14
JunImmutable.js in React: A Comprehensive Guide
Introduction
You are always looking for methods to make your applications more reliable and performant as a React developer. immutable.js is one tool that can assist you in achieving these objectives. Lists, maps, and sets are among the immutable data structures offered by the immutable.js package for JavaScript. In this post, we'll examine the advantages of utilizing immutable js while developing React applications and go over the fundamental ideas and recommended practices for doing so. If you're eager to dive deeper into the integration of immutable.js with React and understand how it can enhance your application's performance and reliability, consider enrolling in a specialized React JS Training program.
Why use immutable js in React?
Let's first explore why utilizing immutable js in React can be advantageous before getting into the specifics. By re-rendering only the relevant components, React is intended to update the user interface efficiently. However, tracking changes and figuring out which parts require updating can be difficult when dealing with mutable data. Here, immutable js is useful.
It offers immutable data structures that you may use to guarantee that your data is preserved in its original form after creation. This makes updating your React components faster and more effective because it eliminates the requirement to compare data to see if it has changed. Furthermore, immutable js offers built-in methods for performing frequent operations on immutable data, improving the readability and maintainability of your code.
Read More - Top 50 Mostly Asked React Interview Question & Answers
Benefits of immutable js in React Development
This is useful for React development for many reasons:
- Immutability guarantees that your data cannot be unintentionally changed, which reduces defects and makes debugging simpler.
- Immutable data makes it easier to follow changes and allows for effective updates, which speeds up rendering and improves performance.
- This encourages functional programming, which makes your code more foreseeable and straightforward to understand.
- You can avoid negative impacts and make it simpler to comprehend how data moves through your program by treating data as immutable.
- Lists, maps, and sets are just a few of the strong and effective collection types offered by immutable js.
- These collection types provide a variety of techniques for data manipulation and querying, simplifying complicated processes.
- When dealing with data manipulation, immutable js lowers the requirement for implementing customized code.
Basic concepts of immutable js
A popular JavaScript library for React development is called immutable js. The fundamental ideas behind using immutable js in React are as follows:
- Immutability: In React components, JavaScript emphasizes the concept of immutable data, where data cannot be modified directly. Modifications, on the other hand, produce new instances of data.
- Performance Improvement: This enables efficient updates and lowers memory usage, improving the performance of React apps. It does immutable js by utilizing permanent data structures using structural sharing.
- Functional Programming: This encourages a functional programming style in React development, making components more predictable and easier to reason about.
- Immutable Data Manipulation: This offers practical ways to query, update, and transform data, streamlining complicated React application tasks.
Immutable collections in React
This offers a set of immutable collection types that may be used in React for more effective and dependable data storage and manipulation. Here is an example of how React uses immutable collections:
import { List, Map } from 'immutable';
class MyComponent extends React.Component {
state = {
data: List(['apple', 'banana', 'orange']),
userInfo: Map({ name: 'John', age: 25, address: '123 Main St' })
};
handleAddItem = () => {
const newData = this.state.data.push('grape');
this.setState({ data: newData });
};
render() {
const { data, userInfo } = this.state;
return (
<div>
<ul>
{data.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
<div>
<p>Name: {userInfo.get('name')}</p>
<p>Age: {userInfo.get('age')}</p>
<p>Address: {userInfo.get('address')}</p>
</div>
<button onClick={this.handleAddItem}>Add Item</button>
</div>
);
}
}
A list of fruits is stored in this example using immutable js, and user data is stored in the Map. Push is used by the handleAddItem function to add an item to the list immutably. The data list is mapped during rendering, and get is used to acquire user information. immutable js guarantees accurate updates and data integrity for React components.
Read More - React developer salary in India
Lists using immutable.js in React
Lists are arranged collections that allow for the presence of redundant values. They make a great option for immutably representing arrays. List manipulation options provided by immutable js include push, pop, shift, & unshift.
