25
AprOperators in Python
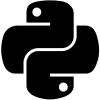
Python Programming For Beginners Free Course
Operators in Python: An Overview
Operators in Python Programming are the fundamental concepts that are important to know. These operators are needed to perform various operations in Python such as arithmetic calculations, logical evaluations, bitwise manipulations, etc. In this Python Tutorial, you will get to know about various Types of operators in Python with Example. If you are new to python and want to learn it from scratch, our Python Certification Training will help you with that.
Read More: Top 50 Python Interview Questions and Answers
Types of operators in Python
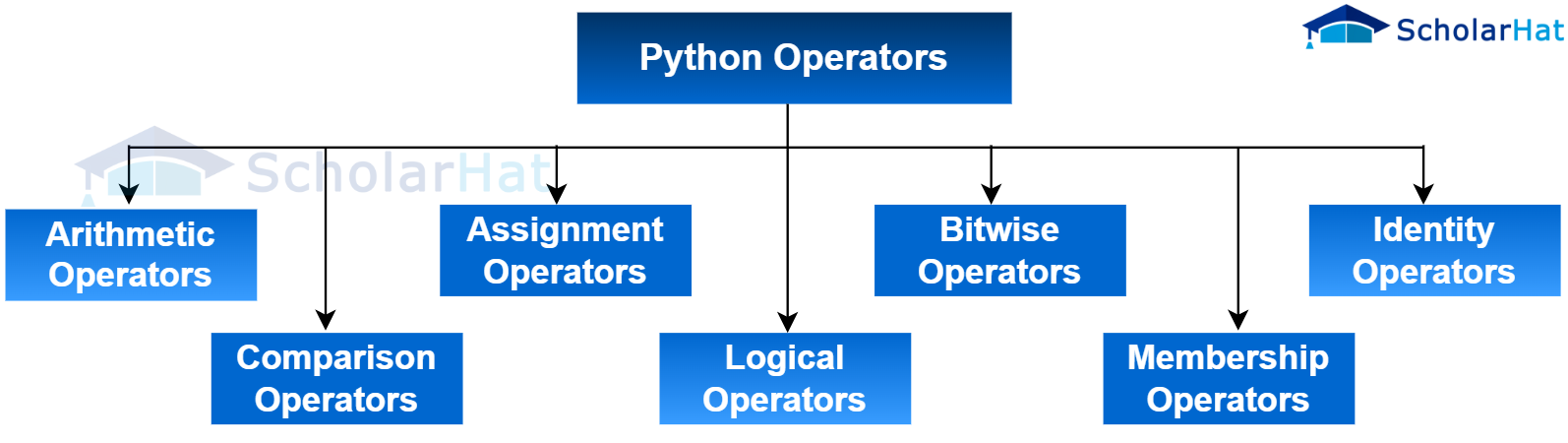
In Python Programming, Operators are those symbols that are used to carry out certain operations on operands. These operations could be arithmetic, logical or bitwise. There are some of the basic operators used in Python that you should definitely know that are as follows:
Arithmetic Operators
The Arithmetic Operators are used to perform certain mathematical operations such as addition and subtraction. Use these operators on numeric operands. Following are the basic arithmetic operators used in Python:
Operator | Description | Syntax |
Addition (+) | Used for adding two operands. | a + b |
Subtraction (-) | Used for subtracting the second operand from the first. | a - b |
Multiplication (*) | Used for multiplying two operands. | a * b |
Division (/) | Used for dividing first operand by the second. It will always return a floating point number. | a / b |
Floor Division (//) | Used for dividing first operand by the second. It returns a rounded off integer as the answer. | a // b |
Modulus (%) | It also divides first operand by the second and returns the remainder of the division. | a % b |
Exponentiation (**) | Used for raising the first operand to the power of the second. | a ** b |
Note:Python follows PEMDAS as the standard order of operations where Parentheses, Exponentiation, Multiplication, Division, Addition, Subtraction is performed in sequence.
Example of Arithmetic Operators in Python
# Arithmetic Operators Example
# Addition
a = 5
b = 4
sum_result = a + b
print("Addition:", sum_result)
# Subtraction
difference = a - b
print("Subtraction:", difference)
# Multiplication
product = a * b
print("Multiplication:", product)
# Division
quotient = a / b
print("Division:", quotient)
# Floor Division
floor_division = a // b
print("Floor Division:", floor_division)
# Modulus
remainder = a % b
print("Modulus:", remainder)
# Exponentiation
exponent = a ** b
print("Exponentiation:", exponent)
Explanation:
Here, we have defined variables 'a' & 'b' with values 5 and 4 respectively. Then, we have performed different arithmetic function with these variables and printed the results as below output.
