13
JulTypes of Loops in Python - For, While Loop
Loops in Python are used to repeat a block of code multiple times, helping you avoid writing the same lines again and again. They play a key role in controlling how a program runs, especially when you need to go through lists, run conditions, or perform repeated tasks. The two main types of loops in Python are the for loop, which works well with sequences like lists or strings, and the while loop, which runs as long as a condition remains true.
In the Python tutorial, learning loops in Python is important for beginners because they are used in everything from simple scripts to complex applications. They make your code more efficient, readable, and easier to manage.
What is a loop in Python?
A loop in Python is a control flow statement that allows you to execute a piece of code repeatedly until a specific condition is met. Loops in Python are necessary for operations that require repetitive execution, such as iterating through a Python list of items or doing calculations many times.
Read More - 50 Python Interview Questions and Answers
Different Types of Loops in Python
To understand the Loop Structures in Python, you need to know that there are 3 Types of Loops in Python:
- Python while loop
- Python for loop
- Python nested loop
1. Python while loop
A while loop in Python is used to repeat a block of code as long as a certain condition is true. It keeps running the loop body again and again until the condition becomes false. This is useful when you don't know in advance how many times the loop should run.
Syntax of the Python while loop
while expression:
statement(s)
Example of Python while loop in Python Compiler
count = 0
while (count < 10):
print ('The count is:', count)
count = count + 1
print ("Good bye!")
A while loop is a control flow statement that allows you to repeatedly run a block of code as long as a given condition is true. The condition in this case is count 10. This signifies that the loop will keep running as long as the variable count is less than 10.
Output
The count is: 0
The count is: 1
The count is: 2
The count is: 3
The count is: 4
The count is: 5
The count is: 6
The count is: 7
The count is: 8
The count is: 9
Good bye!
Read More - Python Developer Salary
2. Python for loop
A for loop in Python is used to go through a sequence of items, like a list, a string, or a range of numbers. It repeats the block of code once for each item in the sequence. This makes it very useful when you already know how many times you want to loop.
Syntax of Python for loop
for iterating_var in sequence:
statements(s)
Example of Python for loop
for letter in 'Python': # First Example
print ('Current Letter :', letter)
fruits = ['guava', 'apple', 'mango']
for fruit in fruits: # Second Example
print ('Current fruit :', fruit)
print ("Good bye!")
The code in the above Python Editor consists of up to two for loops that traverse over a Python string and a list in Python. The first loop loops through the string 'Python', printing the current letter to the terminal. The second loop iterates through the 'fruits' list, printing the current fruit to the terminal. Finally, the code outputs to the terminal the message "Goodbye!"
Output
Current Letter : P
Current Letter : y
Current Letter : t
Current Letter : h
Current Letter : o
Current Letter : n
Current fruit : guava
Current fruit : apple
Current fruit : mango
Good bye!
3. Python Nested loop
A nested loop in Python means using one loop inside another loop. It is commonly used when you need to repeat actions in a multi-level structure, like printing patterns or working with multi-dimensional lists (like 2D arrays).
Syntax for nested for loop
for iterating_var in sequence:
for iterating_var in sequence:
statements(s)
statements(s)
Syntax for a Nested while loop
while expression:
while expression:
statement(s)
statement(s)
Example of Python Nested Loop
i = 2
while(i < 100):
j = 2
while(j <= (i/j)):
if not(i%j): break
j = j + 1
if (j > i/j) : print (i, " is prime")
i = i + 1
print ("Good bye!")
Using a nested while loop, this code snippet determines prime numbers between 2 and 100. The outer loop iterates through numbers ranging from 2 to 100, while the inner loop determines if each number is divisible by any number ranging from 2 to its square root. If a number in the inner loop is not divisible by any other number, it is considered prime and written to the console. The outer loop is repeated until it reaches 100, at which point "Goodbye!" is printed.
Output
2 is prime
3 is prime
5 is prime
7 is prime
11 is prime
13 is prime
17 is prime
19 is prime
23 is prime
29 is prime
31 is prime
37 is prime
41 is prime
43 is prime
47 is prime
53 is prime
59 is prime
61 is prime
67 is prime
71 is prime
73 is prime
79 is prime
83 is prime
89 is prime
97 is prime
Good bye!
Loop Control Statements in Python
In Python, loop control statements are used to alter the regular flow of a loop. They enable you to skip iterations, end the loop early, or do nothing at all. Python's main three loop control statements are:- Break
- Continue
- Pass
1. Break
The break statement is used to quickly stop the loop regardless of whether the loop condition is still true. This signifies that the loop will be terminated and the next statement outside of the loop will be executed.Example of Break Statement in Python
for i in range(10):
if i == 5:
break
print(i)
Output
0
1
2
3
4
2. Continue
The continue statement is used to skip the current loop iteration and go to the next iteration. This implies that the code from the current iteration will not be performed, and the loop will continue to iterate until the loop condition is satisfied.Example of Continue Statement in Python Online Editor
for i in range(10):
if i % 2 == 0:
continue
print(i)
Output
1
3
5
7
9
3. Pass
The pass statement is a null statement that has no effect. It can be utilized as a placeholder in control statements or empty loops.Example of Pass Statement in Python
for i in range(10):
# Do nothing
pass
This code will not print anything because the pass statement does nothing. It is frequently used as a placeholder in control statements or empty loops.
Summary
In conclusion, Loops in Python are a powerful feature that helps you run code multiple times without repeating yourself. Whether you're using a for loop to go through a list or a while loop to repeat code until a condition is met, loops make your programs more efficient and easier to manage. Learning how to use loops is an important step for every Python programmer, as they are used in almost every real-world project.
FAQs
Take our Python skill challenge to evaluate yourself!
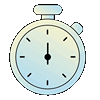
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.