18
JulUnderstanding useState in React
React useState Hook
React is counted among the most commonly used libraries in the domain of front-end development. It is mainly used for creating user interfaces. React has a component-based structure that makes the code easy to reuse and maintain. At the core of React’s components lies the idea of state, representing the data that can change with time and it affects how components are rendered.
In the past, when developers wanted to keep track of state in their React applications, they had to create class components and use the this.state method. But now with the latest version, we have React Hooks which provide a more sophisticated yet concise means of managing the state within functional components. Out of these hooks, useState is seen as the most important one. In this React Tutorial, we will try to explore React useState and React useState syntax with examples. To learn more about various aspects of React with ReactJS Certification Training.
Read More: React Hooks Interview Questions & Answers |
What is useState in React?
useState is one of the Hooks in React which is used to manage the state within the functional components. It is a way through which we can declare state variables and update them within functional components, without the need for class-based syntax.
Import useState in React
import React, { useState } from 'react';
Syntax of React useState
The basic syntax of useStae looks like this:const [state, setState] = useState(initialState);
- state- It is the current state value.
- setState- It is the function used for updating the state.
- useState- It takes the initial value of the state variable as an argument.
Example of React useState:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export default Counter;
Update State using useState Hook
Update useState
setCount(count + 1);
Read More: React Roadmap: Roadmap for Beginners to Learn React |
Why use React useState
- Simplicity- With useState, there is no need to use class components or this.syntax syntax. State variables can be declared directly within the component function with functional components.
- Readability- Using useState makes the functional components more concise and easier to understand. State-related logic is encapsulated within the component function, making it clear where state is defined and modified.
- Ease of Use- The syntax for useState is straightforward. We just need to declare a state variable and a corresponding setter function, both of which are used within the component to read and update state values.
- Encapsulation- Each call to useState creates a separate instance of state that allows components to manage multiple independent state variables. This helps in the encapsulation and separation of concerns within the component.
- Compatibility with Functional Components- Since useState is a hook designed specifically for functional components, it aligns well with modern React development practices.
Updating Objects and Arrays in State using React useState
Updating Objects
import React, { useState } from 'react';
function Example() {
const [user, setUser] = useState({ name: 'John', age: 30 });
// Update user's age
const updateUserAge = () => {
setUser(prevUser => ({
...prevUser,
age: prevUser.age + 1
}));
};
return (
<div>
<p>Name: {user.name}</p>
<p>Age: {user.age}</p>
<button onClick={updateUserAge}>Increase Age</button>
</div>
);
}
export default Example;
Updating Arrays
import React, { useState } from 'react';
function Example() {
const \[items, setItems\] = useState(\['apple', 'banana', 'cherry'\]);
// Add a new item
const addItem = () => {
setItems(prevItems => \[...prevItems, 'orange'\]);
};
// Remove an item
const removeItem = (itemToRemove) => {
setItems(prevItems => prevItems.filter(item => item !== itemToRemove));
};
return (
<div>
<ul>
{items.map((item, index) => (
<li key\={index}>
{item}
<button onClick\={() => removeItem(item)}>Remove</button>
</li>
))}
</ul>
<button onClick\={addItem}>Add Item</button>
</div>
);
}
export default Example;
Read More: |
Summary
FAQs
Take our React skill challenge to evaluate yourself!
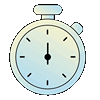
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.