Understanding Python While Loop with Examples
Python while Loop
Python while loop is a control flow statement used in Python Language. Previously, in Types of Loops in Python, we saw in brief that the while Loop is an entry-controlled loop. This loop is used to iterate a part of the code while the given condition remains true. The test takes place before each iteration.
In this Python tutorial, we will be learning What is Python while loop, while loop syntax in Python. We will also try to understand Python while loop programs. You can explore more about different other concepts of Python programming by enrolling in our Python Certification Training.
Read More: Top 50 Python Interview Questions and Answers
What is Python while loop?
Python while loop repeatedly carries out a series of instructions till a condition is true. When the condition becomes false, the line immediately after the loop in the program is executed.
Flowchart of Python while loop
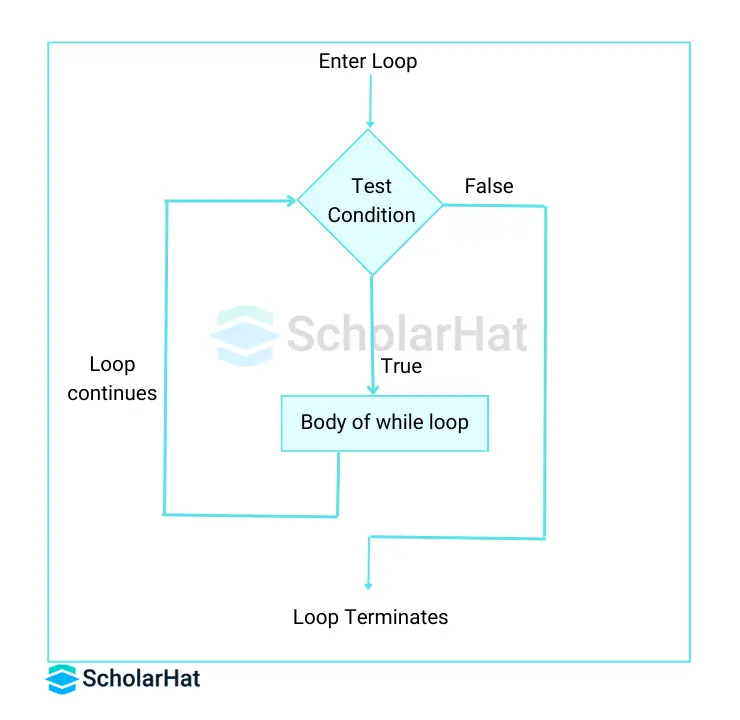
- In Python while loop, statement(s) may be a single statement or a block of statements with a uniform indent.
- When a while loop is executed, the expression is first evaluated in a Boolean context and if it is true, the loop body is executed.
Python while loop syntax
while expression:
statement(s)
Here,
- The Python while loop evaluates the condition.
- The condition may be any expression, and true is any non-zero value.
- If the condition turns true, the body of the while loop is executed.
- Once again the condition is evaluated.
- The cycle continues until the condition is False.
- Once the condition evaluates to False, the loop terminates.
Read More: Python Developer Salary
Python while loop Example
number = 5
product = 1
while number != 0:
product *= number
number-=1
print('The product is', product)
In the above program in the Python Editor:
- The number variable is initialized to 5.
- The loop will run till the number does not become 0.
- When the number becomes 0, the loop terminates and the program displays the product.
Output
The product is 120
Infinite while Loop in Python
If the condition of a loop is always true, the loop becomes infinite. Therefore, the loop's body is run infinite times until the memory is full.
i = 0
while True:
i += 1
print("i is:", i)
Output
i is: 1
i is: 2
i is: 3
i is: 4
i is: 5
...
Control Statements in Python with Examples
We have learned that loop control statements are used to alter the regular flow of a loop. They enable you to skip iterations, end the loop early, or do nothing at all. In general, the control statements can be categorized into three types that are:
- Conditional Statements (if, elif, else) The conditional statements are the decision making statements that are based on particular conditions. You can put specific conditions on different blocks of code and execute them.
- Looping Statements (for, while) The loops in Python are useful when you want to execute a block of code repeatedly.
- Control Flow Statements (break, continue, pass) The control flow statements are used for altering the flow of control within the loops or conditional statements.
Example Program 1:(simple if-else statement)
# Define a variable
x = 10
# Check if x is greater than 5
if x > 5:
print("x is greater than 5")
else:
print("x is not greater than 5")
It will check if variable 'x' is greater than 5 or not and print the block of code accordingly.
Output
x is greater than 5
Example Program 2:(nested if-elif-else statement)
# Define a variable
x = 5
# Check multiple conditions
if x > 5:
print("x is greater than 5")
elif x == 5:
print("x is equal to 5")
else:
print("x is less than 5")
It will check if variable 'x' is greater than 5, then go to the 'elif' code to check if it is equal to 5. If no condition is met, 'else' code will be printed.
