14
JunProps in React
Props in React
Have you ever wondered how to pass data between components in React? Props in React are used to send information from one component to another. With Props in React, you can make components more dynamic and reusable, helping you build more powerful and interactive applications.
So in this React Tutorial, we are going to explore props in React including What are Props in React, its uses, syntax, and Best Practices for working with props. Let's see one-by-one.
What are Props?
- React's core concept of props makes it possible to pass data from one component in Reactto another.
- In essence, they are a collection of properties that receive values and are transmitted to child components.
- Similar to passing arguments to a function, think of props as a means to provide your component's parameters.
Read More - Top 50 Mostly Asked React Interview Questions & Answers
How to Declare and Use Props
When a component is rendered in React, an attribute's value is assigned to specify a prop. With the help of props, we are able to build reusable elements that can be rendered with new information each time they are utilized.
Here are some extra considerations regarding props:
- Despite the fact that there may only be one prop, it is always passed as an object.
- The child component cannot modify props.
- Any type of data, including texts, numbers, objects, and arrays, can be passed with props.
Here is an easy example showing how to define and make use of props in React:
Example
import React from 'react';
// Functional component that accepts props
const Greeting = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
// Parent component that renders the Greeting component
const App = () => {
return (
<div>
<Greeting name="John" />
<Greeting name="Jane" />
<Greeting name="Alice" />
</div>
);
};
export default App;
Explanation
- Greeting and App are the two elements in this example.
- The name prop is displayed as a heading by Greeting, and the App creates many Greeting components with various names.
- "Hello, John!" "Hello, Jane!" and "Hello, Alice!" are displayed when the app is rendered.
- Props transfer information and settings between parent and child components.
- In this case, the name prop in Greeting personalizes the greeting.
The Unidirectional Flow of Props
- Props follow a unidirectional flow in React.
- Which means that they are passed from parent components to child components.
- This flow guarantees predictable and controlled data propagation.
- The only thing that child components can do with the props they get is to ingest and display the information.
Props vs. State
- Props in React are used to pass data from one component to another, typically from a parent to a child component. They are read-only, meaning that the receiving component cannot change the props it receives.
- State in React, on the other hand, is used to store and manage data that can change over time within a component. A component can update its own state, and when the state changes, it triggers a re-render of the component.
Aspects | Props in React | State in React |
Purpose | Pass data from parent to child components | Store data that changes within a component |
Mutability | Immutable (cannot be changed by the receiving component) | Mutable (can be changed within the component) |
Who manages it | Managed by the parent component | Managed by the component itself |
Usage | Used to pass information down the component tree | Used for managing dynamic data that affects rendering |
Re-rendering | A change in props triggers a re-render of the child component | A change in state triggers a re-render of the component |
Passing props in reacting and accessing props in react
For creating reusable and dynamic components in React, passing and accessing props is an essential idea. A parent component passes properties, often known as props, to a child component in order to offer data or settings.
Following are the steps for passing props in React and accessing props in React:
- Declare the properties in the child component's props interface. The child component's expected props are defined via the props interface, an object
- Give the child component the prop. Use the props keyword to pass a prop to a child component.
- Utilize the child's component's prop. The props object can be used to access a prop in the child component.
Passing props in react & accessing props in react has the following benefits:
- Reusability: Props make it possible to build reusable parts, cutting down on code duplication.
- Dynamic Behaviour: To create interactive user interfaces, components can render various data and change functionality based on props.
- Communication: Props make it possible for components to communicate with one another, passing information and configuration between them.
- Data Flow Control: Props follow a unidirectional flow, which makes controlling data flow simpler to understand and implement.
- Encapsulation: Props promote the encapsulation of component logic & data, which enhances testability and maintainability.
As an example of passing props in React and accessing props in React, consider this:
Example
// ParentComponent.js
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
const name = 'John';
const age = 25;
return (
<div>
<h1>Parent Component</h1>
<ChildComponent name={name} age={age} />
</div>
);
};
export default ParentComponent;
// ChildComponent.js
import React from 'react';
const ChildComponent = (props) => {
return (
<div>
<h2>Child Component</h2>
<p>Name: {props.name}</p>
<p>Age: {props.age}</p>
</div>
);
};
export default ChildComponent;
Explanation
This example shows how the ParentComponent produces the ChildComponent while passing the two props, name and age. By using the props object, the ChildComponent can access these props and view their values. "John" is entered as the name and "25" as the age.
Pass data from one component to another
In React, there are three primary methods for passing data from one component to another:
- Props: Passing data from the parent component to a child component via props is the most popular method. Because props are read-only, the data that is sent to the child component cannot be changed.
