11
JulAddition of Two Numbers in Python
How to Add Two Numbers in Python?
Adding numbers in Python is one of the simplest arithmetic operations in Python. After having learned about variables, data types, input and output, and various operators in Python, it's time to implement them.
In this Python tutorial, we'll practically understand all the methods to add two numbers in Python. But, before proceeding, you must be thorough with the topics mentioned above. Additionally, enrolling in a Python for Data Scientists with AI training can help you build a strong foundation and apply Python effectively in data science and AI projects.
If not refer to these:
- What are Python Variables - Types of Variables in Python Language.
- Data Types in Python
- What are Operators in Python - Types of Operators in Python ( With Examples )
Ways to Perform Addition of Two Numbers in Python
1. Using the "+" Operator
This is the simplest addition method in Python. Place the "+" arithmetic operator between two numbers you want to add and you will get the sum.
Program to Add Two Numbers in Python
num1 = 5
num2 = 3
result_addition = num1 + num2
print("Addition of num1 and num2: ", result_addition)
We have two numbers inside the variables num1 and num2. Both of them are added and the result is stored in the result_addition variable.
Output
Addition of num1 and num2: 8
Using User Input
We take two numbers from a user through the Python input() function and store them in the variables. We add those numbers using the "+" operator.
Program to Add Two Numbers in Python Using User Input
num1 = input('Enter first number: ')
num2 = input('Enter the second number: ')
sum = float(num1) + float(num2)
print('The sum of {0} and {1} is {2}'.format(num1, num2, sum))
We have two numbers inside the variables num1 and num2. Both are added and the result is stored in the sum variable.
Output
Enter first number: 67
Enter the second number: 89
The sum of 67 and 89 is 156.0
2. Using the "+=" Operator
The "+=" is the addition assignment operator in Python. It adds the right operand to the left operand and assigns the result to the left operand.
Program Implementing the "+=" Operator to Add Two Numbers in Python
num1 = 58
num2 = 100
# addition using the '+=' operator
num1 += num2
print("The sum of", num1, "and", num2, "is", num1)
We have two numbers inside the variables num1 and num2. num1 is added with num2 and the result gets stored in num1.
Output
The sum of 158 and 100 is 158
3. Using User-Defined Function
We can create a function to add two numbers in Python. The function will perform addition on the two numbers given as arguments using the "+" operator.
Program Implementing Function to Add Two Numbers in Python
def add(a,b):
return a+b
num1 = 100
num2 = 507
#function calling and store the result into sum
sum = add(num1,num2)
print("Sum of {0} and {1} is {2};" .format(num1, num2, sum))
The function add() takes two arguments two numbers inside the variables num1 and num2. Both are added and the result is stored in the sum variable.
Read More: Python Functions
Output
Sum of 100 and 507 is 607
4. Using operator.add() Method
The operator module provides functions corresponding to the built-in operators. We can add two numbers using the operator.add() method. It'll take two numbers as arguments and add them.
Program Implementing operator.add() Method to Add Two Numbers in Python
num1 = 158
num2 = 12
import operator
sum = operator.add(num1,num2)
print("Sum of {0} and {1} is {2}" .format(num1, num2, sum))
The program adds num1 and num2 using the operator.add() method and stores the result in the sum variable.
Output
Sum of 158 and 12 is 170
5. Using Lambda Function
We can use the Python lambda function to add two numbers in Python.
Program Implementing Lambda Function to Add Two Numbers in Python
add_numbers = lambda a, b: a + b
num1 = 145
num2 = 318
# lambda function
sum = add_numbers(num1, num2)
print("The sum of", num1, "and", num2, "is", sum)
The program adds num1 and num2 using the lambda function, add_numbers and stores the result in the sum variable.
Output
The sum of 145 and 318 is 463
6. Using the Built-In sum() Function
The sum() function in Python takes a list of numbers and adds them.
Program Implementing Built-In sum() Function to Add Two Numbers in Python
num1 = 590
num2 = 105
sum = sum([num1, num2])
print("The sum of", num1, "and", num2, "is", sum)
The program adds num1 and num2 using the sum function and stores the result in the sum variable.
Output
The sum of 590 and 105 is 695
Read More: Python Lists: List Methods and Operations
Read More: |
Summary
We saw all possible methods to perform the addition of two numbers in Python. You can use any of the above methods. All are very easy to understand and implement.
Take our Python skill challenge to evaluate yourself!
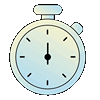
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.