02
MayUnderstanding Collections and Collections Interfaces
Collections in C#
C# Collections are important for developers seeking to manage groups of related objects efficiently. Imagine having the capability to store, retrieve, and manipulate data in various formats, such as lists, dictionaries, or queues, allowing for more organized and flexible code. Collections provide a powerful way to work with groups of objects, offering built-in methods for searching, sorting, and modifying data without the need for additional structures.
In this C# tutorial, I’ll explain the different types of Collections and Collection Interfaces in C#, how they streamline data management by providing versatile data structures, and why mastering them is crucial for writing effective, scalable C# applications.
To deepen your understanding and improve your skills, you can enroll in a C Sharp Online Course Free and start learning today!
What is Collections?
- Collections in C# are unique containers that allow you to store and manage groups of linked items.
- Collections, unlike arrays, are flexible, allowing you to simply add or remove items without regard for a fixed size.
- Collections can be used with any type of data, including numbers, texts, and complex objects.
- C# has several forms of collections, including lists, dictionaries, and queues, each with a distinct function.
For example, a list is ideal for storing items in order, whereas a dictionary allows you to seek up objects using keys. Collections make it easier to manage large amounts of data, allowing you to write cleaner and more efficient code.
Types of Collections
.NET supports two main types of collections: generic and non-generic. Before .NET 2.0, only non-generic collections were available. With the introduction of generics, you now have more options to choose from.
Here’s a quick comparison of some common non-generic and generic collections:
Non-generic | Generic |
ArrayList | List |
HashTable | Dictionary |
SortedList | SortedList |
Stack | Stack |
Queue | Queue |
Read More - C Sharp Interview Questions
Example of Collections:
using System;
using System.Collections;
class Scholarhat {
public static void Main()
{
Queue CourseQueue = new Queue();
CourseQueue.Enqueue("C#");
CourseQueue.Enqueue("Java");
CourseQueue.Enqueue("SQL");
CourseQueue.Enqueue("Python");
CourseQueue.Enqueue("C");
Console.Write("Total number of courses present in the Queue are: ");
Console.WriteLine(CourseQueue.Count);
// Displaying the beginning element of Queue
Console.WriteLine("Course: " + CourseQueue.Peek());
}
}
Output
Total number of courses present in the Queue are: 5
Course: C#
Explanation
In the above program, we illustrate nongeneric collections using a queue. First, we created a queue named "CourseQueue".Then We Inserted the elements into the Queue. Then, we tried to display the count of elements along with the starting course.1. Non-Generic Collections
In non-generic collections, you can store items of different types. These collections are flexible, allowing you to add or remove items at runtime. Here are some commonly used non-generic collections:
1. C# ArrayList
The ArrayList class is a collection that can hold any type of object. It behaves like an array but doesn't have a fixed size. You can add as many items as you need.
Example
using System;
using System.Collections;
public class Program
{
public static void Main()
{
ArrayList al = new ArrayList();
al.Add("Kiran Teja Jallepalli");
al.Add(7);
al.Add(DateTime.Parse("8-Oct-1985"));
foreach (object o in al)
{
Console.WriteLine(o);
}
}
}
Output
Kiran Teja Jallepalli
7
10/8/1985 12:00:00 AM
Explanation
- In this code, an ArrayList is formed to contain items of various types (strings, integers, and DateTime values).
- The Add function is used to add these values to the ArrayList.
- The foreach loop iterates through the collection and prints each element to the terminal.
- This demonstrates how ArrayList can handle heterogeneous data.
2. C# HashTable
The HashTable class stores items in key-value pairs, making it easy to retrieve values using their corresponding keys.
Example
using System;
using System.Collections;
public class Program
{
public static void Main()
{
Hashtable ht = new Hashtable();
ht.Add("ora", "oracle");
ht.Add("vb", "vb.net");
ht.Add("cs", "cs.net");
ht.Add("asp", "asp.net");
foreach (DictionaryEntry d in ht)
{
Console.WriteLine(d.Key + " " + d.Value);
}
}
}
Output
vb vb.net
asp asp.net
cs cs.net
ora oracle
Explanation
- In this code, a Hashtable is used to hold key-value pairs, with each key mapping to a corresponding value (for example, "ora" to "oracle").