- State: State is a method of storing data that the component can modify. Although a state can be sent from a parent component to a child component, it is more typical for the child component to use its own state.
- Context: Using context, several components can share data. Context is a more complex way to convey data, but it can be helpful when you need to communicate data among several unrelated components.
Default Props in react
In React, default props in React are a means to establish default values for properties that might not be given in from the parent component. This can be helpful to make sure that even if the parent component doesn't explicitly indicate it, your components always have a value for a specific prop. You can use the default props property on your component class to define default props. Maps the names of props to their default values using this property's object.
Here are some further concerns with default props in react:
- There is no need for default props.
- If there are no default properties that need to be defined, you don't have to.
- The parent component may override default properties.
- A prop's value passed in by the parent component will take precedence over its default value.
- At runtime, default props in react are examined. As a result, rather than the values that were defined when the component was defined, the default props' values will be those that were defined at the time the component was shown.
Here's an example
Example
import React from 'react';
const Greeting = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
// Default props
Greeting.defaultProps = {
name: 'Guest',
};
const App = () => {
return <Greeting />;
};
export default App;
Explanation
In the example, the Greeting component has a default value of 'Guest' for the name prop. When rendering the Greeting component without providing a name prop, it uses the default value. Thus, if you render the App component, it displays the greeting "Hello, Guest!".
Destructuring Props
React offers a simple mechanism to directly destructure props in the function signature in addition to accessing props through the props object. This makes it easier for us to access specific props and makes the code more readable. We can utilize the prop names without having to prefix them with props by destructuring the props.
Here is an example for destructuring props:
Example
import React from 'react';
const Greeting = ({ name, age }) => {
return (
<div>
<h1>Hello, {name}!</h1>
<p>You are {age} years old.</p>
</div>
);
};
const App = () => {
return <Greeting name="John" age={25} />;
};
export default App;
Explanation
The Greeting component in the example uses destructuring to obtain the name and age props directly. This eliminates referencing props.name and props.age a number of times. In order to personalize the greeting, the App component renders the name prop ("John") & age prop (25) parameters. When several props are supplied to a component, destructuring the props makes the code more compact and readable.
Best Practices for working with props:
There are a few best practices to keep in mind when using props in React:
- Keep props simple: Only pass the necessary data. Don't overload components with too many props.
- Use defaultProps: Set default values for props to ensure your component has fallback values when props are missing.
- Destructure props: Destructure props in your component for cleaner and more readable code.
- Validate props: Use PropTypes to validate the data types of props to avoid errors and improve code quality.
- Avoid mutating props: Never change the props inside the component. Treat them as read-only.
- Pass functions as props: If a child component needs to trigger changes in the parent, pass functions as props to handle the logic.
- Use prop drilling wisely: Avoid passing props through many layers of components. Use context if props need to be shared across many levels.
Resources for further learning and practice
- Official Redux documentation: Read the documentation at https://legacy.reactjs.org/docs/getting-started.html.The official Redux documentation is a comprehensive resource that covers all aspects of Redux, including React Redux.
- Online tutorials and courses: Explore Additional Learning and Practice Resources with us athttps://www.scholarhat.com/training/reactjs-certification-training. There are numerous online tutorials and courses available that specifically focus on React Redux.
Conclusion
In conclusion, props in React allow you to pass data between components, making your app more dynamic. By using props in React correctly, you can ensure smooth communication between components. Always remember to keep props in React simple and avoid changing them inside the component.
Start your learning journey with our free technology courses and build practical skills to create amazing web apps. Take it further with our React certification training courses, and get ready for exciting career opportunities!
Further Read Articles |
React Form Validations |
Routing in React using React-Router |
Hooks in React |
Practice with the following MCQs
Q 1: What are props in React?
Explanation: In React, props (short for properties) are used to pass data from parent components to child components, making the child components dynamic and reusable.
Q 2: Can props be modified inside a component?
Explanation: Props are read-only and cannot be modified by the component that receives them. They are meant to be passed down from parent to child components.
Q 3: How do you pass props to a component in React?
Explanation: In React, props are passed to a component by including them as attributes when the component is used, like <Component name="John" />
.
Q 4: Can props be used to pass functions in React?
Explanation: Functions can be passed as props in React. This allows a child component to call a function defined in the parent component, enabling interaction between them.
Q 5: What is the syntax for passing props to a functional component in React?
function Component(props) {}
Explanation: In a functional component, props are passed as an argument to the function. The syntax function Component(props) {}
is used to access props inside the component.
FAQs
Take our React skill challenge to evaluate yourself!
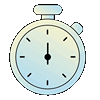
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.