React lists come in extremely handy when working with dynamic or huge data sets. Immutable lists are the best choice in situations requiring frequent changes or searches since they efficiently update and look up data.
immutable js lists are utilized in a React component in the following manner:
import React from 'react';
import { List } from 'immutable';
class MyComponent extends React.Component {
state = {
todos: List([
{ id: 1, task: 'Buy groceries' },
{ id: 2, task: 'Walk the dog' },
{ id: 3, task: 'Do laundry' }
])
};
handleAddTodo = () => {
const newTask = prompt('Enter a new task:');
if (newTask) {
const newTodo = { id: Date.now(), task: newTask };
this.setState(prevState => ({
todos: prevState.todos.push(newTodo)
}));
}
};
handleRemoveTodo = (id) => {
this.setState(prevState => ({
todos: prevState.todos.filter(todo => todo.id !== id)
}));
};
render() {
const { todos } = this.state;
return (
<div>
<ul>
{todos.map(todo => (
<li key={todo.id}>
{todo.task}
<button onClick={() => this.handleRemoveTodo(todo.id)}>Remove</button>
</li>
))}
</ul>
<button onClick={this.handleAddTodo}>Add Todo</button>
</div>
);
}
}
export default MyComponent;
In this example, an array of todo objects with an id & a task are stored in an immutable js List called todos. The handleAddTodo function asks the user to enter a task before adding it immutably to the list of to-do items and creating a new to-do object with a special id. Using the filter approach, the handleRemoveTodo function removes a todo depending on its id. Each task is rendered as a list item via the render method, which maps over the list of tasks. State stays immutable when immutable js Lists are used, ensuring quick updates and accurate data in React components.
Maps using immutable.js in React
Key-value pairs are stored in collections called maps. They are comparable to JavaScript objects but come with more capabilities and improved speed. Key-value pairs can be handled using methods like get, set, delete, and has provided by maps.
When working with complicated data structures or when you frequently need to look up or change information, using maps in React can be helpful. Maps are excellent for situations where effective data retrieval is important because they offer efficient access to values based on their keys.
Here's an example of how immutable js Maps are utilized in a React component:
import React from 'react';
import { Map } from 'immutable';
class MyComponent extends React.Component {
state = {
user: Map({
name: 'John Doe',
age: 25,
address: '123 Main St'
})
};
handleUpdateAge = () => {
this.setState(prevState => ({
user: prevState.user.set('age', prevState.user.get('age') + 1)
}));
};
render() {
const { user } = this.state;
return (
<div>
<p>Name: {user.get('name')}</p>
<p>Age: {user.get('age')}</p>
<p>Address: {user.get('address')}</p>
<button onClick={this.handleUpdateAge}>Increase Age</button>
</div>
);
}
}
export default MyComponent;
This example maintains user data such as name, age, and address in an immutable js Map called "user." The handleUpdateAge function uses the get and set methods to increase the user's age by 1. User data is obtained and shown via the render method. The age value is permanently updated when "Increase Age" is clicked. These Maps guarantee data integrity, quick updates, and immutability of state in React components. If you're interested in mastering the integration of immutable.js with React and learning how it can enhance data management in your applications, consider enrolling in a specialized React JS course for practical insights and hands-on experience.
Sets using immutable.js in React
Unordered collections called sets prevent the duplication of values. They provide techniques for adding, removing, and confirming the existence of values. When working with unique data or when you need to carry out tasks like deduplication or filtering, sets might be helpful.
When working with data that needs to be unique in React or when you want to carry out time-saving tasks like deleting duplicates or testing for a certain value, sets might be useful. As an example, consider the following React component utilizing immutable.js Set:
import React from 'react';
import { Set } from 'immutable';
class MyComponent extends React.Component {
state = {
selectedColors: Set(['red', 'green', 'blue'])
};
handleSelectColor = (color) => {
this.setState(prevState => {
let updatedColors;
if (prevState.selectedColors.has(color)) {
updatedColors = prevState.selectedColors.delete(color);
} else {
updatedColors = prevState.selectedColors.add(color);
}
return {
selectedColors: updatedColors
};
});
};
render() {
const { selectedColors } = this.state;
return (
<div>
<h3>Select Colors:</h3>
<div>
<label>
<input
type="checkbox"
checked={selectedColors.has('red')}
onChange={() => this.handleSelectColor('red')}
/>
Red
</label>
</div>
<div>
<label>
<input
type="checkbox"
checked={selectedColors.has('green')}
onChange={() => this.handleSelectColor('green')}
/>
Green
</label>
</div>
<div>
<label>
<input
type="checkbox"
checked={selectedColors.has('blue')}
onChange={() => this.handleSelectColor('blue')}
/>
Blue
</label>
</div>
<h4>Selected Colors:</h4>
{selectedColors.map(color => (
<div key={color}>{color}</div>
))}
</div>
);
}
}
export default MyComponent;
This example saves a collection of colors in an immutable.js Set called selectedColors. Based on the checkbox selection, the handleSelectColor function adds or subtracts colors from the set. Colors are shown in checkboxes dependent on their inclusion in the set. Individual div elements are rendered for specific colors. These Sets guarantee data integrity, quick updates, and immutability of state in React components.
