11
JulModules in Python
A Python module is a file containing Python code. This file includes functions, classes, and variables, and can also include runnable code. The file name is the module name with the .py extension.
With Modules in Python, you can break big programs into smaller parts to make them easier to read and manage. Using Modules in Python also saves time because you can reuse the same code in different projects.
In the Python tutorial, we will learn how to use Modules in Python, understand why they are useful, and see examples of how to create and import them in your programs.
What are the modules in Python?
Modules in Python are files that contain Python code, such as functions, variables, or classesthat you can use in other Python programs. They help organize code into smaller, reusable parts. You can use built-in modules like math or random, or create your own custom modules to keep your code clean and easy to manage.
Example
def print_func( par ):
print "Hello : ", par
return
Read More - 50 Python Interview Questions and Answers
Python import module
The Python import module is a way to bring code from one file (a module) into another so you can use its functions, variables, or classes. It helps you reuse existing code without writing everything from scratch. Using the import keyword, you can access built-in or custom modules easily in your program.
- You can import built-in modules like math, datetime, or random.
- Custom modules can be created and imported by saving Python code in .py files.
- The import keyword is used to load a module into your program.
- You can also import specific parts using module_name import function_name.
Syntax
import module1[, module2[,... moduleN]
Example in Python Compiler
# Import module support
import support
# Now you can call defined function that module as follows
support.print_func("Urmi")
Output
Hello : Urmi
Python path environment variable
The Python path environment variable is a setting that tells Python where to look for modules and packages when you use the import statement. It includes directories where Python will search for your code files. If your module is not in the standard location, you can add its path to this variable so Python can find and use it. Here are some key points about the Python path environment variable:
- It is stored in the PYTHONPATH environment variable on your system.
- You can add custom folders to PYTHONPATH to import your own modules.
- Python also checks built-in directories listed in sys.path.
- Modifying PYTHONPATH helps when working with large projects or external libraries.
A typical Python path environment variable from a Windows system is like:
set Python path = c:\python20\lib;
A typical Python path environment variable from a UNIX system −
set Python path = /usr/local/lib/python
Read More - Python Developer Salary
Namespace and scope in Python
1. Namespace in Python
A namespace in Python is like a box that stores all the names you create in your program, such as variables and functions. It helps Python know what each name refers to, so there’s no confusion, even if you use the same name in different places. Different namespaces are used in different parts of a program, like inside functions or in the main program.
2. Scope in Python
Scope in Python tells you where a variable or name can be used or accessed in your code. Some variables only work inside a function (local scope), while others work throughout the whole file (global scope). Python checks the scope to decide where it can find the value of a name.
Example
Money = 4500
def AddMoney():
# Uncomment the following line to fix the code:
# global Money
Money = Money + 1
print Money
AddMoney()
print Money
The dir( ) Function in Python
The dir() function in Python is used to list all the names (like variables, methods, or attributes) in the current scope or inside a given object. It helps you understand what functions or variables are available for use. This function is useful for exploring modules, classes, or objects without checking the documentation.
- dir() with no argument shows all names in the current local scope.
- dir(object) lists all attributes and methods of the specified object.
- It helps in inspecting modules, classes, or even built-in objects.
- Very useful for beginners to explore unknown modules or objects.
- Can assist in debugging by showing what names are defined at a certain point.
Example
# Import built-in module math
import math
content = dir(math)
print content
Output
['__doc__', '__file__', '__name__', 'acos', 'asin', 'atan',
'atan2', 'ceil', 'cos', 'cosh', 'degrees', 'e', 'exp',
'fabs', 'floor', 'fmod', 'frexp', 'hypot', 'ldexp', 'log',
'log10', 'modf', 'pi', 'pow', 'radians', 'sin', 'sinh',
'sqrt', 'tan', 'tanh']
The reload() Function in Python
The reload() function in Python is used to reload a previously imported module without restarting the interpreter. This is helpful when you make changes to a module and want to see the updates without closing and reopening your Python session. It is mainly used during development to test changes quickly.
- reload() is part of the importlib module in Python 3 (importlib.reload(module)).
- It re-imports a module that has already been imported earlier.
- Useful for testing updates in a module during development.
- Does not reload modules automatically; you have to call it manually.
- Must first import the module before using reload() on it.
Packages in Python
Packages in Python are a way to organize related Python modules into a single directory. A package is simply a folder that contains multiple Python files (called modules) and a special __init__.py file, which tells Python that the folder is a package. This helps in managing and structuring large codebases by grouping similar functionality together.
- A package is a directory with a collection of related Python modules.
- It must contain an __init__.py file (can be empty) to be recognized as a package.
- Packages help keep your code organized and reusable.
- You can import modules from a package using dot notation (e.g., package.module).
- Packages make it easier to manage large projects with many modules.
Use Cases of Python Modules
- Grouping utility functions used across multiple scripts
- Separating logic like database operations, user interface, and business logic
- Writing APIs or reusable components in large projects
How to Create a Python Module
To create a Python module,
- Write Python code in a file
- Save the file with a .py extension, and you’ve successfully created a user-defined Python module.
