Exploring ExpressJS Routing
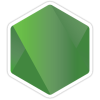
Node.js Course
In this article we are going to explore routing in ExpressJS. Alright then, let’s get started working with ExpressJS Route. Let's create a sample application using Express-generator. In case you need help, please follow our previous article about Getting started with ExpressJS and Express-generator.
What is Routing?
Routing is a way to manage the application URIs. Routing helps us to respond to client requests. When you make a request it handles by the express route middleware. If route found it further process the request else returns 404 error.
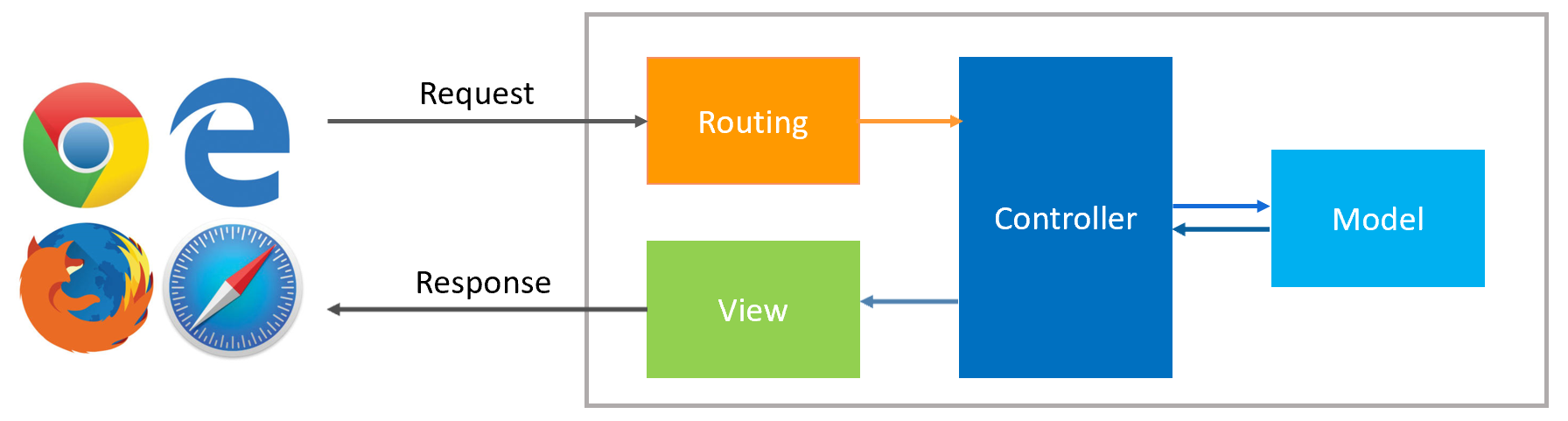
For better understanding routing in terms of the client request, let’s run our newly created sample express application in visual code IDE.
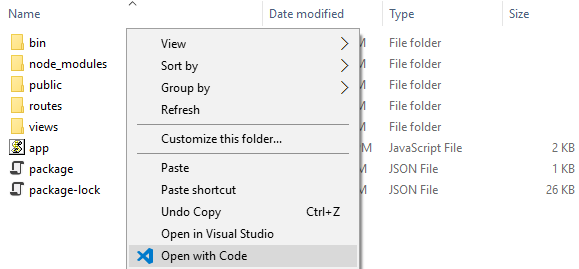
Read More - Advanced Node JS Interview Questions and Answers
If visual code isn’t installed on your machine, please download it by following the link:https://code.visualstudio.com
Let’s open app.js file there’s two predefined route in our generated sample application which is configured to match the URL when client request with a particular URL’s. Following code snippet is to include the exported module by “require()” function.
var indexRouter = require('./routes/index'); var usersRouter = require('./routes/users');
Then initiate the module by using “app.use()” function in below code snippet.
app.use('/', indexRouter); app.use('/users', usersRouter);
Let’s open “indexRouter” file to know how the server is responding when we browse the root path of our application.
var express = require('express'); var router = express.Router(); /* GET home page. */ router.get('/', function(req, res, next) { res.render('index', { title: 'Express' }); }); module.exports = router;
As we can see the very first line of code is including the Express module by “require()” function with creating the object “express” of Express module. Then the next line is to create an instance of “express.Router()” to implement the HTTP router methods like “get, put, post, delete”. Next portion is the callback function which gets called by client request. In response, a compiled view is served by the server to the client browser. Finally, the module export router object to include in another module. In “usersRouter” the response process is same but the method is different, as we can see from below module “res.send()” function is used to send different types of response for client.
var express = require('express'); var router = express.Router(); /* GET users listing. */ router.get('/', function(req, res, next) { res.send('respond with a resource'); }); module.exports = router;
Later in this post we will learn more about request and response object in HTTP method callback function. Let’s create a new route “example” in our sample application by adding a new example.js file in routes folder using visual code like below screen.