Use cases for immutable.js in React
Several use cases for immutable js in React development include:
- Immutable state management: A React application's state can be managed with JavaScript. You can guarantee that the state stays unchanged by employing immutable data structures, which allow for quick updates and avoids accidental alterations.
- Immutable performance optimization: Persistent data structures are offered by js, enabling quick updates and comparisons. Cutting down on pointless re-renders and streamlining the rendering process, can result in better performance.
- Undo/redo capabilities: This makes it simpler to include undo/redo capabilities in React applications. You can quickly go back to earlier states and undo changes by keeping track of earlier states using immutable data structures.
- Memory and caching are immutable: Js data structures are appropriate for caching and memoization in React components. You can cache expensive computations and prevent needless re-computation when the component re-renders by leveraging immutable data.
- Immutable context and objects: This can be used to enforce immutability for props and context values passed to React components. Through the component hierarchy, this maintains data integrity and helps prevent unintentional updates.
Performance Considerations when Using immutable.js
It's important to be mindful of some performance considerations even if immutable js can significantly improve performance and reliability. These data structures are designed to perform well under read-intensive workloads, making it easy to access and query data. However, when it comes to frequent upgrades and alterations, they can take longer. Therefore, when choosing to use immutable js, it is imperative to take into account the particular needs of your application.
immutable js's memory use is another factor to take into account. By sharing data between instances, the permanent data structure approach reduces memory utilization, but it can still use more memory than changeable data structures. Keep memory use in mind while you optimize your code.
Tools and resources for working with immutable.js
Here are some helpful tools and resources for using immutable js in React:
- The official Immutable is the documentation: You can better understand and use the library by using detailed explanations, API references, and examples in the JavaScript documentation.
- immutable js repository on GitHub: Access to the source code, bug tracking, and community conversations are all available through the GitHub repository. It's an excellent tool for staying informed, submitting bug reports, and getting community support.
- React-immutable js: This library makes it easier to combine immutable js with React components by offering useful utility functions and React-specific components.
- immutable js Add-ons: A variety of add-ons for immutable js are available to enhance its functionality and include features like sorting, filtering, and handling asynchronous activities.
- Online Forums and Communities: Engaging with online communities such as Stack Overflow or the immutable js subreddit allows you to seek advice, share experiences, and learn from other developers working with immutable js in React.
- Development Tools and Extensions: Code editors and integrated development environments (IDEs) like Visual Studio Code offer plugins or extensions that give helpful capabilities for working with immutable js, such as syntax highlighting, autocompletion, and linting.
Best practices for using immutable.js in React
It's essential to keep to the following best practices while using immutable js in React:
- Accept Immutability: Treat data as immutable & refrain from making direct changes. When upgrading data, make new copies using immutable js techniques.
- Choose immutable js Structures Instead: Use the List, Map, and Set data structures from immutable js to manage the state. Both efficient operations and immutability are maintained.
- Update state without changing it: Instead of changing the state of an existing object, create new instances of immutable js data structures with the updated values.
- Use immutable js Methods: Become familiar with the set, get, update, & merge methods for secure data manipulation.
- Performance considerations: Use techniques like withMutations to batch operations for improved performance, and try to avoid creating too many objects.
- Integrate React Optimisations: To compare immutable js structures and optimize rendering, use shouldComponentUpdate, React.memo, or other techniques.
- immutable js with State Management: To guarantee consistency and avoid unintentional modifications, use immutable js for state kept in Redux or Context.
- Considerations for Testing: When testing immutable js structures, use immutable js methods rather than making direct comparisons to JavaScript arrays or objects.
Resources for further learning and practice
- Official Redux documentation: Read the documentation at https://legacy.reactjs.org/docs/getting-started.html The official Redux documentation is a comprehensive resource that covers all aspects of Redux, including React Redux.
- Online tutorials and courses: Explore Additional Learning and Practice Resources with us at https://www.scholarhat.com/training/reactjs-certification-training. There are numerous online tutorials and courses available that specifically focus on React Redux.
Conclusion
Immutable.js is a strong package that, in summary, may significantly improve the speed and dependability of your React apps. Use immutable js data structures to adopt immutability, which will help you write cleaner code with better speed and fewer problems. Unlocking the full potential of immutable.js in React requires an understanding of both its fundamental ideas and recommended usage patterns.
Take our React skill challenge to evaluate yourself!
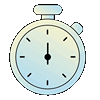
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.