Example :
def greet(name)
return f"Hello, {name}!"
class Calculator:
def add(self, a, b):
return a + b
PI = 3.14159
Now save this file with a .py extension. (e.g. mymodule.py)
How to Import Python Modules with Examples
There are three ways to import modules in Python:
1. Importing an entire module
Example :
import math result = math.sqrt(25) pi_value = math.pi print("Square root of 25:", result) print("Value of pi:", pi_value)
Explanation:
- You import the entire math module. To use its functions/constants, you prefix them with math.
2. Importing specific items from a module:
Example:
from math import sqrt, pi
result = sqrt(49)
print("Square root of 49:", result)
printprint("Value of pi:", pi)
Explanation:
- You import only sqrt and pi from the math module. You don’t need to write math.sqrt()—just sqrt().
3. Re-naming the module while importing
Example:
import math as m
circle_area = m.pi * m.pow(5, 2)
print("Area of circle with radius 5:", circle_area)
Explanation:
- You renamed the math module to m, which makes the code shorter and potentially more readable, especially if the module name is long.
Locating Python Modules
When we import a Python module, the Python interpreter searches through a list of directories known as the module search path, which is stored in the sys.path variable.
Python interpreter searches for the module in the following manner -
- First, it checks the current directory where your script is being executed.
- If the module is not found in the current directory, then Python looks in the directories that are specified by the PYTHONPATH environment variable.
- And at last, it searches the default installation directories where its standard library and other modules are stored.
Types of Python Modules
Python has three main types of modules:
- Built-in modules
- User-defined modules
- Third-party modules
Let’s explore each type of Python module in detail with examples.
Python Built-in Modules
The standard library features several built-in Python modules such as math, random, os, datetime, and others. These modules are pre-installed with Python and provide a variety of functionalities, allowing developers to perform common tasks without having to write code from scratch.
# importing built-in module math
import math
# using square root(sqrt) function contained
# in math module
print(math.sqrt(25))
# importing built-in module random
import random
# printing a random integer between 0 and 5
print(random.randint(0, 5))
import datetime
from datetime import date
import time
# Returns the number of seconds since the
# Unix Epoch, January 1st, 1970
print(time.time()
# importing built-in module os
import os
# Get current working directory
print(os.getcwd())
# importing built-in module sys
import sys
# Get command line arguments
print(sys.argv)
# Get Python version
print(sys.version)
Python User-defined Modules
A user-defined Python module is a user-created file saved with .py extension. These Python files include functions, classes, and variables. These modules help you organize your code, enhance reusability, and increase readability. They enable you to decompose large programs into smaller, more manageable components.
Python Third-party modules:
A third-party module in Python is defined as a module that is neither pre-installed in Python nor created by the user. Such modules are produced by external developers or organizations and enhance Python's features by offering additional functionalities. These modules can be downloaded using pip, the official package installer for Python.
Example HTTP module:
To install a popular third-party module like requests for making HTTP requests, one would use the following command in the terminal: pip install requests To use it in our project:
import requests
response = requests.get("https://www.example.com")
print(response.status_code)
Dir() Function Use in Python Modules:
The dir() function in Python lists all the attributes and methods inside the module. This includes:
- Functions: All functions defined within the module.
- Classes: All classes defined within the module.
- Variables: All global variables defined within the module.
- Imported names: Names imported from other modules into the current one.
- Special attributes: Built-in attributes of the module, such as __name__, __doc__, __file__, etc.
Example:
import math
math_contents = dir(math)
print(math_contents)
Explanation:
- Import math: This line imports the math module from the Python standard library.
- math_contents=dir(math): This line stores all the attributes and methods of the math module into the math_contents variable.
- print(math_contents): This line prints a list of all attributes and methods in the 'math' module
Difference Between Python Modules and Packages:
Feature | Python Module | Python Package |
Definition | A single Python file (.py) | A directory containing multiple modules and an __init__.py file |
Structure | Single file | A folder with multiple .py files |
File Type | .py file | Folder with __init__.py |
Usage | Used to define functions, classes, and variables | Used to organize related modules |
Example | math.py | myproject/ with files like utils.py, models.py, and __init__.py |
So, while a Python module is a single file, a package is a collection of related modules. Knowing the difference between modules and packages in Python is crucial for large projects.
Conclusion
In this article, we explored Python modules in detail. Modules in Python help make your code cleaner, reusable, and easier to manage. By using modules, you can break your program into smaller parts and avoid repeating code. Whether it's built-in or custom, modules in Python are a powerful way to organize and share functionality across different files.
Learning to use modules in Python is an important step toward writing better and more efficient Python programs. If you want to further expand your skills in Python to land a high-paying job, check out our Free Python Certification course.
FAQs
Yes. You can import other modules within your custom Python module just like in a script.
__init__ makes directory a valid Python package. Without it, Python won’t recognize it as a package.
Use importlib.reload(module_name) from the importlib module.
Take our Python skill challenge to evaluate yourself!
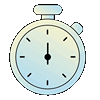
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.