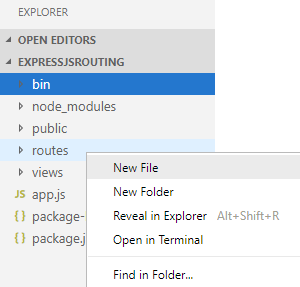
Open “app.js” to include the newly create file like following line
var exampleRouter = require('./routes/example');
Then initiate the imported module by app.use() function.
app.use('/example', exampleRouter);
Finally copy-paste following code to example.js file.
var express = require('express'); var router = express.Router(); //HTTP Methods /* Get */ router.get('/', function(req, res) { res.send('Test Response!!'); }); module.exports = router;
Then go to terminal in visual code with “ctrl+`” shortcut in keyboard then type “npm start” to run the application. We are going to test application using postman, if not exist add it to chrome by following link: https://chrome.google.com/webstore/detail/postman/fhbjgbiflinjbdggehcddcbncdddomop?hl=en
After installation click launch app to run the application then browse with: http://localhost:3000/example
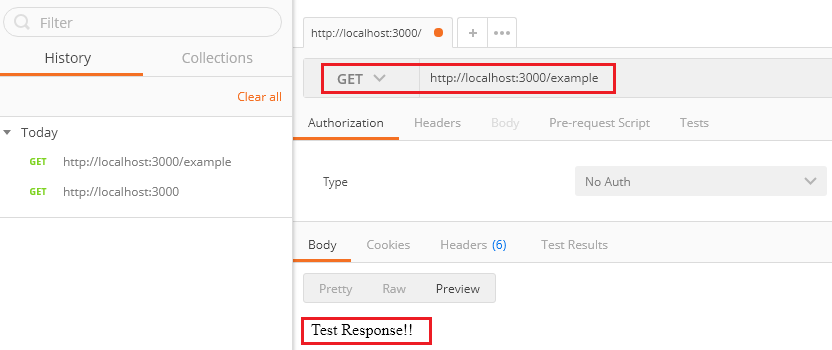
As we can see the server responded with message “Test Response”, at the same time here’s the server response in below screen.
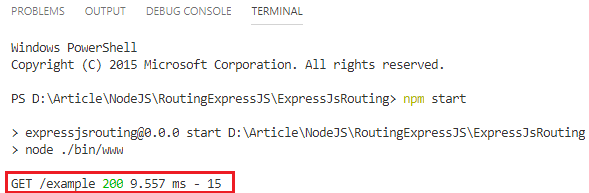
Well, so far we have overview the basic route module, also learned how to add a new route to our sample application, now let’s explore the depth of routing by covering below listed topics
- Route Object
- Route Methods
- Route Paths
- Route Parameters
- Router Route
- Route Handlers
Route Object
Basically router object is an instance of built-in middleware “express.Router()” function in Express which can behave differently like middleware and routes. Following are two different behavior of router object where the middleware function will always get called on any HTTP method call.
//Middleware router.use(); //HTTP Methods router.get();
Finally updated module after adding middleware to example.js file.
var express = require('express'); var router = express.Router(); // Router level Middleware router.use(function(req, res, next) { console.log('Middleware call'); next(); }); //HTTP Methods /* Get */ router.get('/', function(req, res) { res.send('Test Response!!'); }); module.exports = router;
Copy-paste above code to example.js to see the results. Restart the node server to re-initiate the modules. Then browse with URL http://localhost:3000/example
to see the middleware get called each time while any HTTP method is called like below screen.
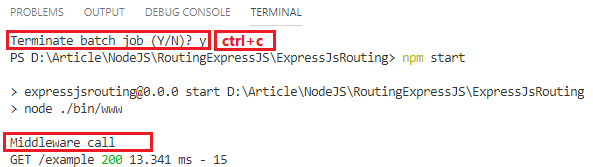
Next, let’s get familiar with different types of router methods that handle different route path.
