04
JulGetting Started with ExpressJS
In this post we are going to start with ExpressJS by getting the explanation of how to start with environment setup, package management, also learning about its versioning, advantages, and disadvantages.
Later we are going to learn how to use a tool like Express-Generator to create the quick application using the command line, after that we are going to explore application architecture of the created application — Node.js Free Certification is also available for further learning.
Ok, now it’s time, let’s get started with express learning, the most popular web framework ExpressJS.
Read More - Node JS Interview Questions and Answers
What is Express.js?
In the startup stage it’s common to raise with those common startup questions like "What is ExpressJS? Why should I use that? Is it easy to developing, maintaining, and implementing?" Well, to me it is simple and easy to developing, maintaining, later section of this post I am going to show how simple and easy to work with ExpressJS.
Let’s get the definition from expressjs website what they define of ExpressJs as "Fast, unopinionated, minimalist web framework for Node.js".
According to their definition one thing is clear that it is fast in performance, next they define express as unopinionated. What does that mean?
That means with ExpressJS developers are flexible to solve complexity in several ways, there’s no specific way to follow.
Lastly express define as a minimalist web framework, which points the simplicity and lightweight of the framework, where Node.js is open source JavaScript runtime environment support on various platforms like Windows, Linux, UNIX, and Mac OS X.
ExpressJS Version History
According to Wikipedia ExpressJS Initial release was November 16, 2010, the latest stable version is 4.16.3 which is released on March 12, 2018 about 5months ago by fixing different issues like incorrect end tag and 404 output for bad path.
Here’s the list of version evaluation, let’s have a quick overview for better knowledge on ExpressJS road-map.
V-4.x API Features
Robust routing
Focus on high performance
Super-high test coverage
HTTP helpers (redirection, caching, etc)
View system supporting 14+ template engines
Content negotiation
Executable for generating applications quickly
V-3.x API Features
Built on Connect
Robust routing
HTTP helpers (redirection, caching, etc)
View system supporting 14+ template engines
Content negotiation
Focus on high performance
Environment based configuration
Executable for generating applications quickly
High test coverage
V-2.x API Features
Robust routing
Redirection helpers
Dynamic view helpers
Application level view options
Content negotiation
Application mounting
Focus on high performance
View rendering and partials support
Environment based configuration
Session based flash notifications
Built on Connect
Executable for generating applications quickly
High test coverage
Get more details by following the link: https://github.com/expressjs/express/blob/master/History.md
.
ExpressJS Advantages
ExpressJS with all those definition like its simplicity, flexibility there’s another thing which is its biggest community. Here’s the list of express advantages:
Fast and easy to start with startup application.
Built in route.
Supports different view engines.
Easy to create RESTful service.
Easy static file serving.
After having all those readings, let’s have some practical knowledge with a sample application.
Creating Express App using express-generator
In this section we are going to have a practice session about how to start with ExpressJS startup application using Express-generator.
To start with that we need to prepare the NodeJS development environment by installing the latest version of NodeJS.
Download latest NodeJS runtime by following the link:https://nodejs.org/en
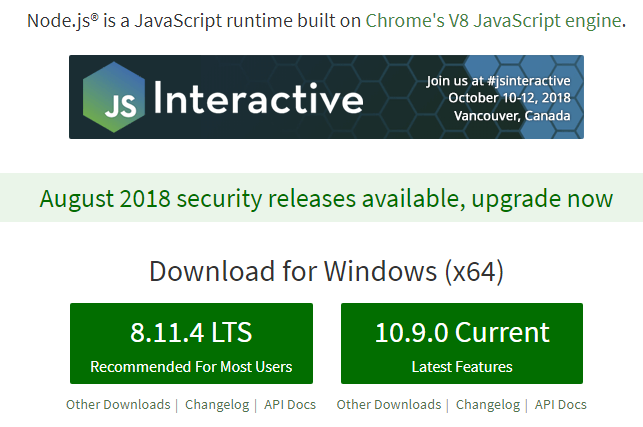
After installation check the version in command prompt by typing "node -v" then press enter, it will show the installed version of NodeJS. Type "node -h" to get more command line options.
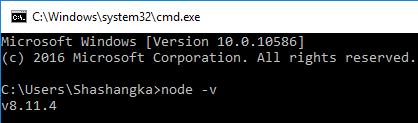
As we can see Node v8.11.4 is installed on my machine. Let’s check ExpressJS version by typing "express --version" also with the npm version by typing "npm -v".
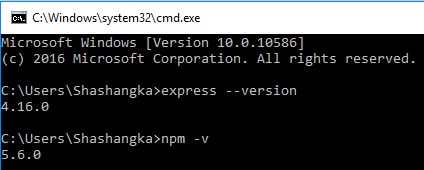
So far we are good to go for next step to use the ExpressJS application generator tool for creating sample startup application with a single command.
Let’s install the global package of express generator using the command line "npm install express-generator -g" here -g mean globally.