Output
x is equal to 5
Example Program 1: (for loop)
# Iterate over a list
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
It will check all the elements of the specified list and print them as shown in below output.
Output
apple
banana
cherry
Example Program 2: (while loop)
# Initialize a counter
count = 0
# Execute the loop while count is less than 5
while count < 5:
print(count)
count += 1
It will execute the block of code till the condition of the while loop is met.
Output
0
1
2
3
4
Example Program 1: (break statement)
# Exit the loop when count becomes 3
for count in range(1, 6):
if count == 3:
break
print(count)
The execution will take place till the 'if' condition is evaluated to be true, then it will terminate the loop completely and proceeds to the first statement following the loop.
Output
1
2
Example Program 2: (continue statement)
# Skip printing even numbers
for count in range(1, 6):
if count % 2 == 0:
continue
print(count)
It will evaluate the 'if' condition and when it turns out to be true, the execution of that value will be skipped continuing to the next one.
Output
1
3
5
Example Program 3: (pass statement)
# Use pass statement to do nothing
for count in range(1, 6):
pass
It will simply skip the preceding block of code.
Using else Statement with while Loop in Python
A Python while loop can have an optional else clause. This else clause executes when the condition becomes false before the control shifts to the main line of execution.
Syntax:
while condition:
# Code block to execute while condition is True
else:
# Code block to execute when condition becomes False
Python while Loop example with else Statement
ctr = 0
while ctr < 5:
print('This is inside the while loop loop')
ctr = ctr + 1
else:
print('This is inside the else block of the while loop')
Here in the Python Online Editor, on the sixth iteration, the ctr becomes 5 which terminates the loop. It then executes the else block and prints the statement inside the else block.
Output
This is inside the while loop loop
This is inside the while loop loop
This is inside the while loop loop
This is inside the while loop loop
This is inside the while loop loop
This is inside the else block of the while loop
Remember: The else clause won’t be executed if the list is broken out with a break statement.
x = 0
while x < 7:
if x == 5:
break
print(x)
x += 1
else:
print("Finally finished!")
Here, there is a break statement that executes when x becomes 5. Hence, the control comes out of the while loop without the execution of the else statement.
Output
0
1
2
3
4
Python while loop with Python list
Python while loop can be used to execute a set of statements for each of the elements in the list in Python.
list = [11, 12, 13, 14]
while list:
print(list.pop())
Output
14
13
12
11
Python While Loop Multiple Conditions
With the help of logical operators in Python, we can merge two or more expressions specifying conditions into a single while loop. This instructs Python on collectively analyzing all the given expressions of conditions. Look at the below example in Python Compiler to understand it better:
# Initialize variables
x = 0
y = 0
# Run the loop until both x and y are less than 5
while x < 5 and y < 5:
print("x:", x, "y:", y)
x += 1
y += 2
Explanation:
Output
x: 0 y: 0
x: 1 y: 2
x: 2 y: 4
Exercises of Python While Loop in Python Compiler
1. Printing all letters except some using Python while loop
i = 0
word = "Welcome to ScholarHat"
while i < len(word):
if word[i] == "e" or word[i] =="o":
i += 1
continue
print("Returned letter",word[i])
i += 1
Output
Returned letter W
Returned letter l
Returned letter c
Returned letter m
Returned letter
Returned letter t
Returned letter
Returned letter S
Returned letter c
Returned letter h
Returned letter l
Returned letter a
Returned letter r
Returned letter H
Returned letter a
Returned letter t
2. Print a Triangle of Stars
n = 5
row = 1
while row <= n:
print("*" * row)
row += 1
Output
*
**
***
****
*****
3. Finding the HCF of two numbers
def compute_hcf(x, y):
# choose the smaller number
if x > y:
smaller = y
else:
smaller = x
for i in range(1, smaller+1):
if((x % i == 0) and (y % i == 0)):
hcf = i
return hcf
num1 = 6
num2 = 36
print("The H.C.F. is", compute_hcf(num1, num2))
Output
The H.C.F. is 6
Summary
In this tutorial, we looked at the while loop in Python programming. We covered the while loop with all the aspects necessary to understand it completely. The illustrations given above will provide you with a good level of clarity on the various parts of the main topic. If you want to know more about different concepts of Python Programming, consider enrolling in our Python Certification Course right now and get your hands on the best comprehensive guide to Python core concepts.
FAQs
Q1. What is while loop in Python?
Q2. What is the use of while loop?
Q3. How to loop while True in Python?
# Infinite loop while True: print("This loop will run indefinitely!") # Additional code here
Q4. What is the syntax of while loop?
while condition: # Code block to execute while condition is True