25
AprException Handling in Python: Try and Except Statement
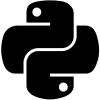
Python Programming For Beginners Free Course
Exception Handling in Python: An Overview
Exception Handling in Python deals with the techniques to deal with unwanted and unexpected events occurring during the program execution. With learning Python, one must be ready to deal with errors, unexpected inputs, or other exceptions. In this blog post on Python tutorial, we'll discuss how to use Python's tools for exception handling.
What is Exception Handling in Python?
Exception handling is a technique in Python for dealing with errors that occur during program execution. It entails spotting potential error situations, responding appropriately to exceptions when they arise, and identifying possible error conditions. Using the try and except keywords, Python provides a structured approach to exception handling.
Read More - 50 Python Interview Questions and Answers
Different Types of Exceptions in Python:
Various built-in Python exceptions can be thrown when an error occurs during program execution. Here are some of the most popular types of Python exceptions:
- SyntaxError: When the interpreter comes across a syntactic problem in the code, such as a misspelled word, a missing colon, or an unbalanced pair of parentheses, this exception is raised.
- TypeError: When an operation or function is done to an object of the incorrect type, such as by adding a string to an integer, an exception is thrown.
- NameError: When a variable or function name cannot be found in the current scope, the exception NameError is thrown.
- IndexError: This exception is thrown when a list, tuple, or other sequence type's index is outside of bounds.
- KeyError: When a key cannot be found in a dictionary, this exception is thrown.
- ValueError: This exception is thrown when an invalid argument or input is passed to a function or method. An example would be trying to convert a string to an integer when the string does not represent a valid integer.
- AttributeError: When an attribute or method is not present on an object, such as when attempting to access a non-existent attribute of a class instance, the exception AttributeError is thrown.
- IOError: This exception is thrown if an input/output error occurs during an I/O operation, such as reading or writing to a file.
- ZeroDivisionError: This exception is thrown whenever a division by zero is attempted.
- ImportError: This exception is thrown whenever a module cannot be loaded or found by an import statement.
Try and Except Statement – Catching Exceptions
- In Python, you may catch and deal with exceptions by using the try and except commands.
- The try and except clauses are used to contain statements that can raise exceptions and statements that handle such exceptions.
Example of Try and Except Statement in Python
try:
number = int(input("Enter a number: "))
result = 10 / number
print("The result is:", result)
except ZeroDivisionError:
print("Division by zero is not allowed.")
except ValueError:
print("Invalid input. Please enter a valid number.")
In this example in the Python Editor, the try block attempts to divide 10 by the input entered by the user. The result is calculated and reported if the input is a valid integer that is not zero. If the input is invalid (for example, a string) or zero, the corresponding except block raises an exception and displays an appropriate error message.
Output
Enter a number: 0
Division by zero is not allowed.
Catching Specific Exception
- To provide handlers for various exceptions, a try statement may contain more than one except clause.
- Please be aware that only one handler will be run at a time.
Example of Catching Specific Exception in Python
try:
file = open("test.txt", "r")
contents = file.read()
print(contents)
file.close()
except FileNotFoundError:
print("File not found.")
except IOError:
print("An error occurred while reading the file.")
This code will attempt to open the file "test.txt" and read its contents. The code will print an error message if the file is not found. If an error occurs while reading the file, the code will display an error message before proceeding to the next line of code.
Output
File not found.
Read More - Python Developer Salary
Try with Else Clause
- Python additionally allows the use of an else clause on a try-except block, which must come after every except clause.
- Only when the try clause fails to throw an exception does the code go on to the else block.
Example of Try with Else Clause in Python
try:
# Open a file named "test.txt" for reading
file = open("test.txt", "r")
# Read the contents of the file
contents = file.read()
# Close the file
file.close()
# Check if the contents of the file contain the word "Python"
if "Python" in contents:
# If the word "Python" is found, print a message
print("The word 'Python' is found in the file.")
else:
# If the word "Python" is not found, print a message
print("The word 'Python' is not found in the file.")
except FileNotFoundError:
# If the file "test.txt" is not found, print an error message
print("The file 'test.txt' could not be found.")
else:
# If no exceptions occur, print a message
print("The file 'test.txt' was successfully read.")
A try block is used in this example to read "test.txt" and look for the word "Python." If the file access is successful, the otherwise block is executed. If an exception occurs, such as FileNotFoundError, the error is handled by the associated except block.
Output
The file 'test.txt' could not be found.
Finally Keyword in Python
- The finally keyword is available in Python, and it is always used after the try and except blocks.
- The try block normally terminates once the final block executes, or after the try block quits because of an exception.
Example of Finally Keyword in Python
def divide_numbers(a, b):
try:
result = a / b
except ZeroDivisionError:
print("Error: Cannot divide by zero!")
else:
print(f"The result of {a} divided by {b} is: {result}")
finally:
print("This block always executes, regardless of exceptions.")
divide_numbers(10, 2)
divide_numbers(5, 0)
The code defines the function divide_numbers, which attempts to divide two numbers, prints the result if successful, handles the division by zero exception, and ensures that a final block of code (finally) always executes, regardless of whether an exception occurred or not.
Output
The result of 10 divided by 2 is: 5.0
This block always executes, regardless of exceptions.
Error: Cannot divide by zero!
This block always executes, regardless of exceptions.
Raising Exception
- The raise statement enables the programmer to compel the occurrence of a particular exception.
- Raise's lone argument specifies the exception that should be raised.
- Either an exception instance or an exception class (a class deriving from Exception) must be present here.
Example of Raising Exception in Python
def divide(a, b):
if b == 0:
raise ZeroDivisionError("Cannot divide by zero.")
return a / b
try:
result = divide(10, 2)
print("The result is:", result)
except ZeroDivisionError as e:
print("Error:", e)
The code in the Python Compiler implements a divide function to conduct division, throwing a ZeroDivisionError if the divisor is 0. It then attempts to call this method, prints the result if successful, and throws an exception if division by zero happens.
Output
The result is: 5.0
Advantages of Exception Handling
- Increased program dependability: By managing exceptions correctly, you can stop your program from crashing or generating wrong results because of unforeseen faults or input.
- Error handling made easier: It is simpler to comprehend and maintain your code when you can separate the error management code from the core program logic using exception handling.
- Simpler coding: With exception handling, you can write code that is clearer and easier to comprehend by avoiding the use of intricate conditional expressions to check for mistakes.
- Simpler debugging: When an exception is raised, the Python interpreter generates a traceback that identifies the precise place in your code where the error happened.
Disadvantages of Exception Handling
- Performance penalty: Because the interpreter must undertake an extra effort to detect and handle the exception, exception handling can be slower than utilizing conditional statements to check for mistakes.
- Code complexity increase: Handling exceptions can make your code more difficult, especially if you have to deal with a variety of exception types or use sophisticated error-handling logic.
- Possible security risks: It's critical to manage exceptions carefully and avoid disclosing too much information about your program because improperly handled exceptions may reveal sensitive information or lead to security flaws in your code.
Summary
Exception handling makes sure that any mistake or unforeseen input does not lead to the crashing of the program. Exception handling can be used to check both syntax levels and validations accordingly. Python Certification can help programmers learn these conventions and become more proficient in the language.