Output
Addition: 9
Subtraction: 1
Multiplication: 20
Division: 1.25
Floor Division: 1
Modulus: 1
Exponentiation: 625
Read More: Python Developer Salary
Comparison Operators
Operator | Description | Syntax |
Equal to (==) | Used for checking if the two operands are equal. | a == b |
Not equal to (!=) | Used for checking if two operands are not equal. | a != b |
Greater than (>) | Used for checking if the left operand is greater than the right operand. | a > b |
Less than (<) | Used for checking if the left operand is less than the right operand. | a < b |
Greater than or equal to (>=) | Used for checking if the left operand is either greater than or equal to the right operand. | a >= b |
Less than or equal to (<=) | Used for checking if the left operand is either less than or equal to the right operand. | a <= b |
Example of Comparison Operators in Python
# Comparison Operators Example
# Variables
a = 5
b = 2
# Equal to (==)
print("Equal to:", a == b)
# Not equal to (!=)
print("Not equal to:", a != b)
# Greater than (>)
print("Greater than:", a > b)
# Less than (<)
print("Less than:", a < b)
# Greater than or equal to (>=)
print("Greater than or equal to:", a >= b)
# Less than or equal to (<=)
print("Less than or equal to:", a <= b)
Explanation:
Output
Equal to: False
Not equal to: True
Greater than: True
Less than: False
Greater than or equal to: True
Less than or equal to: False
Logical Operators
Operator | Description | Syntax |
Logical AND (and) | It will return 'True' if both the operands are true. | a and b |
Logical OR (or) | It will return 'True' if at least one of the operands is true. | a or b |
Logical NOT (not) | It will return the exact opposite of the boolean value of the operand. | not a |
Example of Logical Operators in Python
# Logical Operators Example
# Variables
a = True
b = False
# Logical AND (and)
result_and = a and b
print("Logical AND:", result_and)
# Logical OR (or)
result_or = a or b
print("Logical OR:", result_or)
# Logical NOT (not)
result_not_a = not a
result_not_b = not b
print("Logical NOT for a:", result_not_a)
print("Logical NOT for b:", result_not_b)
Explanation:
Output
Logical AND: False
Logical OR: True
Logical NOT for a: False
Logical NOT for b: True
Bitwise Operators
Operator | Description | Syntax |
Bitwise AND (&) | Used for setting each bit to 1, if both bits are 1. | a & b |
Bitwise OR (|) | Used for setting each bit to 1, if at least on of the bits is 1. | a | b |
Bitwise XOR (^) | Used for setting each bit to 1, if only one of the bits is 1. | a ^ b |
Bitwise NOT (~) | Used for inverting all the bits. | a ~ b |
Left Shift (<<) | It will shift the bits of first operand to the left by the same number of positions as specified by the second operand. | a << b |
Right Shift (>>) | It will shift the bits of first operand to the right by the same number of positions as specified by second operand. | a >> b |
Example of Bitwise Operators in Python
# Bitwise Operators Example
# Variables
a = 5 # Binary: 0101
b = 3 # Binary: 0011
# Bitwise AND (&)
result_and = a & b
print("Bitwise AND:", result_and)
# Bitwise OR (|)
result_or = a | b
print("Bitwise OR:", result_or)
# Bitwise XOR (^)
result_xor = a ^ b
print("Bitwise XOR:", result_xor)
# Bitwise NOT (~)
result_not_a = ~a
result_not_b = ~b
print("Bitwise NOT for a:", result_not_a)
print("Bitwise NOT for b:", result_not_b)
# Left Shift (<<)
result_left_shift_a = a << 1
print("Left Shift for a:", result_left_shift_a)
# Right Shift (>>)
result_right_shift_b = b >> 1
print("Right Shift for b:", result_right_shift_b)
Explanation:
Output
Bitwise AND: 1
Bitwise OR: 7
Bitwise XOR: 6
Bitwise NOT for a: -6
Bitwise NOT for b: -4
Left Shift for a: 10
Right Shift for b: 1
Read More: Python Career Opportunities: Is it worth learning Python in 2024?
