27
JunPython Design Patterns - Basics to Advanced (2025 Guide)
If you’re a Python developer looking to write cleaner, more efficient, and maintainable code, understanding Python Design Patterns is a game changer. Whether you're building a small script or architecting a large-scale application, design patterns help solve common coding problems with proven, reusable solutions
So, in this Python tutorial, we’ll break down the world of Python design patterns step by step. You’ll learn what they are, the rules behind when to use them, and walk through real examples of Creational Patterns, Structural Patterns, and Behavioral Patterns. Plus, if you’re thinking about growing your Python skills for real-world projects or interviews, mastering Python design patterns is a great place to start. Let’s explore them one by one!
Read More: Python Programming Course For Beginners |
What are Python Design Patterns?
Design patterns are common ways to solve problems that come up when you’re building software. They’re like smart tricks that developers use to make their code better. In Python design patterns, you use these ideas to make your code easier to read, fix, and grow.
Types of Design Patterns in Python
There are three main types of design patterns in Python:
1. Creational Design Patterns:
Creational Design Patterns in Python are used to control how objects are created in a program. Instead of creating objects the usual way, these patterns give you flexible and smart ways to build them. They help keep your code clean and make it easy to update when things change.
Types of Creational Design Patterns in Python:
1.1 Factory Method
The Factory Method is a creational design pattern that helps you create objects without directly saying which class to use. Instead, it lets the method or subclass decide which object to create, making things more flexible.
1.2 Abstract Factory Method
1.3 Builder Method
1.4 Prototype Method
1.5 Singleton Method
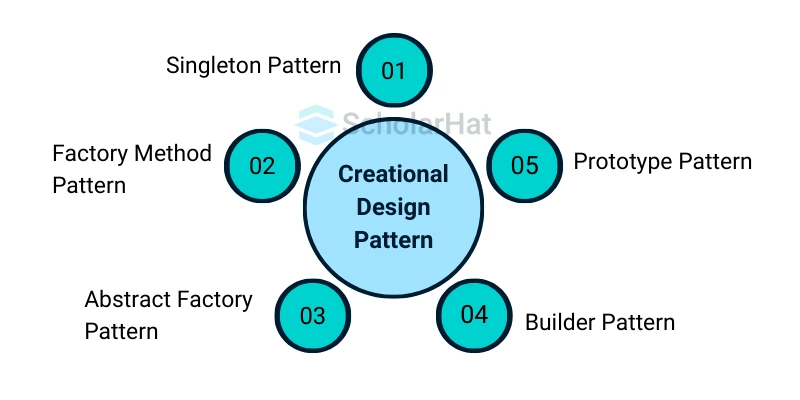
2. Structural Design Patterns in Python:
Structural Design Patterns in Python are all about how you arrange and connect objects to build bigger, more flexible systems. These patterns make it easier to add new features without changing much of the existing code. They help you keep your code organized, especially when multiple parts need to work together. Some common examples include Adapter, Facade, and Proxy.
Types of Structural Design Patterns in Python:
2.1 Adapter Method
2.2. Bridge Method
2.3 Composite Method
2.4 Decorator Method
2.5 Facade Method
2.6 Proxy Method
2.7 Flyweight Method
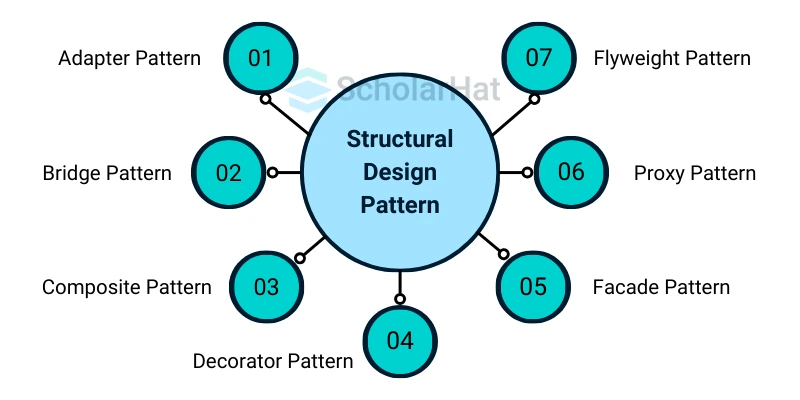
3. Behavioral Design Patterns in Python:
Python design patterns for Behavioral Patterns focus on how objects interact and communicate with one another. These patterns help define how objects share responsibilities, manage behavior, and control the flow of data. They improve flexibility and scalability, especially in more complex systems. Examples of Python design patterns in this category include the Observer, Strategy, and Command patterns.