- The Add method adds key-value pairs to the Hashtable.
- A foreach loop iterates across the Hashtable, printing both the keys and their associated values to the terminal.
- This example shows how to utilize a Hashtable to manage key-value data efficiently.
3. C# SortedList
The SortedList class combines features of both ArrayList and HashTable, storing items as key-value pairs in a sorted order.
Example
using System;
using System.Collections;
public class Program
{
public static void Main()
{
SortedList sl = new SortedList();
sl.Add("ora", "oracle");
sl.Add("vb", "vb.net");
sl.Add("cs", "cs.net");
sl.Add("asp", "asp.net");
foreach (DictionaryEntry d in sl)
{
Console.WriteLine(d.Key + " " + d.Value);
}
}
}
Output
asp asp.net
cs cs.net
ora oracle
vb vb.net
Explanation
- In this code, a SortedList is used to store key-value pairs, with the keys automatically sorted ascending.
- The Add method adds key-value pairs to the SortedList.
- A foreach loop iterates through the sorted key-value pairs, printing each key and its corresponding value to the console.
- This example shows how a SortedList keeps a sorted collection of key-value pairs for effective data management.
4. C# Stack
A Stack follows the Last In, First Out (LIFO) principle, where the last item added is the first one to be removed.
Example
using System;
using System.Collections;
public class Program
{
public static void Main()
{
Stack stk = new Stack();
stk.Push("cs.net");
stk.Push("vb.net");
stk.Push("asp.net");
stk.Push("sqlserver");
foreach (object o in stk)
{
Console.WriteLine(o);
}
}
}
Output
sqlserver
asp.net
vb.net
cs.net
Explanation
- In this code, a stack collection is used to store elements in Last-In-First-Out (LIFO) order.
- The Push method places things at the top of the stack.
- Using a foreach loop, the stack's elements are iterated and written to the console.
- The stack stores and displays the items in the reverse order that they were added, exhibiting the Stack collection's LIFO behavior.
5. C# Queue
A Queue works on the First In, First Out (FIFO) principle, meaning the first item added is the first one to be removed.
Example
using System;
using System.Collections;
public class Program
{
public static void Main()
{
Queue q = new Queue();
q.Enqueue("cs.net");
q.Enqueue("vb.net");
q.Enqueue("asp.net");
q.Enqueue("sqlserver");
foreach (object o in q)
{
Console.WriteLine(o);
}
}
}
Output
cs.net
vb.net
asp.net
sqlserver
Explanation
- In this code, entries are stored in a Queue collection in First-In-First-Out (FIFO) order.
- The Enqueue method moves elements to the end of the queue.
- Using a foreach loop, the queue's elements are iterated and reported to the console.
- The queue shows the items in the same order they were added, demonstrating the FIFO characteristic of the Queue collection.
2. Generic Collections
Generic collections are more type-safe than non-generic collections. You specify the type of items you want to store, which helps prevent runtime errors. Here are some commonly used generic collections:
1. C# List
The List class is a flexible array that can store a specific type of item.
Example
using System;
using System.Collections.Generic;
public class Program
{
public static void Main()
{
List lst = new List();
lst.Add(100);
lst.Add(200);
lst.Add(300);
lst.Add(400);
foreach (int i in lst)
{
Console.WriteLine(i);
}
}
}
Explanation
- This code creates a list to store integers.
- The Add method adds four integer numbers to the list (100, 200, 300, and 400).
- The foreach loop iterates through the list and prints each value to the console.
- This shows how a generic List<int> is used in C# to store and access integers.
2. C# Dictionary
The Dictionary class is a collection of key-value pairs where both the key and the value are strongly typed.
Example
using System;
using System.Collections.Generic;
public class Program
{
public static void Main()
{
Dictionary dct = new Dictionary();
dct.Add(1, "cs.net");
dct.Add(2, "vb.net");
dct.Add(3, "asp.net");
dct.Add(4, "sqlserver");
foreach (KeyValuePair kvp in dct)
{
Console.WriteLine(kvp.Key + " " + kvp.Value);
}
}
}
Output
1 cs.net
2 vb.net
3 asp.net
4 sqlserver
Explanation
- In this code, key-value pairs are stored in a dictionary.