Route Methods
In express app router object methods implement the routing mechanism, below is the list of most popular routing methods to overview
head() : HTTP get data without the response body
get() : HTTP get data
put() : HTTP put requests for updating existing data on submitting form
delete() : HTTP delete request
For more details please follow the link: https://expressjs.com/en/4x/api.html#app.METHOD
router.method(“path”, handlerfunction(req, res))
Here “router” is the route object of “express.Router()” and then the method call like “router.get()”. The defined method has two arguments, one is “path” and another one is “handlersfunction”. Single route can have multiple handler function, later we are going to discuss on it. Following example is for HTTP request methods like Get, Post, Put, and Delete.
ExampleThe following method “route.all()” isn’t a HTTP method, which will get called if any of the HTTP method is requested by client.
//any route router.all('/*', function (req, res, next) { console.log('all'); next(); });HTTP Methods
GET() method is basically data or resource getting request which is served by server on particular request with status code of 200, which mean “OK”. It’ll response with 404 status code if the request is invalid. Here’s the list of starting number meaning of status code.
1×× Informational
2×× Success
3×× Redirection
4×× Client Error
5×× Server Error
Learn more on status code by following link: https://httpstatuses.com
//HTTP Methods /* Get */ router.get('/', function(req, res) { res.send('Test Response!!'); });
POST() method is used to send data from client to server which also response with a status code of 200. If request is invalid, status code 404 is responded like GET() method.
/* Post */ router.post('/', function(req, res) { res.send('Operation Post'); });
PUT() method is used to update existing data of single entity in server, finally response with a status code of 200. If request is invalid, status code 404 is responded like GET(), POST() method.
/* Put */ router.put('/', function(req, res) { res.send('Operation Put'); });
DELETE() method is used to delete data from server, it has also response status code of 200. If request is invalid, status code 404 is responded like other HTTP method.
/* Delete */ router.delete('/', function(req, res) { res.send('Operation Delete'); });
Here you may noticed that different methods are working with the same URL “/”, which is different HTTP verbs request that is identified by request Uniform Resource Identifier (URI) make each request unique. In callback function from above methods as we can see there’s two parameters, the first parameter is HTTP request object (req) which supports passing values through
Query string
Request parameters
Request body
HTTP headers
Then the second one is response object (res) for sending response to corresponding request. Here’s the list of response method
res.end() : End the response process.
res.json() : Send a JSON response.
res.jsonp(): Send a JSON response with JSONP support.
res.redirect() : Redirect a request
res.render() : Render a view template
res.send() : Send a response of various types
res.sendStatus() : Set the response status code and send its string representation as the response body.
Learn more on response method by following link: http://expressjs.com/en/guide/routing.html#response-methods
Route Path
There are two type of route path in Express
- String based
? (optional character)
+ (repeat character)
* (random character)
() (grouping character)
- Regular expression
/ (enclosed with slashes)
Below examples are shown with both type of route with accepting different patterns.
String Based Use of optional (?) characterrouter.get('/path/sh?ashangka', function(req, res) { res.send('here h is optional by h?'); });
accept
/example/path/sashangka
/example/path/Shashangka
router.get('/path/shashangka+', function(req, res) { res.send('here is repeating by a+'); });
accept
/example/path/shashangka
/example/path/shashangkaa
accept
/example/path/shasangka
/example/path/shasaangka
router.get('/path/sha(sha)?ngkas', function(req, res) { res.send('here () is for grouping, ? is for optional'); }); router.get('/path/sha(sha)?ngka+s', function(req, res) { res.send('here () is for grouping, ? is for optional, + is for repeating'); });
accept
/example/path/shangkas
/example/path/shashangkas
/example/path/shangkaaaaaas
/example/path/shashangkaaaaaas
Regular Expression
router.get(/^\/path\/sha(sha|sa)ngkar$/, function(req, res) { res.send('match shasangkar, shashangkar'); });
As you may noticed in regular expression is enclosed with slashes like /regex/ not with double quotes.
accept
/example/path/shasangkar
/example/path/shashangkar
Route Parameters
Basically route parameters is nothing but passing information in a particular location with the URL path like following example.
// GET: /example/profile/shashangka router.get('/profile/:id', function (req, res) { res.send(req.params); console.log('response with profile: ' + req.params.id); });
We can pass more than one parameter like below method.
