02
MayRedux Middleware
Introduction to Redux middleware
A powerful state management library for JavaScript apps is called Redux. It makes it simpler to create complicated applications since it offers a predictable mechanism to handle the application state. Redux's middleware design, which enables developers to expand and improve Redux's functionality, is one of its core characteristics. To master Redux and its advanced capabilities, consider enrolling in the Best React JS Course available for comprehensive training and practical knowledge.
Between the time an action is dispatched and the time it reaches the reducer, middleware is present. Actions can be modified, or intercepted, or new actions can be sent out. This enables developers to incorporate extra functionality into the Redux flow, such as logging or handling asynchronous operations.
Why we need middleware in redux
The following shows the necessity of middleware in Redux:
- Extensibility: Middleware injects additional logic between the dispatch of actions and the execution of reducers, providing features like logging, handling side effects, and asynchronous processes.
- Asynchronous Operations: Middleware that handles asynchronous operations while maintaining the Redux flow, such as Redux Thunk or Redux Saga, enables actions to be dispatched asynchronously, such as retrieving data from an API.
- Side Effect Management: By offering a regulated and organized method, middleware aids in managing side effects brought on by operations, such as making API queries or communicating with external libraries.
- Action Transformation: Before actions are sent on to reducers, middleware intervenes and transforms them. This enables data transformation, aggregates several actions, or applies conditional logic based on predetermined criteria.
Read More - Top 50 Mostly Asked React Interview Question & Answers
Understanding the basics of thunk and react thunk
With the help of the middleware called Thunk, you may create action creators that return functions rather than action objects. Before dispatching the actual action, this function can be used to carry out asynchronous actions like performing API calls. Applications using Redux frequently employ thunk middleware because it makes handling asynchronous actions easier.
React Thunk is a particular type of Thunk middleware designed specifically for React applications. It allows you to design asynchronous action creators in a more easy and declarative manner and effortlessly integrates with Redux. You can concentrate on creating the action & let React Thunk handle the asynchronous actions rather than having to manage them manually.
Exploring the benefits of using thunk middleware in Redux
The use of thunk middleware in Redux has many benefits:
- Redux's thunk middleware makes managing asynchronous actions simpler.
- Thunk enables programmers to write action producers that handle asynchronous tasks like API requests.
- Thunk improves the readability and maintainability of code.
- Thunk makes it possible to defer the execution of an action until a certain condition is satisfied.
- It is possible to delay dispatching an action until, say, a specific API request completes successfully.
- Thunk offers flexibility in managing the dispatch of actions.
- Redux DevTools is compatible with thunk middleware.
- A browser extension called Redux DevTools is used to inspect and troubleshoot Redux state changes.
- Thunk smoothly integrates with Redux DevTools to make asynchronous action debugging easy.
Introduction to redux-saga and its role in managing side effects
Redux has several asynchronous action handling choices than the well-liked thunk middleware. Redux Saga is one of them. A middleware called Redux Saga focuses on controlling side effects in Redux applications.
Side effects are operations that have an effect outside of the Redux store, such as using an API, gaining access to local storage within the browser, or collaborating with external libraries. The coordination of numerous activities and dealing with asynchronous programs make managing side effects difficult at times.
Redux Saga responds to these difficulties by presenting the idea of sagas. A saga is a type of generator function that responds to certain actions and produces side effects as a result. It gives complex asynchronous flows a declarative handling method, making it simpler to think through and verify side effects in your application.
Read More - React developer salary in India
Installing and setting up redux-saga in your React application
- You must first install Redux Saga before you can use it in your React application. Run the following command in your project directory to accomplish this.
npm install redux-saga
- Redux Saga may be configured in your application after installation by building a saga middleware & adding it to your Redux store. Here's an example of how to accomplish it:
import { createStore, applyMiddleware } from 'redux';
import createSagaMiddleware from 'redux-saga';
import rootReducer from './reducers';
import rootSaga from './sagas';
const sagaMiddleware = createSagaMiddleware();
const store = createStore(rootReducer, applyMiddleware(sagaMiddleware));
sagaMiddleware.run(rootSaga);
export default store;
In this example, we use the createSagaMiddleware function to create a saga middleware. The applyMiddleware function from Redux is then used to apply this middleware to our Redux store. Finally, we use the run method of the saga middleware to execute our root saga.
Working with saga helpers for handling asynchronous actions
Several utility functions are offered by Redux Saga to make handling asynchronous actions simpler. TakeEvery is one of the most utilized utility functions. TakeEvery enables you to keep an eye out for particular actions and launch a story each time one is completed.
Here's an example of how to handle asynchronous activities with takeEvery:import { takeEvery, put } from 'redux-saga/effects';
import { fetchDataSuccess, fetchDataError } from './actions';
import { FETCH_DATA_REQUEST } from './actionTypes';
import { fetchData } from './api';
function* fetchDataSaga() {
try {
const data = yield call(fetchData);
yield put(fetchDataSuccess(data));
} catch (error) {
yield put(fetchDataError(error));
}
}
function* rootSaga() {
yield takeEvery(FETCH_DATA_REQUEST, fetchDataSaga);
}
export default rootSaga;
In this example, the fetchData function from our API module is used to define a fetchDataSaga that calls an API. The obtained data is sent along with a fetchDataSuccess action if the API request is successful. We dispatch a fetchDataError action along with the error object if an error occurs. To dive deeper into practical examples like this and learn how to effectively integrate Redux with React, consider enrolling in a specialized React Course for hands-on experience and in-depth knowledge.