Assignment Operators
Operator | Description | Syntax |
Assignment (=) | Used for assigning value of the right operand to the left operand. | a = b |
Addition Assignment (+=) | Used for adding the value of the right operand with the left operand and assigning the result value to the left operand. | a += b; a = a + b |
Subtraction Assignment (-=) | Used for subtracting the value of the right operand from the left operand and assigning the result value to the left operand. | a -= b; a = a - b |
Multiplication Assignment (*=) | Used for multiplying the value of left operand by the value of right operand and assigning the result value to the left operand. | a *= b; a = a * b |
Division Assignment (/=) | Used for dividing the value of left operand by the value of right operand and assigning the result value to the left operand. | a /= b; a = a / b |
Modulus Assignment (%=) | Used for computing modulus of the left operand with the right operand and assigning the result value to the left operand. | a %= b; a = a % b |
Floor Division Assignment (//=) | Used for performing floor division of the left operand by the right operand and assigning the result value to the left operand. | a //= b; a = a // b |
Exponentiation Assignment (**=) | Used for raising the power of the right operand to the left operand and assigning the result value to the left operand. | a **= b; a = a ** b |
Example of Assignment Operators in Python
# Assignment Operators Example
# Variables
a = 10
b = 5
# Assignment (=)
a = 10
print("Assignment (=):", a)
# Addition Assignment (+=)
a += 3
print("Addition Assignment (+=):", a)
# Subtraction Assignment (-=)
a -= 2
print("Subtraction Assignment (-=):", a)
# Multiplication Assignment (*=)
a *= 4
print("Multiplication Assignment (*=):", a)
# Division Assignment (/=)
a /= 2
print("Division Assignment (/=):", a)
# Modulus Assignment (%=)
a %= 3
print("Modulus Assignment (%=):", a)
# Floor Division Assignment (//=)
a //= 2
print("Floor Division Assignment (//=):", a)
# Exponentiation Assignment (**=)
a **= 2
print("Exponentiation Assignment (**=):", a)
# Assignment (=) with variable b
b = a
print("Assignment (=) with variable b:", b)
Explanation:
Output
Assignment (=): 10
Addition Assignment (+=): 13
Subtraction Assignment (-=): 11
Multiplication Assignment (*=): 44
Division Assignment (/=): 22.0
Modulus Assignment (%=): 1.0
Floor Division Assignment (//=): 0.0
Exponentiation Assignment (**=): 0.0
Assignment (=) with variable b: 0.0
Identity Operators
Operator | Description | Syntax |
Identity (is) | It will return 'True', only if both operands are referring to the same object. | a is b |
Not identity (is not) | It will return 'True', only if both operands are not referring to the same object. | a is not b |
Example of Identity Operators in Python
# Identity Operators Example
# Variables
a = 4
b = 5
# Identity (is)
print("Identity (is):")
print("a is b:", a is b) # False
print("a is not b:", a is not b) # True
# Update variable b to reference the same object as a
b = a
# Check identity after update
print("\nAfter updating variable b:")
print("a is b:", a is b) # True
print("a is not b:", a is not b) # False
Explanation:
Output
Identity (is):
a is b: False
a is not b: True
After updating variable b:
a is b: True
a is not b: False
Membership Operators
Operator | Description | Syntax |
In | It will return 'True', if the specified value is present in the sequence. | a in sequence |
Not In | It will return 'True', if the specified value is not present in the sequence. | a not in sequence |
Example of Membership Operators in Python
# Membership Operators Example
# List
numbers = [1, 2, 3]
# In operator
print(2 in numbers) # True
# Not in operator
print(4 not in numbers) # True
# String
string = "Hello"
# In operator with string
print('e' in string) # True
# Not in operator with string
print('z' not in string) # True
Explanation:
Output
True
True
True
True
Best Practices
Guidelines for using operators effectively
- You must use parentheses to write more readable and clearer expressions.
- Python allows for chaining operators but using it in excess will only make the code more complex and difficult to read so avoid it as much as you can.
- Use names for variables that clearly describe the purpose of the operands.
- Pay extra attention while using Membership and Identity operators.
- Use Bitwise operators only when necessary as they can be a little complicated to use.
- Test your code thoroughly to check if all the operators are working correctly or not.
Tips for writing clean and efficient code
- It is always advisable to sticking to the coding standards and style guidelines like PEF 8 (Python), Google's Python Style Guide.
- Make variable names as descriptive and meaningful as possible.
- Maintain the conciseness of your functions so that it is easy to read, test and debug.
- Avoid repetition of the code.
- Write comments explaining the logic wherever needed clearly and concisely.