14
JunWhat is Render in React? Beginner’s Tutorial with Examples
Render in React: An Overview
A key concept that powers dynamic user interfaces in web applications is rendering elements in React. As the underlying data changes, React enables developers to quickly refresh the user interface (UI). React speeds up and streamlines the rendering process by employing a virtual DOM. Developers can write JavaScript code that resembles HTML using the syntax extension JSX, which makes it easier to create React elements. To keep the UI in sync with the application's state, these elements are rendered into the actual DOM using the ReactDOM.render() method. For an in-depth understanding, consider taking a React course. Render in react technique quickly updates the virtual DOM, enabling responsive, optimized user interfaces that take data and state changes into account. Developers may easily create dynamic and responsive user interfaces because of React's rendering features.
Read More - Top 50 React Interview Question & Answers
What are React elements?
The most basic components of React applications are React elements. They stand in for the user interface that you want to see on the screen. Simple JavaScript objects known as "React elements" explain what you want to view, such as HTML tags, components, and other React elements.
DOM elements and React elements are not the same. React elements are separate from the DOM and may be efficiently constructed and managed by React, whereas DOM elements are a component of the HTML structure.
Render in React
The process of converting JSX (JavaScript XML) into actual HTML elements that are displayed in the browser is referred to as rendering in the React framework. React's virtual DOM effectively refreshes and re-renders only the required components, creating user interfaces that are optimized and responsive.
React elements vs. React components
The difference between React components and elements are as follows:
- The basic building blocks of a React component are known as React elements. A reusable UI element that can be made up of one or more React elements is known as a React component.
- While elements are the actual UI that is displayed on the screen, components contain the logic and behavior of a certain UI element.
The Basics of Render in React
How does React rendering work?
- React elements are converted into genuine HTML elements through the process of "rendering," which allows them to be shown on the screen.
- To effectively manage and update the UI, React makes use of a virtual DOM (Document Object Model).
- In comparison to the real DOM, the virtual DOM is a thin copy.
- When a React element is rendered, React creates a virtual representation of the user interface and compares it with the current state of the real DOM.
- The smallest set of adjustments required by React to update the DOM is then determined, and they are effectively applied.
Here is a simple example of how an element render in react:
import React from 'react';
import ReactDOM from 'react-dom';
const element = <h1>Hello, World!</h1>;
ReactDOM.render(element, document.getElementById('root'));
Here, we import the libraries for React and ReactDOM. Hello, World! is a React element that we create and store. We render the element to the 'root' HTML element using ReactDOM.render(). This results in the heading "Hello, World!" to appear on the web page.
Read More - React developer salary in India
Using the ReactDOM.render() function
React offers the ReactDOM.render() function to render in React elements into the DOM. The target DOM element and the React element that needs to be rendered are the two inputs this function accepts.
Understanding the root DOM node
All of the React elements are typically rendered in a single root DOM node in a React application. Your React application's entry point is this root DOM node. Usually, an <div> element with the id "root" serves as the root DOM node. React can quickly locate and handle the rendered elements in the DOM due to this convention.
The root DOM node can be specified in your HTML file as follows:
- <div id="root">/div>
By defining this root DOM node, you give React a specific area to render and update the user interface.
Rendering Simple Elements
- Creating a basic React element: You can utilize JSX, a JavaScript syntax extension that enables you to put HTML-like code inside your JavaScript code, for creating a React element.
- Rendering the element into the DOM: The ReactDOM.render() function can be used to render a React element into the DOM once it has been constructed.
Update react native
The immutability of React elements
Elements in React are regarded as being immutable, which implies that once they have been generated, they cannot be changed. The predictability and stability of the UI are guaranteed by this immutability. You must construct a new element and feed it to ReactDOM.render() in order to update the user interface. Then React will make the required adjustments to update the DOM by comparing the new element with the one that already exists.
Creating a new element for updates
React requires updating the component's state or props, causing a re-render, and rendering the changed element into the DOM using ReactDOM.render() to create a new element for changes.
Here is an easy example showing how to add a new element for update react native:
import React from 'react';
import ReactDOM from 'react-dom';
// Define a functional component
const Timer = () => {
const [count, setCount] = React.useState(0);
React.useEffect(() => {
const timer = setInterval(() => {
setCount(prevCount => prevCount + 1);
}, 1000);
return () => {
clearInterval(timer);
};
}, []);
return <h1>{count}</h1>;
};
// Render the Timer component into the root element of the HTML
ReactDOM.render(<Timer />, document.getElementById('root'));
This example imports React and ReactDOM, defines a Timer component that updates a count variable using the useState and useEffect hooks once every second, renders the count value in an h1 element, and makes use of ReactDOM.Real-time updates of the count in the DOM are produced by using the render() method to display the Timer component in the designated root element.
Re-rendering and updating the UI
React will quickly update the DOM to reflect the changes by comparing the updated element with the original one when the modified element is re-rendered using ReactDOM.render(). You can make interactive and dynamic UIs with React by updating elements & re-rendering them. Performance is optimized because only the relevant UI elements are updated thanks to React's effective rendering technology.
Here is an easy example showing how to update react native:
import React from 'react';
import ReactDOM from 'react-dom';
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
handleClick() {
this.setState(prevState => ({
count: prevState.count + 1
}));
}
render() {
return (
<div>
<h1>Count: {this.state.count}</h1>
<button onClick={() => this.handleClick()}>Increment</button>
</div>
);
}
}
ReactDOM.render(<App />, document.getElementById('root'));
In this example, a React component named App that monitors a count state is used. By using the handleClick method and utilizing setState(), the count value, which is initially set to 0, is updated when the button is clicked. This causes the component to be re-rendered, updating the count to be shown on the screen. For an in-depth learning, consider taking a React JS Training.
