are faster since they just need a single operand. This makes them excellent for actions that must be completed fast, such as increasing a counter.
- Pre- and Post-Increment/Decrement: As unary operators support both pre- and post-increment and decrement, they are helpful in a wide range of applications. The pre-increment operator increases the value of a variable before it is used in an expression, whereas the post-increment operator increases the value of a variable after it has been used in an expression.
- Updating Primitive Types: Unary operators can change the value of primitive types such as int, long, float, double, etc.
- Unary Operator in Java
- Arithmetic Operators in Java
- Relational Operators in Java
- Logical Operators in Java
- Ternary Operator in Java