After successful installation let’s create a sample application named "FirstExpressapp" with the command in command prompt.
Go to application folder where the sample application will create then press n hold Shift+Right mouse click to show option "Open command window here" like below image. Click on it, this will open the command window with relative folder path.
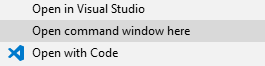
Now type the command line "express FirstExpressapp" to create a new sample startup application.
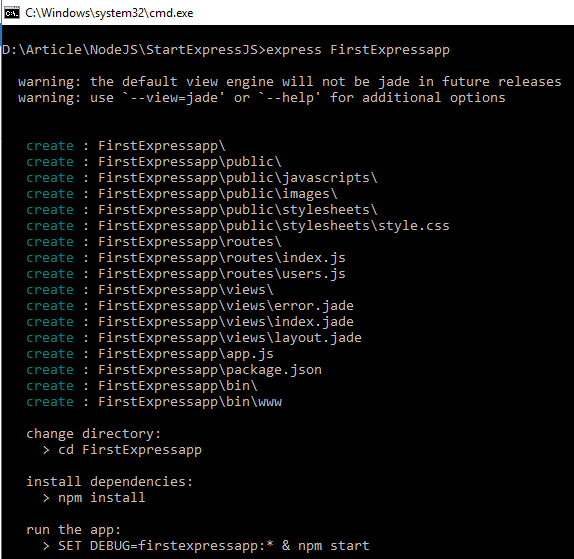
As we can see startup application is created in the desired folder. Next step to install NPM packages to our application for package dependencies.
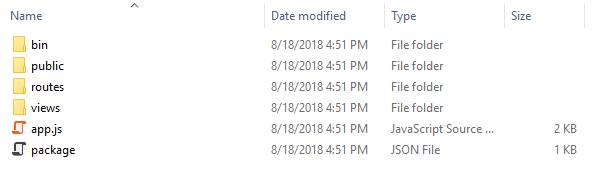
Change the directory like below image to point the application source where NPM packages are going to installed.

It’s time to start the application after all those installation steps. Type "SET DEBUG=firstexpressapp:* & npm start" or simply type "npm start" to start the application.

Open the browser type "localhost:3000" in URL bar then hit enter you will see the welcome message in browser.
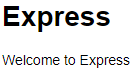
Next section we are going to explore the application folder and architecture, let’s open the application using "Visual Code" source code editor.
Download Visual Code from following link: https://code.visualstudio.com/download
.
Exploring Express App Architecture
In application folder we can see below list of folders.
bin
nodejs_modules
public
routes
views
let’s have explanation of all those auto generated folder by express-generator.
bin
This is the bootstrap point of ExpressJS application, configured in package.json, application will automatically bootstrap ./bin/www script file when we start the application using "npm start" command. Below is the code snippet of package.json where startup script is configured with bin folder.
{ "name": "firstexpressapp", "version": "0.0.0", "private": true, "scripts": { "start": "node ./bin/www" } }
node_modules
This is the package folder for our application, when we run "npm install" command it’ll download all package dependencies to application "node_modules" local folder. Below is the code snippet of package.json where listed package dependencies are used in application.
{ "name": "firstexpressapp", "version": "0.0.0", "private": true, "scripts": { "start": "node ./bin/www" }, "dependencies": { "cookie-parser": "~1.4.3", "debug": "~2.6.9", "express": "~4.16.0", "http-errors": "~1.6.2", "jade": "~1.11.0", "morgan": "~1.9.0" } }
public
This is the folder where we are containing all static resources like images, scripts, css which are permitted to access publicly.
Below code snippet from app.js file demonstrate how using express.static() method static file is served from public folder.
var express = require('express'); var path = require('path'); var app = express(); app.use(express.static(path.join(__dirname, 'public'))); module.exports = app;
Here express.static is middleware initiated by app.use() method.
routes
This is where all the route files are listed. From the below code snippet in app.js route modules are imported by require() function then it is initiated by app.use() method where first argument is the path and the second is the imported module instance which is resolving the invoked path.
var express = require('express'); var path = require('path'); var indexRouter = require('./routes/index'); var usersRouter = require('./routes/users'); var app = express(); app.use(express.static(path.join(__dirname, 'public'))); app.use('/', indexRouter); app.use('/users', usersRouter); module.exports = app;
views
This folder is contained with template engine. According to route the res.render() function compiling the view engine to html. Here, first argument is the view name and second one is the view options.
var express = require('express'); var router = express.Router(); /* GET home page. */ router.get('/', function(req, res, next) { res.render('index', { title: 'Express' }); }); module.exports = router;
In our sample application the view engine use as "jade", which configured in app.js with views directory name.
var express = require('express'); var path = require('path'); var indexRouter = require('./routes/index'); var usersRouter = require('./routes/users'); var app = express(); // view engine setup app.set('views', path.join(__dirname, 'views')); app.set('view engine', 'jade'); app.use(express.static(path.join(__dirname, 'public'))); app.use('/', indexRouter); app.use('/users', usersRouter); module.exports = app;
Summary
In final words, time to get back those points which we have learned. Here’s the listed recapping points:
Now we know how to setup the nodeJS development environment from scratch.
Learnt about ExpressJS with latest release also the advantages of using ExpressJS.
Using command line we have learned how to quick start ExpressJS application with ExpressJS-Generator tools.
Knowledge of installing NPM, express routes, view engine.
Finally we have explanation about all those application folder that is created by generator.
Hope this will help them to clarify the development environment, application architecture, package managing who are getting started with ExpressJS — and you can even enroll in a Free Node.js Course With Certification to further boost your skills.
Take our Nodejs skill challenge to evaluate yourself!
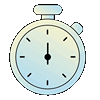
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.