// GET: /example/profile/shashangka/age/34 router.get('/profile/:id/age/:age(\\d+)', function (req, res) { res.send(req.params); console.log('response with profile: ' + req.params.id + ' age:' + req.params.age); }); // GET: /example/profile/shashangka/34 router.get('/profile/:id/:age(\\d+)', function (req, res) { res.send(req.params); console.log('response with profile: ' + req.params.id + ' age:' + req.params.age); }); // GET: /example/age/34-50 router.get('/age/:from(\\d+)-:to(\\d+)', function (req, res) { res.send(req.params); console.log('response with age: ' + req.params.from + ' to ' + req.params.to); }); // GET: /example/profilename/shashangka.shekhar router.get('/profilename/:firstname.:lastname', function (req, res) { res.send(req.params); console.log('response with profilename: ' + req.params.firstname + ' ' + req.params.lastname); });
Now we know how to pass value through url, also learned how to access the values by using of “req.params” object.
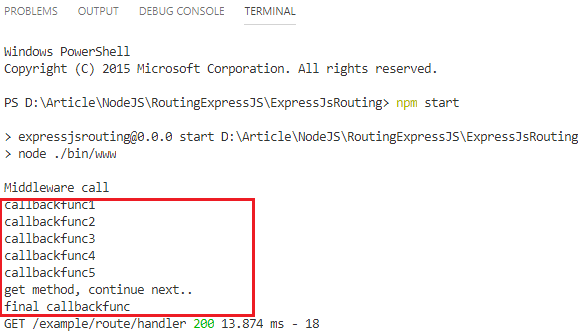
Router Route
In route method section we have seen different HTTP methods implemented with repeating same route path like “router.get()”, “router.post()”, “router.putt()”, “router.delete()”, which is bad and also may cause type error. By using “router.route()” function we can avoid those like following example.
router.route('/data') .get(function (req, res) { res.send('Operation Get'); }) .post(function (req, res) { res.send('Operation Post'); }) .put(function (req, res) { res.send('Operation Put'); }) .delete(function (req, res) { res.send('Operation Delete'); });
Route Handlers
If we want to execute multiple callback function with a single request we can use array of call back function like below example. The only thing we must consider carefully is to use the “next()“ function to continue with the next callback function.
Definitionrouter.method(“path”, [arrayofhandlerfunction])Example
var callbackfunc1 = function (req, res, next) { console.log('callbackfunc1'); next(); }; var callbackfunc2 = function (req, res, next) { console.log('callbackfunc2'); next(); }; var callbackfunc3 = function (req, res, next) { console.log('callbackfunc3'); next(); }; var callbackfunc4 = function (req, res, next) { console.log('callbackfunc4'); next(); }; var callbackfunc5 = function (req, res, next) { console.log('callbackfunc5'); next(); }; router.get('/data/handler', [callbackfunc1, callbackfunc2, callbackfunc3, callbackfunc4, callbackfunc5], function (req, res, next) { console.log('get method, continue next..') next() }, function (req, res) { console.log('final callbackfunc') res.send('final callbackfunc') });
As we can see from below screen how the final callback function is executed after processing array of callback function one by one.
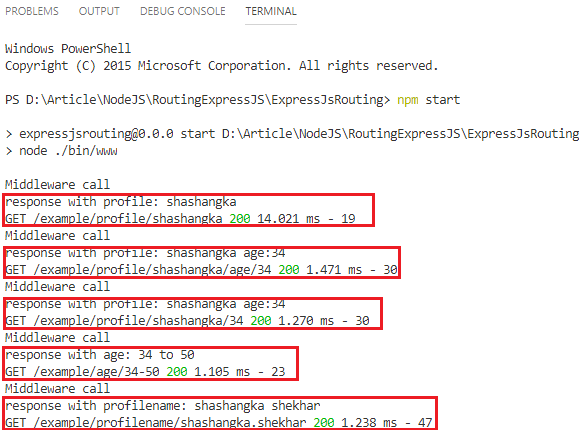
Summary
In final words, time to get back those points which we have learned. Here’s the listed recapping points
Now we know how to add new route with the knowledge of basic routing mechanism
Learned about creating route object, methods
Knowledge about HTTP request, response
Passing value through URL and retrieving them using route parameters
Hope this will help to build Express application with routing from scratch.
Take our free nodejs skill challenge to evaluate your skill
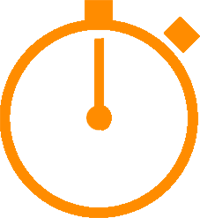
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.