Read More: Python For Data Science and AI Certification Training |
Types of Behavioral Design Patterns in Python:
3.1 Command Method
The Command Method is a behavioral design pattern that turns a request into a stand-alone object. It allows you to parameterize objects with actions, queue requests, and log the requests. This pattern helps in decoupling the sender and receiver of a request.
3.2 Observer Method
3.3 Mediator Method
3.4 Memento Method
3.5 Observer method
The Observer Method is a behavioral design pattern that allows one object (the subject) to notify multiple dependent objects (observers) when its state changes. It is useful for building event-driven systems, where changes in one part of the program need to be reflected in others without tightly coupling the components.
3.6 State Method
3.7 Strategy Method
The Strategy Method is a behavioral design pattern that allows you to define a family of algorithms and make them interchangeable. This pattern lets you select an algorithm at runtime, making it easier to change the behavior of an object without modifying its code.
3.8 Template Method
3.9 Visitor Method
3.10 Interpreter Design Pattern
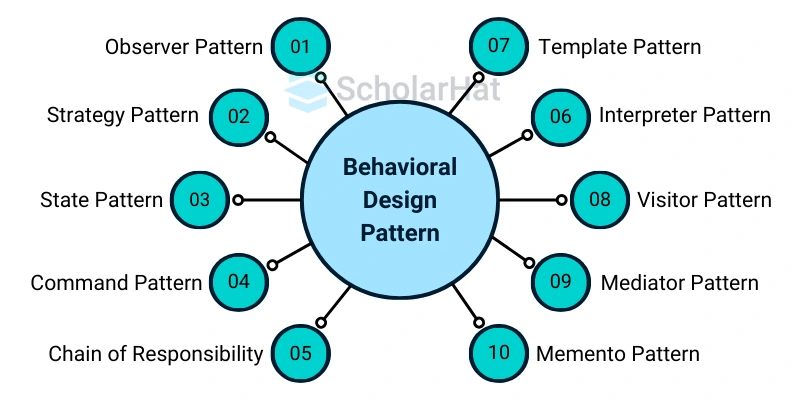
When to Use Design Patterns in Python
- Recurring Problems: Python design patterns solve common problems with ready-to-use solutions, so you don’t have to keep making the same fixes. They help save time and effort by providing proven methods to deal with recurring issues.
- Flexibility and Reusability: Python design patterns help you make code that is easy to change and reuse without messing up other parts of the system. This makes your code more adaptable for future updates and changes.
- Design Principles: Design patterns follow basic rules like keeping things simple and separate, making your code easier to manage. These principles ensure that your code stays organized and scalable as the project grows.
- Communication: Python design patterns like Mediator and Observer make it easier for objects to talk to each other without getting too complicated. This improves the structure of your code and reduces unnecessary dependencies.
- Performance: Python design patterns like Flyweight and Singleton help save memory and make your program run faster, especially when it gets bigger. By reusing objects and managing resources better, performance improves significantly.
When not to Use Python Design Patterns
- Over-Engineering: Using design patterns in Python when they aren't necessary can lead to over-engineering, making your code more complicated than it needs to be, especially for simple problems.
- Premature Optimization: Applying design patterns too early can cause premature optimization. It’s important to first ensure the solution works well, and then, if needed, refactor with design patterns for future scalability.