Comparing sagas vs promises in Redux middleware
- In Redux middleware, promises are yet another method for dealing with asynchronous operations. While promises give a simple method for handling asynchronous code, they don't have some of the declarative & testability advantages that sagas have.
- How complex asynchronous processes are handled by sagas & promises is one of their primary differences. When using promises, you frequently end up with nested then-calls or lengthy chains of promises, which can rapidly get confusing and difficult to understand.
- Sagas, on the other hand, give you more declarative control over the definition of complicated asynchronous flows. You may choreograph numerous actions & side effects in a readable & testable way by using generators & the different assistance functions offered by Redux Saga.
Available choices for Redux middleware and why thunk react stands out
- Thunk, Redux-Saga, and Redux Observable are popular choices when selecting Redux middleware for asynchronous activities:
- Thunk is a popular option for novices and developers looking for a simple approach because of its reputation for simplicity and ease of use.
- Thunk's popularity among users is increased by its smooth integration with Redux and React.
- Advanced functionalities are available with Redux Saga and Redux Observable, although they have a longer learning curve.
- Thunk middleware is a great option if you're new to Redux or want something simpler.
- Redux middleware's potential is efficiently unlocked through thunk middleware.
- When choosing a middleware solution, take into account the complexity of your project and your level of knowledge with Redux.
- Thunk offers a strong base for managing asynchronous actions and can be enhanced as necessary.
How to unlock the potential of Redux middleware with thunk react
- To get the most out of Redux middleware with a thunk in React, organize your code in a modular and reusable way.
- The readability, maintainability, and testing of asynchronous activities are all enhanced by modular programming.
- To access information from the Redux store, use selectors. Selectors improve state management by encapsulating data access and transformation mechanisms.
- Selectors make updating and maintaining the state of your application easier.
- Utilize the Redux & thunk middleware debugging tools.
- Powerful capabilities are available in Redux DevTools for inspecting and troubleshooting state changes in Redux.
- Tools for debugging offer insightful information on how asynchronous actions affect the state of your application.
- Improve your knowledge of your code's operation and improve the effectiveness of problem-solving.
Best Practices of Redux Middleware
- Keep middleware focused and modular: Maintain middleware's focus and modularity by breaking it down into smaller, more focused functions to address particular issues. This enhances reusability and maintainability.
- Properly order middleware: Middleware should be properly arranged in the intended execution sequence. This makes sure that each middleware function is given the right input and can carry out its intended role.
- Handle errors gracefully: Error handling middleware should be used to catch and handle errors in a centralized and consistent manner. This aids in preventing the disruption of the application flow due to unhandled errors.
- Use asynchronous middleware: To handle asynchronous operations, use middleware such as Redux Thunk, Redux-Saga, or Redux Observable. These modules give Redux users strong tools for controlling intricate asynchronous flows.
- Use selectors for efficient data retrieval: Implement selectors to encapsulate code for retrieving and altering data from the Redux store to retrieve data efficiently. Performance is enhanced, and state management is made easier.
- Test your middleware: To ensure that your middleware is reliable and correct, test it using testing frameworks and procedures. To confirm that each middleware function behaves as expected, write tests for it.
- Ensure middleware is current: Maintain the most recent versions of the middleware libraries you use, as well as Redux. Update your middleware frequently to benefit from bug fixes, performance enhancements, and new features.
- Utilise debugging tools: To examine and troubleshoot state changes and activities, employ tools like Redux DevTools. This aids in comprehending how the state of the application is impacted by your middleware operations.
- Keep track of your middleware: Make sure to fully describe the function, inputs, outputs, & any configurable options of your middleware. This documentation supports future comprehension, upkeep, and developer collaboration.
Resources for further learning and practice
- Official Redux documentation: Read the documentation at https://legacy.reactjs.org/docs/getting-started.html The official Redux documentation is a comprehensive resource that covers all aspects of Redux, including React Redux.
- Online tutorials and courses: Explore Additional Learning and Practice Resources with us at https://www.scholarhat.com/training/reactjs-certification-training. There are numerous online tutorials and courses available that specifically focus on React Redux.
Summary
Redux's Thunk React is a potent middleware for dealing with asynchronous operations. Thunk makes side effect management simpler by enabling you to create action creators that return functions, which improves the readability and maintainability of your code. Redux Saga and Redux Observable are two other options, although Sagas vs Promises and Thunk stand out for their usability. As a result of its easy Redux and React integration, developers frequently choose it.
Follow standard practices, such as structuring your code in a modular style and utilizing selectors to fetch data from the Redux store, to maximize the potential of Redux middleware using thunk react. Use the numerous debugging tools that are available as well to learn important details about how the state of your application changes.
Take our React skill challenge to evaluate yourself!
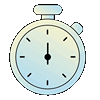
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.