Access Modifiers in TypeScript
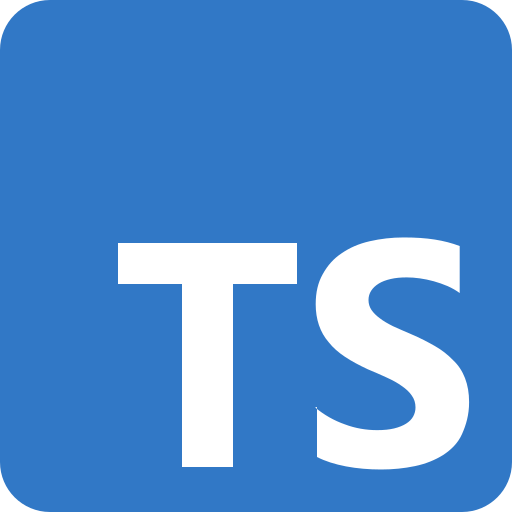
TypeScript Programming Course
Access Modifiers in TypeScript: An Overview
TypeScript access modifiers are keywords that affect the visibility of class members such as properties and methods. They decide who has access to these members and where they can get it. This Typescript tutorial helps with encapsulation, an essential concept of object-oriented programming that promotes modularity and code security.Types of Access Modifiers in Typescript
There are 3 Types of Access Modifiers in Typescript:
- Public
- Private
- Protected
1. Public Access Modifier in Typescript
The public access modifier allows members of a class to be accessed from anywhere in the program, both inside and outside the class. It is the default access modifier for TypeScript class members, so you don't need to explicitly declare it unless you want to emphasize accessibility.Example of Public Access Modifier in Typescript
class Person {
public name: string; // Accessible anywhere in the program
constructor(name: string) {
this.name = name;
}
}
const myPerson = new Person("Alice");
console.log(myPerson.name); // Output: Alice
In the Person class, the name is declared public, making it available outside of the class. Using dot notation, we may get the name of a Person object (myPerson).2. Private Access Modifier in Typescript
The private access modifier limits a class member's visibility to the class itself. It promotes encapsulation and data protection by preventing direct access from outside the class.Example of Private Access Modifier in Typescript
class InventoryItem { private quantity: number; // Only accessible within InventoryItem
constructor(quantity: number) {
this.quantity = quantity;
}
public decreaseQuantity(amount: number) {
if (amount <= this.quantity) {
this.quantity -= amount; // Can access private member inside method
} else {
throw new Error("Insufficient quantity");
}
}
}
InventoryItem declares quantity as private, preventing direct access from the outside. The public decreaseQuantity method can change the quantity while verifying its value (accessing quantity).3. Protected Access Modifier in Typescript
Members can be accessed within the class and by subclasses, but not directly from outside the class hierarchy, thanks to the protected access modifier. It encourages inheritance as well as regulated access to shared functions.Example of Protected Access Modifier in Typescript
class User { protected age: number; // Accessible in User and subclasses
constructor(age: number) {
this.age = age;
}
}
class Employee extends User {
public getRetirementAge(): number {
return this.age + 65; // Access protected member from subclass
}
}
const newUser = new User(25);
// newUser.age is NOT accessible (protected)
const newEmployee = new Employee(30);
console.log(newEmployee.getRetirementAge()); // Output: 95
Age is protected in User, enabling access within User and its subclass Employee.Employee's getRetirementAge method calculates retirement age using age (protected).Readonly Access Modifier in Typescript
After initialization, the readonly access modifier marks a property as immutable, enabling it to be read but not directly updated. This improves data security and makes state management easier.Example of Readonly Access Modifier in Typescript
class User { readonly id: string; // Can be read but not assigned to later
constructor(id: string) {
this.id = id;
}
// Only methods can modify internal state (if needed)
changeUsername(newUsername: string) {
// Simulate internal state change (avoid direct assignment)
// ...
}
}
const user = new User("abc123");
console.log(user.id); // Output: abc123
// user.id = "xyz456"; // Error: Cannot assign to 'id' because it is readonly
user.changeUsername("new_username"); // Updating state through internal logic
The id property in User is marked as readonly, which prevents direct assignment after initialization within the constructor. The changeUsername method shows how internal logic can adjust the state without modifying the id directly.Summary
Access modifiers in TypeScript regulate member visibility, increasing encapsulation & code security. Public members are available everywhere, private members are only accessible internally, protected members extend to subclasses, and readonly members prevent direct updates. Choosing the appropriate modifier for each member guarantees that the code is organized, secure, and modular.FAQs
Q1. What access modifiers does TypeScript support?
Q2. What are the TypeScript method modifiers?
Q3. What is the difference between public and private in TypeScript?
Q4. What is the TypeScript default access modifier?
Q5. What is the distinction between protected and readonly in TypeScript?
Take our free typescript skill challenge to evaluate your skill
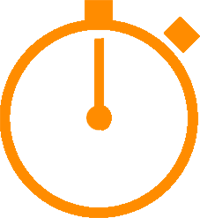
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.