The Efficiency of React Rendering
React is renowned for its effective rendering abilities since it makes use of the virtual DOM & an effective diffing algorithm.
The power of the virtual DOM
- React makes use of a virtual DOM, which is a simplified version of the real DOM. React determines the minimal set of modifications required when an element is modified or re-rendered by comparing the virtual DOM with the current state of the actual DOM.
- React can effectively update only the DOM elements that have changed thanks to this diffing approach, which has a minimal performance impact.
- The rendering process is optimized by React's virtual DOM, which serves as a barrier between the user interface and the real DOM.
Benefits of React rendering
For developers, React's effective rendering system has various advantages:
- Better performance: React's virtual DOM & diffing method reduces the number of DOM updates, making user interfaces (UIs) quicker and more responsive.
- Improved developer productivity: React's declarative vocabulary and quick rendering process let programmers construct UI components without worrying about DOM manipulation at a low level.
- Code reuse: React's component-based architecture encourages code reuse and enables programmers to build modular, reusable user interface components.
- Scalability: React is suited for developing large-scale apps that need frequent UI updates due to its effective rendering techniques.
Here is a simple example of how effectively React renders:
import React, { useState } from 'react';
const ParentComponent = () => {
const [items, setItems] = useState(['Item 1', 'Item 2', 'Item 3']);
const handleClick = () => {
// Simulating an update to the list of items
const updatedItems = [...items, 'New Item'];
setItems(updatedItems);
};
return (
<div>
<button onClick={handleClick}>Add Item</button>
<ul>
{items.map((item, index) => (
<ChildComponent key={index} item={item} />
))}
</ul>
</div>
);
};
const ChildComponent = React.memo(({ item }) => {
console.log(`Rendering ${item}`);
return <li>{item}</li>;
});
export default ParentComponent;
The ParentComponent in the example uses map() to render a list of elements. Each item is sent to ChildComponent as a prop, and React.memo() is used to wrap each component for memorization. The list is updated when the "Add Item" button is clicked, adding a new item. The list is only updated and reordered when necessary thanks to React.memo() and unique key props. Rendering is made more efficient by leveraging key props and React.memo(). By eliminating wasteful re-rendering of the full list, only the new item is displayed.
Advanced Rendering Techniques
React offers a variety of modern rendering techniques that can improve your UI's adaptability and interactivity.
- Conditional rendering: You can render elements or components based on particular circumstances or states using conditional rendering. You can dynamically control what is rendered based on user interactions, the availability of data, or any other criteria by using conditional statements or logical operators.
- Arrays of elements to be rendered and mapped: With React, you can render arrays of elements by iterating through the array and rendering each element dynamically using the map() function.
- Rendering elements with props: Data can be passed from a parent component to its child components via props. As a result, you may make customizable and reusable React components.
Best Practices of Rendering Elements in React:
The following are some best practices for rendering elements in React:
- Make use of JSX: JSX enables you to create JavaScript code that is similar to HTML, making it simpler to read and manage.
- Encapsulate Logic in Components: Break your user interface down into smaller, reusable components, and then encapsulate logic within each one. This enhances the comprehension and modularity of the code.
- Prefer Functional Components: Use functional components rather than class components unless you require lifecycle methods or state. They are less complicated and simpler to comprehend.
- Avoid Direct DOM Manipulation: React should handle component rendering and updates using the virtual DOM to avoid direct DOM manipulation. Avoid using jQuery or other direct DOM modifications unless necessary.
- Give Lists Unique Keys: Give each element of the list a different "key" argument when drawing lists with a map(). In response to changes, this optimizes list updating and reordering.
- Use React Fragments or Short Syntax: When rendering many objects without a parent container. By doing this, extra wrapper elements in the DOM are avoided.
- Use Conditional Rendering: To conditionally rendered components based on specified conditions, use conditional statements (if-else or ternary operators). This enables material to be displayed dynamically depending on the status of the application.
- Optimise Re-renders: Use PureComponent or shouldComponentUpdate() to compare props and state to determine whether an update is required, and avoid needless re-renders.
- Use React's Memoization Techniques: Memoize components, values, as well as functions using React.useCallback(), memo(), and useMemo(), accordingly. When dealing with the same inputs again, memorization reduces superfluous computations and re-rendering.
- Profile and Optimize Performance: Make regular use of internal or external performance tools to profile and improve the performance of your React application. To enhance rendering performance, locate performance bottlenecks and implement the required optimizations.
Resources for further learning and practice
- Official Redux documentation: Read the documentation at https://legacy.reactjs.org/docs/getting-started.html The official Redux documentation is a comprehensive resource that covers all aspects of Redux, including React Redux.
- Online tutorials and courses: Explore Additional Learning and Practice Resources with us at https://www.scholarhat.com/training/react-certification-training. There are numerous online tutorials and courses available that specifically focus on React Redux.
Summary
We have covered all the essentials of React's rendering parts in this extensive overview. We began by defining React elements, render in React, and how they differ from components. The fundamentals of updating rendered elements and the effectiveness of render in react were then covered in depth. We talked about advanced rendering methods that React render, rendering best practices, & integrating React into current applications.
Take our React skill challenge to evaluate yourself!
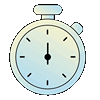
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.