- Unfamiliarity: If you or your team is not familiar with a specific design pattern, using it might cause confusion and errors, making the code harder to maintain and understand.
- Project Constraints: If your project has tight time or resource constraints, implementing design patterns might add unnecessary complexity and delay, instead of focusing on delivering a simple working solution.
- Changing Requirements: In projects with frequent requirement changes, applying design patterns too early may lead to unnecessary revisions as the pattern may no longer fit with evolving needs.
How to Start Learning Python Design Patterns
Learning design patterns in Python is a great way to write cleaner and more reliable code. They give you smart ways to solve common coding problems, so you don’t have to start from scratch every time. Using patterns makes your Python projects easier to manage and grow.
Understand the basics: Learn key OOP concepts like classes, objects, methods, attributes, inheritance, and encapsulation. These are the building blocks for understanding design patterns in Python.
Familiarize yourself with the core patterns: Learn the three primary types of design patterns: creational, such as Singleton and Factory; structural, like Adapter and Decorator; and behavioral, such as Observer and Strategy. These categories help you apply the right pattern based on the problem you're solving.
Use online resources: Explore practical examples of design patterns through trusted coding websites and tutorials. Seeing how experienced developers apply these patterns in real-world projects can deepen your understanding and clear up any confusion.
Implement patterns yourself: Once you've studied examples, start using design patterns in your own Python code. Applying them in real projects helps reinforce what you've learned and builds your confidence in solving design problems.
Read More: Data Structures in Python |
Real-World Examples and Applications
When you are working on large or complex software in Python, using the right Python design patterns can make your code clean, easy to manage, and more powerful. Below are some real-world places where Python design patterns are used:
- Singleton Pattern: The Singleton Pattern is often used in logging systems. You want only one logger object to manage all logs in the app, so you don't create multiple loggers by mistake.
- Factory Pattern: Factory Pattern is used when you are building different types of objects, but want to keep the code simple and avoid repetition. This is common in web frameworks like Django for creating different types of views.
- Observer Pattern: The Observer Pattern is useful when many parts of your app need to update after one change. You see this in apps where user interfaces change in real-time, like in chat applications or dashboards.
- Decorator Pattern: The Decorator Pattern is great when you want to add new features to your functions without changing the actual function. It's used a lot in Flask and Django to add things like login checks or logging.
- Strategy Pattern: The Strategy Pattern helps when your code needs to switch between different ways of doing something. It’s used in machine learning models where you want to try different algorithms without changing too much code.
Best Practices for Using Python Design Patterns
- Start with the Problem: Use a pattern only if the problem repeats. Don’t overthink it.
- Keep Code Simple and Clean: Patterns like Factory or Singleton can make your code easy to read.
- Use Python’s Features: Python gives you tools like decorators and modules; use them instead of forcing complex patterns.
- Use Patterns to Avoid Repeat Code: If you're copying logic, try a pattern to reduce duplication.
- Don’t Use All Patterns: Just use what fits. Simple code is better than complex code.
When to Use a Python Design Pattern
If you need to... | Use this pattern |
Create objects in a clean and flexible way | Factory / Builder |
Keep only one shared object in your app | Singleton |
Change how something works while running | Strategy / State |
Replace long if-else or switch statements | Chain of Responsibility |
React to events like clicks or changes | Observer / Command |
Summary:
Mastering Python Design Patterns is essential for developers in 2025. Learning key patterns like Singleton, Factory, and Observer will help you write cleaner, more efficient code, improve your coding skills, and help you stand out in the competitive job market. Start following our roadmap today to become a Python design pattern expert. Additionally, you can boost your Python skills with the Free Python Programming Course for Beginners and explore Data Science with Python in the certification course.
Let’s climb to the top test your knowledge and conquer every question
Q 1: Which pattern ensures one instance?
FAQs
Take our Python skill challenge to evaluate yourself!
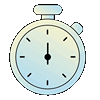
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.