- The Add method adds entries using integer keys and string values.
- The foreach loop traverses the dictionary using KeyValuePair to retrieve both keys and values.
- Each key-value pair is written to the console, demonstrating how dictionaries assign keys to associated values.
3. C# SortedList
You can also use a generic SortedList, which stores items in sorted order as key-value pairs.
Example
using System;
using System.Collections.Generic;
public class Program
{
public static void Main()
{
SortedList sl = new SortedList();
sl.Add("ora", "oracle");
sl.Add("vb", "vb.net");
sl.Add("cs", "cs.net");
sl.Add("asp", "asp.net");
foreach (KeyValuePair kvp in sl)
{
Console.WriteLine(kvp.Key + " " + kvp.Value);
}
}
}
Output
asp asp.net
cs cs.net
ora oracle
vb vb.net
Explanation
- This code creates a SortedList to store key-value pairs in which both the keys and values are strings.
- The Add method is used to create entries, and the keys are sorted automatically.
- The foreach loop iterates across the list, accessing and printing each key-value pair using KeyValuePair.
- This demonstrates how a SortedList sorts entries based on their keys.
4. C# Stack
You can also use a generic Stack, which is type-safe.
Example
using System;
using System.Collections.Generic;
public class Program
{
public static void Main()
{
Stack stk = new Stack();
stk.Push("cs.net");
stk.Push("vb.net");
stk.Push("asp.net");
stk.Push("sqlserver");
foreach (string s in stk)
{
Console.WriteLine(s);
}
}
}
Output
sqlserver
asp.net
vb.net
cs.net
Explanation
- In this code, string elements are stored in a Stack collection using the Push method, which moves elements to the top of the stack.
- The foreach loop cycles around the stack, obtaining and printing each element in the last-in, first-out (LIFO) order.
- This example shows basic stack operations and iteration in C#.
5. C# Queue
Similarly, you can use a generic Queue.
Example
using System;
using System.Collections.Generic;
public class Program
{
public static void Main()
{
Queue q = new Queue();
q.Enqueue("cs.net");
q.Enqueue("vb.net");
q.Enqueue("asp.net");
q.Enqueue("sqlserver");
foreach (string s in q)
{
Console.WriteLine(s);
}
}
}
Output
cs.net
vb.net
asp.net
sqlserver
Explanation
- In this code, a Queue collection is used to store string elements, with the Enqueue method adding elements to the end of the queue.
- The foreach loop cycles through the queue, obtaining and printing each element in the first-in, first-out (FIFO) manner.
- This example shows fundamental queue operations, including iteration in C#.
What are Collection Interfaces?
All of the collection types use some common interfaces. These common interfaces define the basic functionality for each collection class. The key collections interfaces are : IEnumerable, ICollection, IDictionary, and IList.
IEnumerable acts as a base interface for all the collection types that is extended by ICollection. ICollection is further extended by IDictionary and IList.
All collections interfaces are not implemented by all the collections. It depends on the collection's nature.
The IEnumerable Interface: Syntax
public IEnumerator GetEnumerator( )
{
return (IEnumerator) new ListBoxEnumerator(this);
}
- Here, The Enumerator must implement the IEnumerator methods and properties.
- These can be implemented either directly by the container class or by a separate class.
- The latter approach is preferred because it encapsulates this responsibility in the Enumerator class.
The ICollectionInterface: Syntax
ICollection names = new List();
names.Add("Pragati");
names.Remove("surbhi");
Console.WriteLine(names.Count); // Outputs the count of items
The IDictionary: Syntax
IDictionary ages = new();
ages["Pragati"] = 18;
ages.Add("surbhi", 17);
Console.WriteLine(ages["Pragati"]); // Access value by key
The IList: Syntax
IList numbers = new List { 1, 2, 3,4,5 };
numbers[0] = 10; // Access and modify elements by index
Console.WriteLine(numbers.IndexOf(3)); // Outputs the index of '5'
Summary
FAQs
Take our Csharp skill challenge to evaluate yourself!
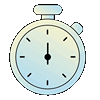
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.