Python Dictionaries (With Examples) : A Comprehensive Tutorial
Dictionary in Python: An Overview
What is a dictionary in Python?
A dictionary in Python is a group of key-value pairs, sometimes referred to as a hash table. Every key is distinct and corresponds to a single value. Dictionary entries can have their values modified after they are created, which is known as mutability. Data is organized and stored using dictionaries. They can be used to store object-specific information such as attributes and methods.
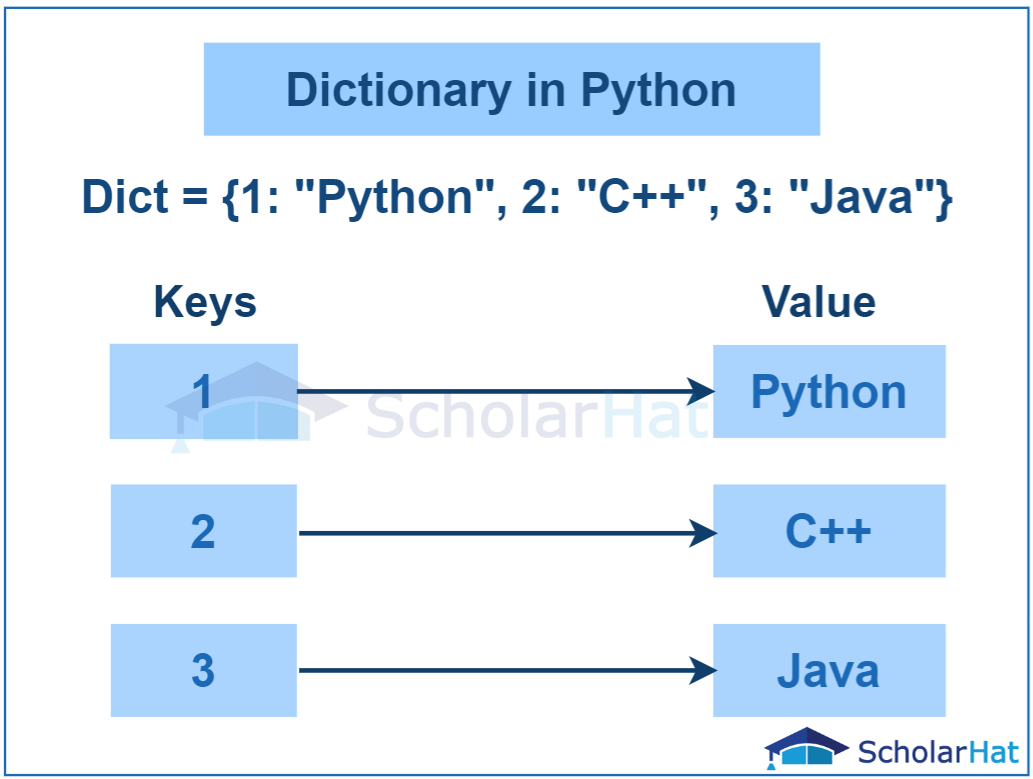
Read More - 50 Python Interview Questions
Creating a Dictionary in Python
In Python, creating a dictionary is a simple procedure. Two techniques to create a dictionary are as follows:
- Using curly braces
- Using the dict() function
1. Using curly braces
Curly braces {} are used in this method to define the dictionary. You specify key-value pairs, separated by commas, inside the curly braces. The data you wish to save is the value, and the key serves as the value's identification.
Example of Creating Dictionary using Curly Braces in Python Editor
country_capitals = {"France": "Paris", "Germany": "Berlin", "Italy": "Rome"}
print(country_capitals)
Output
{'France': 'Paris', 'Germany': 'Berlin', 'Italy': 'Rome'}
2. Using the dict() functionUsing the built-in dict() function, you create the dictionary in this method. Key-value pairs are the only argument accepted by the function.
Example of Creating Dictionary using dict() Function in Python
country_capitals = dict([("France", "Paris"), ("Germany", "Berlin"), ("Italy", "Rome")])
print(country_capitals)
Output
{'France': 'Paris', 'Germany': 'Berlin', 'Italy': 'Rome'}
Access Value in Dictionary in Python
- To access the objects in a dictionary, use its key name.
- You can use the key inside square brackets.
- The element can also be accessed from a dictionary with the help of a Python function named get().
- This function returns the value after accepting the key as an input.
Example of Accessing Values in Dictionary in Python
dictionary = {"name": "Mohan Kumar", "age": 35, "city": "New Delhi"}
name = dictionary["name"]
print(name)
Output
Mohan Kumar
Read More - Python Developer Salary
Adding elements to a Dictionary
- In Python, the assignment operator (=) is the most popular and straightforward way to add elements to a dictionary.
- This method includes directly assigning the required value to the specified key within the dictionary.
Example of Adding Elements to a Dictionary in Python
student_info = {}
student_info["name"] = "Mohan Kumar"
student_info["age"] = 35
print(student_info)
The code first generates an empty dictionary called "student_info," adds key-value pairs for "name" and "age," and then prints the student's information from the dictionary.
Output
{'name': 'Mohan Kumar', 'age': 35}
Update Dictionary in Python
- The most popular and effective approach to updating a dictionary is using the update() method.
- It updates the dictionary with key-value pairs that are passed in as an iterable object.
- A key's value is rewritten if it is already in the dictionary.
- A new key-value pair is added to the dictionary if a key is missing.
Example of Updating Dictionary in Python
dictionary = {'name': 'Mohan Kumar', 'age': 35, 'city': 'New Delhi'}
update_data = {'age': 32, 'country': 'India'}
dictionary.update(update_data)
print(dictionary)
A dictionary is initialized with personal data by the code. The 'age' property is then updated, and a new 'country' key with matching values is added. It prints the updated dictionary at the end.
Output
{'name': 'Mohan Kumar', 'age': 32, 'city': 'New Delhi', 'country': 'India'}
Delete Dictionary Elements in Python
- In Python, deleting dictionary elements involves removing specific key-value pairs from the dictionary.
- The specified key-value pair is immediately deleted from the dictionary by using the del keyword.
- If the given key is not present in the dictionary, a KeyError is raised.
Example of Deleting Dictionary Elements in Python Compiler
dictionary = {"a": 1, "b": 2, "c": 3}
del dictionary["b"]
print(dictionary)
Output
{'a': 1, 'c': 3}
Built-in Dictionary Functions
Sr.No. | Function | Description |
1 | cmp(dict1, dict2) | Compares elements of both dict. |
2 | len(dict) | Gives the total length of the dictionary which would be equal to the number of items in the dictionary |
3 | str(dict) | Produces a printable string representation for that dictionary |
4 | type(variable) | Returns the type of the passed variable. If there is a passed variable in a dictionary, then it would return a dictionary type. |
Built-in Dictionary methods
Sr.No. | Methods | Description |
1 | dict.clear() | Removes all elements of dictionary dict |
2 | dict.copy() | Returns a shallow copy of dictionary dict |
3 | dict.fromkeys() | Create a new dictionary with keys from seq and values set to value. |
4 | dict.get(key, default=None) | For "key" key, returns value or default if the key is not in the dictionary |
5 | dict.has_key(key) | Returns true if key in dictionary dict, false otherwise |
6 | dict.items() | Returns a list of dict's (key, value) tuple pairs |
7 | dict.keys() | Returns list of dictionary dict's keys |
8 | dict.setdefault(key, default=None) | Similar to get(), but will set dict[key]=default if "key" is not already in dict |
9 | dict.update(dict2) | Adds dictionary dict2's key-values pairs to dict |
10 | dict.values() | Returns list of dictionary dict's values |